Spring 源码学习~ 循环依赖(面试必问系列,学 java 基础的心得体会
package com.example;
import com.example.config.AppConfiguration;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import com.example.bean.A;
/**
<pre>
</pre>
<pre>
@author mazq
修改记录
修改后版本: 修改人: 修改日期: 2020/11/05 10:22 修改内容:
</pre>
*/
public class TestApplication {
public static void testCircularReferences() {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext();
context.register(AppConfiguration.class);
//context.setAllowCircularReferences(false);
context.refresh();
A bean = context.getBean(A.class);
System.out.println(bean);
}
public static void main(String[] args) {
// 测试 Sprin 循环依赖
testCircularReferences();
}
}
经过测试,一直在循环调用:
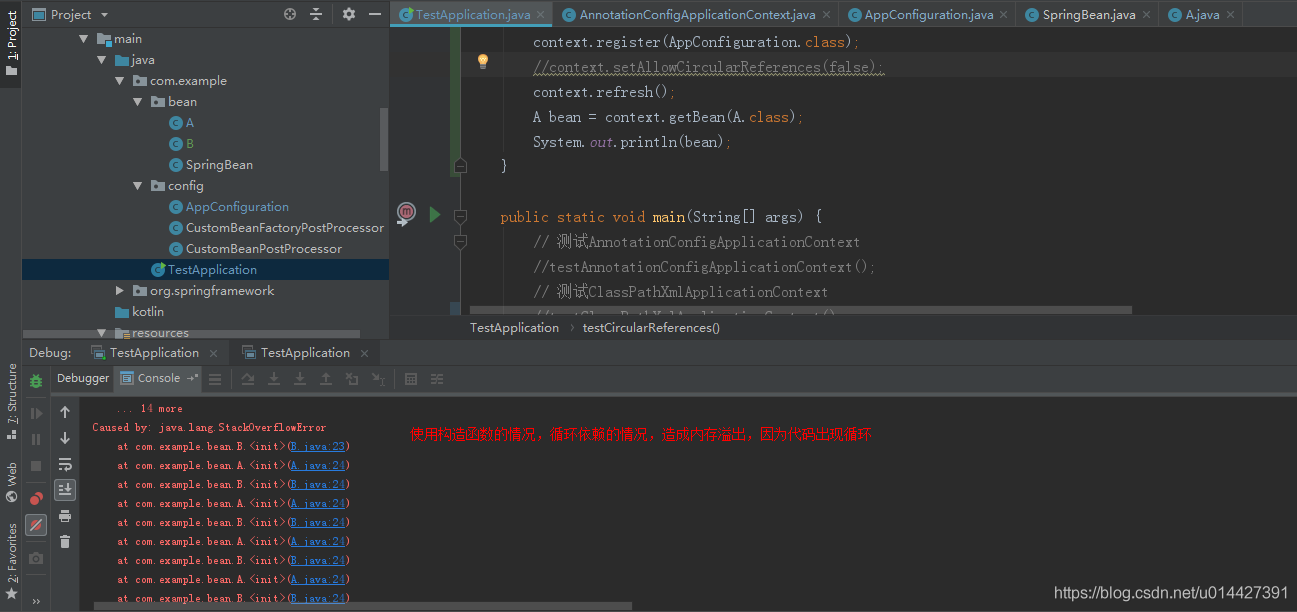
4、循环依赖解决方法
==============
对于这种情况,Spring 有处理方法?答案是有的,方法就是通过 @Autowired 注解,当然 bean 要是单例的,多例的情况不支持,原因后面分析
@Component
public class A {
@Autowired
B b;
public A() {
System.out.println("A class is create");
}
}
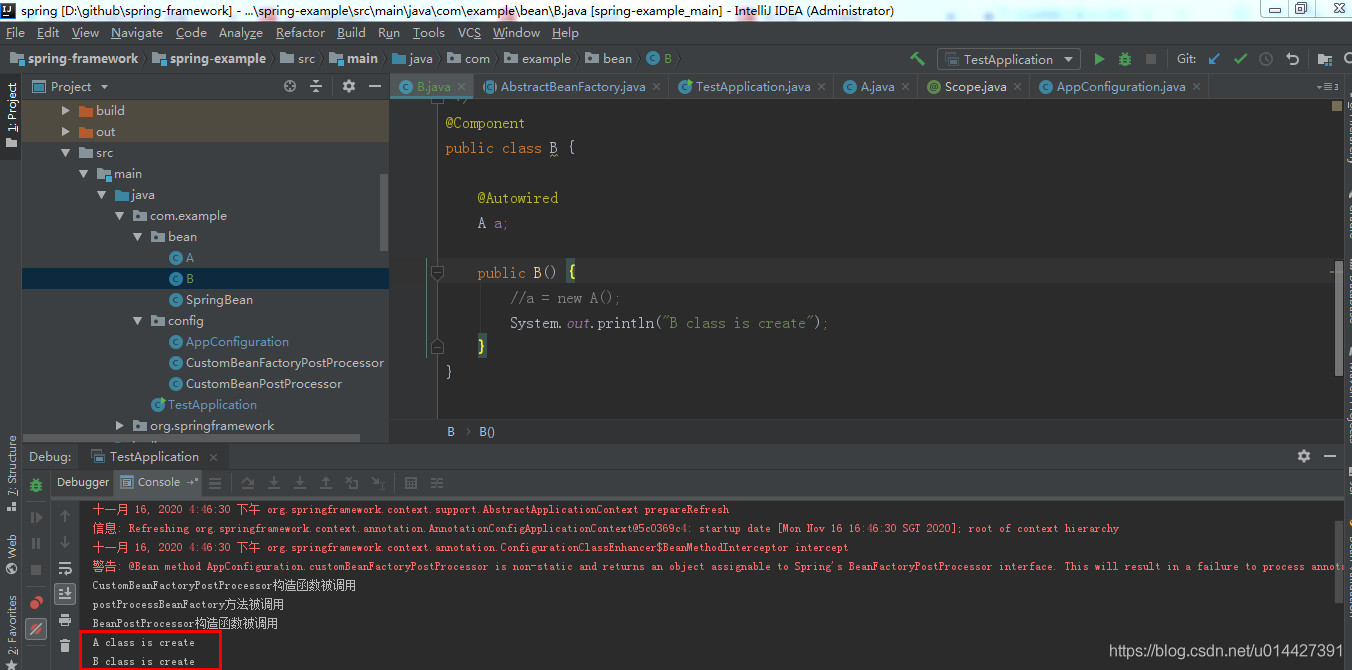
补充:除了 @Autowired 方法,我们还可以通过 set 方法处理循环依赖问题,当然也是仅支持单例 bean,多例的情况不支持
5、关闭 Spring 循环依赖
==================
有个疑问?Spring 的循环依赖支持,默认情况是开启?是否有什么开关控制?通过源码学习,可以通过 setAllowCircularReferences 设置
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext();
context.register(AppConfiguration.class);
// 关闭 Spring 循环依赖支持
context.setAllowCircularReferences(false);
context.refresh();
通过测试,设置不开启这个属性的时候,即使加上 @Autowired,代码还是抛异常了
6、prototype(多例)循环依赖
=======================
在多例的情况,Spring 能支持循环依赖?加上 @Scope("prototype"),将 bean 变成多例的
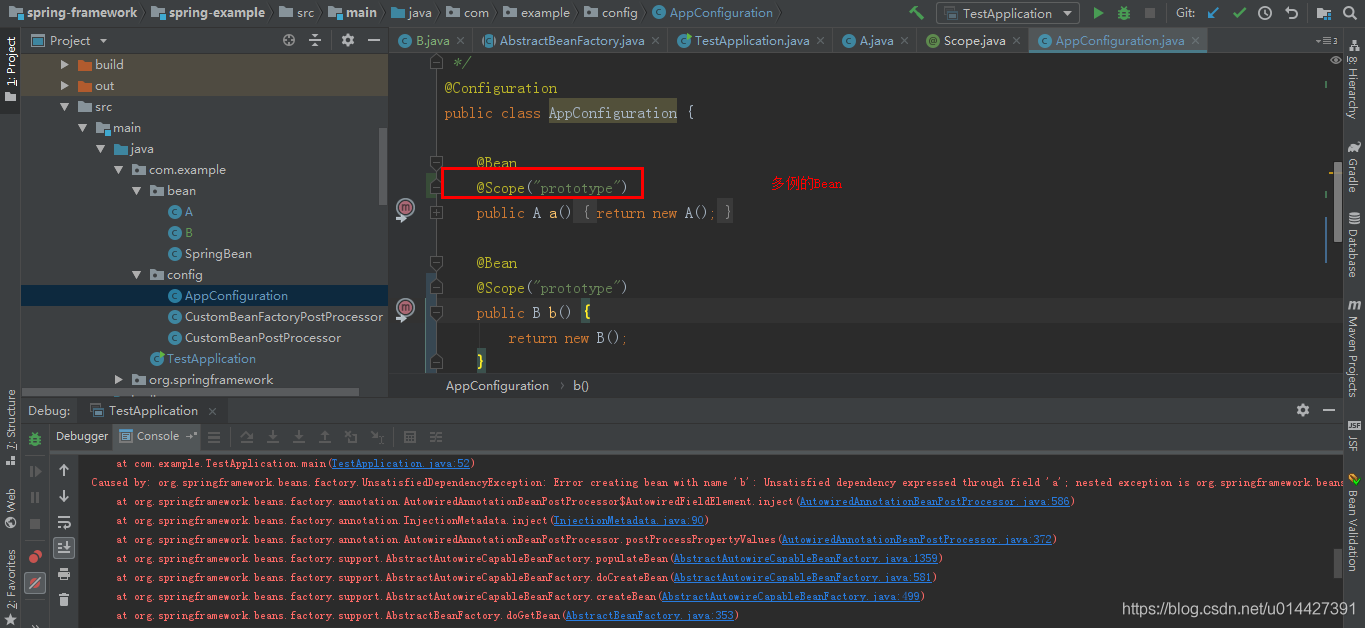
经过测试:多例的情况会抛出异常,即使加上了 @Autowired,原因请看下文
7、Spring 循环依赖特征
==================
ok,经过前面例子的验证,到这来,可以对 Spring 的循环依赖特点进行归纳:
Spring 中的循环依赖场景构造器的循环依赖,通过构造函数 Field 属性的循环依赖,通过 set 方法
Spring 的循环依赖是默认开启的(setAllowCircularReferences)
Spring 对单例和多例 Bean 的支持单例 Bean(singleton) :只能通过 @Autowired 和 set 方法支持多例 Bean(prototype):默认不支持,直接抛异常 BeanCurrentlyInCreationException
8、Spring 循环依赖原理
==================
我们通过实验进行了验证,也归纳出了 Spring 循环依赖的特点,然后具体原因是什么?我们只能通过源码学习得到答案
在上一章的学习中,我们对 Bean 的创建有了一个粗略的了解,所以,顺着这条路线,跟下源码:
在前面的学习,我们知道了{@link org.springframework.beans.factory.support.AbstractBeanFactory#doGetBean}这个方法就是 Spring Bean 创建的真正执行方法
protected <T> T doGetBean(
String name, @Nullable Class<T> requiredType, @Nullable Object[] args, boolean typeCheckOnly)
throws BeansException {
// 处理 BeanName,前面说的 FactoryBean 带‘&’符号,要在这里进行转换
String beanName = transformedBeanName(name);
Object bean;
// Eagerly check singleton cache for manually registered singletons.
// 从 map(singletonObjects)里获取单例 bean,确定是否已经存在对应实例
Object sharedInstance = getSingleton(beanName);
if (sharedInstance != null && args == null) {
if (logger.isDebugEnabled()) {
if (isSingletonCurrentlyInCreation(beanName)) {
logger.debug("Returning eagerly cached instance of singleton bean '" + beanName +
"' that is not fully initialized yet - a consequence of a circular reference");
}
else {
logger.debug("Returning cached instance of singleton bean '" + beanName + "'");
}
}
// 两种情况:普通的 bean,直接从 singletonObjects 返回 sharedInstance
//如果是 FactoryBean,返回其创建的对象实例
bean = getObjectForBeanInstance(sharedInstance, name, beanName, null);
}
else {
// Fail if we're already creating this bean instance:
// We're assumably within a circular reference.
// 校验是否是多例(Prototype)的 Bean,多例的 bean 是不支持循环依赖的
// 为了避免循环依赖,遇到这种情况,直接抛出异常
if (isPrototypeCurrentlyInCreation(beanName)) {
throw new BeanCurrentlyInCreationException(beanName);
}
// Check if bean definition exists in this factory.
// 检查 BeanFactory 是否存在这个 BeanDefinition
BeanFactory parentBeanFactory = getParentBeanFactory();
if (parentBeanFactory != null && !containsBeanDefinition(beanName)) {
// Not found -> check parent.
// 当前容器找不到 BeanDefinition,去 parent 容器查询
String nameToLookup = originalBeanName(name);
if (parentBeanFactory instanceof AbstractBeanFactory) {
return ((AbstractBeanFactory) parentBeanFactory).doGetBean(
nameToLookup, requiredType, args, typeCheckOnly);
}
else if (args != null) {
// Delegation to parent with explicit args.
// 返回 parent 容器的查询结果
return (T) parentBeanFactory.getBean(nameToLookup, args);
}
else {
// No args -> delegate to standard getBean method.
return parentBeanFactory.getBean(nameToLookup, requiredType);
}
}
if (!typeCheckOnly) {
//typeCheckOnly 为 false 的情况,将 beanName 放在一个 alreadyCreated 的集合
markBeanAsCreated(beanName);
}
try {
RootBeanDefinition mbd = getMergedLocalBeanDefinition(beanName);
checkMergedBeanDefinition(mbd, beanName, args);
// Guarantee initialization of beans that the current bean depends on.
// 校验是否配置了 depends-on
String[] dependsOn = mbd.getDependsOn();
if (dependsOn != null) {
for (String dep : dependsOn) {
// 存在循环引用的情况,要抛出异常
if (isDependent(beanName, dep)) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"Circular depends-on relationship between '" + beanName + "' and '" + dep + "'");
}
// 正常情况,注册依赖关系
registerDependentBean(dep, beanName);
try {
// 初始化被依赖项
getBean(dep);
}
catch (NoSuchBeanDefinitionException ex) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"'" + beanName + "' depends on missing bean '" + dep + "'", ex);
}
}
}
// Create bean instance.
// 单例的 Bean
if (mbd.isSingleton()) {
sharedInstance = getSingleton(beanName, () -> {
try {
// 创建单例 bean
return createBean(beanName, mbd, args);
}
catch (BeansException ex) {
// Explicitly remove instance from singleton cache: It might have been put there
// eagerly by the cre
ation process, to allow for circular reference resolution.
// Also remove any beans that received a temporary reference to the bean.
destroySingleton(beanName);
throw ex;
}
});
bean = getObjectForBeanInstance(sharedInstance, name, beanName, mbd);
}
// 多例的 Bean,scope = protoType
else if (mbd.isPrototype()) {
// It's a prototype -> create a new instance.
Object prototypeInstance = null;
try {
// 多例的情况,创建 bean 之前添加标记(用于循环依赖校验)
beforePrototypeCreation(beanName);
// 执行多例 Bean 创建
prototypeInstance = createBean(beanName, mbd, args);
}
finally {
// 创建原型(多例)bean 之后擦除标记
afterPrototypeCreation(beanName);
}
bean = getObjectForBeanInstance(prototypeInstance, name, beanName, mbd);
}
// 如果不是单例 bean 也不是多例的 bean,委托给对应的实现类
else {
String scopeName = mbd.getScope();
if (!StringUtils.hasLength(scopeName)) {
throw new IllegalStateException("No scope name defined for bean ′" + beanName + "'");
}
Scope scope = this.scopes.get(scopeName);
if (scope == null) {
throw new IllegalStateException("No Scope registered for scope name '" + scopeName + "'");
}
try {
Object scopedInstance = scope.get(beanName, () -> {
beforePrototypeCreation(beanName);
try {
// 执行 bean 创建
return createBean(beanName, mbd, args);
}
finally {
afterPrototypeCreation(beanName);
}
});
bean = getObjectForBeanInstance(scopedInstance, name, beanName, mbd);
}
catch (IllegalStateException ex) {
throw new BeanCreationException(beanName,
"Scope '" + scopeName + "' is not active for the current thread; consider " +
"defining a scoped proxy for this bean if you intend to refer to it from a singleton",
ex);
}
}
}
catch (BeansException ex) {
cleanupAfterBeanCreationFailure(beanName);
throw ex;
}
}
// Check if required type matches the type of the actual bean instance.
// 检查一下类型是否正确,不正确抛出异常,正确返回实例
if (requiredType != null && !requiredType.isInstance(bean)) {
try {
T convertedBean = getTypeConverter().convertIfNecessary(bean, requiredType);
if (convertedBean == null) {
throw new BeanNotOfRequiredTypeException(name, requiredType, bean.getClass());
}
return convertedBean;
}
catch (TypeMismatchException ex) {
if (logger.isDebugEnabled()) {
logger.debug("Failed to convert bean '" + name + "' to required type '" +
ClassUtils.getQualifiedName(requiredType) + "'", ex);
}
throw new BeanNotOfRequiredTypeException(name, requiredType, bean.getClass());
}
}
return (T) bean;
}
源码比较复杂,所以可以带着疑问来跟,首先以单例 Bean 的情况:#doGetBean.getSingleton
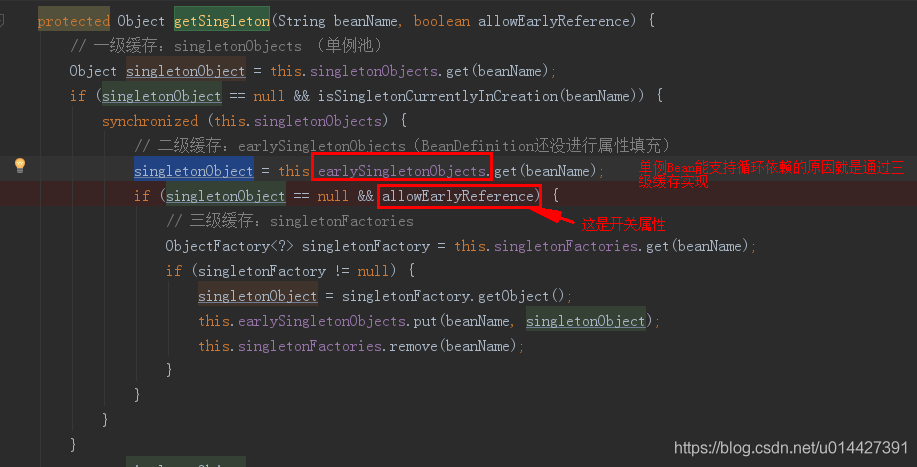
protected Object getSingleton(String beanName, boolean allowEarlyReference) {
// 一级缓存:singletonObjects (单例池)
Object singletonObject = this.singletonObjects.get(beanName);
if (singletonObject == null && isSingletonCurrentlyInCreation(beanName)) {
synchronized (this.singletonObjects) {
// 二级缓存:earlySingletonObjects(BeanDefinition 还没进行属性填充)
singletonObject = this.earlySingletonObjects.get(beanName);
if (singletonObject == null && allowEarlyReference) {
// 三级缓存:singletonFactories
ObjectFactory<?> singletonFactory = this.singletonFactories.get(beanName);
if (singletonFactory != null) {
singletonObject = singletonFactory.getObject();
this.earlySingletonObjects.put(beanName, singletonObject);
this.singletonFactories.remove(beanName);
}
}
}
}
return singletonObject;
}
在某些情况,循环依赖会造成循环调用,所以需要怎么解决?
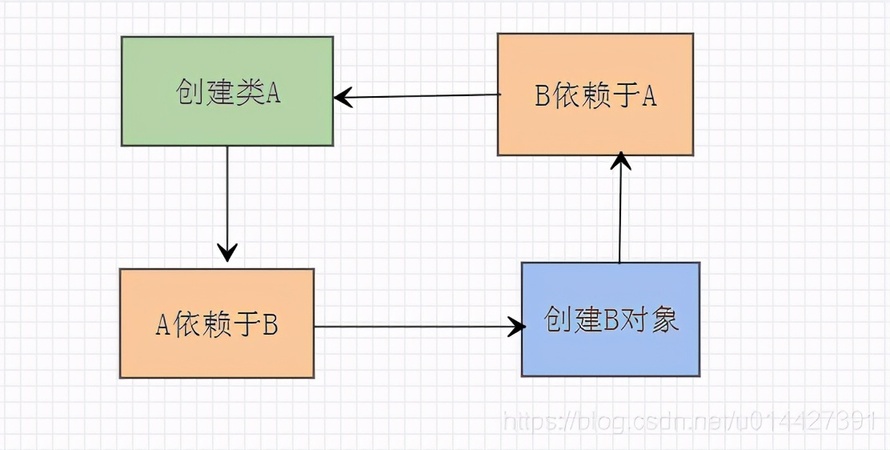
Spring 框架的方法是使用了三级缓存,其实最关键的是 earlySingletonObjects
一级缓存:singletonObjects,这是 Spring BeanDefinition 的单例池,首先只保存单例 Bean 的 BeanDefinition,而且这个 Bean 是一个真正的 bean,也就是进行过属性填充的
二级缓存:earlySingletonObjects,early 从单词意思来说,这个缓存是在 singletonObjects 之前的,也就是 BeanDefinition 还没进行属性填充等等操作,Spring 引入这个缓存的目的就是为了处理单例 bean 的循环依赖问题
三级缓存:singletonFactories,缓存的是 ObjectFactory,表示对象工厂,为什么要加上这个缓存?原因比较复杂,涉及到 AOP 等等原因,因为我还没理解清楚,所以本文不说明
加上了 earlySingletonObjects 缓存之后,Spring 就能支持单例 bean 的循环依赖,参考语雀某大佬的笔记,画图表示:
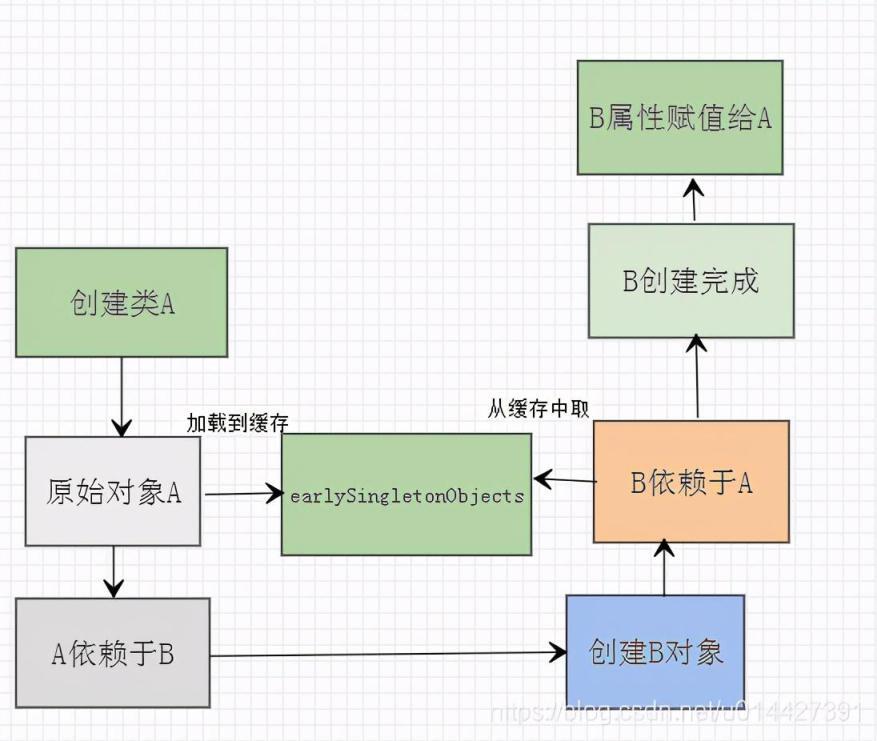
带着疑问来跟一下多例 Bean 的情况:
Spring 框架是不支持多例 bean 的循环依赖的,原因跟下代码:#doGetBean
// Fail if we're already creating this bean instance:
// We're assumably within a circular reference.
// 校验是否是多例(Prototype)的 Bean,多例的 bean 是不支持循环依赖的
// 为了避免循环依赖,遇到这种情况,直接抛出异常
if (isPrototypeCurrentlyInCreation(beanName)) {
throw new BeanCurrentlyInCreationException(beanName);
评论