Koltin47
配置就完成了。
5.代码中使用包,拷贝到 libs 下面,然后右键 add as library,build.gradile 上会有引入的包
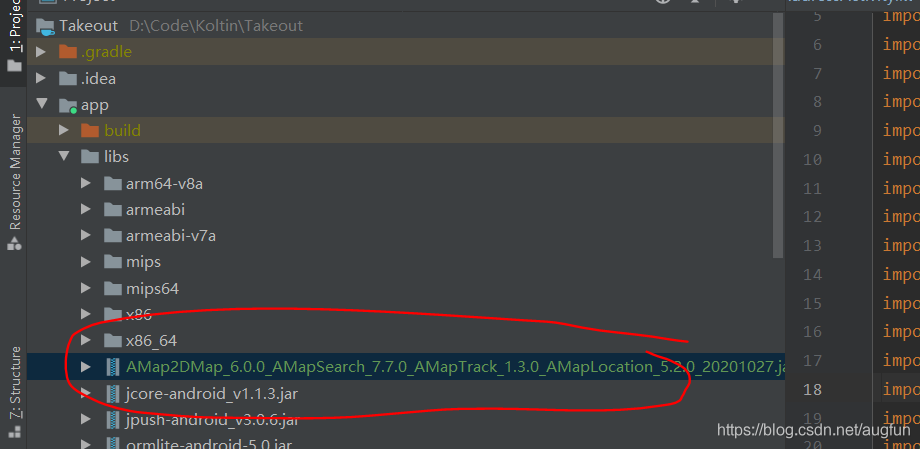

6.Manifist 文件中配置,与高德的说明书操作一致,只需要导入 key 就可以了
代码如下:
AddOrEditAddressActivity.java 跳转到地图展示的主界面
package com.example.takeout.ui.activity
import android.content.DialogInterface
import android.content.Intent
import android.graphics.Color
import android.os.Bundle
import android.text.Editable
import android.text.TextUtils
import android.text.TextWatcher
import android.util.TypedValue
import android.view.View
import android.widget.Toast
import androidx.appcompat.app.AlertDialog
import androidx.appcompat.app.AppCompatActivity
import com.example.takeout.R
import com.example.takeout.model.beans.RecepitAddressBean
import com.example.takeout.model.dao.AddressDao
import com.example.takeout.utils.CommonUtil
import kotlinx.android.synthetic.main.activity_add_edit_receipt_address.*
import org.jetbrains.anko.toast
class AddOrEditAddressActivity : AppCompatActivity(), View.OnClickListener {
override fun onClick(v: View?) {
when (v?.id) {
R.id.ib_back -> finish()
R.id.ib_add_phone_other -> rl_phone_other.visibility = View.VISIBLE
R.id.ib_delete_phone -> et_phone.setText("")
R.id.ib_delete_phone_other -> et_phone_other.setText("")
R.id.ib_select_label -> selectLabel()
R.id.btn_ok -> {
val isOk = checkReceiptAddressInfo()
if (isOk) {
if (intent.hasExtra("addressBean")) {
updateAddress()
}else {
//新增地址
insertAddress()
}
}
}
R.id.btn_location_address -> {
val intent = Intent(this, MapLocationActivity::class.java)
startActivityForResult(intent, 1001)
}
}
}
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if(resultCode == 200){
if(data!=null) {
val title = data.getStringExtra("title")
val address = data.getStringExtra("address")
et_receipt_address.setText(title)
et_detail_address.setText(address)
}
}
}
private fun updateAddress() {
var username = et_name.text.toString().trim()
var sex = "女士"
if (rb_man.isChecked) {
sex = "先生"
}
var phone = et_phone.text.toString().trim()
var phoneOther = et_phone_other.text.toString().trim()
var address = et_receipt_address.text.toString().trim()
var detailAddress = et_detail_address.text.toString().trim()
var label = tv_label.text.toString()
addressBean.username = username
addressBean.sex = sex
addressBean.phone = phone
addressBean.phoneOther = phoneOther
addressBean.address = address
addressBean.detailAddress = detailAddress
addressBean.label = label
addressDao.updateRecepitAddressBean(addressBean)
toast("更新地址成功")
finish()
}
private fun insertAddress() {
var username = et_name.text.toString().trim()
var sex = "女士"
if (rb_man.isChecked) {
sex = "先生"
}
var phone = et_phone.text.toString().trim()
var phoneOther = et_phone_other.text.toString().trim()
var address = et_receipt_address.text.toString().trim()
var detailAddress = et_detail_address.text.toString().trim()
var label = tv_label.text.toString()
addressDao.addRecepitAddressBean(RecepitAddressBean(999, username, sex, phone, phoneOther, address, detailAddress, label, "38"))
toast("新增地址成功")
finish()
}
val titles = arrayOf("无", "家", "学校", "公司")
val colors = arrayOf("#778899", "#ff3399", "#ff9933", "#33ff99")
lateinit var addressDao: AddressDao
private fun selectLabel() {
val builder = AlertDialog.Builder(this)
builder.setTitle("请选择地址标签")
builder.setItems(titles, object : DialogInterface.OnClickListener {
override fun onClick(dialog: DialogInterface?, which: Int) {
tv_label.text = titles[which].toString()
tv_label.setBackgroundColor(Color.parseColor(colors[which]))
tv_label.setTextColor(Color.BLACK)
}
})
builder.show()
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_add_edit_receipt_address)
processIntent()
addressDao = AddressDao(this)
if (CommonUtil.checkDeviceHasNavigationBar(this)) {
activity_add_address.setPadding(0, 0, 0, 48.dp2px())
}
btn_location_address.setOnClickListener(this)
ib_back.setOnClickListener(this)
ib_add_phone_other.setOnClickListener(this)
ib_delete_phone.setOnClickListener(this)
ib_delete_phone_other.setOnClickListener(this)
ib_select_label.setOnClickListener(this)
btn_ok.setOnClickListener(this)
et_phone.addTextChangedListener(object : TextWatcher {
override fun afterTextChanged(s: Editable?) {
if (!TextUtils.isEmpty(s)) {
ib_delete_phone.visibility = View.VISIBLE
} else {
ib_delete_phone.visibility = View.INVISIBLE
}
}
override fun beforeTextChanged(s: CharSequence?, start: Int, count: Int, after: Int) {
}
override fun onTextChanged(s: CharSequence?, start: Int, before: Int, count: Int) {
}
})
et_phone_other.addTextChangedListener(object : TextWatcher {
override fun afterTextChanged(s: Editable?) {
if (!TextUtils.isEmpty(s)) {
ib_delete_phone_other.visibility = View.VISIBLE
} else {
ib_delete_phone_other.visibility = View.INVISIBLE
}
}
override fun beforeTextChanged(s: CharSequence?, start: Int, count: Int, after: Int) {
}
override fun onTextChanged(s: CharSequence?, start: Int, before: Int, count: Int) {
}
})
}
lateinit var addressBean: RecepitAddressBean
private fun processIntent() {
if (intent.hasExtra("addressBean")) {
addressBean = intent.getSerializableExtra("addressBean") as RecepitAddressBean
tv_title.text = "修改地址"
ib_delete.visibility = View.VISIBLE
ib_delete.setOnClickListener {
addressDao.deleteRecepitAddressBean(addressBean)
toast("删除此地址成功")
finish()
}
et_name.setText(addressBean.username)
val sex = addressBean.sex
if ("先生".equals(sex)) {
rb_man.isChecked = true
} else {
rb_women.isChecked = true
}
et_phone.setText(addressBean.phone)
et_phone_other.setText(addressBean.phoneOther)
et_receipt_address.setText(addressBean.address)
et_detail_address.setText(addressBean.detailAddress)
tv_label.text = addressBean.label
}
}
fun Int.dp2px(): Int {
return TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP,
toFloat(), resources.displayMetrics).toInt()
}
fun checkReceiptAddressInfo(): Boolean {
val name = et_name.getText().toString().trim()
if (TextUtils.isEmpty(name)) {
Toast.makeText(this, "请填写联系人", Toast.LENGTH_SHORT).show()
return false
}
val phone = et_phone.getText().toString().trim()
if (TextUtils.isEmpty(phone)) {
Toast.makeText(this,
"请填写手机号码", Toast.LENGTH_SHORT).show()
return false
}
if (!isMobileNO(phone)) {
Toast.makeText(this, "请填写合法的手机号", Toast.LENGTH_SHORT).show()
return false
}
val receiptAddress = et_receipt_address.getText().toString().trim()
if (TextUtils.isEmpty(receiptAddress)) {
Toast.makeText(this, "请填写收获地址", Toast.LENGTH_SHORT).show()
return false
}
val address = et_detail_address.getText().toString().trim()
if (TextUtils.isEmpty(address)) {
Toast.makeText(this, "请填写详细地址", Toast.LENGTH_SHORT).show()
return false
}
return true
}
fun isMobileNO(phone: String): Boolean {
val telRegex = "[1][358]\d{9}"//"[1]"代表第 1 位为数字 1,"[358]"代表第二位可以为 3、5、8 中的一个,"\d{9}"代表后面是可以是 0~9 的数字,有 9 位。
return phone.matches(telRegex.toRegex())
}
}
MapLocationActivity.java 地图展示的主界面,加载地图的相关信息,并监听地图相关的初始化,获取到地图的数据信息使用 RecycleView 展示加载得到的数据
package com.example.takeout.ui.activity
import android.Manifest
import android.content.pm.PackageManager
import android.os.Bundle
import android.util.Log
import androidx.appcompat.app.AppCompatActivity
import androidx.core.app.ActivityCompat
import androidx.core.content.ContextCompat
import androidx.recyclerview.widget.LinearLayoutManager
import com.amap.api.location.AMapLocation
import com.amap.api.location.AMapLocationClient
import com.amap.api.location.AMapLocationListener
import com.amap.api.maps2d.AMap
import com.amap.api.maps2d.CameraUpdateFactory
import com.amap.api.maps2d.MapView
import com.amap.api.maps2d.model.LatLng
import com.amap.api.services.core.LatLonPoint
import com.amap.api.services.core.PoiItem
import com.amap.api.services.poisearch.PoiResult
import com.amap.api.services.poisearch.PoiSearch
import com.example.takeout.R
import com.example.takeout.ui.adapter.AroundRvAdapter
import kotlinx.android.synthetic.main.activity_map_location.*
import org.jetbrains.anko.toast
class MapLocationActivity : AppCompatActivity(), AMapLocationListener,
PoiSearch.OnPoiSearchListener {
override fun onPoiItemSearched(poiItem: PoiItem?, rcode: Int) {
}
override fun onPoiSearched(poiResult: PoiResult?, rcode: Int) {
if (rcode == 1000) {
if (poiResult != null) {
val poiItems: ArrayList<PoiItem> = poiResult.pois!!
adapter.setPoiItemList(poiItems)
}
}
}
override fun onLocationChanged(aMapLocation: AMapLocation?) {
if (aMapLocation != null) {
toast(aMapLocation.address)
Log.d("aMapLocation", "aMapLocation===" + aMapLocation.address)
//移动地图到当前位置
aMap.moveCamera(
CameraUpdateFactory.changeLatLng(
LatLng(
aMapLocation.latitude,
aMapLocation.longitude
)
)
)
aMap.moveCamera(CameraUpdateFactory.zoomTo(16f))
doSearchQuery(aMapLocation)
mLocationClient.stopLocation()
}
}
private fun doSearchQuery(aMapLocation: AMapLocation) {
val query = PoiSearch.Query("", "", aMapLocation.city)
//keyWord 表示搜索字符串,
//第二个参数表示 POI 搜索类型,二者选填其一,选用 POI 搜索类型时建议填写类型代码,码表可以参考下方(而非文字)
//cityCode 表示 POI 搜索区域,可以是城市编码也可以是城市名称,也可以传空字符串,空字符串代表全国在全国范围内进行搜索
query.pageSize = 30// 设置每页最多返回多少条 poiitem
query.pageNum = 1//设置查询页码
val poiSearch = PoiSearch(this, query)
//搜索范围
poiSearch.bound =
PoiSearch.SearchBound(LatLonPoint(aMapLocation.latitude, aMapLocation.longitude), 350)
poiSearch.setOnPoiSearchListener(this)
poiSearch.searchPOIAsyn();
}
//声明 AMapLocationClient 类对象
lateinit var mLocationClient: AMapLocationClient
//地图控制器
lateinit var aMap: AMap
lateinit var adapter: AroundRvAdapter
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_map_location)
val mapView = findViewById(R.id.map) as MapView
rv_around.layoutManager = LinearLayoutManager(this)
adapter = AroundRvAdapter(this)
rv_around.adapter = adapter
mapView.onCreate(savedInstanceState)// 此方法必须重写
aMap = mapView.map
checkPermision()
}
private val WRITE_COARSE_LOCATION_REQUEST_CODE: Int = 10
private fun checkPermision() {
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION)
!= PackageManager.PERMISSION_GRANTED
) {
//申请 WRITE_EXTERNAL_STORAGE 权限
ActivityCompat.requestPermissions(
this, arrayOf(Manifest.permission.ACCESS_COARSE_LOCATION),
WRITE_COARSE_LOCATION_REQUEST_CODE
);//自定义的 code
} else {
initLocation()
}
}
override fun onRequestPermissionsResult(
requestCode: Int,
permissions: Array<out String>,
grantResults: IntArray
) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults)
if (grantResults[0] == PackageManager.PERMISSION_GRANTED) {
//用户在对话框中点击允许
initLocation()
} else {
finish()
toast("需要有定位权限才能成功定位")
}
}
private fun initLocation() {
//初始化定位
mLocationClient = AMapLocationClient(getApplicationContext());
//设置定位回调监听
mLocationClient.setLocationListener(this);
//启动定位
评论