SpringBoot 中的 yaml 语法及静态资源访问问题,mysql 面试笔试题
Person 类:
@Data
@Component
@ConfigurationProperties(prefix = "person")
public class Person {
private String userName;
private Boolean boss;
private Date birth;
private Integer age;
private Pet pet;
private String[] interests;
private List<String> animal;
private Map<String, Object> score;
private Set<Double> salarys;
private Map<String, List<Pet>> allPets;
}
Pet 类:
@Data
public class Pet {
private String name;
private Double weight;
}
在 recources 资源目录下创建 application.yaml 文件,使用 yaml 表示上述的属性:
person:
user-name: xbhog
boss: false
birth: 2021/7/27
age: 18
pet: {name: 阿毛,weight: 23}
interests: [唱歌,跳舞,玩游戏]
animal:
jerry
mario
score:
english: 30
math: 70
#第二种写法:score: {english: 30,math: 70}
salarys:
277
8999
10000
all-pets: #该 allPet 有两个 k(sick、health),每个 key 包含一个 list
sick:
{name: tom}
{name: jerry,weight:47}
health: [{name: mario,weight: 47}]
创建一个 controller 来测试我们的配置文件有没有生效:
因为我们之前已将将 person 中的属性映射到了 application.yaml 文件中,并且把 person 加入到容器中。
@Component
@ConfigurationProperties(prefix = "person")
所以我们在 myconfig 中做测试:
package com.xbhog.controller;
import com.xbhog.popj.Car;
import com.xbhog.popj.Person;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
@Autowired //自动配置,找到容器中的 person
Person person;
@RequestMapping("/person")
public Person person(){
return person;
}
}
结果如图所示:
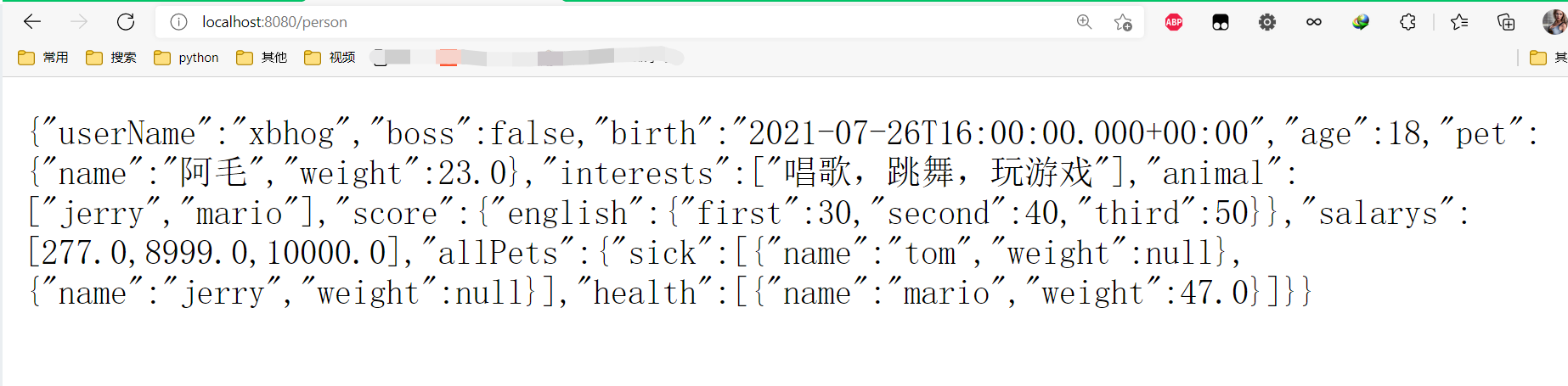
静态资源的访问问题
只有静态资源放在以下的文件目录中才可以:
/static/、public/、 resources/ 、META-INF/resources
最后一个测试没有成功,访问 META-INF/resources/img.png 返回 404,如果有感兴趣的小伙伴可以测试一下。
访问的方法是:当前项目根路径/ + 静态资源名 (localhost:8080/xxx.img)
假如我们的请求路由跟图片的名字重复了,spring boot 是先请求哪个呢?
我们创建一个 controller:
package com.xbhog.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class mycontro {
@RequestMapping("/publicimg.png")
public String demo(){
return "asss";
}
}
让它与 public 文件夹下面的图片相同:
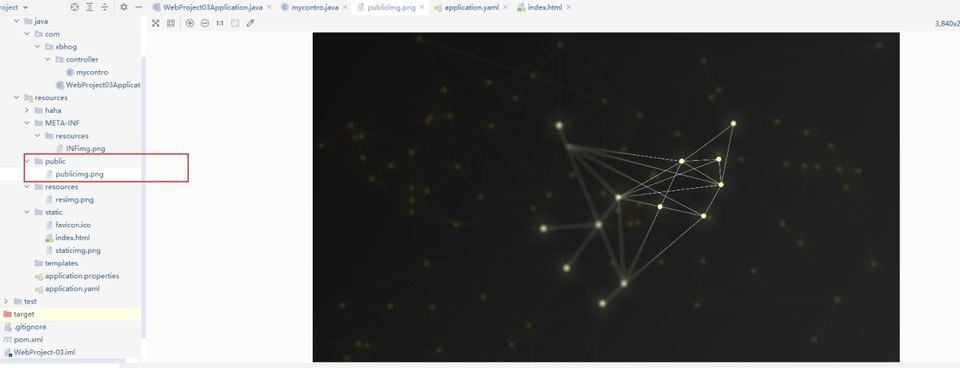
开启主程序测试结果如下:

当我们随便请求一个
不存在的图片,会返回给我们 404.
从上面可以看出来,请求进来,先去找 Controller 看能不能处理。不能处理的所有请求又都交给静态资源处理器。静态资源也找不到则响应 404 页面
改变默认的静态资源的路径:
在 application.yaml 文件中:
spring:
mvc:
static-path-pattern: /res/**
这样我们访问资源的时候必须啊要加 res 前缀.
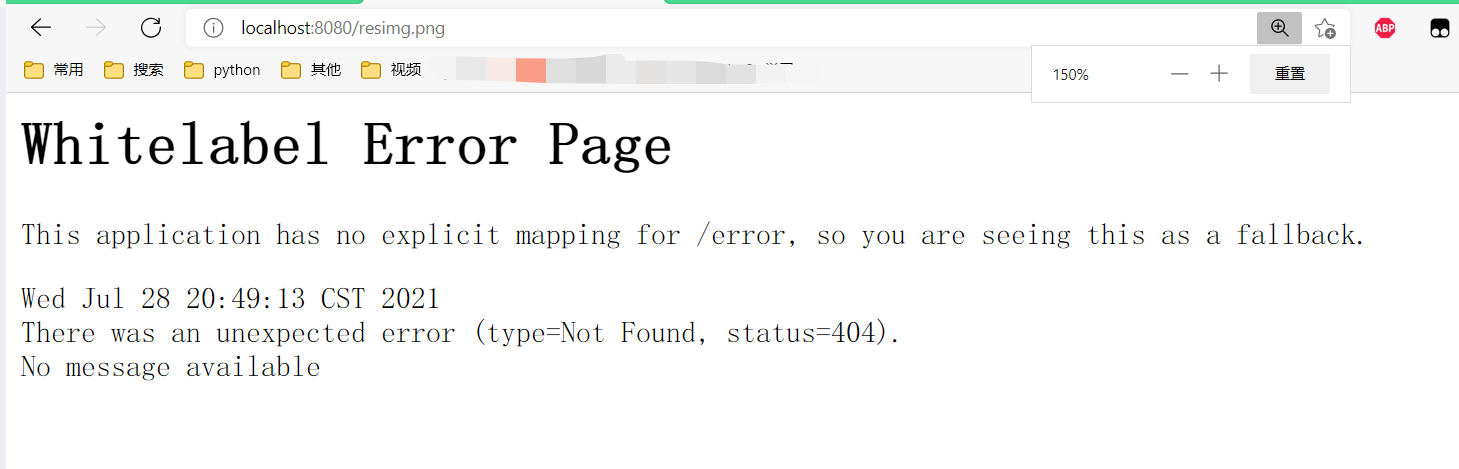
评论