} catch (InterruptedExceptione) {
e.printStackTrace();
}
return this.name + ":" + Thread.currentThread() . getName();
@Override
protected String getFallback() {
return"失败了";
}
复制代码
}
重新执行调用代码,可以发现返回的内容是“失败了”,证明已经触发了回退。
# 三、 信号量策略配置
信号量策略配置方法如以下代码所示:
```java
public MyHystrixCommand(String name) {
super ( HystrixCommand . Setter
.Wi thGroupKey(Hystr ixCommandGroupKey .Factory .asKey( "MyGroup"))
. andCommandPropertiesDefaults (
Hystr ixCommandProperties . Setter( )
. withExecut ionIsolationStrategy(
Hystr ixCommandProperties
. ExecutionIsolat ionStrategy . SEMAPHORE
)
)
);
this.name = name;
}
复制代码
之前在 run 方法中特意输出了线程名称,通过这个名称就可以确定当前是线程隔离还是信号量隔离。
四、 线程隔离策略配置
系统默认采用线程隔离策略,我们可以通过andThreadPoolPropertiesDefaults
配置线程池的一些参数,如以下代码所示:
public MyHystrixCommand(String name) {
super(HystrixCommand.Setter.withGroupKey (
HystrixCommandGroupKey.Factory .asKey( "MyGroup"))
.andCommandPropertiesDefaults (
HystrixCommandProperties.Setter()
.withExecutionIsolationStrategy (
HystrixCommandProperties.ExecutionIsolationStrategy.THREAD
)
) . andThreadPoolPropertiesDefaults (
HystrixThreadPoolProperties. Setter()
. withCoreSize(10 )
. withMaxQueueSize(100)
.withMax. imumSize(100)
)
);
this.name = name ;
}
复制代码
五、 结果缓存
缓存在开发中经常用到,我们常用 Redis 这种第三方的缓存数据库来对数据进行缓存处理。Hystrix 中也为我们提供了方法级别的缓存。通过重写getCacheKey
来判断是否返回缓存的数据,getCacheKey
可以根据参数来生成,这样同样的参数就可以都用到缓存了。
改造之前的 MyHystrixCommand
,在其中增加 getCacheKey
的重写实现,如以下代码所示:
@verride
protected String getCacheKey() {
return String.valueOf(this.name);
}
复制代码
在上面的代码中,我们创建对象时传进来的 name 参数作为缓存的 key。
为了证明能够用到缓存,在 run 方法中加一行输出,在调用多次的情况下,如果控制台只输出了一次,那么可以知道后面的都是走的缓存逻辑,如以下代码所示:
@override
protected String run() {
System.err.print1n("get data");
return this.name + ":" + Thread. currentThread().getName();
}
复制代码
执行 main 方法,发现报错了:
根据错误提示可以知道,缓存的处理取决于请求的上下文,我们必须初始化Hystrix-RequestContext
。
改造 main 方法中的调用代码,初始化HystrixRequestContext
,如以下代码所示:
public static void main(String[] args)throws InterruptedException, ExecutionException {
HystrixRequestContext context = HystrixRequestContext. initializeContext();
String result = new MyHystrixCommand("yinjihuan").execute();
System.out.println(result);
Future<string>future = new MyHystrixCommand("yinjihuan") .queue();
System.out.println(future.get());
context . shutdown() ;
}
复制代码
改造完之后重写执行 main 方法,可以做正常运行了,输出结果如下
可以看到只输出一次 get data ,缓存生效。
六、 缓存清除
在上一节中我们学习了如何使用 Hystrix 来实现数据缓存功能。有缓存必然就有清除缓存的动作,当数据发生变动时,必须将缓存中的数据也更新掉,不然就会产生脏数据的问题。同样,Hystrix 也有清除缓存的功能。
增加一个支持缓存清除的类,如以下代码所示:
public class ClearCacheHystrixCommand extends HystrixCommand<String> {
private final String name ;
private static final HystrixCommandKey GETTER_ KEY =
HystrixCommandKey.Factory . asKey("MyKey");
public ClearCacheHystrixCommand (String name) {
super ( HystrixCommand . Setter .withGroupKey ( HystrixCommandGroupKey .
Factory .asKey( "MyGroup" )) . andCommandKey (GETTER_ KEY )
);
this.name = name;
}
public static void fushCache(String name) {
HystrixRequestCache.getInstance ( GETTER_ KEY ,HystrixConcurrencyStrategyDefault. getInstance()). clear (name);
}
@Override
protected String getCacheKey() {
return String.valueOf(this.name);
}
@Override
protected String run() {
System.err.println("get data");
return this.name + ":" + Thread.currentThread(). getName();
}
@Override
protected String getFallback() {
return "失败了";
}
}
复制代码
flushCache方法
就是清除缓存的方法,通过HystrixRequestCache
来执行清除操作,根据getCacheKey
返回的 key 来清除。
修改调用代码来验证清除是否有效果,如以下代码所示:
HystrixRequestContext context = HystrixRequestContext.initializeContext();
String result = new ClearCacheHystrixCommand("yinjihuan"). execute();
System.out.println(result);
ClearCacheHystrixCommand.flushCache("yinjihuan");
Future<string> future = new ClearCacheHystrixCommand("yinjihuan").queue();
System.out.println( future.get());
复制代码
执行两次相同的 key,在第二次执行之前调用缓存清除的方法,也就是说第二次用不到缓存,输出结果如下:
由上可以看到,输出两次 get data,这证明缓存确实被清除了。可以把ClearCache-HystrixCommandflushCache
这行代码注释掉再执行一次,就会发现只输出了一次 get data,缓存是有效的,输入结果如下:
get data
yinjihuan:hystrix-MyGroup-1
yinjihuan:hystrix-MyGroup-1
复制代码
七、 合并请求
Hystrix 支持将多个请求自动合并为一个请求(见下方代码),利用这个功能可以节省网络开销,比如每个请求都要通过网络访问远程资源。如果把多个请求合并为一个一起执行,将多次网络交互变成一次,则会极大节省开销。
public class MyHystrixCollapser extends HystrixCollapser<List<String>,String, String>{
private final String name;
public MyHystrixCollapser(String name) {
this.name = name;
}
@Override
public String getRequestArgument() {
return name;
}
@Override
protected HystrixCommand<List<String>>createCommand( final Collection<CollapsedRequest<String,String>> requests){
return new BatchCommand(requests);
}
@override
protected void mapResponseToRequests(List<String>batchResponse,Collection<CollapsedRequest<string, String>> requests){
int count = 0;
for (CollapsedRequest<string, String> request : requests) {
request.setResponse(batchResponse.get(count++));
}
}
private static final class BatchCommand extends HystrixCommand<List<String>>{
private final Collection<CollapsedRequest<String,String>> requests;
private BatchCommand(Collection<CollapsedRequest<String, String>> requests) {
super(Setter.withGroupKey(HystrixComnandGroupKey.Factory.asKey(”ExampleGroup"))
.andCommandKey (
HystrixCommandKey.Factory.asKey("GetValueForKey")));
this. requests = requests;
}
@Override
protected List<String> run() {
System.out.println("真正执行请求......");
ArrayList<String> response = new ArrayList<String>();
for(CollapsedRequest<string, String> request : requests){
response.add("返回结果:"+ request.getArgument());
### 最后
**经过日积月累, 以下是小编归纳整理的深入了解Java虚拟机文档,希望可以帮助大家过关斩将顺利通过面试。**
由于整个文档比较全面,内容比较多,篇幅不允许,下面以截图方式展示 。如有需要获取资料文档的朋友,[可以点击这里免费获取](https://gitee.com/vip204888/java-p7)
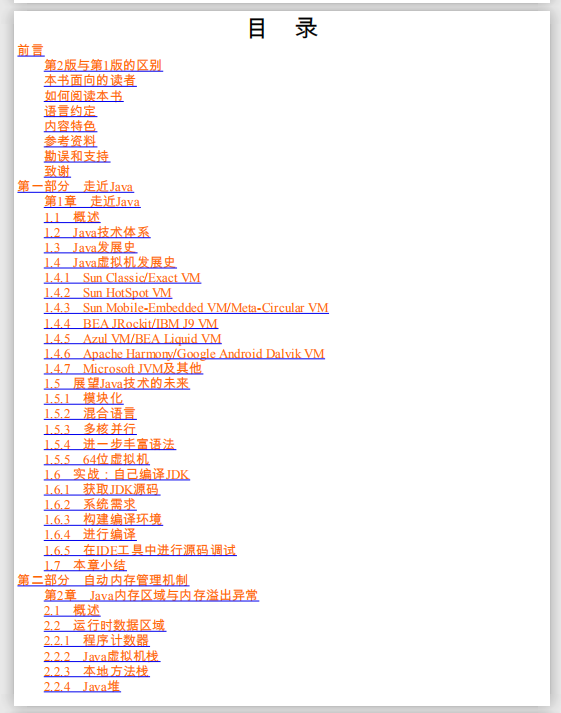
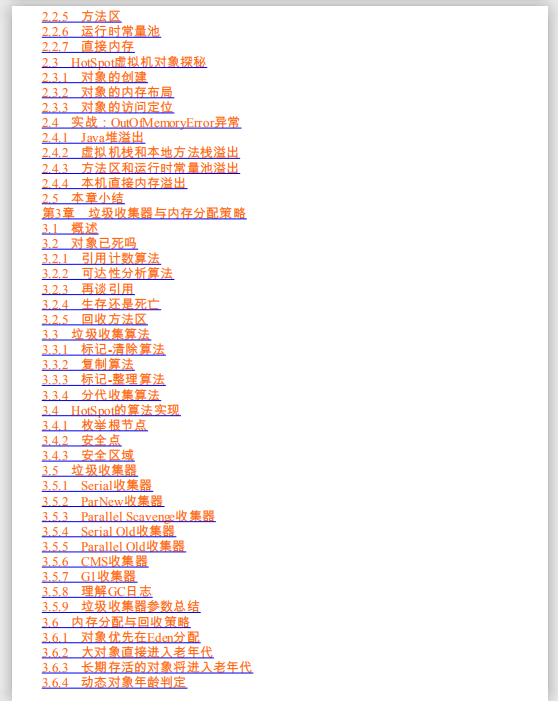
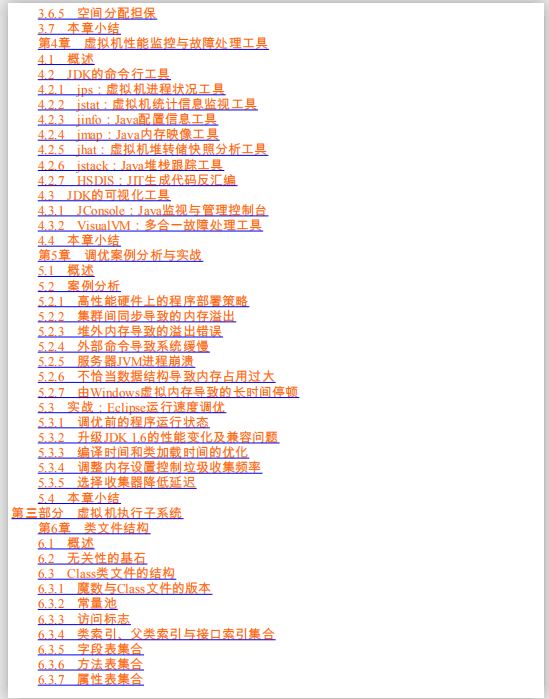
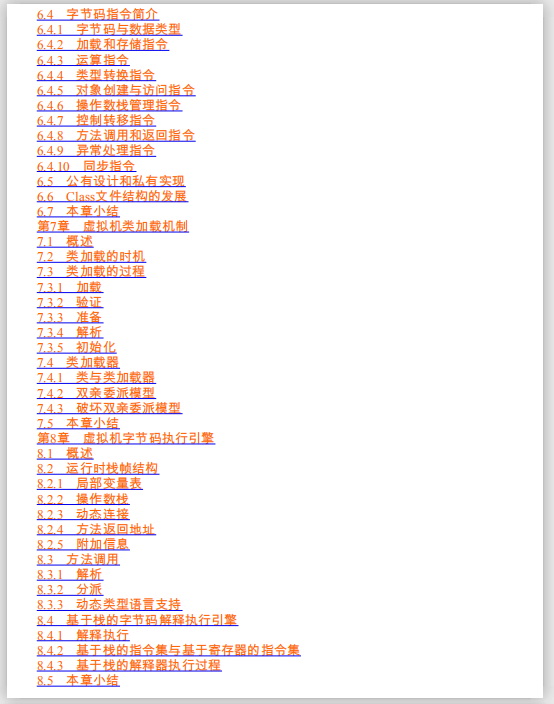
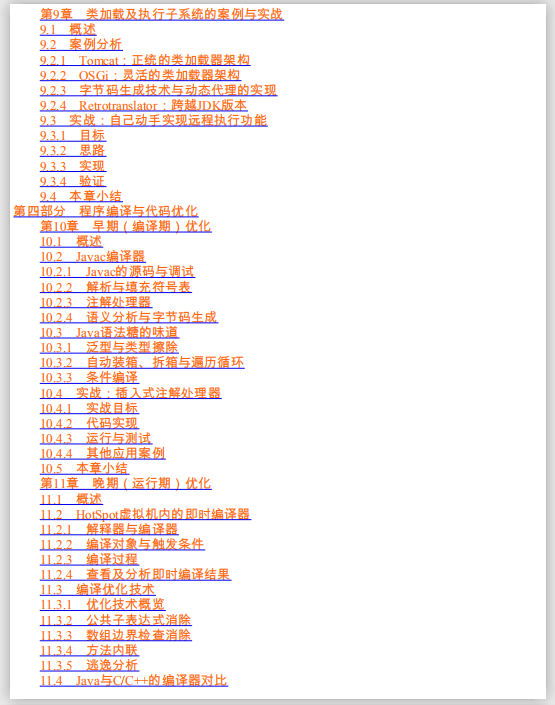
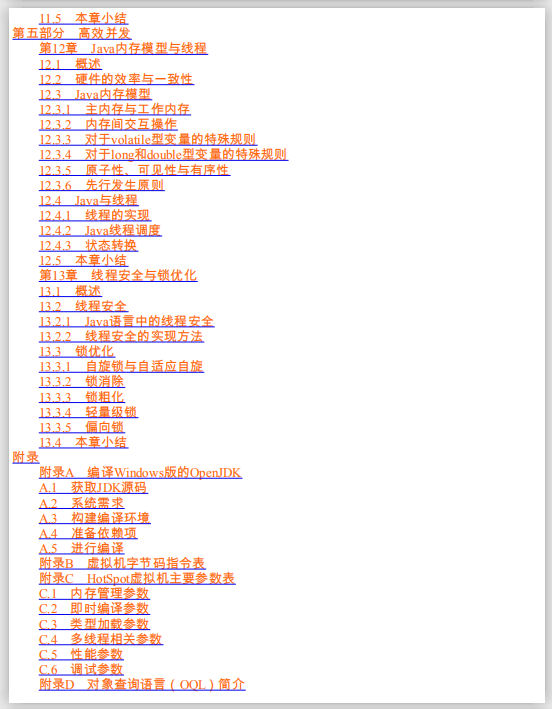
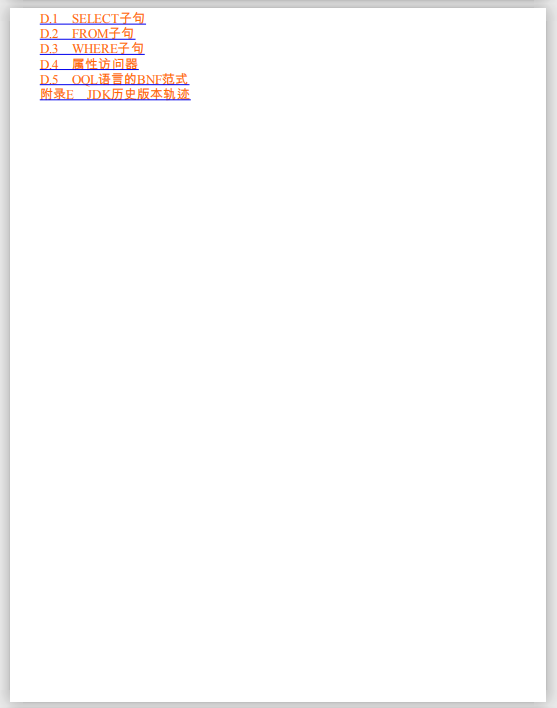
**由于篇幅限制,文档的详解资料太全面,细节内容太多,所以只把部分知识点截图出来粗略的介绍,每个小节点里面都有更细化的内容!**
复制代码
评论