Java EE 开发系列教程 - 添加 JPA 模块
选择 Upload a new deployment。点击 Next。
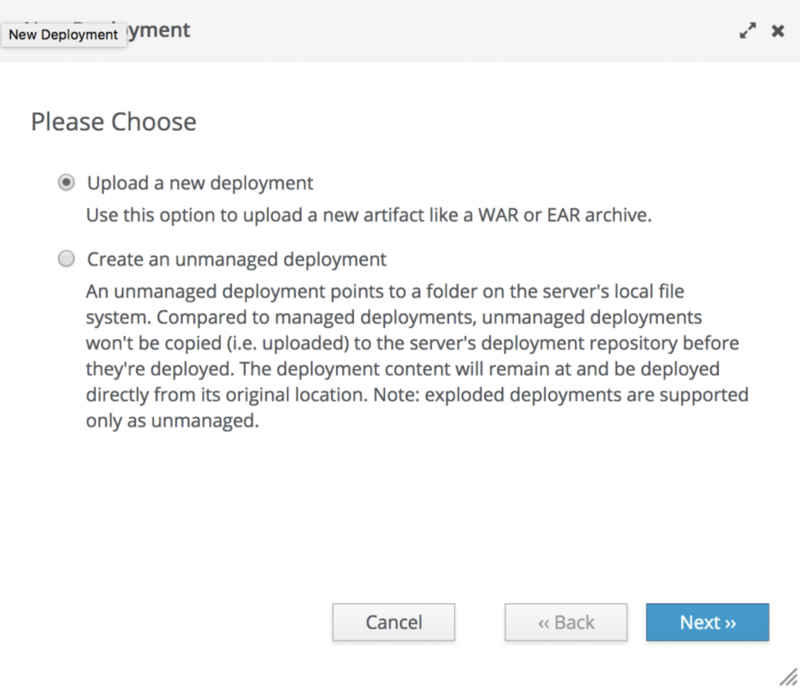
选择解压得到的 jar 文件。点击 Next。
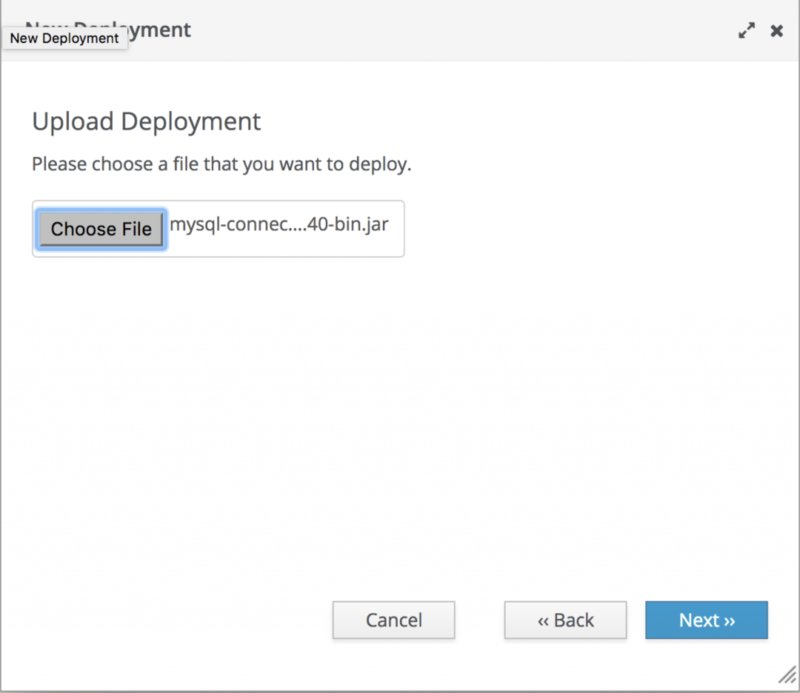
保持默认,点击 Finish。
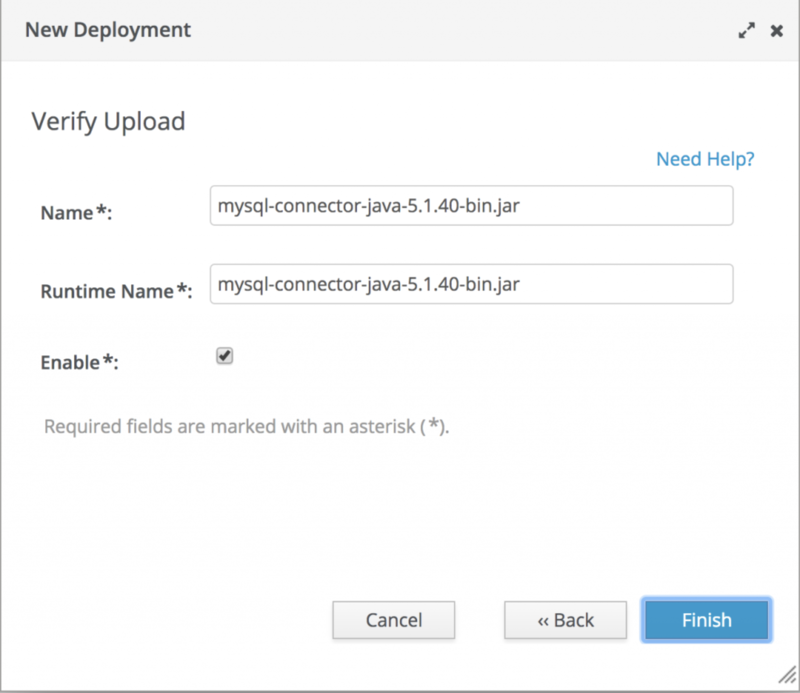
回到首页,点击 Create a datasource 旁边的 start 链接。在随后出现的页面中选择 Subsystems -> Datasources -> Non-XA。点击右边的 Add。
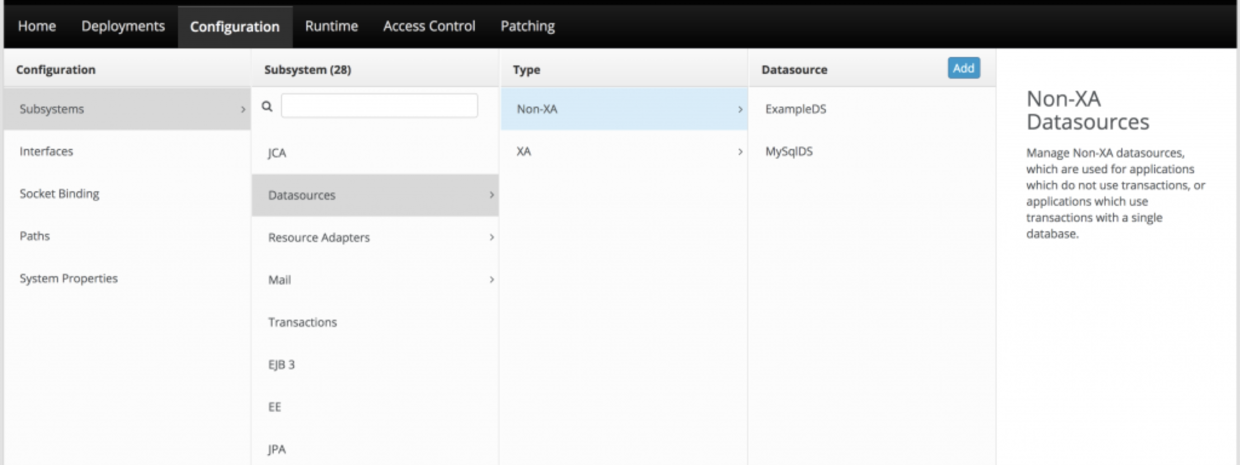
选择 MySQL datasource.?点击 Next。

指定一个 Datasource 名称和 JNDI 名称。 JNDI 名称十分重要,会在 JPA 连接到 JDBC Resource 的时候用到。点击 Next。
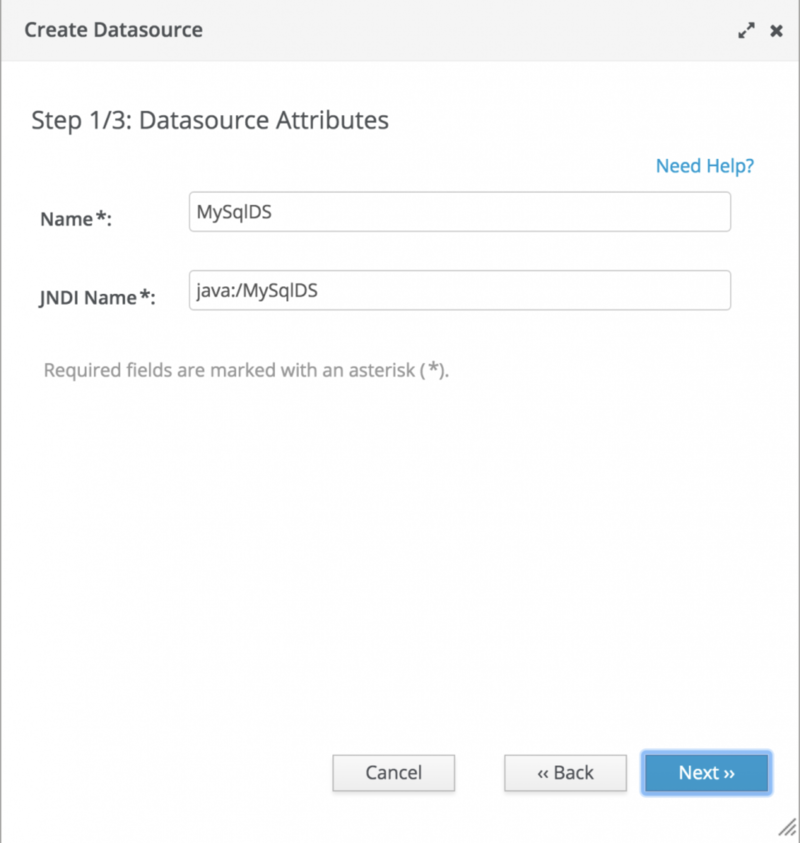
选择 Detected driver 并选择第一个检测出来的驱动。点击 next。
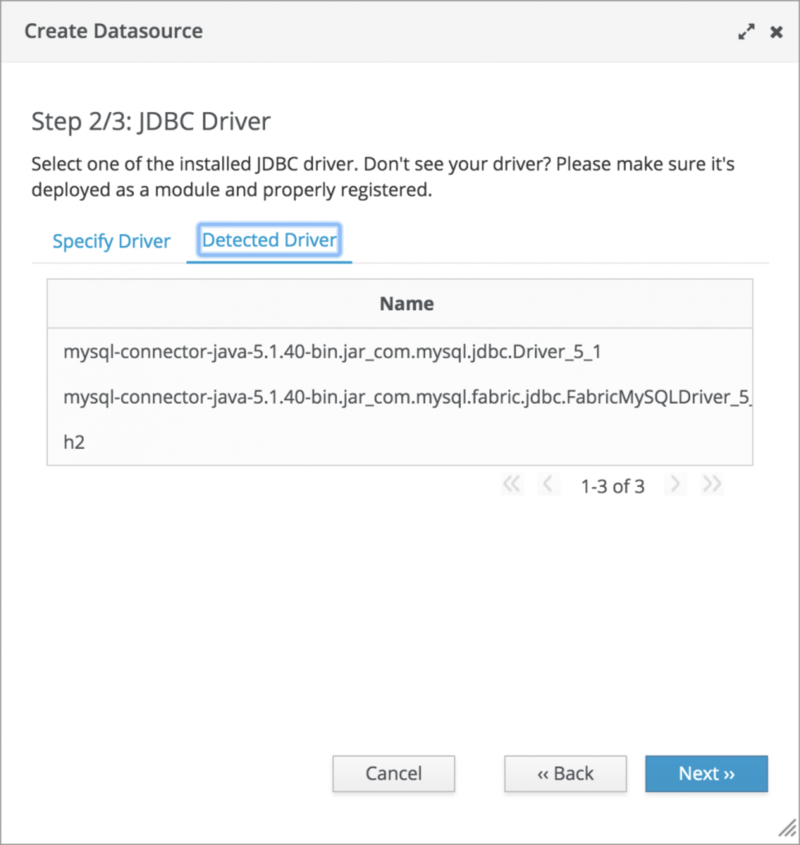
定义连接 URL。这里我创建的数据库名称为 notebook,在配置 URL 之前,先在 mysql 中使用“create database notebook”命令创建此数据库。指定 MySQL 用户名和密码之后,点击 next。
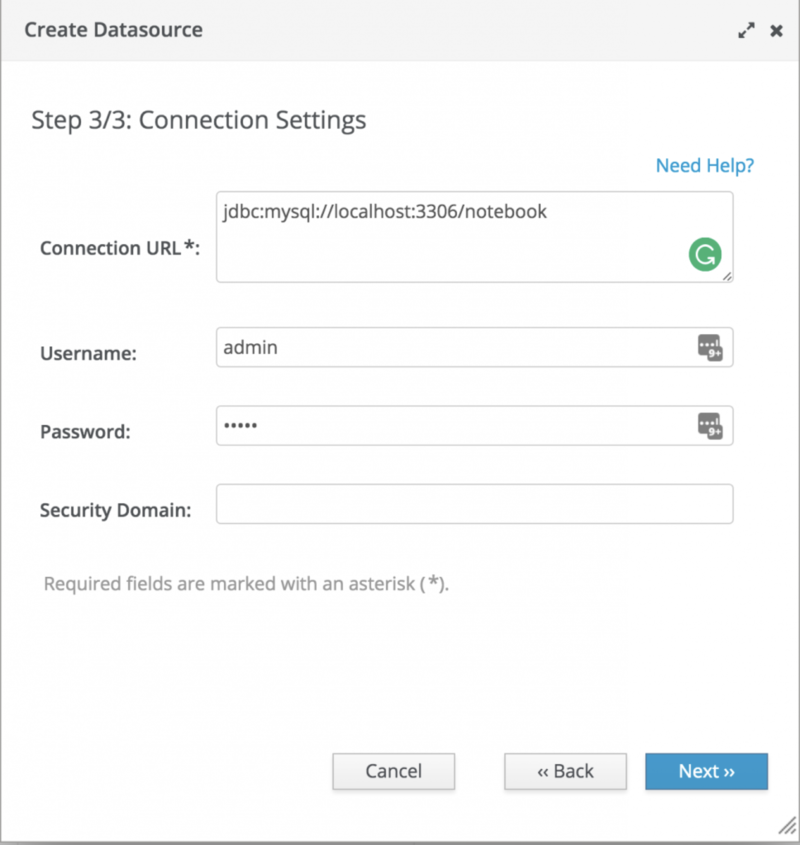
查看配置概要,如果正确点击 finish。
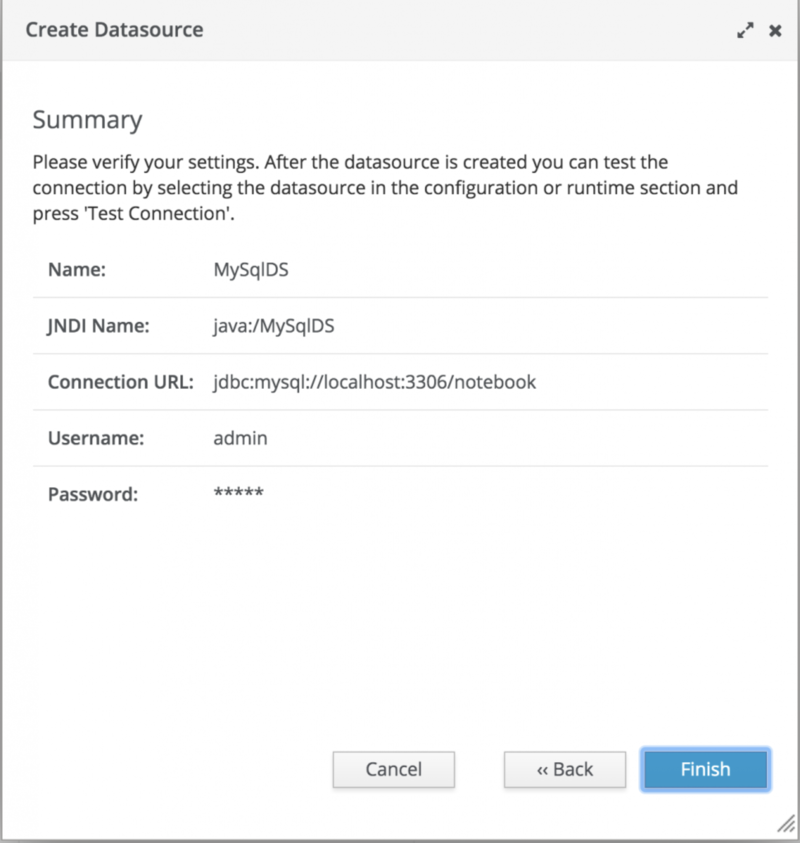
可通过下图的方式测试是否连接成功:
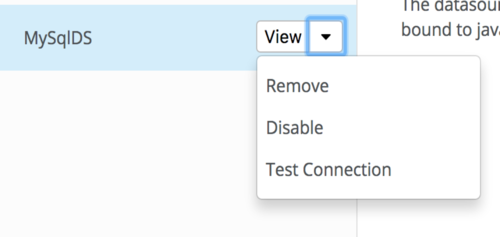
如果成功连接到数据库,会弹出下图提示框:
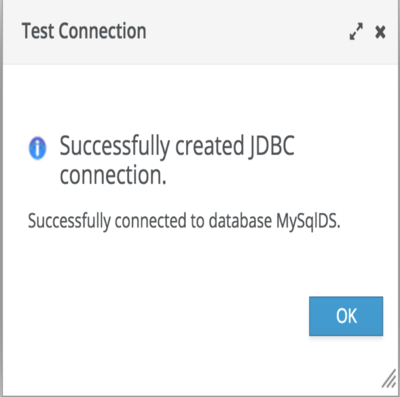
创建 JPA module
============
从 IntelliJ 中创建一个 Maven 模块。使用下图所示的 groupId, artifactId, 并选择择之前所创建的 notebookRoot 做为父项目:?com.zxuqian:notebookRoot:0.0.1-SNAPSHOT
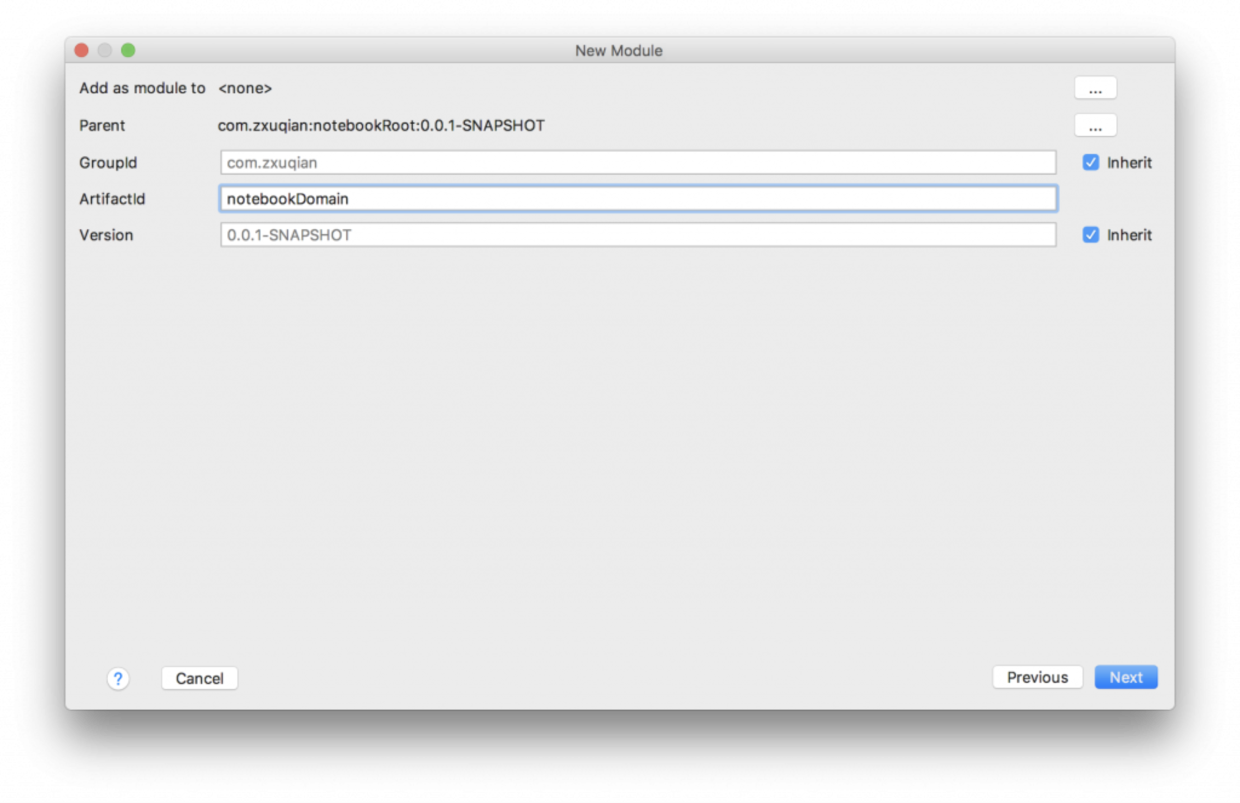
创建完成后,修改 pom.xml 文件内容:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.zxuqian</groupId>
<artifactId>notebookDomain</artifactId>
<packaging>jar</packaging>
<parent>
<groupId>com.zxuqian</groupId>
<artifactId>notebookRoot</artifactId>
<version>0.0.1-SNAPSHOT</version>
<relativePath>../notebookRoot/pom.xml</relativePath>
</parent>
<build>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.5.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
<dependencies>
<dependency>
<groupId>org.hibernate.javax.persistence</groupId>
<artifactId>hibernate-jpa-2.1-api</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
此模块打包为 Jar 项目,为 Java EE 所要求的 JPA 模块打包结构。
因为 wildfly 运行环境提供 JPA 实现,所以这里 JPA 依赖的 scope 为 provided,仅在编译时提供。
创建一个 Entity
Entity 是 Java 对象和数据库表建立关系的桥梁,使用 JPA 注解来配置如何生成对应的数据库表。首先创建一个 class,类名为 User,并填入如下代码:
package com.zxuqian.notebook.domain;
import java.io.Serializable;
import java.util.Date;
import javax.persistence.*;
/**
Entity implementation class for Entity: User
*/
@Entity
public class User implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private long id;
private String username;
private String password;
private Date dateOfBirth;
private String email;
private String phone;
public User() {
super();
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public Date getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(Date dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
}
此类为 POJO(simple plain old java bean),意思为此类提供一组属性,并提供用这些属性初始化对象的构造方法,以及访问和设置这些属性的 getter 和 setter 方法。Entity 需要实现 Serializable 接口以便 JPA 把对象序列化到数据库和从数据库中反序列化出来。
@Entity 注解表明此类为 Entity,默认使用类名作为表名。
@Id 注解声明此成员变量为数据库中的主键,使用 IDENTITY 生成策略,即 JPA 负责生成 ID 的数值。
在 src/META-INF 目录, 创建名为?persistence.xml?的文件,填入如下内容:
<?xml version="1.0" encoding="UTF-8"?>
<persistence version="2.1" xmlns="http://xmlns.jcp.org/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd">
<persistence-unit name="notebookDomain">
<jta-data-source>java:/MySqlDS</jta-data-source>
<properties>
<property name="javax.persistence.schema-generation.database.action" value="drop-and-create"/>
<property name="javax.persistence.schema-generation.scripts.action" value="none"/>
</properties>
</persistence-unit>
</persistence>
此文件为 JPA 的配置文件。 <jta-data-source>
?使用在 wildfly 下创建的 JNDI 名称。 javax.persistence.schema-generation.database.action?属性的值,drop-and-create?,表示数据库表会在每次项目部署后重新创建。 javax.persistence.schema-generation.scripts.action?表示是否生成 SQL 文件。
创建 EAR 模块
因为 JSF 模块和 JPA 模块为独立的两个项目,所以我们需要把它们打包成一个 EAR 包部署到 wildfly 服务器中。它包含的 JSF 和 JPA 模块会自动被 wildfly 识别并部署。创建此项目(groupId 和 artifacitId 请参考下方 pom 文件),并在 pom.xml 中填入下方代码:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.zxuqian</groupId>
<artifactId>notebookEAR</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>ear</packaging>
<build>
<plugins>
<plugin>
<artifactId>maven-ear-plugin</artifactId>
<version>2.10</version>
<configuration>
<earSourceDirectory>EarContent</earSourceDirectory>
<version>7</version>
<defaultLibBundleDir>lib</defaultLibBundleDir>
<modules>
<webModule>
<groupId>com.zxuqian</groupId>
<artifactId>notebook</artifactId>
<contextRoot>/notebook</contextRoot>
</webModule>
</modules>
</configuration>
</plugin>
<plugin>
<groupId>org.wildfly.plugins</groupId>
<artifactId>wildfly-maven-plugin</artifactId>
<version>1.2.0.Alpha2</version>
<configuration>
<jbossHome>/Users/zxuqian/development/tools/wildfly-10.1.0.Final</jbossHome>
</configuration>
</plugin>
</plugins>
</build>
<dependencies>
<dependency>
<groupId>com.zxuqian</groupId>
<artifactId>notebook</artifactId>
<version>0.0.1-SNAPSHOT</version>
<type>war</type>
</dependency>
<dependency>
<groupId>com.zxuqian</groupId>
<artifactId>notebookDomain</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
</project>
评论