初学字典 -python
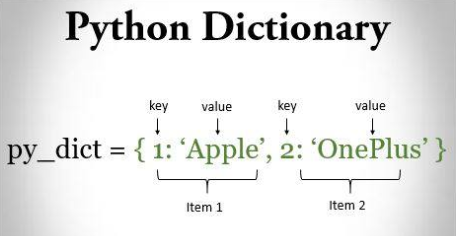
#字典:当索引不好用时
'''
当索引不好用时,用字典序列存储数据是很有个优势的
为什么?
字典是通过键-值对的数据结构存数据
而且键是唯一的,不重复的
这样在一定的数据量下,他的查找效率是很高的
'''
names={'a':'2','a':'3',1:1,"":0}
print(names) #结果:{'': 0, 1: 1}
'''
字典的特性归纳:
1、键值是唯一的,不重复的,如果存在重复,则最后一个为准
2、键和值都是空字符串的,会舍弃掉
3、元组可以作为键,但序列不能作为键
'''
d = dict(name ='GUMby',age=42)
print(str(d))
''''
字典的创建方式有哪几种
1、d = {'aaa':1,'bbbb':c}
2、dict(aaa=1,'ccc'='c')
3、通过 zip 方法,将两个列表转为字典
'''
list1=[1,2,3,4,5]
list2=['a','b','c','d',]
d2= dict(zip(list1,list2))
print(d2)
list3=((1,2,3),2,3,4,5)
list4=['a','b','c','d',]
d2= dict(zip(list3,list4))
print(d2)
'''
字典的操作方法
字典的基本行为在很多方面与序列 sequence 类似
len(d): 返回的字典中项的数量
d[k] :获取关联到键 k 上的值
d[k]=v:将 v 关联到键 k 上
del d[k]:删除键为 k 的项
k in d :检查的中是否含有键为 k 的项
字典与列表的不同:
1、键类型::自带你的键不一定为整形数据,键可以是任意的不可变类型,如浮点型,字符串,元组
2、自动添加:即使键起初在字典中并不存在,也可以赋值,这样杨自带你就会建立新的项
而列表在不适用 append 方法的情况下,是不能将值关联列表范围之外的索引上
3、成员资格:使用 in 关键字查找功能,字典是查键,而列表是查值
'''
a=[]
#a[2]=10 #运行提示:index out of range
a.append(10)
b={}
b[10]=2
b['ceil']=360
print(a,' ',b)
'''
字典格式化字符串 %(键值)s
'''
stringFormat = "ceil's phone number is %(ceil)s"
print(stringFormat % b)
b['title']='My home page'
b['text']='Welcome to my home page'
template = '''<html>
<head><title>%(title)s</title></head>
<body>
<h1>%(title)s</h1>
<p>%(text)s</p>
</body>
'''
print (template % b)
'''
字典的方法
clear:清空字典所有的项,注意:如果这个字典
copy:返回一个具有相同键值的新字典,显现的是 shallow copy ,不是 deep copy
fromkeys:使用给定的键建立新的字典
get:获取某个键对应的值,比较宽松,如键不存在,则返回 None
has_key:检查字典中是否含有特定的键 python3.0 以后的版本是没有这个方法的
items:将所有的项以列表形式返回
iteritems:返回一个迭代器 #与 items 一样的,在 python3.0 以后版本废除了
pop:获取给定键的值,并从字典中移除
poptiem:弹出随机的项,因为字典没有“最后的元素”或者其他有关顺序的概念
setdefault:可以获取与给定键相关联的值,当键不存在的时候,setdefault 返回默认值并更新字典
如果键存在,则不更新字典,并返回键关联的值
update:利用一个字典更新另外一个字典
values:以列表的形式返回字典中的值
itervalues:返回值的迭代器 在 python3.0 以后版本废除了
'''
#clear :
testdict =dict(aa='b',d='aa',f='gggg')
x = testdict
print('x:',x)
#returnValue = testdict.clear()
returnValue = x.clear()
print(testdict,'clear 返回值',returnValue)
print(testdict,'clear 返回值'+str(returnValue))
print('x:',x) #两个或两个以上的对象指向了同一个字典,其中一个对象使用 clear 方法,那么其他的对象的字典也会清空
#copy
print('copy----------------')
testdict =dict(aa='b',d='aa',f=['1','2','3'])
x = testdict.copy()
testdict['aa'] ='cccc' #值为不可变对象,指挥变更自己的值,不会影响 copy 对象
testdict['f'][1]='aaaa' #值为列表,可变对象,所有的 copy 都会变更,这就是浅赋值
print(testdict,x)
#深赋值 deep copy
from copy import deepcopy
testdict =dict(aa='b',d='aa',f=['1','2','3'])
xdeep = deepcopy(testdict)
xshallow = testdict.copy()
testdict['f'].pop()
print(testdict,'\n',xdeep,xshallow)
#formkeys
tempdict =dict.fromkeys(['aaa','bbb'])
tempdict2 =dict.fromkeys(['aaa','bbb'],'空的')
#{'aaa': None, 'bbb': None} {'aaa': '空的', 'bbb': '空的'}
print(tempdict,tempdict2)
#get 由于是比较宽松的查找,不会报错
testdict =dict(aa='b',f=['1','2','3'],d='aa')
print(testdict.get('aaaa')) #不会爆 keyerror,返回的是 None,也可以返回指定的值
print(testdict.get('aaaa','空的'))
#print(testdict['aaaaa']) # 字典中不存在的键,会报 keyerror
#items
i= testdict.items()
print(i)
for ii in i:
print(ii) #返回的是列表中元素是元组 一个迭代器
print('------------------pop')
#pop :删除一个键,然后返回这个键对应的值
#print(testdict.pop('aa'))
listRange = range(1,100,2)
dd = {}.fromkeys(listRange,'空的')
print(dd)
print(dd.popitem())
#setdefault
print(testdict.setdefault('aa','N/A'))
print(testdict.setdefault('aaa','N/A'))
#values
print(dd.values())
评论