Java lambda 表达式用法介绍,linux 教程第四版思考题答案
输出:
Value of Pi = 3.1415
在上面的例子中,
我们已经创建了一个名为MyInterface
的功能接口。它包含一个名为getPiValue()
的抽象方法
在主类中,我们声明了对MyInterface
的引用。注意,我们可以声明接口的引用,但不能实例化接口。就是,
// it will throw an error
MyInterface ref = new myInterface();
// it is valid
MyInterface ref;
然后,我们为引用指定了一个 lambda 表达式。
ref = () -> 3.1415;
最后,我们使用引用接口调用方法getPiValue()
System.out.println("Value of Pi = " + ref.getPiValue());
[](
)带参数的 Lambda 表达式
到目前为止,我们已经创建了没有任何参数的 lambda 表达式。但是,与方法类似,lambda 表达式也可以有参数。例如[《求职面试笔试宝典》](
)
(n) -> (n%2)==0
这里,括号内的变量 n 是传递给 lambda 表达式的参数。lambda 主体接受参数并检查它是偶数还是奇数。
示例 4:使用带参数的 lambda 表达式
@FunctionalInterface
interface MyInterface {
// abstract method
String reverse(String n);
}
public class Main {
public static void main( String[] args ) {
// declare a reference to MyInterface
// assign a lambda expression to the reference
MyInterface ref = (str) -> {
String result = "";
for (int i = str.length()-1; i >= 0 ; i--)
result += str.charAt(i);
return result;
};
// call the method of the interface
System.out.println("Lambda reversed = " + ref.reverse("Lambda"));
}
}
输出:
Lambda reversed = adbmaL
##通用功能接口
到目前为止,我们使用的函数接口只接受一种类型的值。例如
@FunctionalInterface
interface MyInterface {
String reverseString(String n);
}
上面的函数接口只接受字符串并返回字符串。但是,我们可以使函数接口通用,以便接受任何数据类型。如果您不确定泛型,请访问 Java 泛型。
示例 5:通用函数接口和 Lambda 表达式
// GenericInterface.java
@FunctionalInterface
interface GenericInterface<T> {
// generic method
T func(T t);
}
// GenericLambda.java
public class Main {
public static void main( String[] args ) {
// declare a reference to GenericInterface
// the GenericInterface operates on String data
// assign a lambda expression to it
GenericInterface<String> reverse = (str) -> {
String result = "";
for (int i = str.length()-1; i >= 0 ; i--)
result += str.charAt(i);
return result;
};
System.out.println("Lambda reversed = " + reverse.func("Lambda"));
// declare another reference to GenericInterface
// the GenericInterface operates on Integer data
// assign a lambda expression to it
GenericInterface<Integer> factorial = (n) -> {
int result = 1;
for (int i = 1; i <= n; i++)
result = i * result;
return result;
};
System.out.println("factorial of 5 = " + factorial.func(5));
}
}
输出:
Lambda reversed = adbmaL
factorial of 5 = 120
在上面的示例中,我们创建了一个名为GenericInterface
的通用函数接口。它包含一个名为func()
的泛型方法。
在这里,在主类中,
GenericInterface<String>reverse-
创建对接口的引用。该接口现在对字符串类型的数据进行操作。GenericInterface<Integer>factorial-
创建对接口的引用。在本例中,接口对整型数据进行操作。
[](
)Lambda 表达式和 StreamAPI
新的java.util.stream
包已添加到 JDK8 中,它允许 java 开发人员执行搜索、筛选、映射、减少或操作列表等集合。
例如,我们有一个数据流(在本例中是一个字符串列表),其中每个字符串都是国家名称和国家地点的组合。现在,我们可以处理这些数据流,只从尼泊尔检索地方。
为此,我们可以通过 stream API 和 Lambda 表达式的组合在 stream 中执行批量操作。
示例 6:演示将 lambdas 与 stream API 一起使用
import java.util.ArrayList;
import java.util.List;
public class StreamMain {
// create an object of list using ArrayList
static List<String> places = new ArrayList<>();
// preparing our data
public static List getPlaces(){
// add places and country to the list
places.add("Nepal, Kathmandu");
places.add("Nepal, Pokhara");
places.add("India, Delhi");
places.add("USA, New York");
places.add("Africa, Nigeria");
return places;
}
public static void main( String[] args ) {
List<String> myPlaces = getPlaces();
System.out.println("Places from Nepal:");
// Filter places from Nepal
myPlaces.stream()
.filter((p) -> p.startsWith("Nepal"))
.map((p) -> p.toUpperCase())
.sorted()
.forEach((p) -> System.out.println(p));
}
}
输出:
Places from
Nepal:
NEPAL, KATHMANDU
NEPAL, POKHARA
在上面的示例中,请注意以下语句:
myPlaces.stream()
.filter((p) -> p.startsWith("Nepal"))
.map((p) -> p.toUpperCase())
.sorted()
.forEach((p) -> System.out.println(p));
这里,我们使用流API的filter()
、map()
和forEach()
等方法。这些方法可以将 lambda 表达式作为输入。
我们还可以根据上面学习的语法定义自己的表达式。这使我们能够像上面的示例中所看到的那样,大幅减少代码行。[《求职面试笔试宝典》](
)
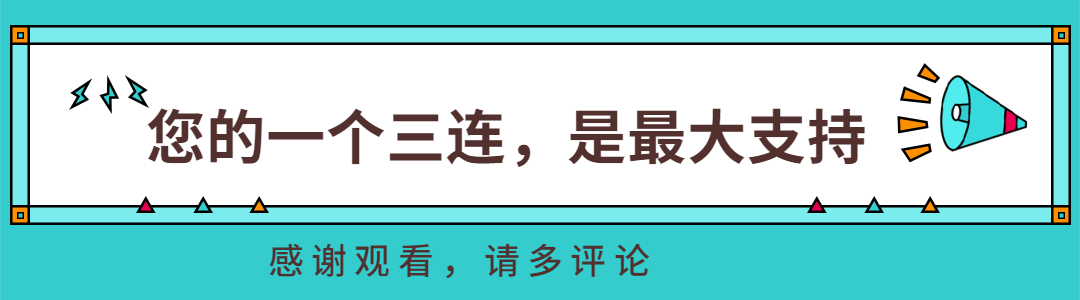
评论