第一个 spark 应用开发详解 (java 版)
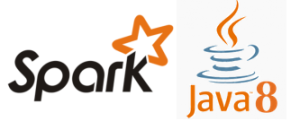
欢迎访问我的 GitHub
这里分类和汇总了欣宸的全部原创(含配套源码):https://github.com/zq2599/blog_demos
WordCount 是大数据学习最好的入门 demo,今天就一起开发 java 版本的 WordCount,然后提交到 Spark2.3.2 环境运行;
版本信息
操作系统:CentOS7;
JDK:1.8.0_191;
Spark:2.3.3;
Scala:2.11.12;
Hadoop:2.7.7;
Maven:3.5.0;
关于 hadoop 环境
本次实战用到了 hadoop 的 hdfs,关于 hadoop 的部署,请参考《Linux部署hadoop2.7.7集群》;
关于 spark 环境
本次实战用到了 spark2.3.3,关于 spark 集群的部署,请参考《部署spark2.2集群(standalone模式)》;请注意,由于 2.3.3 版本的 spark-core 的 jar 包不支持 scala2.12,所以在部署 spark 的时候,scala 版本请使用 2.11;
关于本次实战开发的应用
本次实战开发的应用是经典的 WorkCount,也就是指定一个文本文件,统计其中每个单词出现的次数,再取出现次数最多的 10 个,打印出来,并保存在 hdfs 文件中;
本次统计单词数用到的文本
本次用到的 txt 文件,是我在网上找到的 pdf 版本的《乱世佳人》英文版,然后导出为 txt,读者您可以自行选择适合的 txt 文件来测试;
在 hdfs 服务所在的机器上执行以下命令,创建 input 文件夹:
在 hdfs 服务所在的机器上执行以下命令,创建 output 文件夹:
把本次用到的 text 文件上传到 hdfs 服务所在的机器,再执行以下命令将文本文件上传到 hdfs 的/input 文件夹下:
源码下载
接下来详细讲述应用的编码过程,如果您不想自己写代码,也可以在 GitHub 下载完整的应用源码,地址和链接信息如下表所示:
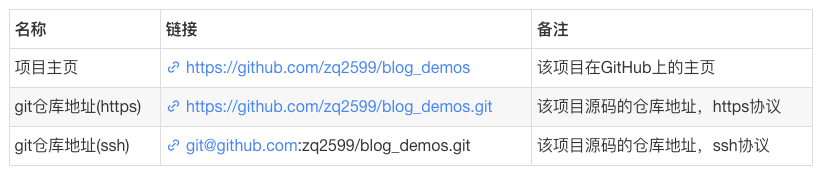
这个 git 项目中有多个文件夹,本章源码在 sparkwordcount 这个文件夹下,如下图红框所示:
开发应用
基于 maven 创建一个 java 应用 sparkwordcount,pom.xml 的内容如下:
创建 WrodCount 类,关键代码位置都有注释,就不再赘述了:
在 pom.xml 目录下执行以下命令,编译构建 jar 包:
构建成功后,在 target 目录下生成文件 sparkwordcount-1.0-SNAPSHOT.jar,上传到 spark 服务器的**~/jars/**目录下;
假设 spark 服务器的 IP 地址为 192.168.119.163,在 spark 服务器执行以下命令即可提交任务:
上述命令的最后三个参数,是 java 的 main 方法的入参,具体的使用请参照 WordCount 类的源码;
提交成功后立即开始执行任务,看到类似如下信息表示任务完成:
往前翻滚一下控制台输出的信息,如下所示,可以见到单词统计的前十名已经输出在控制台了:
在 hdfs 服务器执行查看文件的命令,可见/output 下新建了子目录 20190208213610:
查看子目录,发现里面有两个文件:
上面看到的**/output/20190208213610/part-00000**就是输出结果,用 cat 命令查看其内容:
可见与前面控制台输出的一致;
在 spark 的 web 页面,可见刚刚执行的任务信息:
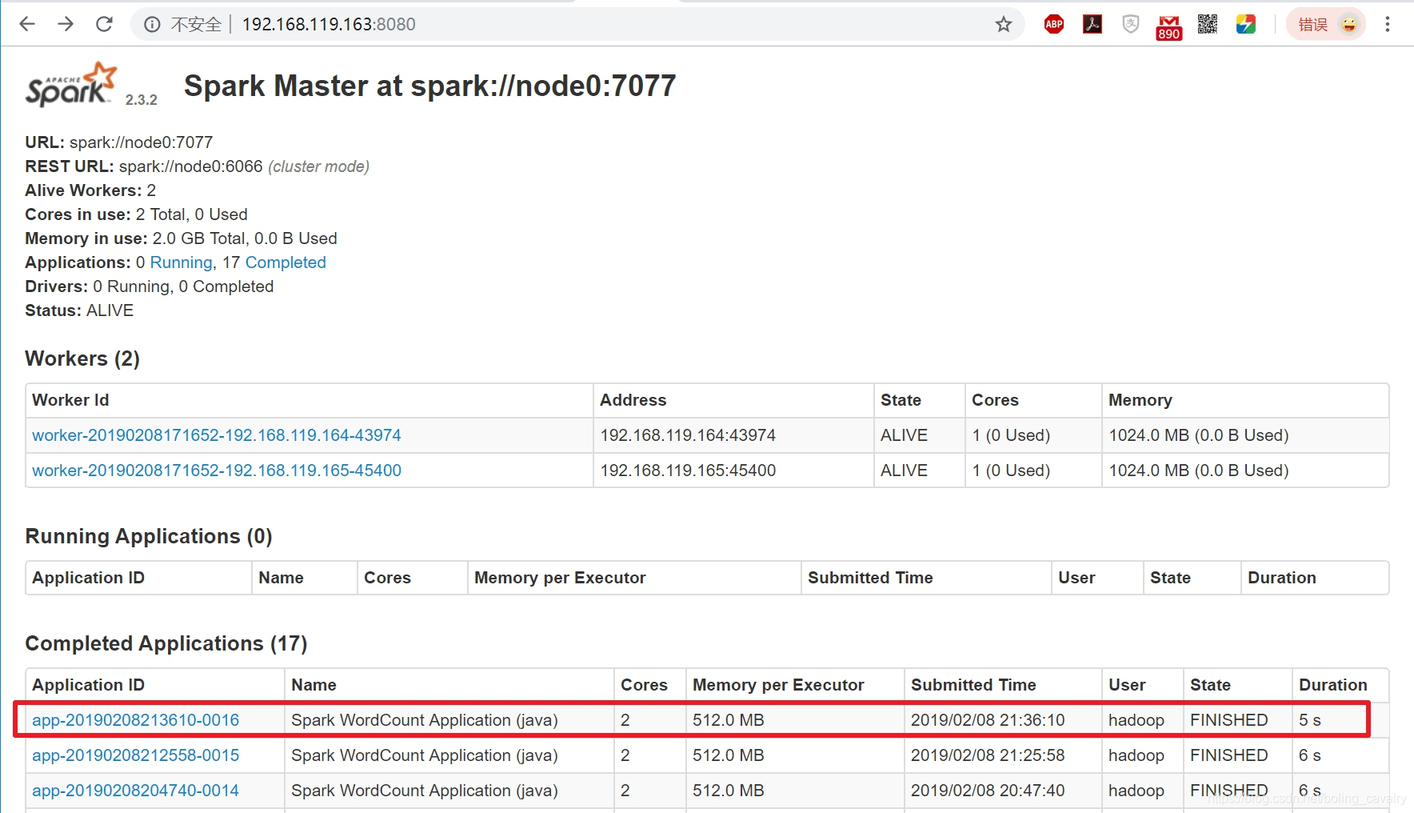
至此,第一个 spark 应用的开发和运行就完成了,接下来的文章中,咱们一起来完成更多的 spark 实战;
欢迎关注 InfoQ:程序员欣宸
版权声明: 本文为 InfoQ 作者【程序员欣宸】的原创文章。
原文链接:【http://xie.infoq.cn/article/5927a4d64accf862bcfb8ec16】。文章转载请联系作者。
评论