【LeetCode】实现 strStr()Java 题解
发布于: 2021 年 04 月 20 日
题目描述
实现 strStr() 函数。
给你两个字符串 haystack 和 needle ,请你在 haystack 字符串中找出 needle 字符串出现的第一个位置(下标从 0 开始)。如果不存在,则返回 -1 。
示例 1:
输入:haystack = "hello", needle = "ll"
输出:2
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/implement-strstr
复制代码
代码
public class DayCode {
public static void main(String[] args) {
String haystack = "hello", needle = "ll";
int ans = new DayCode().strStr(haystack, needle);
System.out.println("ans is " + ans);
int ans1 = new DayCode().strStr1(haystack, needle);
System.out.println("ans is " + ans1);
int ans2 = new DayCode().strStr2(haystack, needle);
System.out.println("ans is " + ans2);
}
/**
* 时间复杂度 O(m * n)
* 空间复杂度 O(1)
*
* @param haystack
* @param needle
* @return
*/
public int strStr(String haystack, String needle) {
int n = haystack.length();
int m = needle.length();
for (int start = 0; start + m <= n; start++) {
if (haystack.substring(start, start + m).equals(needle)) {
return start;
}
}
return -1;
}
/**
* 时间复杂度 O(m * n)
* 空间复杂度 O(1)
*
* @param haystack
* @param needle
* @return
*/
public int strStr1(String haystack, String needle) {
int n = haystack.length();
int m = needle.length();
for (int i = 0; i + m <= n; i++) {
boolean flag = true;
for (int j = 0; j < m; j++) {
if (haystack.charAt(i + j) != needle.charAt(j)) {
flag = false;
break;
}
}
if (flag) {
return i;
}
}
return -1;
}
/**
* 时间复杂度 O(m + n)
* 空间复杂度 O(1)
*
* @param haystack
* @param needle
* @return
*/
public int strStr2(String haystack, String needle) {
int n = haystack.length();
int m = needle.length();
if (m == 0) {
return 0;
}
int[] pi = new int[m];
for (int i = 1, j = 0; i < m; i++) {
while (j > 0 && needle.charAt(i) != needle.charAt(j)) {
j = pi[j - 1];
}
if (needle.charAt(i) == needle.charAt(j)) {
j++;
}
pi[i] = j;
}
for (int i = 0, j = 0; i < n; i++) {
while (j > 0 && haystack.charAt(i) != needle.charAt(j)) {
j = pi[j - 1];
}
if (haystack.charAt(i) == needle.charAt(j)) {
j++;
}
if (j == m) {
return i - m + 1;
}
}
return -1;
}
}
复制代码
总结
在字符串考察题目中,实现字符串查找是很常见的题目,需要熟练掌握。
整体思路是遍历查找匹配。观察分析暴力法,发现有很多的重复计算。我们可以使用 KMP 算法来减少重复计算,提升查找效率。
坚持每日一题,加油!
划线
评论
复制
发布于: 2021 年 04 月 20 日阅读数: 16
版权声明: 本文为 InfoQ 作者【HQ数字卡】的原创文章。
原文链接:【http://xie.infoq.cn/article/4cfc559ebe18c58c415fd6798】。文章转载请联系作者。
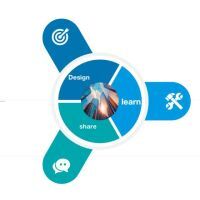
HQ数字卡
关注
还未添加个人签名 2019.09.29 加入
LeetCode,略懂后端的RD
评论