【Spring 学习笔记 (五)】Spring Bean 作用域和生命周期
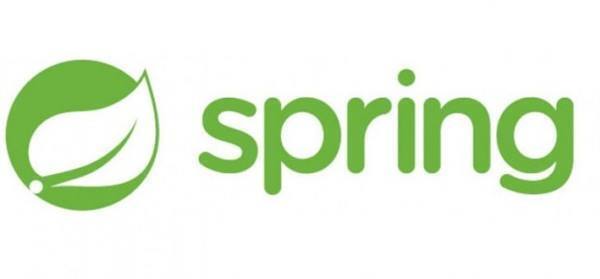
写在前面😘
大一电子信息工程新生,请多多关照,希望能在 InfoQ 社区记录自己的学习历程!
【Spring 学习笔记】 系列教程基于
Spring 5.2.10.RELEASE
讲解。
一、Spring Bean 作用域
常规的 Spring IoC 容器中 Bean 的作用域有两种:
singleton
(单例)和prototype
(非单例)注:基于 Web 的容器还有其他种作用域,在这就不赘述了。
1、singleton(单例)
singleton
是 Spring 默认的作用域。当 Bean 的作用域为 singleton 时,Spring IoC 容器中只会存在一个共享的 Bean 实例。可以更好地重用对象,节省重复创建对象的开销。设置方式:将 <bean> 元素的 scope 属性设置为
singleton
(其实也可以不用设置,因为 spring 默认就是单例模式)
案例 1
创建 Dept 类
编写 Spring 配置文件,并将 scope 属性设置为
singleton
编写运行程序
结果如下,可以发现打印出的是同一个对象
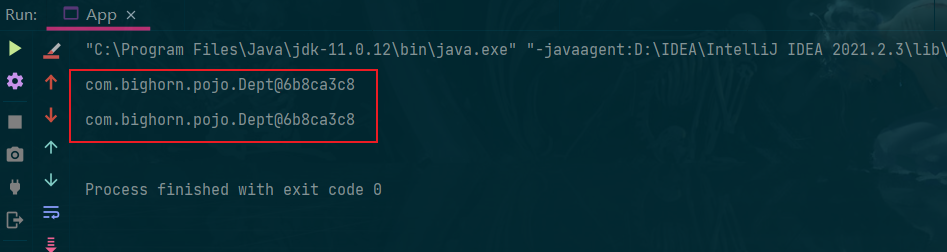
2、prototype(原型)
prototype
表示原型(非单例)模式。当 Bean 的作用域为 prototype 时,Spring 容器会在每次请求该 Bean 时,都创建一个新的 Bean 实例。设置方式:将 <bean> 元素的 scope 属性设置为
prototype
案例 2
只需修改 scope 属性为
prototype
,其他代码不变。
运行结果如下👇
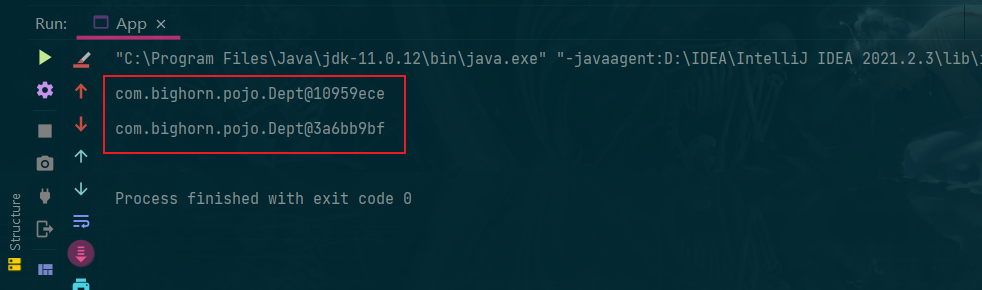
3、小结
spring bean 默认为单例,避免了对象的频繁创建与销毁,达到了 bean 对象的复用,性能高。
像表现层、业务层、数据层、工具类对象只需要调用方法,比较适合交给 Spring IoC 容器管理
但是像那种需要封装实例的域对象,因为会引发线程安全问题,不适合交给 Spring IoC 容器管理。
二、Spring Bean 生命周期
Spring Bean生命周期
:Spring Bean 对象从创建到销毁的整体过程。Spring Bean 生命周期大致可以分为以下 5 个阶段:1.Bean 的实例化、2.Bean 属性赋值、3.Bean 的初始化、4.Bean 的使用、5.Bean 的销毁
Spring 根据 Bean 的作用域来选择 Bean 的管理方式。
对于 singleton 作用域的 Bean ,Spring IoC 容器能够一直追踪 bean 的生命周期;
对于 prototype 作用域的 Bean ,Spring IoC 容器只负责创建,然后就将 Bean 的实例交给客户端代码管理,Spring IoC 容器将不再跟踪其生命周期。
综上所述: 为了更好研究如何控制 bean 周期,下面案例中创建的 bean 默认都使用单例模式。
1、如何关闭容器
由于ApplicationContext
类中没有关闭容器的方法,所以想要关闭容器需要用到ApplicationContext
的子类——ClassPathXmlApplicationContext
类。该类又有两种方法可以关闭容器👇
1.1close 关闭容器
close()
方法,在调用的时候关闭容器
1.2 注册钩子关闭容器
registerShutdownHook()
方法,在 JVM 退出前调用关闭容器
2、生命周期回调
Bean 的生命周期回调方法主要有两种:
初始化回调方法:在 Spring Bean 被初始化后调用,执行一些自定义的回调操作。
销毁回调方法:在 Spring Bean 被销毁前调用,执行一些自定义的回调操作。
我们可以通过以下 2 种方式自定义 Bean 的生命周期回调方法:
通过接口实现
通过 XML 配置实现
3、通过接口设置生命周期
我们可以在 Spring Bean 的 Java 类中,通过实现 InitializingBean
和 DisposableBean
接口,指定 Bean 的生命周期回调方法。
案例 1
创建 User 类,并实现
InitializingBean
,DisposableBean
接口,重写afterPropertiesSet()
和destroy()
方法。代码如下👇
编写 spring 配置文件
编写运行程序
运行结果如下👇
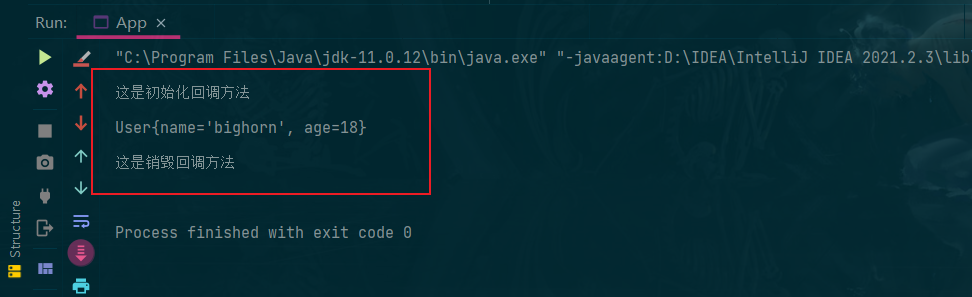
4、通过 xml 设置生命周期
注意:由于通过接口设置生命周期的方式会导致代码的耦合性过高,所以通常情况下,我们会通过 xml 设置生命周期。
通过 <bean> 元素中的 init-method
和 destory-method
属性,指定 Bean 的生命周期回调方法。
案例 2
创建 User 类,这次不需要继承那两个接口了,但要在添加两个普通方法(方法名可任意):
init()
和destory()
代表初始化和销毁方法。代码如下👇
编写 spring 配置文件,在<bean>元素里添加
init-method
和destroy-method
属性,并指定 User 类中自定义的 init 和 destory 方法(关键)
运行程序和运行结果都与案例 1 相同,这里就少些笔墨介绍了
写在后面🍻
感谢观看啦✨
有什么不足,欢迎指出哦💖
版权声明: 本文为 InfoQ 作者【倔强的牛角】的原创文章。
原文链接:【http://xie.infoq.cn/article/4e31edae2717dce8e3fe857a9】。文章转载请联系作者。
评论