Java 基础 19 IO 基础
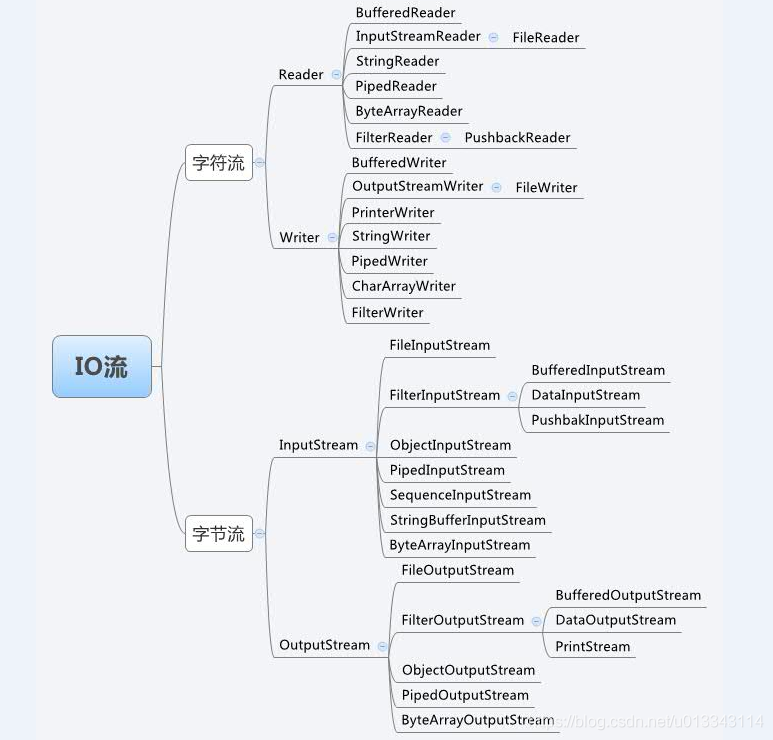
按操作数据的类型分类:
字节流
一般用于操作二进制数据,数据的单位是 byte(视频、音频、图片、exe、dll 等文件)
以 InputStream 或 OutputStream 结尾
字符流
一般用于操作文本数据,数据的单位是 char(txt、xml、html 等文件)
以 Reader 或 Writer 结尾
按数据的流向分类:
输入流
从磁盘文件或网络流到 Java 程序中,用于读取数据
所有字节输入流的父类是 InputStream
所有字符输入流的父类是 Reader
输出流
从 Java 程序流向磁盘文件或网络,用于写入数据
所有字节输出流的父类是 OutputStream
所有字符输出流的父类是 Writer
[](()文件读写
==================================================================
[](()文件读取
文件输入流 FileInputStream
作用是:读取磁盘文件或网络的数据
构造方法:
FileInputStream(File file)
FileInputStream(String filepath)
主要方法:
int read(byte[] buffer) 读取文件数据,保存到字节数组中,返回值是读取长度-1 代表读取完毕。
void close() 关闭文件流
try-with-resource
IO 流使用完后必须关闭,否则文件流无法得到释放,close 方法调用要写在 finally 中。
为简化关闭流的代码,Java1.7 引入了 try-with-resource 语法,让资源在 try-catch 完毕后自动关闭。
语法:
try(类 对象 = new 类()){
可能出现异常的代码
}catch(异常类型 对象){
处理异常的代码
}
注意:关闭的对象必须实现 Closable 接口,IO 流都实现了该接口。
文件读取的过程
创建文件输入流
创建 byte 数组
循环调用 read 方法读取数据,存入 byte 数组
操作 byte 数组
读取完毕关闭文件流
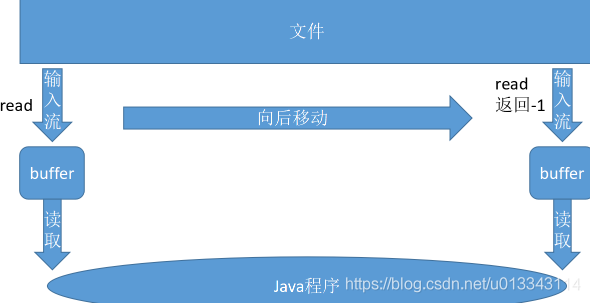
/**
读取文件内容
@param filepath
*/
public void read(String filepath){
//1、创建文件输入流
try(FileInputStream fis = new FileInputStream(filepath)) {
//2、创建 byte 数组
byte[] buffer = new byte[1024];
//3、循环调用 read 方法读取数据,存入 byte 数组
int len = 0;
while((len = fis.read(buffer)) != -1){
//4、操作 byte 数组,如果是文字将 byte[]转换为 String
String str = new String(buffer,0,len,"UTF-8");
System.out.println(str);
}
} catch (Exception e) {
e.printStackTrace();
}
}
@Test
public void testRead(){
read("C:/xpp/Test6.java");
}
[](()文件写入
文件输出流 FileOutputStream
作用是:将数据写入磁盘文件或网络
构造方法:
FileOutputStream(File file)
FileOutputStream(String filepath)
主要方法:
write(byte[] buffer,int offset,int length) 写入文件,offset 开始位置,length 写入长度
write(byte[] buffer)
close() 关闭流
文件写入步骤:
创建文件输出流
将字符串转换为 byte 数组
调用 write 写入文件
关闭文件流
/**
写入磁盘文件
@param filepath
@param content
*/
public void write(String filepath,String content){
//1、创建文件输出流
try (FileOutputStream fos = new FileOutputStream(filepath)){
//2、将字符串转换为 byte 数组 3、调用 write 写入文件
fos.write(content.getBytes());
} catch (Exception e) {
e.printStackTrace();
}
}
@Test
public void testWrite(){
write("C:/xpp/xxxx.txt","这是我要写入的内容:Hello JavaEE!");
}
[](()文件的复制
文件的复制是在读取文件的同时,将数据写入到另一个文件中
思路:
1、创建输入流和输出流
2、通过输入流读取数据到 byte 数组中
3、同时将 byte 数组中的数据写入输出流
4、循环 1、2、3
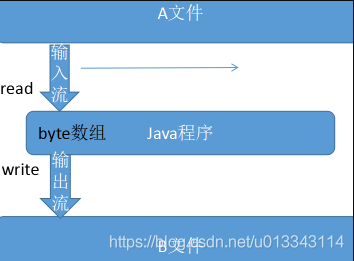
/**
复制文件
@param source 原始文件路径
@param target 目标文件路径
*/
public void copy(String source,String target){
//创建文件输入流、文件输出流
try(FileInputStream fis = new FileInputStream(source);
FileOutputStream fos = new FileOutputStream(target)){
//创建 byte 数组
byte[] buffer = new byte[1024];
int len = 0;
//循环从输入流读取数据
while((len = fis.read(buffer)) != -1){
//写入到输出流中
fos.write(buffer, 0, len);
}
}catch(Exception ex){
ex.printStackTrace();
}
System.out.println("复制完毕");
}
@Test
public void testCopy(){
copy("C:\xpp\InletexEMCFree.exe","C:\xpp\InletexEMCFree2.exe");
}
[](()缓冲
================================================================
[](()什么是缓冲
前提是内存的读写速度要远高于磁盘的读写速度,缓冲就是内存中的一块空间,通过缓冲可以提高数据的读写速度。
[](()为什么需要缓冲
如果没有缓冲,文件读写是直接从磁盘上进行的,速度比较慢;缓冲就是在内存建立一个空间,读写之前将一部分磁盘上的数据导入到缓冲内存中,后面读写就直接从缓冲中进行,减少了直接从磁盘读写的次数,从而提高读写效率。
缓冲流:在普通的 IO 流基础上,加入内存缓冲区,提高 《一线大厂 Java 面试题解析+后端开发学习笔记+最新架构讲解视频+实战项目源码讲义》无偿开源 威信搜索公众号【编程进阶路】 IO 效率。
[](()字节缓冲流
BufferedInputStream 缓冲输入流
创建方法:
new BufferedInputStream(InputStream in)
new BufferedInputStream(InputStream in,int bufferSize)
说明:
参数 InputStream 是一个字节输入流,传入缓冲输入流中后,就添加了缓冲区。
参数 bufferSize 可以设置缓冲区大小
BufferedOutputStream 缓冲输出流
创建方法:
new BufferedOutputStream(OutputStream out)
new BufferedOutputStream(OutputStream out,int bufferSize)
下面案例用 JUnit 测试使用非缓冲流和缓冲流进行文件复制的效率对比:
public class TestIO {
/**
不使用缓冲复制文件
@param source
@param target
*/
public void copyWithoutBuffer(String source,String target){
System.out.println("不使用缓冲复制文件");
//创建文件输入流和输出流
try(FileInputStream in = new FileInputStream(source);
FileOutputStream out = new FileOutputStream(target)){
//定义字节数组
byte[] buffer = new byte[100];
int len = 0;
//读取数据
while((len = in.read(buffer)) != -1){
//写入数据
out.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
}
}
/**
使用缓冲复制文件
@param source
@param target
*/
public void copyWithBuffer(String source,String target){
System.out.println("使用缓冲复制文件");
//创建缓冲输入流和输出流
try (BufferedInputStream in = new BufferedInputStream(new FileInputStream(source));
BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(target))) {
//定义字节数组
byte[] buffer = new byte[100];
int len = 0;
//读取数据
while ((len = in.read(buffer)) != -1) {
//写入数据
out.write(buffer, 0, len);
}
} catch (IOException e) {
评论