Spring Boot 快速入门(一)
2. 配置启动项
创建 SpringbootTestApplication 类,作为我们的启动项 ,需要添加@SpringBootApplication
注解
//声明类是一个 SpringBoot 引导类
@SpringBootApplication
public class MySpringBootApplication {
//main 是 Java 程序的入口
public static void main(String[] args){
//run 方法 表示运行 springboot 的引导 run 参数就是 SpringBoot 引导类的字节码对象
SpringApplication.run(MySpringBootApplication.class,args);
}
}
启动测试:
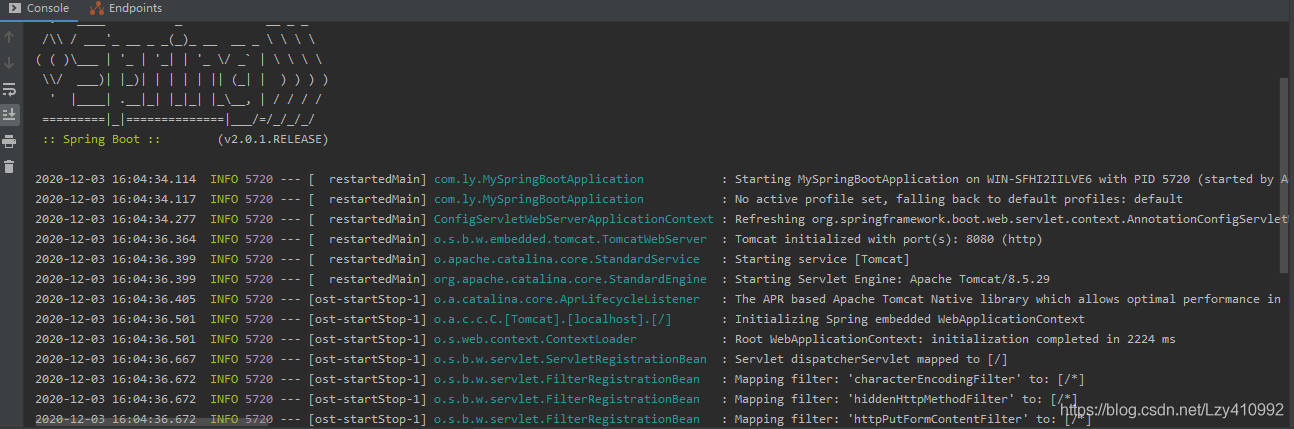
创建 Controller 测试在 web 浏览器中打开
@Controller
public class TestController {
@RequestMapping("/test")
@ResponseBody
public String quick(){
return "Hello SpringBoot";
}
}
@RestController // 使用该注解后不需要再使用 @ResponseBody 注解
public class TestController {
@RequestMapping("/test")
public String quick(){
return "Hello SpringBoot";
}
}
在页面访问 http://localhost:8080/test ,访问成功!
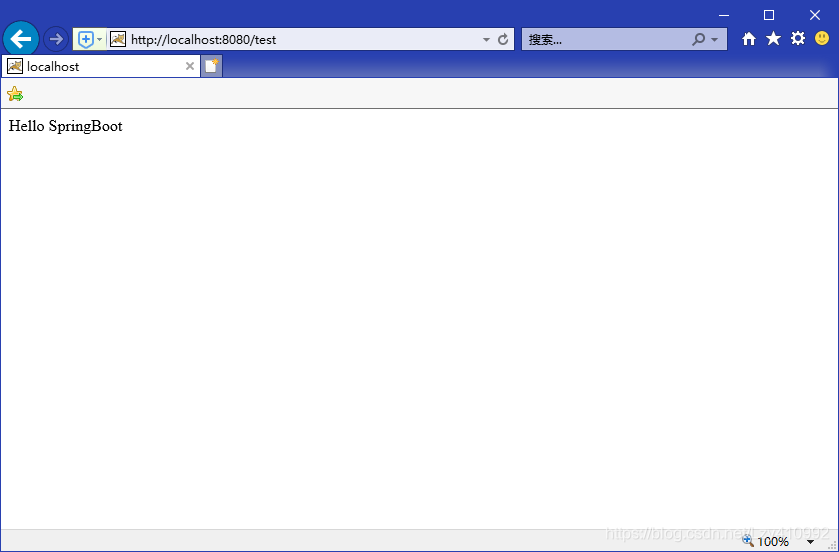
SpringBoot 工程的热部署:
在我们开发过程中会频繁修改类或页面中的信息,而每次修改过后都要重新启动才能生效,非常麻烦,通过热部署的方式,可以让我们在修改过代码之后不需要重启就能生效,相关配置如下:
<!--@ConfiguaritionProperties 的执
行器配置:根据配置文件时,给出对应提示-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
在配置过后,还需要对 IDEA 进行自动编译设置:Settings->Build->Compiler,勾选Build project automatically
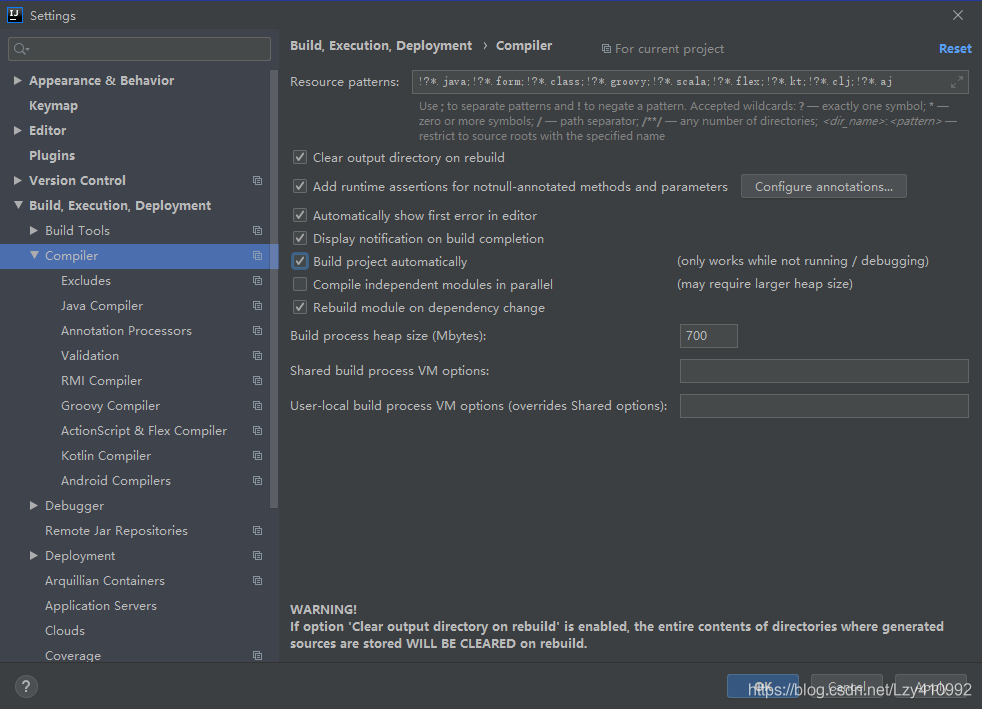
使用快捷键Shift+Ctrl+Alt+/
,选择Registry...
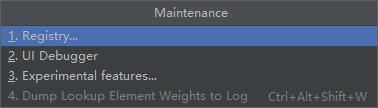
勾选compiler.automake.allow.when.app.running
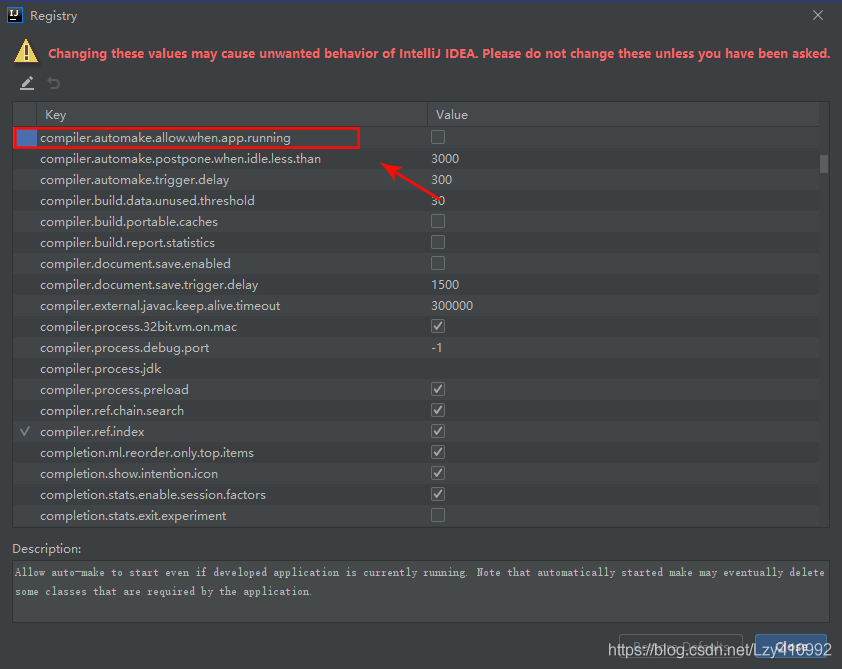
使用 IDEA 快速搭建 SpringBoot 工程:
1. 新建工程,选择Spring Initializr
,这里可以选择默认的 URL: https://start.spring.io,如果构建过程中出现问题可以选择阿里云的 URL:https://start.aliyun.com/
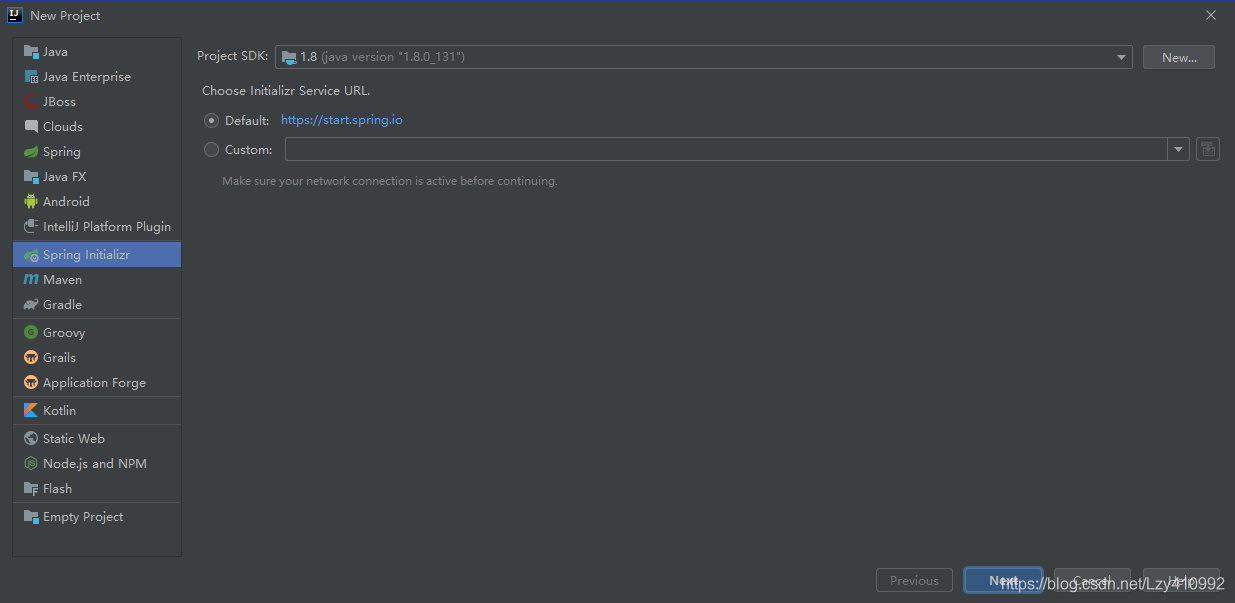
2.创建项目,注意这里 Artifact 名称只能是全大写或全小写
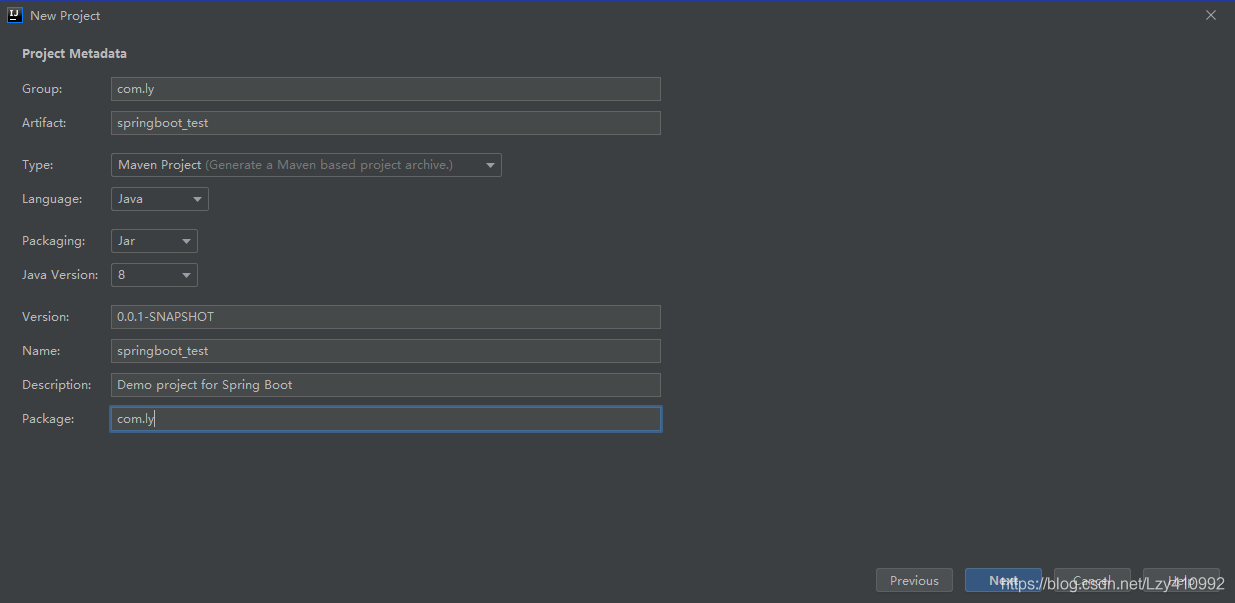
3. 添加相关模板引擎
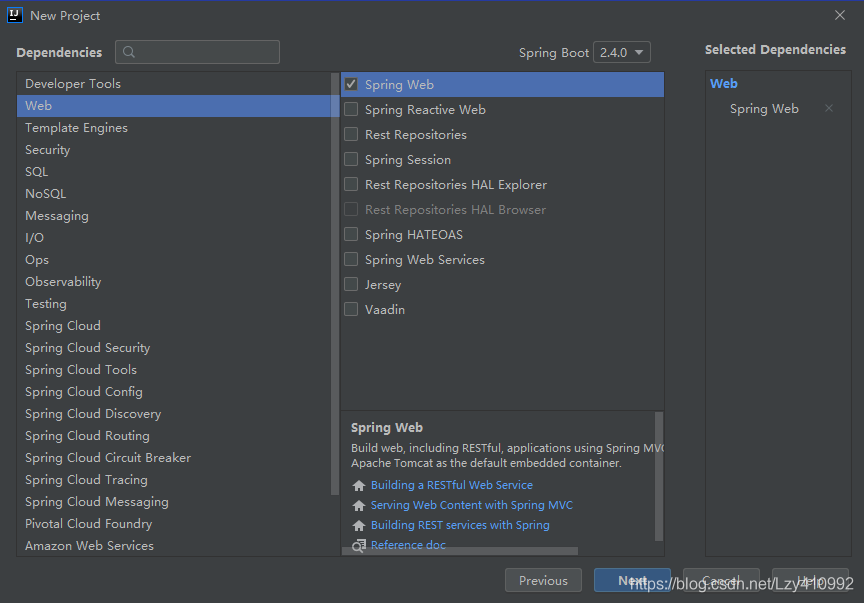
4. 搭建完成,测试运行
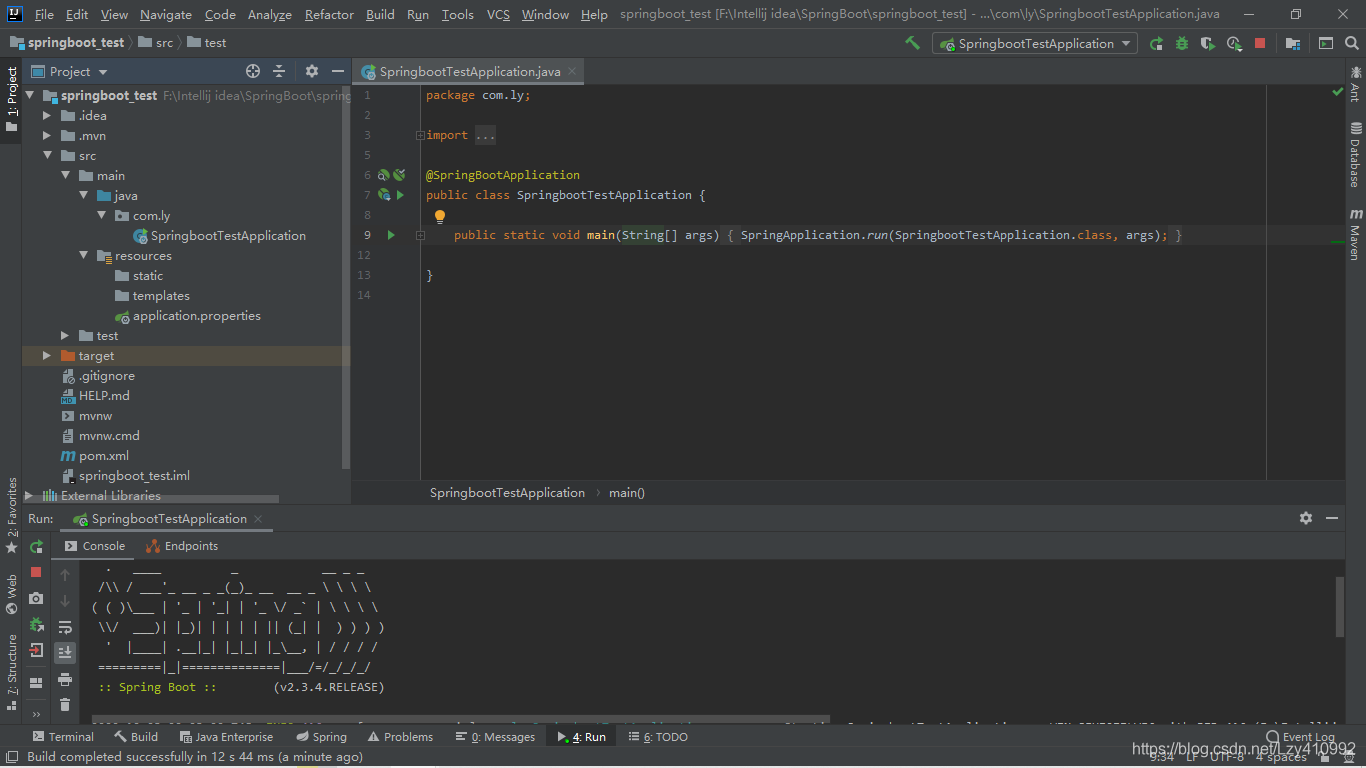
1. 起步依赖原理分析
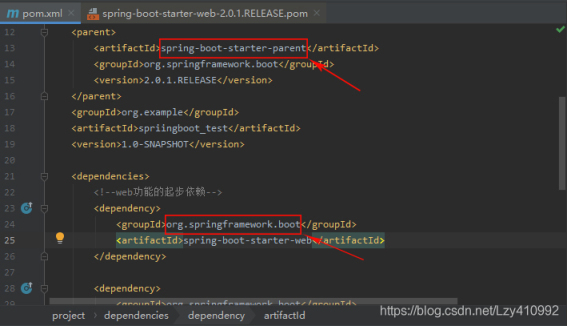
分析 spring-boot-starter-parent:
按住 Ctrl 点击 pom.xml 中的spring-boot-starter-parent
,跳转到了spring-boot-starter-parent的pom.xml
,xml 配置如下:
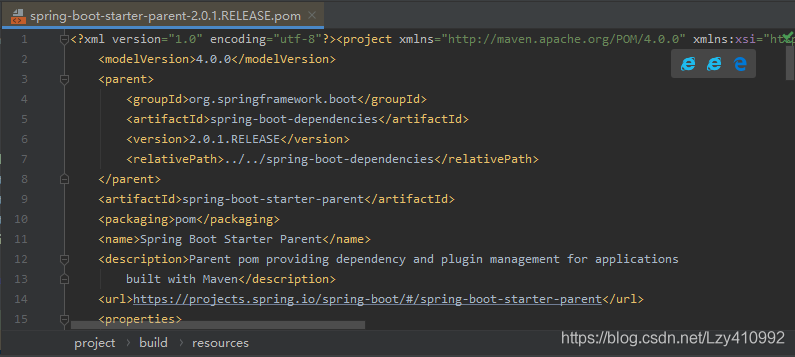
按住 Ctrl 点击 pom.xml 中的 spring-boot-starter-dependencies,跳转到了 spring-boot-starter-dependencies 的 pom.xml,xml 配置如下:
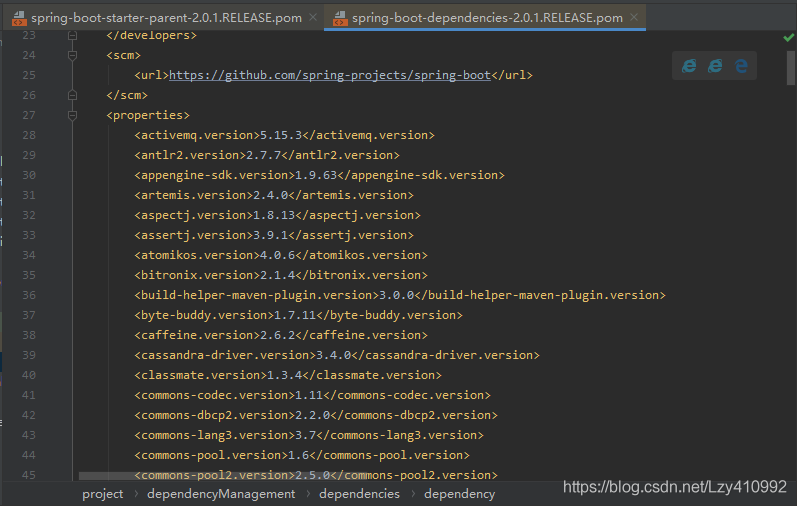
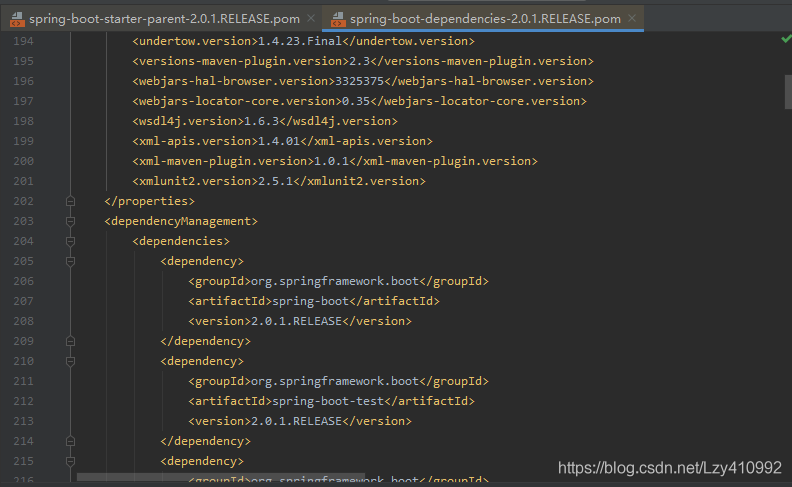
从上图中我们可以看到,一部分坐标的版本、依赖管理、插件管理已经定义好,所以我们的 SpringBoot 工程继承 spring-boot-starter-parent 后已经具备版本锁定等配置了,所以起步依赖的作用就是进行依赖的传递。
2. 分析 spring-boot-starter-web
启动器:为了让 SpringBoot 帮我们完成各种自动配置,我们必须引入 SpringBoot 提供的自动配置依赖,我们称为启动器。spring-boot-starter-parent 工程将依赖关系声明为一个或者多个启动器,我们可以根据项目需求引入相应的启动器,因为我们是 web 项目,这里我们引入 web 启动器:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
在上方代码中,并没有在这里指定版本信息,因为 SpringBoot 的父工程已经对版本进行了管理了。SpringBoot 会根据 spring-boot-starter-web 这个依赖自动引入的,而且所有的版本都已经管理好,不会出现冲突。接下来我们可以查看以下代码:按住 Ctrl 点击 pom.xml 中的spring-boot-starter-web
,跳转到了spring-boot-starter-web的pom.xml
,观察 xml 中的配置(只摘抄了部分重点配置):
<?xml version="1.0" encoding="UTF-8"?>
<project xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd" xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
、、、、、、、、、
<dependencies>
、、、、、、、、、、
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.0.5.RELEASE</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.0.5.RELEASE</version>
<scope>compile</scope>
</dependency>
</dependencies>
</project>
从上面的 spring-boot-starter-web 的 pom.xml 中我们可以发现,spring-boot-starter-web 就是将 web 开发要使用的 spring-web、spring-webmvc 等坐标进行了“打包”,这样我们的工程只要引入 spring-boot-starter-web 起步依赖的坐标就可以进行 web 开发了,同样体现了依赖传递的作用。
2. 自动配置原理分析
使用 Ctrl 点击查看启动类上的注解@SpringBootApplication
//声明类是一个 SpringBoot 引导类
@SpringBootApplication
public class MySpringBootApplication {
//main 是 Java 程序的入口
public static void main(String[] args){
//run 方法 表示运行 springboot 的引导 run 参数就是 SpringBoot 引导类的字节码对象
SpringApplication.run(MySpringBootApplication.class);
}
}
发现 @SpringBootApplication 其实是一个组合注解,这里重点的注解有 3 个:
@SpringBootConfiguration:声明配置类
@EnableAutoConfiguration:开启自动配置
@ComponentScan:开启注解扫描(扫描与其同级)
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@SpringBootConfiguration
@EnableAutoConfiguration
@ComponentScan(
excludeFilters = {@Filter(
type = FilterType.CUSTOM,
classes = {TypeExcludeFilter.class}
), @Filter(
type = FilterType.CUSTOM,
classes = {AutoConfigurationExcludeFilter.class}
)}
)
public @interface SpringBootApplication {
@AliasFor(
annotation = EnableAutoConfiguration.class
)
Class<?>[] exclude() default {};
@AliasFor(
annotation = EnableAutoConfiguration.class
)
String[] excludeName() default {};
@AliasFor(
annotation = ComponentScan.class,
attribute = "basePackages"
)
String[] scanBasePackages() default {};
@AliasFor(
annotation = ComponentScan.class,
attribute = "basePackageClasses"
)
Class<?>[] scanBasePackageClasses() default {};
}
@SpringBootConfiguration中的代码:
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Configuration
public @interface SpringBootConfiguration {
}
通过这段代码中我们可以看出,该注解中还是应用了 @Configuration 这个注解。其作用就是声明当前类是一个配置类,然后 Spring 会自动扫描到添加了 @Configuration 的类,并且读取其中的配置信息。而 @SpringBootConfiguration 是来声明当前类是 SpringBoot 应用的配置类,项目中只能有一个。所以一般我们无需自己添加。
@EnableAutoConfiguration中的代码:
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@AutoConfigurationPackage
@Import({AutoConfigurationImportSelector.class})
public @interface EnableAutoConfiguration {
String ENABLED_OVERRIDE_PROPERTY = "spring.boot.enableautoconfiguration";
Class<?>[] exclude() default {};
String[] excludeName() default {};
}
在 @EnableAutoConfiguration 注解中我们可以看到一个 @Import 注解,其作用是当前配置文件中引入其他配置类。这里引入了 AutoConfigurationImportSelector 类,在这个类中有相应的方法用来加载文件中的配置,之后 @EnableAutoConfiguration 可以帮助 SpringBoot 应用将所有符合条件的 @Configuration 配置都加载到当前 SpringBoot 创建并使用的 IOC 容器中,进而实现 @EnableAutoConfiguration 自动配置的功能。
加载的文件:(spring-boot-autoconfigure.jar/META-INF/*)
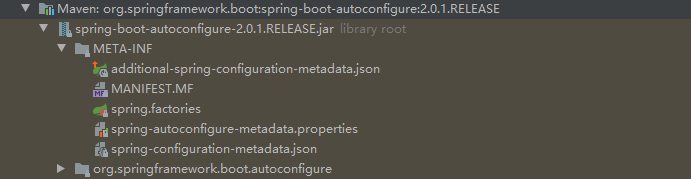
SpringBoot 内部对大量的第三方库或 Spring 内部库进行了默认配置,这些配置是否生效,取决于我们是否引入了对应库所需的依赖,如果有那么默认配置就会生效。我们在使用 SpringBoot 构建项目时,只需要引入所需依赖,配置部分交给了 SpringBoot 处理。
@ComponentScan中的代码:
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.TYPE})
@Documented
@Repeatable(ComponentScans.class)
public @interface ComponentScan {
@AliasFor("basePackages")
String[] value() default {};
@AliasFor("value")
String[] basePackages() default {};
Class<?>[] basePackageClasses() default {};
Class<? extends BeanNameGenerator> nameGenerator() default BeanNameGenerator.class;
Class<? extends ScopeMetadataResolver> scopeResolver() default AnnotationScopeMetadataResolver.class;
ScopedProxyMode scopedProxy() default ScopedProxyMode.DEFAULT;
String resourcePattern() default "**/*.class";
boolean useDefaultFilters() default true;
ComponentScan.Filter[] includeFilters() default {};
ComponentScan.Filter[] excludeFilters() default {};
boolean lazyInit() default false;
@Retention(RetentionPolicy.RUNTIME)
@Target({})
public @interface Filter {
FilterType type() default FilterType.ANNOTATION;
@AliasFor("classes")
Class<?>[] value() default {};
@AliasFor("value")
Class<?>[] classes() default {};
String[] pattern() default {};
}
}
@ComponentScan 注解的作用类似与<context:component-scan>
标签,通过 basePackageClasses 或者 basePackages 属性来指定要扫描的包。如果没有指定这些属性,那么将从声明这个注解的类所在的包开始,扫描包及子包,而我们的 @ComponentScan 注解声明的类就是 main 函数所在的启动类,因此扫描的包是该类所在包及其子包。一般启动类会放在一个比较浅的包目录中。
SpringBoot 是基于约定的,其中有许多配置都有默认值,而这些默认配置是可以进行覆盖的,我们可以使用 application.properties 或者 application.yml(application.yaml)进行重新配置。
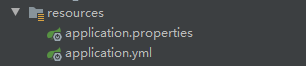
SpringBoot 默认会从 Resources 目录下依次加载application.yml文件、application.yaml、application.properties文件
,后加载的文件会对前面的文件进行覆盖。
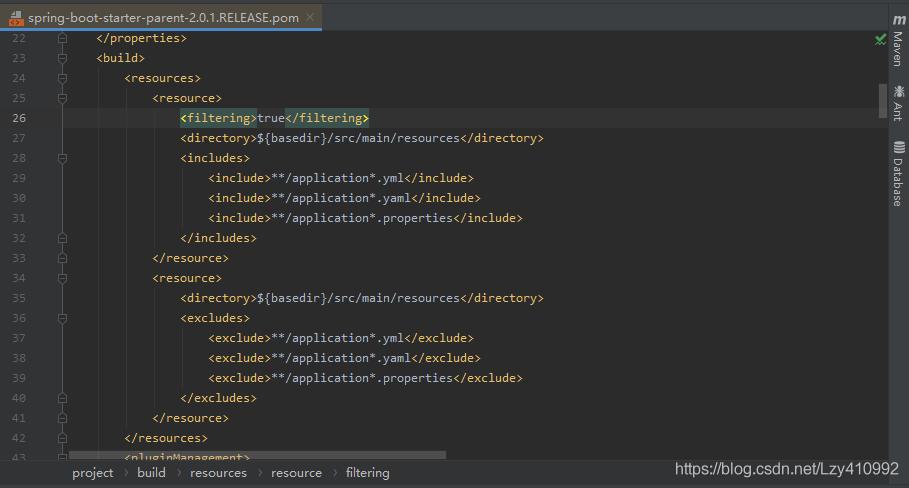
通过 application.properties 配置默认端口和访问路径:
#服务器端口号
server.port=8081
#当前 web 应用的名称
server.servlet.context-path=/demo
application.yml 配置文件:
YML 文件格式是 YAML (YAML Aint Markup Language)编写的文件格式(文件拓展名 YML 文件的扩展名.yml
、.yaml
),YAML 是一种直观的能够被电脑识别的的数据数据序列化格式,并且容易被人类阅读,容易和脚本语言交互的,可以被支持 YAML 库的不同的编程语言程序导入。YML 文件是以数据为核心的,比传统的 xml 方式更加简洁。
语法格式:key: value
,value 之前必须有一个空格
yml 文件中通过空格来代表属性之间的层级关系,属性前的空格个数不限定,相同缩进代表同一个级别
#普通数据的配置
name: zhangsan
#对象配置
person:
name: zhangsan
age: 18
addr: beijing
#或者:行内对象配置
#person: {name: zhangsan,age: 18, addr: beijing}
#配置数组、集合(普通字符串)
city:
beijing
tianjin
chongqing
changhai
#或者:city: [beijing,tianjin,chongqing,shanghai]
#配置数组、集合(对象数据)
student:
name: tom
age: 18
addr: beijing
name: lucy
age: 17
addr: tianjin
#或者:student: [{name: tom,age: 18,addr: beijing},{name: luck,age: 17,addr: tianjin}]
#Map 配置,与对象配置一样
map:
key1: value1
key2: value2
#配置端口
server:
port: 8082
配置文件与配置类的属性映射方式
使用注解 @Value 映射,我们可以通过 @Value 注解将配置文件中的值映射到一个 Spring 管理的 Bean 的字段上:
application.yml 配置
person:
name: zhangsan
age: 18
实体 Bean 代码如下:
@Controller
public class Test1Controller {
@Value("${name}")
private String name;
@Value("${person.addr}")
private String addr;
@RequestMapping("/test1")
@ResponseBody
public String test1(){
//获取配置文件的信息
return "name:"+name+",addr="+addr;
}
}
打开浏览器访问,注意路径和端口号:
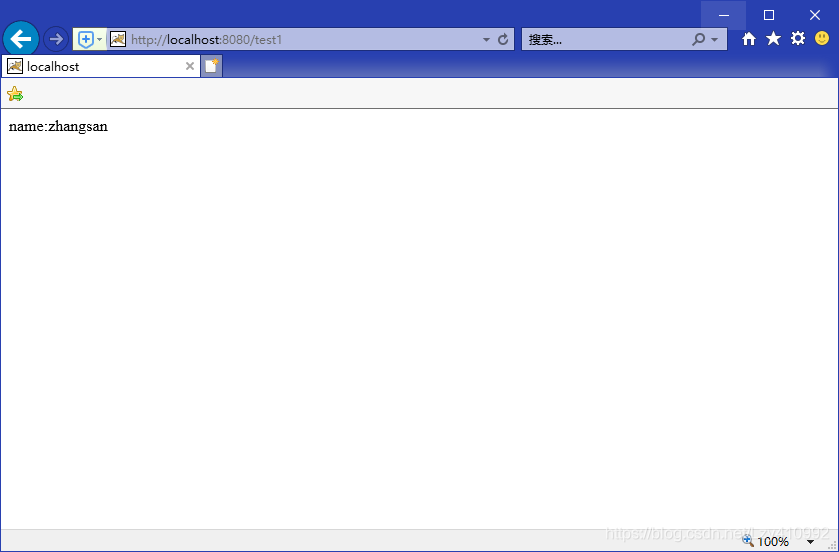
使用注解 @ConfigurationProperties 映射:
通过注解@ConfigurationProperties(prefix="配置文件中的key的前缀")
可以将配置文件中的配置自动与实体进行映射
application.yml 配置如下:
person:
name: zhangsan
age: 18
addr: beijing
实体 Bean 代码如下:
@Controller
@ConfigurationProperties(prefix = "person")
public class Test2Controller {
private String name;
private Integer age;
private String addr;
@RequestMapping("/test2")
@ResponseBody
public String test1(){
//获取配置文件的信息
return "name:"+name+",age="+age+",addr="+addr;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getAddr() {
return addr;
}
public void setAddr(String addr) {
this.addr = addr;
}
}
打开浏览器访问,注意路径和端口号:
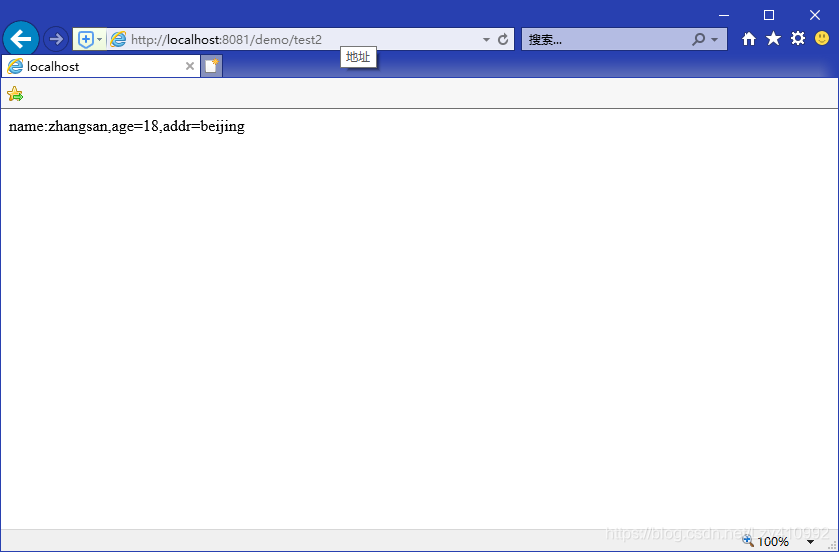
注意:使用 @ConfigurationProperties 方式可以进行配置文件与实体字段的自动映射,但需要字段必须提供 set 方法才可以,而使用 @Value 注解修饰的字段不需要提供 set 方法
在 pom.xml 中添加相关依赖:
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.6</version>
</dependency>
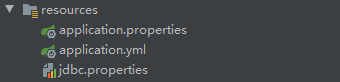
1. 通过 @Value 使用 jdbc.properties 文件进行配置
在 resources 目录下新建一个 jdbc.properties 文件,并配置相关信息:
jdbc.driverClassName=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/boot?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8
jdbc.username=root
jdbc.password=520992
评论