Bmob 后端云 +ImageLoader 框架实现图文列表
dependencies {
classpath 'com.android.tools.build:gradle:3.3.1'
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
google()
jcenter()
//Bmob 的 maven 仓库地址--必填
maven { url "https://raw.github.com/bmob/bmob-android-sdk/master" }
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
2.在 app 的 build.gradle 文件中添加依赖文件
dependencies {
implementation fileTree(include: ['*.jar'], dir: 'libs')
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'com.android.support.test:runner:1.0.2'
androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2'
implementation 'cn.bmob.android:bmob-sdk:3.6.9'
implementation 'io.reactivex.rxjava2:rxjava:2.2.2'
implementation 'io.reactivex.rxjava2:rxandroid:2.1.0'
implementation 'com.squareup.okio:okio:2.1.0'
implementation 'com.google.code.gson:gson:2.8.5'
implementation 'com.squareup.okhttp3:okhttp:3.12.0'
implementation 'com.nostra13.universalimageloader:universal-image-loader:1.9.5'
}
配置 AndroidManifest.xml
在你的应用程序的 AndroidManifest.xml 文件中添加相应的权限:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
配置 ContentProvider
同样在的 AndroidManifest.xml 文件中添加相应的权限:
<provider
android:name="cn.bmob.v3.util.BmobContentProvider"
android:authorities="com.example.list_dome.BmobContentProvider">
</provider>
初始化 BmobSDK
在应用的 onCreate 方法中初始化 BmobSDK
Bmob.initialize(this, "4943e6a9dd93e0df1aee0fc6d54239d9");
新建 list 表
对以上都配置完成以后,请到应用的数据库中新建 list 表,此表中有两列分别为
(列名:name 属性:String 描述:每列的文字信息)
(列名:icon 属性:File 描述:每列的)
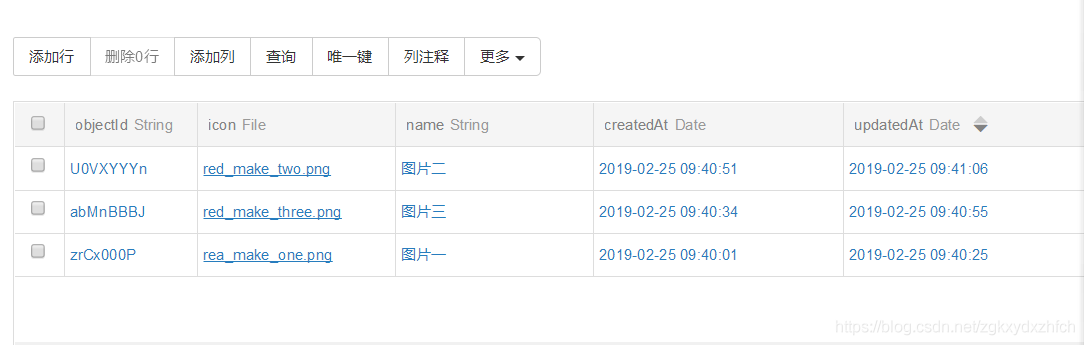
3.2 在项目中新建 list 类
新建的 list 类应与在 Bmob 云后端建立 list 表一 一对应
public class list extends BmobObject {
private String name;
private BmobFile icon;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public BmobFile getIcon() {
return icon;
}
public String getIconUrl(){
return icon.getFileUrl();
}
public void setIcon(BmobFile icon) {
this.icon = icon;
}
}
其中类中的 BmobFile 对应表中的 File,代码块中的 getIconUrl()方法用来获取图片的 url。
3.3 在项目中导入 ImageLoader 框架
第一种方法:导入 imageloader 的 jar 包
第二种方法:在 Android Studio 中导入依赖使用快捷键 Alert+ctrl+shift+s,然后点击 Dependencies 再点击右面的“+”号选择“Library dependency”,把 com.nostra13.universalimageloader:universal-image-loader:1.9.5 复制在输入框中。
缓存图片配置(初始化 ImageLoader)
imageLoader.init(ImageLoaderConfiguration.createDefault(MainActivity.this));
3.4 编写 ListView 适配器
以下代码是对 ListView 适配器进行的编写,如果想要了解详细的 ListView 适配器请点击链接[
点击跳转到 ListView 适配器详细编写博客]( )
实例化 ImageLoader 对象
// 创建 ImageLoader 对象
private ImageLoader imageLoader = ImageLoader.getInstance();
主页面的布局文件
<?xml version="1.0" encoding="utf-8"?>
<Relat
iveLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:paddingBottom="16dp"
android:paddingTop="16dp">
<ListView
android:id="@+id/main_list_view"
android:layout_width="match_parent"
android:layout_height="match_parent">
</ListView>
</RelativeLayout>
列表每项布局文件
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="100dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="10dp">
<ImageView
android:id="@+id/title_pic"
android:layout_width="80dp"
android:layout_height="60dp"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:src="@mipmap/ic_launcher"/>
<TextView
android:id="@+id/title_content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:textSize="16sp"
android:layout_marginLeft="10dp"
android:layout_toRightOf="@id/title_pic"
/>
</RelativeLayout>
</RelativeLayout>
适配器编写
//适配器用来进行视图与数据的适配作用
public class NewListAdapter extends BaseAdapter {
private List<list> lists= new ArrayList<>();
public NewListAdapter(List<list> list) {
this.lists=list;
}
@Override
public int getCount() {
return lists.size();
}
@Override
public Object getItem(int position) {
return lists.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(final int position, View convertView, ViewGroup parent) {
ViewHolder viewHolder = new ViewHolder();
if (convertView == null) {
LayoutInflater layoutInflater = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = layoutInflater.inflate(R.layout.item_news_list, null);
viewHolder.titleContent = (TextView) convertView.findViewById(R.id.title_content);
viewHolder.icon = (ImageView) convertView.findViewById(R.id.title_pic);
convertView.setTag(viewHolder);
}else {
viewHolder = (ViewHolder) convertView.getTag();
}
// 创建 DisplayImageOptions 对象并进行相关选项配置
DisplayImageOptions options = new DisplayImageOptions.Builder()
.showImageOnLoading(R.drawable.ic_launcher_background)// 设置图片下载期间显示的图片
.showImageForEmptyUri(R.drawable.ic_launcher_background)// 设置图片 Uri 为空或是错误的时候显示的图片
.showImageOnFail(R.drawable.ic_launcher_background)// 设置图片加载或解码过程中发生错误显示的图片
.cacheInMemory(true)// 设置下载的图片是否缓存在内存中
.cacheOnDisk(true)// 设置下载的图片是否缓存在 SD 卡中
.displayer(new RoundedBitmapDisplayer(20))// 设置成圆角图片
.build();// 创建 DisplayImageOptions 对象
// 使用 ImageLoader 加载图片
imageLoader.displayImage(lists.get(position).getIcon().getFileUrl(),viewHolder.icon);
viewHolder.titleContent.setText(lists.get(position).getName());
return convertView;
}
public class ViewHolder{
TextView titleContent;
ImageView icon;
}
}
其中 displayImage(参数 1,参数 2)方法用来加载图片,参数 1 为想要加载图片的 url(图片的 url 通过 getFileUrl()方法获取),参数 2 为 ImageView 控件。
重写 onDestroy()方法回收缓存在内存中的图片
@Override
protected void onDestroy() {
// 回收该页面缓存在内存中的图片
评论