【Spring 基础注解】对象创建相关注解,java 开发实战经典答案百度云
<context:component-scan base-package="com.yusael"/>>
作用:替换原有 Spring 配置文件中的 <bean>
标签
id
属性:在@Component
中提供了默认的设置方式:首单词首字母小写(UserDAO --> userDAO)class
属性:通过反射获得的class
的内容
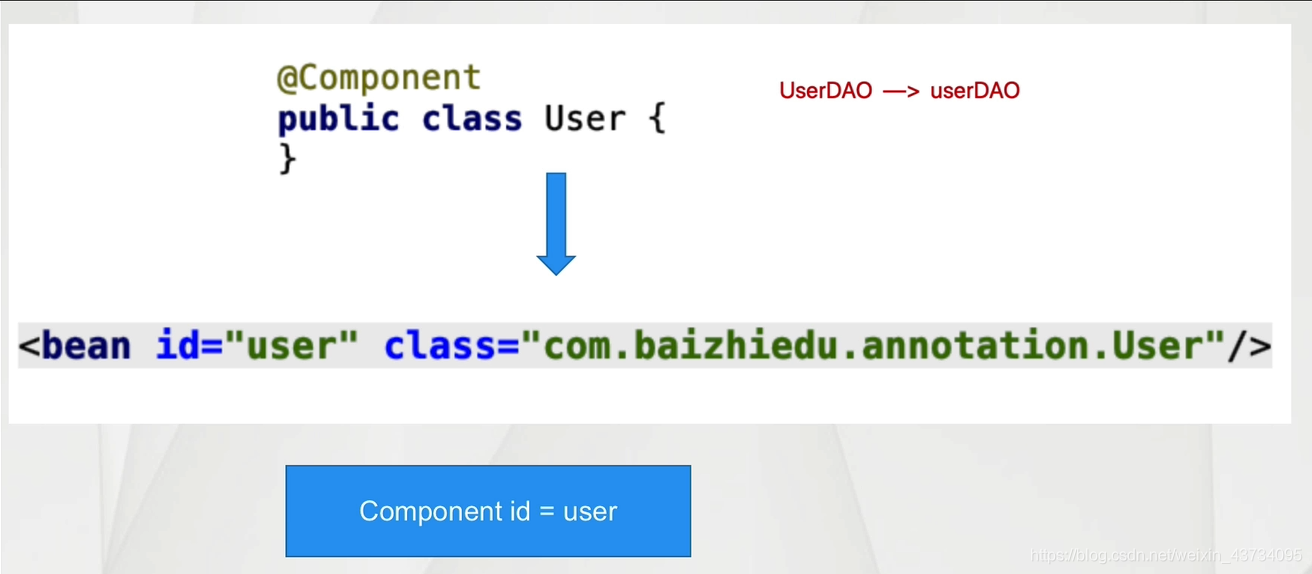
@Component
细节:
如何显式指定工厂创建对象的 id 值
@Component("u")
Spring 配置文件可以覆盖注解配置内容(相同的
id
和class
)
applicationContext.xml
<bean id="user" class="com.yusael.bean.User">
<property name="id" value="10"/>
</bean>
id 值、class 值 要和 注解 中配置的一样才会覆盖,
否则 Spring 会创建新的对象。
@Repository、@Service、@Contoller
@Repository
、@Service
、@Controller
都是 @Component
的 衍生注解。
本质上这些衍生注解就是 @Component
,通过源码可以看见他们都使用了 @Component
;
它们的存在是为了:更加准确的表达一个类型的作用。
@Repository
public class UserDAO {}
@Service
public class UserService {}
@Controller
public class UserController {}
注意:Spring 整合 Mybatis 开发过程中,不使用 @Repository
、@Component
作用:控制简单对象创建次数
注意:不添加 @Scope
,Spring 提供默认值 singleton
XML 配置:
<bean id="customer" class="com.yusael.Customer" scope="singleton | prototype"/>
注解:
// 创建单例对象
@Component
@Scope("singleton")
public class Customer {}
// 创建多例对象
@Component
@Scope("prototype")
public class Customer {}
作用:延迟创建单实例对象
注意:一旦使用 @Lazy
注解后,Spring 会在使用这个对象的时候,才创建这个对象。
XML 配置:
<bean id="account" class="com.yusael.Account" lazy="true"/>
注解:
@Component
@Lazy
public class Account {
public Account() {
System.out.println("Account.Account");
}
}
@PostConstruct、@PreDestroy:生命周期注解
初始化相关方法: @PostConstruct
InitializingBean
<bean init-method=""/>
销毁方法:@PreDestory
DisposableBean
<bean destory-method=""/>
注意:
上述的两个注解并不是 Spring 提供的,由 JSR(JavaEE 规范)520 提供
再次验证,通过注解实现了接口的契约性
=========================================================================
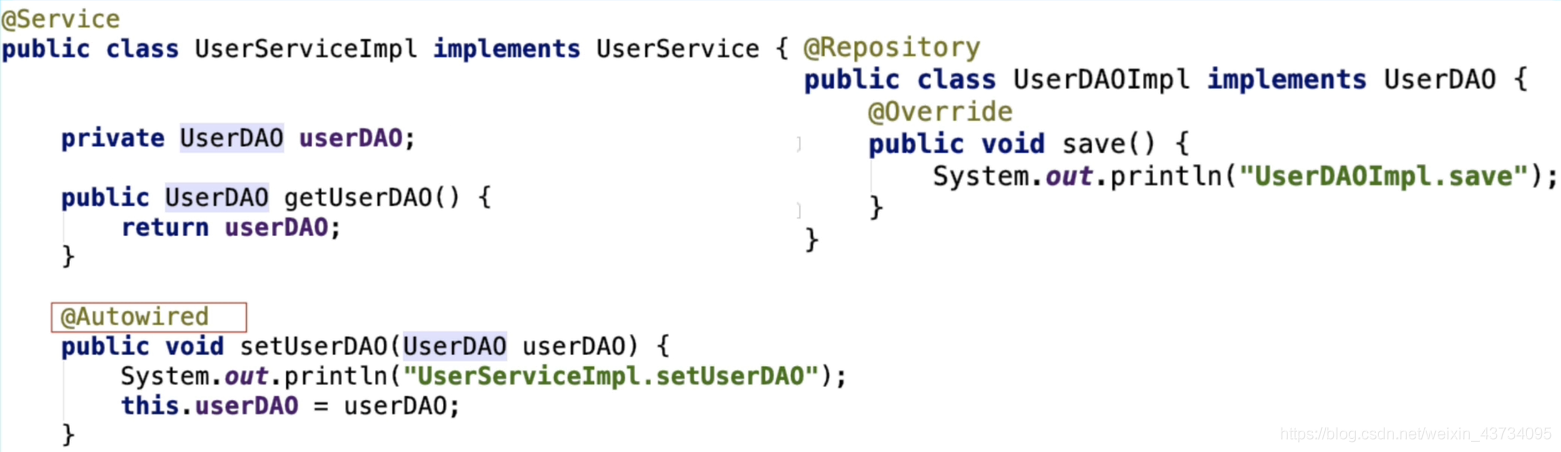
@Autowired
注解 基于类型进行注入 [推荐]:
注入对象的类型,必须与目标成员变量类型相同或者是其子类(实现类)
@Autowired
private UserDAO userDAO;
@Autowired
、@Qualifier
注解联合实现 基于名字进行注入 [了解]
注入对象的 id 值,必须与
@Qualifier
注解中设置的名字相同
@Autowired
@Qualifier("userDAOImpl")
private UserDAO userDAO;
@Autowired
注解放置位置:
放置在对应成员变量的 set 方法上,调用 set 方法赋值(在 set 里写的代码会被执行)
直接放置在成员变量上,Spring 通过反射直接对成员变量进行赋值 [推荐]
JavaEE 规范中类似功能的注解:
JSR250 提供的
@Resource(name="xxx")
基于名字进行注入
等价于 @Autowired
与 @Qualifier
联合实现的效果
注意:@Resource
注解如果名字没有配对成功,会继续 按照类型进行注入
@Resource(name="userDAOImpl")
private UserDAO userDAO;
JSR330 提供的
@Injection
作用与@Autowired
完全一样,一般用在 EJB3.0 中
方法一:@value
注解的基本使用:
设置 xxx.properties
id = 10
name = suns
Spring 的工厂读取这个配置文件
<context:property-placeholder location=""/>
代码中进行注入
属性 @Value("${key}")
方法二:使用 @PropertySource
取代 xml 配置:
设置 xxx.properties
id = 10
name = suns
在实体类上应用 @PropertySource("classpath:/xx.properties")
代码
属性 @Value("${key}")
@value
注解使用细节:
@Value
注解不能应用在静态成员变量上,如果使用,获取的值为 null@Value
注解 + Properties 这种方式,不能注入集合类型
Spring 提供新的配置形式 YAML(YML) (更多的用于 SpringBoot 中)
=========================================================================
这样配置,会扫描当前包及其子包 :
<context:component-scan base-package="com.yusael"/>
<context:component-scan base-package="com.yusael">
<context:exclude-filter type="" expression=""/>
</context:component-scan>
type="xxx"
,xxx
有以下几个可选项:
assignable
:排除特定的类型annotation
:排除特定的注解
aspectj
:切入点表达式,比较常用
包切入点: com.yusael.bean..*
类切入点: *..User
regex
:正则表达式,不常用,与切入点类似custom
:自定义排除策略,常用于框架底层开发(在 SpringBoot 源码中大量使用)
排除策略可以叠加使用:
<context:component-scan base-package="com.yusael">
<context:exclude-filter type="assignable" expression="com.yusael.bean.User"/>
<context:exclude-filter type="aspectj" expression="com.yusael.injection..*"/>
</context:component-scan>
<context:component-scan base-package="com.yusael" use-default-filters="false">
<context:include-filter type="" expression=""/>
</context:component-scan>
与排除方式使用的区别:
use-default-filters="false"
让 Spring 默认的注解扫描方式失效<context:include-filter type="" expression=""/>
用于指定扫描哪些注解
type="xxx"
与排除方式完全一样,可以参考上面
包含策略也可以叠加使用:
评论