我看 JAVA 之 并发编程【一】
发布于: 1 小时前
我看 JAVA 之 并发编程【一】
线程与进程
进程是资源分配的最小单位,线程是 CPU 调度的最小单位
进程:白话说就是计算机中运行的一个程序,一个进程可以包含多个线程。
线程:是操作系统能够进行运算调度的最小单位,包含在进程之中,是进程中的实际运作单位。
Callable
每当大家面试的时候,经常会被问道实现多线程有几种方式?一般都会说两种:继承 Thread 类 or 实现 Runnable 接口。那么,还有人说通过实现 Callable 的 call 接口 也可以实现多线程,并且还可有返回值!这是为什么呢?让我们一探究竟。
FutureTask futureTask = new FutureTask(new Callable() {
@Override
public String call() throws Exception {
System.out.println("hello...");
return "call return";
}
});
futureTask.run();
复制代码
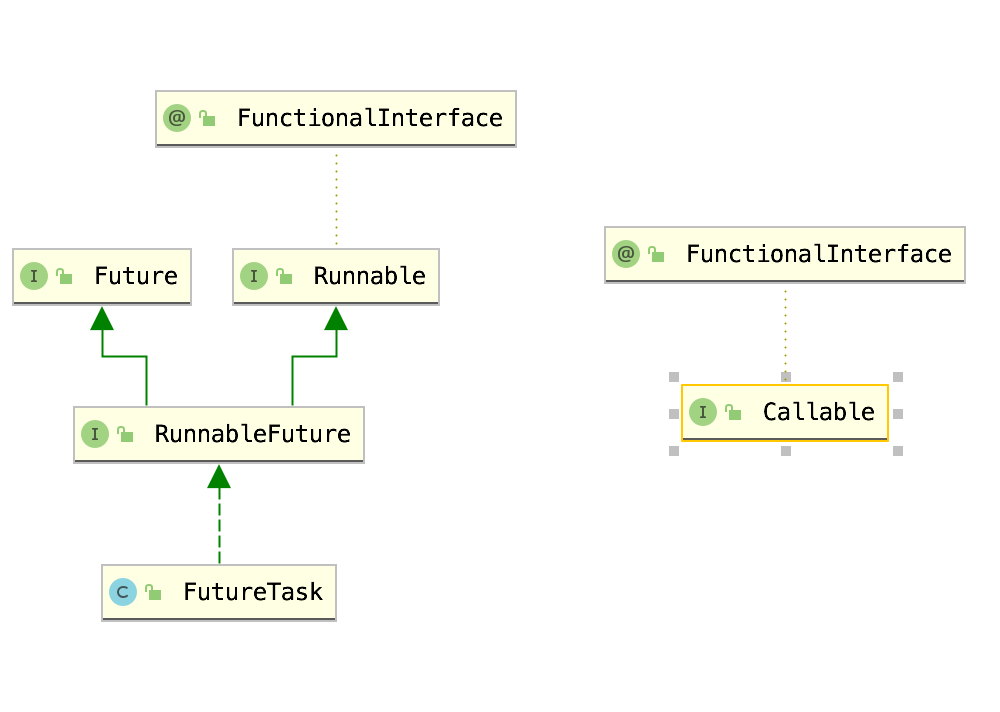
通过上面的类图发现 FutureTask 间接实现了 Runnable 接口,那是不是说 Callable 多线程方案实际还是 Runnable 方案呢?接下来只能看 FutureTask 的源码了。
public class FutureTask<V> implements RunnableFuture<V> {
/**
* 任务的状态
*
* Possible state transitions:
* NEW -> COMPLETING -> NORMAL
* NEW -> COMPLETING -> EXCEPTIONAL
* NEW -> CANCELLED
* NEW -> INTERRUPTING -> INTERRUPTED
*/
private volatile int state; //任务状态,volatile
private static final int NEW = 0;
private static final int COMPLETING = 1;
private static final int NORMAL = 2;
private static final int EXCEPTIONAL = 3;
private static final int CANCELLED = 4;
private static final int INTERRUPTING = 5;
private static final int INTERRUPTED = 6;
/** callable,实际的业务在callable.call()方法中 */
private Callable<V> callable;
/** outcome为callable.call()的返回值接收对象,通过get()获得 */
private Object outcome; // non-volatile, protected by state reads/writes
/** The thread running the callable; CASed during run() */
private volatile Thread runner;
/** Treiber stack of waiting threads */
private volatile WaitNode waiters;
复制代码
run()方法,核心逻辑
/**
* 核心还是Runnable接口的run方法
* run方法内部调用callable的call方法,执行业务
* 最后将call方法的返回值set到FutureTask的outcome属性
*/
public void run() {
//1. 进入run方法,cas改变任务状态
if (state != NEW ||
!RUNNER.compareAndSet(this, null, Thread.currentThread()))
return;
try {
Callable<V> c = callable;
if (c != null && state == NEW) {
V result;
boolean ran;
try {
result = c.call();//2. 调用callable的call方法
ran = true;
} catch (Throwable ex) {
result = null;
ran = false;
setException(ex);
}
if (ran)
set(result);//3. 设置结果给FutureTask的outcome属性
}
} finally {
// runner must be non-null until state is settled to
// prevent concurrent calls to run()
runner = null;
// state must be re-read after nulling runner to prevent
// leaked interrupts
int s = state;
if (s >= INTERRUPTING)
handlePossibleCancellationInterrupt(s);
}
}
复制代码
等待结束或打断 or 超时终止,如下:
/**
* Awaits completion or aborts on interrupt or timeout.
* 等待结束或打断or超时终止
*/
private int awaitDone(boolean timed, long nanos)
long startTime = 0L; // Special value 0L means not yet parked
WaitNode q = null;
boolean queued = false;
for (;;) {//死循环
int s = state;
if (s > COMPLETING) {
if (q != null)
q.thread = null;
return s;
}
else if (s == COMPLETING)
// We may have already promised (via isDone) that we are done
// so never return empty-handed or throw InterruptedException
Thread.yield();
else if (Thread.interrupted()) {// interrupt打断
removeWaiter(q);
throw new InterruptedException();
}
else if (q == null) {
if (timed && nanos <= 0L)
return s;
q = new WaitNode();
}
else if (!queued)
queued = WAITERS.weakCompareAndSet(this, q.next = waiters, q); //加入到WAITERS
else if (timed) { //使用超时等待
final long parkNanos;
if (startTime == 0L) { // first time
startTime = System.nanoTime();
if (startTime == 0L)
startTime = 1L;
parkNanos = nanos;
} else {
long elapsed = System.nanoTime() - startTime;
if (elapsed >= nanos) {
removeWaiter(q);
return state;
}
parkNanos = nanos - elapsed;
}
// nanoTime may be slow; recheck before parking
if (state < COMPLETING)
LockSupport.parkNanos(this, parkNanos);
}
else
LockSupport.park(this);
}
}
复制代码
通过以上代码分析,Callable 接口实现多线程,仍然是实现 Runnable 接口的方式来实现,Callable.call()仅仅是提供业务处理的封装,核心应该关注 FutureTask。
划线
评论
复制
发布于: 1 小时前阅读数: 4
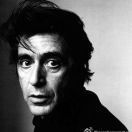
awen
关注
Things happen for a reason. 2019.11.15 加入
还未添加个人简介
评论