Spring-- 基于 IOC 的 CRUD 操作
需求:实现账户的 CRUD 操作
技术要求:
使用 spring 的 IOC 实现对象的管理
使用 QueryRunner 作为持久层解决方案
使用 c3p0 数据源
开发流程:
创建数据库表
导入相关依赖
创建账户实体类
配置表现层与持久层
通过 XML 或注解实现 CRUD 操作
测试代码
创建相关的数据库表:
create database spring;
use spring;
Drop table if exists account;
create table account(
id int primary key auto_increment,
name varchar(40),
money float
)character set utf8 collate utf8_general_ci;
insert into account (name,money) values('aaa',1000);
insert into account (name,money) values('bbb',1000);
insert into account (name,money) values('ccc',1000);
数据库表:
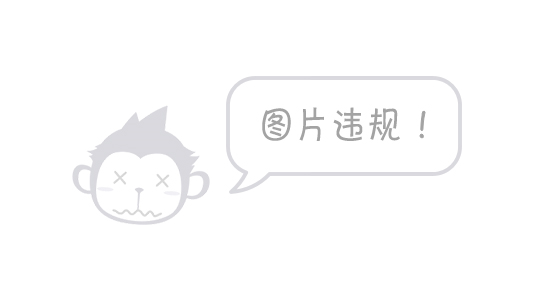
项目结构:
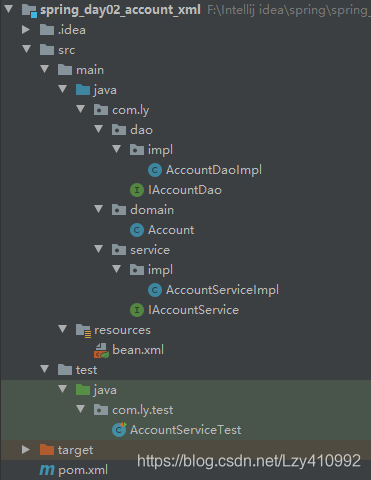
在 pom.xml 中添加相应的依赖:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>spring_day02_account_xml</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>commons-dbutils</groupId>
<artifactId>commons-dbutils</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.11</version>
</dependency>
<dependency>
<groupId>c3p0</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.1.2</version>
</dependency>
</dependencies>
</project>
创建 account 实体类:
/**
@Author: Ly
@Date: 2020-07-20 18:55
*/
public class Account implements Serializable {
private Integer id;
private String name;
private Float money;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Float getMoney() {
return money;
}
public void setMoney(Float money) {
this.money = money;
}
@Override
public String toString() {
return "Account{" +
"id=" + id +
", name='" + name + ''' +
", money=" + money +
'}';
}
}
创建并实现业务层接口:
/**
@Author: Ly
@Date: 2020-07-21 16:29
账户业务层的接口
*/
public interface IAccountService {
/**
查询所有
@return
*/
List<Account> findAllAccount();
/**
查询一个
@param accountId
@return
*/
Account findAccountById(Integer accountId);
/**
保存
@param account
*/
void saveAccount(Account account);
/**
更新
@param account
*/
void updateAccount(Account account);
/**
删除
@param accountId
*/
void deleteAccount(Integer accountId);
}
/**
@Author: Ly
@Date: 2020-07-21 16:31
账户的业务成实现类
*/
public class AccountServiceImpl implements IAccountService {
private IAccountDao accountDao;
public void setAccountDao(IAccountDao accountDao) {
this.accountDao = accountDao;
}
public List<Account> findAllAccount() {
return accountDao.findAllAccount();
}
public Account findAccountById(Integer accountId) {
return accountDao.findAccountById(accountId);
}
public void saveAccount(Account account) {
accountDao.saveAccount(account);
}
public void updateAccount(Account account) {
accountDao.updateAccount(account);
}
public void deleteAccount(Integer accountId) {
accountDao.deleteAccount(accountId);
}
}
创建并实现持久层接口:
/**
@Author: Ly
@Date: 2020-07-21 16:33
账户的持久层接口
*/
public interface IAccountDao {
/**
查询所有
@return
*/
List<Account> findAllAccount();
/**
查询一个
@param accountId
@return
*/
Account findAccountById(Integer accountId);
/**
保存
@param account
*/
void saveAccount(Account account);
/**
更新
@param account
*/
void updateAccount(Account account);
/**
删除
@param accountId
*/
void deleteAccount(Integer accountId);
}
/**
账户的持久层实现类
*/
public class AccountDaoImpl implements IAccountDao {
private QueryRunner runner;
public void setRunner(QueryRunner runner) {
this.runner = runner;
}
public List<Account> findAllAccount() {
try {
return runner.query("select * from account",new BeanListHandler<Account>(Account.class));
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public Account findAccountById(Int
eger accountId) {
try {
return runner.query("select * from account where id= ?",new BeanHandler<Account>(Account.class),accountId);
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public void saveAccount(Account account) {
try {
runner.update("insert into account(name,money) values(?,?)",account.getName(),account.getId());
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public void updateAccount(Account account) {
try {
runner.update("update account set name=?,money=? where id=?",account.getName(),account.getMoney(),account.getId());
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public void deleteAccount(Integer accountId) {
try {
runner.update("delete from account where id=?",accountId);
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
配置 bean.xml 文件:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="accountService" class="com.ly.service.impl.AccountServiceImpl">
<property name="accountDao" ref="accountDao"></property>
</bean>
<bean id="accountDao" class="com.ly.dao.impl.AccountDaoImpl">
<property name="runner" ref="runner"></property>
</bean>
<bean id="runner" class="org.apache.commons.dbutils.QueryRunner" scope="prototype">
<constructor-arg name="ds" ref="dataSource"></constructor-arg>
</bean>
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="com.mysql.cj.jdbc.Driver"></property>
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/spring?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8"></property>
<property name="user" value="root"></property>
<property name="password" value="520992"></property>
</bean>
</beans>
测试代码:
/**
@Author: Ly
@Date: 2020-07-27 17:17
*/
public class AccountServiceTest {
@Test
public void testFindAll(){
//1.获取容器
ApplicationContext ac=new ClassPathXmlApplicationContext("bean.xml");
//2.得到业务层对象
IAccountService as=ac.getBean("accountService",IAccountService.class);
//3.执行方法
List<Account> accounts=as.findAllAccount();
for(Account account:accounts){
System.out.println(account);
}
}
@Test
public void testFindOne(){
//1.获取容器
ApplicationContext ac=new ClassPathXmlApplicationContext("bean.xml");
//2.得到业务层对象
IAccountService as=ac.getBean("accountService",IAccountService.class);
//3.执行方法
Account account=as.findAccountById(1);
System.out.println(account);
}
@Test
public void testSave(){
Account account=new Account();
account.setName("sss");
account.setMoney(123f);
//1.获取容器
ApplicationContext ac=new ClassPathXmlApplicationContext("bean.xml");
//2.得到业务层对象
IAccountService as=ac.getBean("accountService",IAccountService.class);
//3.执行方法
as.saveAccount(account);
评论