《项目开发团队分配管理软件》,nginx 面试题阿里
--------------------团队成员列表---------------------
TDI/ID ?姓名 ? ?年龄 ? ? ?工资 ? ? ? 职位 ? ? ?奖金 ? ? ? ?股票 ?
2/4 ? ? 刘强东 ? 24 ? ? ?7300.0 ? ?程序员 ?
3/2 ? ? 马化腾 ? 32 ? ? ?18000.0 ?架构师 ? 15000.0 ?2000 ?
4/6 ? ? 任志强 ? 22 ? ? ?6800.0 ? ?程序员 ?
5/12 ? 杨致远 ? 27 ? ? ?600.0 ? ? ?设计师 ? 4800.0
-----------------------------------------------------
TeamService 类的设计:
功能:关于开发团队成员的管理:添加、删除等(还需要自行实现)。
说明:
counter 为静态变量,用来为开发团队新增成员自动生成团队中的唯一 ID,即 memberId。(提示:应使用增 1 的方式)
MAX_MEMBER:表示开发团队最大成员数
team 数组:用来保存当前团队中的各成员对象 (也可以用一个新的集合)
total:记录团队成员的实际人数
TeamService 类的设计:
getTeam()方法:返回当前团队的所有对象
返回:包含所有成员对象的数组,数组大小与成员人数一致
addMember(e: Employee)方法:向团队中添加成员
参数:待添加成员的对象
异常:添加失败, TeamException 中包含了失败原因
removeMember(memberId: int)方法:从团队中删除成员
参数:待删除成员的 memberId
异常:找不到指定 memberId 的员工,删除失败
另外,可根据需要自行添加其他方法或重载构造器
TeamView 类的设计
说明:
listSvc 和 teamSvc 属性:供类中的方法使用
enterMainMenu ()方法:主界面显示及控制方法。
以下方法仅供 enterMainMenu()方法调用:
listAllEmployees ()方法:以表格形式列出公司所有成员
getTeam()方法:显示团队成员列表操作
addMember ()方法:实现添加成员操作
deleteMember ()方法:实现删除成员操作?
4.开发项目管理模块
在 domain 包中完成项目实体类 Project 的创建
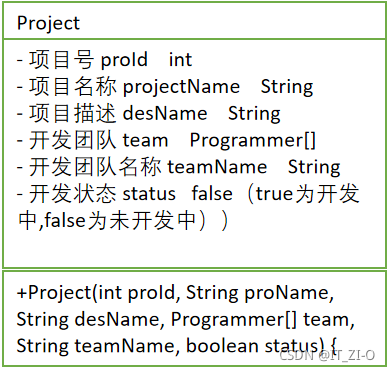
在 service 包中完成项目操作类 ProjectService 的创建
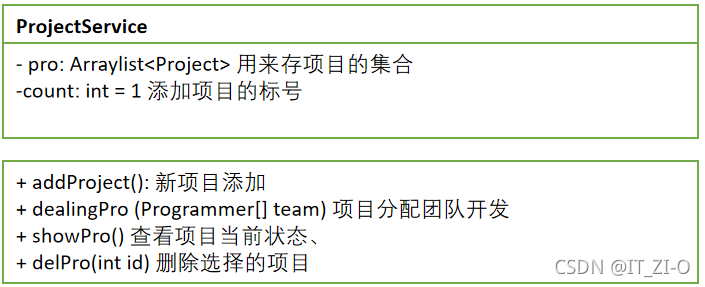
其他还需要的方法属性可自行添加
IndexView 类的设计?
最后在 view 包中编写项目程序运行主界面类 IndexView
将前面 4 个模块的内容装在一起,并运行软件,操作基本功能,调试 bug,项目开发完成。
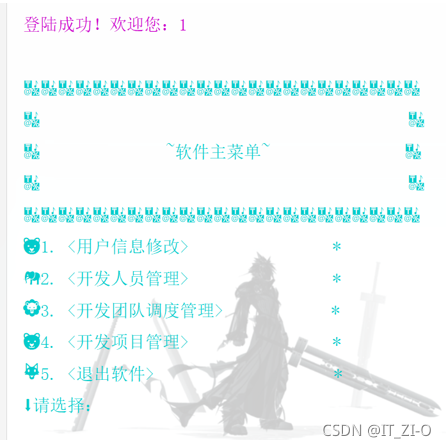
五、架构展开
======
如果没有参考代码的话,对于我们应该如何进行展开呢?
让我们来看看----系统功能结构:
很一目了然,我们能看出,我们需要做的就是创建四个模块,最后收成一个整体,让各个子体关联上,文本运行正常无 BUG 就完成了。
所以我建议的是,一个模块一个模块的做,然后做好一个用 main 方法测试通过,最后想办法凑一堆,让它跑!!!!
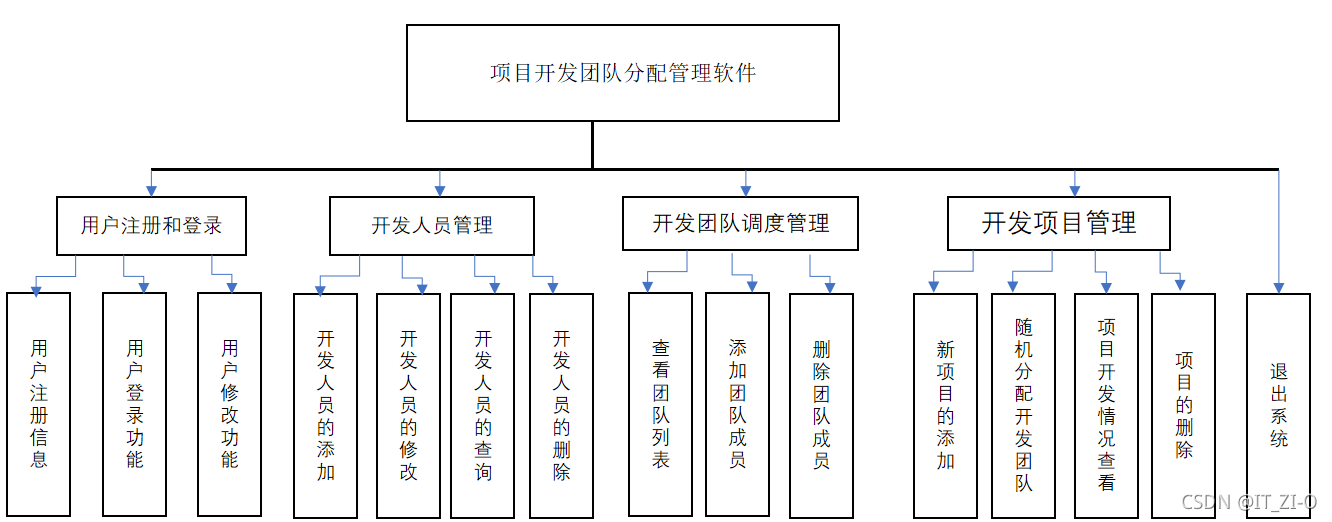
系统流程:
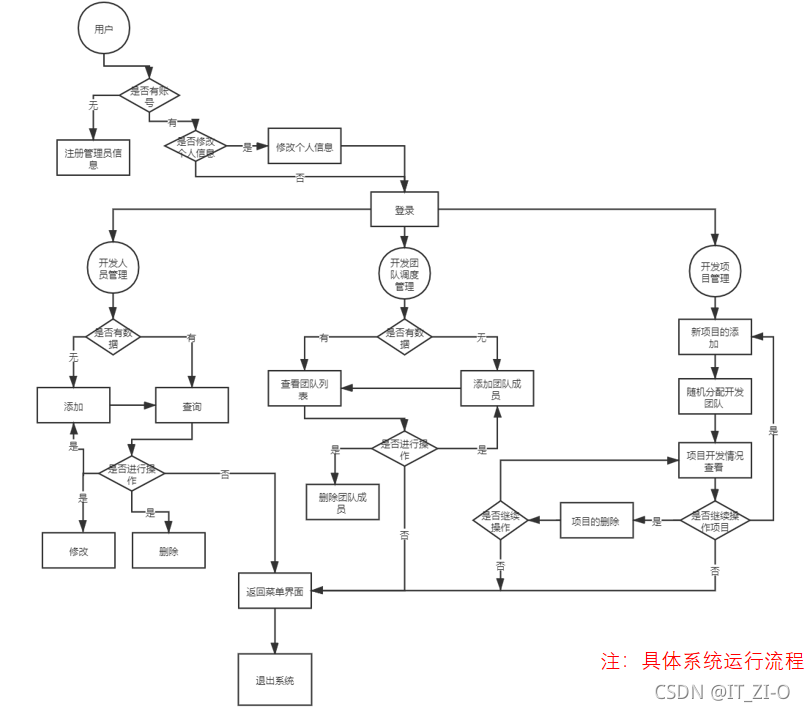
六、代码实现
======
1、domain 包下:
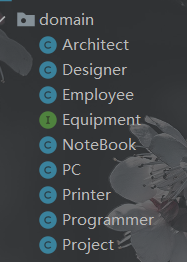
Employee(雇员父类):
package domain;
/*
*雇员类
*/
public class Employee{
public int id;
public String name;
public int age;
public double salary;//工资
public Employee() {
}
public Employee(int id, String name, int age, double salary) {
this.id = id;
this.name = name;
this.age = age;
this.salary = salary;
}
//构造器
public void setId(int id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
this.age = age;
}
public void setSalary(double salary) {
this.salary = salary;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public double getSalary() {
return salary;
}
//方法
public String getDetails(){
return id+"\t"+name+"\t"+" "+age+"\t\t"+salary;
}
@Override
public String toString() {
return getDetails();
}
}
programmer(程序员类):
package domain;
/*
*程序员类
*/
public class Programmer extends Employee {
private int memberld;//用来记录成员加入开发团队后在团队中的 ID
private boolean status = true;//Status 是项目中人员的状态,先赋值为 true,当添加到团队时为 false
public Equipment equipment;
public Programmer(int id, String name, int age, double salary, Equipment equipment) {
super(id,name,age,salary);
this.equipment=equipment;
}
public Programmer(int id, String name, int age, double salary, int memberld, boolean status, Equipment equipment) {
super(id, name, age, salary);
this.equipment = equipment;
}
public Programmer() {
}
public int getMemberId() {
return memberld;
}
public void setMemberId(int memberId) {
this.memberld = memberId;
}
public Boolean getStatus() {
return status;
}
public void setStatus(Boolean status) {
this.status = status;
}
public Equipment getEquipment() {
return equipment;
}
public void setEquipment(Equipment equipment) {
this.equipment = equipment;
}
protected String getMemberDetails() {
return getMemberId() + "/" + getDetails();
}
public String getDetailsForTeam() {
return getMemberDetails() + "\t 程序员";
}
@Override
public String toString() {
return getDetails() + "\t 程序员\t" + status + "\t\t\t\t\t" + equipment.getDescription();
}
}
Designer(设计师类):
package domain;
/*
*设计师
*/
public class Designer extends Programmer{
public double bonus;//奖金
public Designer() {
}
public Designer(int id, String name, int age, double salary, Equipment equipment, double bonus) {
super(id, name, age, salary, equipment);
this.bonus = bonus;
}
public double getBonus() {
return bonus;
}
public void setBonus(double bonus) {
this.bonus = bonus;
}
@Override
public String getDetailsForTeam() {
return getMemberDetails()+"\t 设计师\t"+getBonus();
}
@Override
public String toString() {
return getDetails() + "\t 设计师\t" + getStatus() + "\t" +
getBonus() +"\t\t\t" + getEquipment().getDescription();
}
}
Architect(架构师类):
package domain;
/*
*架构师类
*/
public class Architect extends Designer{
public int stock;//公司奖励的股票数量
public Architect(){
}
public Architect(int id, String name, int age, double salary, Equipment equipment, double bonus, int stock) {
super(id, name, age, salary, equipment, bonus);
this.stock = stock;
}
public int getStock() {
return stock;
}
public void setStock(int stock) {
this.stock = stock;
}
@Override
public String getDetailsForTeam() {
return getMemberDetails() + "\t 架构师\t" +
getBonus() + "\t" + getStock();
}
@Override
public String toString() {
return getDetails() + "\t 架构师\t" + getStatus() + "\t" +
getBonus() + "\t" + getStock() + "\t" + getEquipment().getDescription();
}
}
接口 Equipment(电子设备):
package domain;
/*
*接口
*/
public interface Equipment {//电子设备
String getDescription();//类型
}
接口实现类 Notebook(笔记本电脑):
package domain;
import view.TSUtility;
/*
*笔记本电脑
*/
public class NoteBook implements Equipment {
public String model;//机器的型号
public double price;//价格
public NoteBook() {
super();
}
public NoteBook(String model, double price) {
super();
this.model = model;
this.price = price;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public NoteBook addNoteBook(){
System.out.println("请输入需要配置的笔记本电脑的型号");
String model= TSUtility.readKeyBoard(10,false);
System.out.println("请输入需要配置的笔记本电脑的价格(不大于六位数)");
double price=TSUtility.readDouble();
System.out.println("设备添加成功!");
return new NoteBook(model,price);
}
@Override
public String getDescription() {
return model+"("+price+")";
}
}
接口实现类 PC(台式电脑):
package domain;
/*
台式电脑
*/
import view.TSUtility;
public class PC implements Equipment{
public String model;//机器型号
public String display;//显示器名称
public PC() {
super();
}
public PC(String model, String display) {
super();
this.model = model;
this.display = display;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public String getDisplay() {
return display;
}
public void setDisplay(String display) {
this.display = display;
}
public PC addPC(){
System.out.println("请输入你需要配置的台式电脑的型号");
String model= TSUtility.readKeyBoard(10,false);
System.out.println("请输入你需要配置的台式电脑的显示屏的名称");
String display=TSUtility.readKeyBoard(10,false);
System.out.println("设备添加成功!");
return new PC(model,display);
}
@Override
public String getDescription() {
return model+"("+display+")";
}
}
接口实现类 Printer(打印机):
package domain;
import view.TSUtility;
import java.time.format.TextStyle;
/*
*打印机
*/
public class Printer implements Equipment{
public String name;
public String type;//机器类型
public Printer() {
}
public Printer(String name, String type) {
this.name = name;
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public Printer addPrinter(){
System.out.println("请输入你需要配置的打印机名称");
String name= TSUtility.readKeyBoard(10,false);
System.out.println("请输入你需要配置的打印机机器类型");
String type=TSUtility.readKeyBoard(10,false);
System.out.println("设备配置成功!");
return new Printer(name,type);
}
@Override
public String getDescription() {
return name+"("+type+")";
}
}
项目实体类 project:
package domain;
/*
项目实体类
*/
import java.util.Arrays;
public class Project {
private int proId;//项目号
private String projectName;//项目名称
private String desName;//项目描述
private Programmer[] team;//开发团队
private String teamName;//开发团队名称
private boolean status=false;//开发状态(true 为开发中,false 为未开发)
public Project() {
}
public Project(int proId, String projectName, String desName, Programmer[] team, String teamName, boolean status) {
this.proId = proId;
this.projectName = projectName;
this.desName = desName;
this.team = team;
this.teamName = teamName;
this.status = status;
}
public int getProId() {
return proId;
}
public void setProId(int proId) {
this.proId = proId;
}
public String getProjectName() {
return projectName;
}
public void setProjectName(String projectName) {
this.projectName = projectName;
}
public String getDesName() {
return desName;
}
public void setDesName(String desName) {
this.desName = desName;
}
public Programmer[] getTeam() {
return team;
}
public void setTeam(Programmer[] team) {
this.team = team;
}
public String getTeamName() {
return teamName;
}
public void setTeamName(String teamName) {
this.teamName = teamName;
}
public boolean isStatus() {
return status;
}
public void setStatus(boolean status) {
this.status = status;
}
public String des(){
return "Project 项目{" +
"proId 项目号=" + proId +
", projectName 项目名称='" + projectName + ''' +
", desName 项目描述='" + desName + ''' +
", team 开发团队=" + Arrays.toString(team) +
", teamName 开发团队名称='" + teamName + ''' +
", status 开发团队状态=" + status +
'}';
}
@Override
public String toString() {
des();
if (status){
return "项目【" + projectName + "】"+ "---->正在被团队【" + teamName + "】开发中!";
}else {
return des()+"项目【" + projectName + "】---->"+ "未被开发!";
}
}
}
2、service 包下:
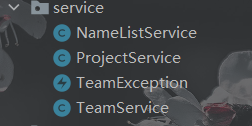
异常类 TeamException:
package service;
public class TeamException extends Exception{
public TeamException(){}
public TeamException(String message) {
super(message);
}
}
开发人员管理类 NameListService:
package service;
/*
开发人员管理模块
*/
import domain.*;
import view.TSUtility;
import java.util.ArrayList;
public class NameListService {
//装雇员的集合
private static ArrayList<Employee> employees = new ArrayList<Employee>();
//添加员工的 ID
private int count = 1;
//初始化默认值(代码块)
{
if(employees.isEmpty()){
employees.add(new Employee(count, "马云 ", 22, 3000));
employees.add(new Architect(++count,"马化腾",32,18000,new NoteBook("联想 T4",6000),60000,5000));
employees.add(new Programmer(++count, "李彦宏", 23, 7000, new PC("戴尔", "NEC 17 寸")));
employees.add(new Programmer(++count, "刘强东", 24, 7300, new PC("戴尔", "三星 17 寸")));
employees.add(new Designer(++count, "雷军 ", 50, 10000, new Printer("激光", "佳能 2900"), 5000));
employees.add(new Programmer(++count, "任志强", 30, 16800, new PC("华硕", "三星 17 寸")));
employees.add(new Designer(++count, "柳传志", 45, 35500, new PC("华硕", "三星 17 寸"), 8000));
employees.add(new Architect(++count, "杨元庆", 35, 6500, new Printer("针式", "爱普生 20k"), 15500, 1200));
employees.add(new Designer(++count, "史玉柱", 27, 7800, new NoteBook("惠普 m6", 5800), 1500));
employees.add(new Programmer(++count, "丁磊 ", 26, 6600, new PC("戴尔", "NEC17 寸")));
employees.add(new Programmer(++count, "张朝阳 ", 35, 7100, new PC("华硕", "三星 17 寸")));
employees.add(new Designer(++count, "杨致远", 38, 9600, new NoteBook("惠普 m6", 5800), 3000));
}
}
public NameListService() {
}
public NameListService(ArrayList<Employee> employees, int count) {
this.employees = employees;
this.count = count;
}
public ArrayList<Employee> getEmployees() {
return employees;
}
public void setEmployees(ArrayList<Employee> employees) {
this.employees = employees;
}
public int getCount() {
return count;
}
public void setCount(int count) {
this.count = count;
}
//得到所有员工数据集合
public ArrayList<Employee> getAllEmployees() {
return employees;
}
//得到当前员工
public Employee getEmployee(int id) throws TeamException {
for (int i = 0; i < employees.size(); i++) {
if(employees.get(i).getId() == id){
return employees.get(i);
}
}
throw new TeamException("该员工不存在");
}
public void addEmployee(){
System.out.println("请输入需要添加的雇员的职位:");
System.out.println("1(无职位)");
System.out.println("2(程序员)");
System.out.println("3(设计师)");
System.out.println("4(架构师)");
String a=String.valueOf(TSUtility.readMenuSelection());
if(a.equals("1")){//无职位
System.out.println("当前雇员职位分配为:无");
System.out.println("请输入当前雇员的姓名:");
String name=TSUtility.readKeyBoard(4,false);
System.out.println("请输入当前雇员的年龄:");
int age=TSUtility.readInt();
System.out.println("请输入当前雇员的工资:");
double salary=TSUtility.readDouble();
Employee employee=new Employee(++count,name,age,salary);
employees.add(employee);
System.out.println("人员添加成功!");
TSUtility.readReturn();
}else if(a.equals("2")){//程序员
System.out.println("当前雇员的职位分配为:程序员");
System.out.println("请输入当前雇员的姓名:");
String name=TSUtility.readKeyBoard(4,false);
System.out.println("请输入当前雇员的年龄:");
int age=TSUtility.readInt();
System.out.println("请输入当前雇员的工资:");
double salary=TSUtility.readDouble();
System.out.println("请为当前雇员配置一台好的台式电脑");
PC pc=new PC().addPC();
Programmer programmer=new Programmer(++count,name,age,salary,pc);
employees.add(programmer);
TSUtility.readReturn();
}else if(a.equals("3")){//设计师
System.out.println("当前雇员职位分配为:设计师");
System.out.println("请输入当前雇员的姓名:");
String name=TSUtility.readKeyBoard(4,false);
System.out.println("请输入当前雇员的年龄:");
int age=TSUtility.readInt();
System.out.println("请输入当前雇员的工资:");
double salary=TSUtility.readDouble();
System.out.println("请为当前设计师配置一台好的笔记本电脑:");
NoteBook noteBook=new NoteBook().addNoteBook();
System.out.println("请输入当前设计师的奖金:");
double bonus=TSUtility.readDouble();
Designer designer=new Designer(++count,name,age,salary,noteBook,bonus);
employees.add(designer);
System.out.println("人员添加成功!");
TSUtility.readReturn();
}else {//架构师
System.out.println("当前雇员的职位分配为:架构师");
System.out.println("请输入当前雇员的姓名:");
String name=TSUtility.readKeyBoard(4,false);
System.out.println("请输入当前雇员的年龄:");
int age=TSUtility.readInt();
System.out.println("请输入当前雇员的工资:");
double salary=TSUtility.readDouble();
System.out.println("请为当前架构师配置一台好的打印设备:");
Printer printer=new Printer().addPrinter();
System.out.println("请设置当前架构师的奖金:");
double bonus=TSUtility.readDouble();
System.out.println("请设置当前架构师的股票:");
Integer stock=TSUtility.readstock();
Architect architect=new Architect(++count,name,age,salary,printer,bonus,stock);
employees.add(architect);
System.out.println("人员添加成功!");
TSUtility.readReturn();
}
}
//员工的删除
public void delEmployee(int id) throws TeamException {
boolean flag=false;
for (int i = 0; i < employees.size(); i++) {
if(employees.get(i).getId() == id){
employees.remove(i);
for (i = id; i <= employees.size(); i++) {
//动态 ID,随着人员删除 ID 相应变化(内涵:找到索引减一的那个值,此时这个值对应的就是删除 ID 后,接上来的那个 ID,只要将这个 ID 减一,就能继续升序而不断开)
employees.get(i-1).setId(employees.get(i-1).getId()-1);
}
flag=true;
}
}
if(flag){
System.out.println("删除成功!");
count--;
}else {
throw new TeamException("该员工不存在");
}
}
//员工的查看
public void showEmployee() throws InterruptedException {
TSUtility.loadSpecialEffects();
System.out.println("ID\t 姓名\t 年龄\t 工资\t 职位\t 状态\t 奖金\t 股票\t 领用设备");
for (int i = 0; i < employees.size(); i++) {
System.out.println(""+employees.get(i));
}
}
//修改员工信息(姓名,年龄,工资)
public void modifyEmployee(int id){
boolean flag=false;
for (int i = 0; i < employees.size(); i++) {
Employee emp=employees.get(i);
if(employees.get(i).getId() == id){
System.out.println("姓名("+emp.getName()+")(回车直接跳过修改):");
String name=TSUtility.readString(4,emp.getName());
System.out.println("年龄("+emp.getAge()+")(回车直接跳过修改):");
int age=Integer.parseInt(TSUtility.readString(2,emp.getAge()+""));
System.out.println("工资("+emp.getSalary()+")(回车直接跳过修改):");
double salary=Double.parseDouble(TSUtility.readString(6,emp.getSalary()+""));
emp.setName(name);
emp.setAge(age);
emp.setSalary(salary);
employees.set(i,emp);
flag=true;
}
}
if(flag){
System.out.println("修改成功!");
}else {
try {
throw new TeamException("该员工不存在");
} catch (TeamException e) {
e.printStackTrace();
}
}
}
}
团队人员管理类 Teamservice:
package service;
/*
团队内容判断管理模块
*/
import domain.Architect;
import domain.Designer;
import domain.Employee;
import domain.Programmer;
public class TeamService {
//静态变量,用来为开发团队新增成员自动生成团队中的唯一 ID,即 memberId。(提示:应使用增 1 的方式)
private static int counter = 1;
//开发团队最大成员数,final 静态为常量,变量名全大写
private final int MAX_MEMBER = 5;
//数组,程序员数组
Programmer[] team = new Programmer[MAX_MEMBER];
//团队实际人数
private int total = 0;
public TeamService() {
}
public TeamService(int counter, Programmer[] team, int total) {
this.counter = counter;
this.team = team;
this.total = total;
}
public Programmer[] getTeam() {
Programmer[] team = new Programmer[total];
for (int i = 0; i < total; i++) {
team[i] = this.team[i];
}
return team;
}
//初始化当前团队成员数组
public void clearTeam() {
team = new Programmer[MAX_MEMBER];
counter = 1;
total = 0;
this.team = team;
}
//增加团队成员
public void addMember(Employee e) throws TeamException {
if (total >= MAX_MEMBER) {
throw new TeamException("成员已满,无法添加");
}
if (!(e instanceof Programmer)) {
throw new TeamException("该成员不是开发人员,无法添加");
}
Programmer p = (Programmer) e;
if (isExist(p)) {
throw new TeamException("该员工已在本团队中");
}
if (!(p.getStatus())) {
throw new TeamException("该员工已是某一团队成员");
}
//团队中人员要求,至多一位架构师,至多两位设计师,至多三位程序员
int numArchitect = 0;
int numDesigner = 0;
int numProgrammer = 0;
for (int i = 0; i < total; i++) {
if (team[i] instanceof Architect) {
numArchitect++;
} else if (team[i] instanceof Designer) {
numDesigner++;
} else if (team[i] instanceof Programmer) {
numProgrammer++;
}
}
if (p instanceof Architect) {
if (numArchitect >= 1) {
throw new TeamException("团队中至多只能有一位架构师");
}
} else if (p instanceof Designer) {
if (numDesigner >= 2) {
throw new TeamException("团队中至多只能有两位设计师");
}
} else if (p instanceof Programmer) {
if (numProgrammer >= 3) {
throw new TeamException("团队中至多只能有三位程序员");
}
}
//添加到数组
p.setStatus(false);
p.setMemberId(counter++);
team[total++] = p;
}
//判断团队中是否已经存在这个成员
public boolean isExist(Programmer p) {
for (int i = 0; i < total; i++) {
if (team[i].getId() == p.getId()) {
return true;
}
}
return false;
}
//删除指定 memberld 的成员(已经在团队内的成员)
public void removeMember(int memberld) throws TeamException {
int n = 0;
//找到指定 T
ID(memberld)的员工删除,遍历,找不到报异常
for (; n < total; n++) {
if (team[n].getMemberId() == memberld) {
team[n].setStatus(true);
break;
}
}
if (n == total) {
throw new TeamException("找不到该成员,无法删除");
}
for (int i = n+1; i < total; i++) {
team[i-1]=team[i];
}
team[--total]=null;
int a =1;
for(int i =0;i<total;i++){
team[i].setMemberId(a++);
}
}
}
项目开发管理类 ProjectService:
package service;
/*
项目开发管理模块
*/
import domain.Programmer;
import domain.Project;
import view.TSUtility;
import java.util.ArrayList;
import java.util.Random;
public class ProjectService {
private ArrayList<Project> pro = new ArrayList<>();
private int count = 1;
int a = 1;
int b = 1;
int c = 1;
int d = 1;
//添加项目
public void addProject() throws InterruptedException {
System.out.println("项目参考:-------------------------------------");
System.out.println("1.小米官网:开发完成类似于小米官网的 web 项目");
System.out.println("2.公益在线商城:猫宁 Morning 公益商城是中国公益性在线电子商城.");
System.out.println("3.博客系统:Java 博客系统,让每一个有故事的人更好的表达想法!");
System.out.println("4.在线协作文档编辑系统:一个很常用的功能,适合小组内的文档编辑。");
System.out.println("------------------------------------------------------------");
TSUtility.readReturn();
System.out.println("请输入你想添加的项目序号: ");
char ch = TSUtility.readMenuSelection();
switch (ch) {
case '1':
Project p1 = new Project();
p1.setProId(count++);
p1.setProjectName("小米官网");
p1.setDesName("开发完成类似于小米官网的 web 项目");
if (a == 1) {
pro.add(p1);
TSUtility.loadSpecialEffects();
System.out.println("已添加项目:" + p1.getProjectName());
a++;
} else {
System.out.println("你添加的项目已经被添加,请添加其他的项目!");
}
break;
case '2':
Project p2 = new Project();
p2.setProId(count++);
p2.setProjectName("公益在线商城");
p2.setDesName("猫宁 Morning 公益商城是中国公益性在线电子商城");
if (b == 1) {
pro.add(p2);
TSUtility.loadSpecialEffects();
System.out.println("已添加项目:" + p2.getProjectName());
b++;
} else {
System.out.println("你添加的项目已经被添加,请添加其他的项目!");
}
break;
case '3':
Project p3 = new Project();
p3.setProId(count++);
p3.setProjectName("博客系统");
p3.setDesName("Java 博客系统,让每一个有故事的人更好的表达想法!");
if (c == 1) {
pro.add(p3);
TSUtility.loadSpecialEffects();
System.out.println("已添加项目:" + p3.getProjectName());
} else {
System.out.println("你添加的项目已经被添加,请添加其他的项目!");
}
break;
case '4':
Project p4 = new Project();
p4.setProId(count++);
p4.setProjectName("在线协作文档编辑系统");
p4.setDesName("一个很常用的功能,适合小组内的文档编辑。");
if (d == 1) {
pro.add(p4);
TSUtility.loadSpecialEffects();
System.out.println("已添加项目:" + p4.getProjectName());
} else {
System.out.println("你添加的项目已经被添加,请添加其他的项目!");
}
break;
default:
System.out.println("该项目不存在!");
break;
}
}
//项目分配团队开发
public void dealingPro(Programmer[] team) {
if (pro == null) {
System.out.println("没有项目,请先添加项目!!!");
return;
}
System.out.println("当前团队人员有:");
for (int i = 0; i < team.length; i++) {
System.out.println(team[i]);
}
System.out.println("请为当前团队创建一个团队名称:");
String teamName = TSUtility.readKeyBoard(6, false);
//分配项目随机
if (team.length!=0) {
Random r = new Random();
int RandomNum = r.nextInt(pro.size());
Project project = this.pro.get(RandomNum);
if (!(project.equals(null))) {
project.setTeamName(teamName);
project.setTeam(team);
project.setStatus(true);
pro.set(RandomNum, project);
}
}
}
//查看项目当前状态
public void showPro() throws InterruptedException {
TSUtility.loadSpecialEffects();
if (pro.size() == 0) {
System.out.println("当前没有项目,请添加项目!!!");
}
for (int i = 0; i < pro.size(); i++) {
System.out.println(pro.get(i));
}
}
//删除选择的项目
public void delPro(int id) throws TeamException {
boolean flag = false;
for (int i = 0; i < pro.size(); i++) {
if ((pro.get(i).getStatus())) {
if (pro.get(i).getProId() == id) {
pro.remove(i);
for (i = id; i < pro.size(); i++) {
pro.get(i - 1).setProId(pro.get(i - 1).getProId() - 1);//动态 ID 改变
}
flag = true;
}
} else {
throw new TeamException("当前项目正在被开发,无法删除!");
}
}
if (flag) {
System.out.println("删除成功!");
count--;
} else {
try {
throw new TeamException("该项目不存在!");
} catch (TeamException e) {
e.printStackTrace();
}
}
}
//遍历集合所有元素得到所有数据
public ArrayList<Project> getAllPro() {
for (int i = 0; i < pro.size(); i++) {
System.out.println(pro.get(i));
}
return pro;
}
}
3、view 包下:
用户登录/注册界面 LoginView:
package view;
/*
*用户登录/注册界面
*/
import java.util.Scanner;
@SuppressWarnings("all")
public class LoginView {
//需要初始化值,让 String 类型的默认初始化不是为 null;
private String userName="";//用户名
private String password="";//登录密码
//用户注册信息
public void register() throws InterruptedException {
TSUtility.loadSpecialEffects();
System.out.println("开始注册...");
Scanner sc = new Scanner(System.in);
System.out.println("请输入你要注册的账户名称(名称不大于四位):");
String userName = TSUtility.readKeyBoard(4, false);
this.userName=userName;
System.out.println("请输入你的登录密码(位数不大于八位):");
String password = TSUtility.readKeyBoard(8, false);
this.password=password;
System.out.println("注册成功,请重新登录。");
}
//用户登录
public void Login() throws InterruptedException {
//登录失败的限制次数
int count = 5;
boolean flag = true;
//登录界面的循环
while (flag) {
System.out.println("********************??");
System.out.println("*** <登录界面> ***");
System.out.println("*** (: ***??");
System.out.println("********************??");
//账号信息的输入与登录判断
System.out.println("请输入你的账户名称");
String userName = TSUtility.readKeyBoard(4, false);
System.out.println("请输入你的登录密码");
String password = TSUtility.readKeyBoard(8, false);
if (this.userName.length() == 0 || this.password.length() == 0) {
System.out.println("未检测到你的账号信息,请重新输入或注册");
register();
} else if (this.userName.equals(userName) && this.password.equals(password)) {
TSUtility.loadSpecialEffects();
System.out.println("登录成功,欢迎你:" + userName);
flag = false;
} else {
count--;
if (count <= 0) {
System.out.println("登录次数为零,无法登录,退出。");
return;
}
System.out.println("登录失败,用户名不匹配或密码错误,还剩余" + count + "次登录机会,请重新输入");
}
}
}
//用户修改
public void revise() throws InterruptedException {
boolean flag = true;
//修改界面的循环
while (flag) {
System.out.println("********************??");
System.out.println("*** <修改界面> ***");
System.out.println("*** (: ***??");
System.out.println("********************??");
System.out.println("请输入你需要修改的类型:");
System.out.println("1(修改用户名)");
System.out.println("2(修改密码名)");
System.out.println("3(修改用户名和密码名)");
System.out.println("4(不修改,退出)");
Scanner sc = new Scanner(System.in);
String num = sc.next();
if (num.equals("1")) {
System.out.println("请输入你需要修改的新账户名称:");
String userName = TSUtility.readKeyBoard(4, false);
this.userName = userName;
System.out.println("修改成功!");
} else if (num.equals("2")) {
System.out.println("请输入你需要修改的新账户密码:");
String password = TSUtility.readKeyBoard(8, false);
this.password = password;
System.out.println("修改成功!");
} else if (num.equals("3")) {
System.out.println("请输入你需要修改的新账户名称:");
String userName = TSUtility.readKeyBoard(4, false);
this.userName = userName;
System.out.println("请输入你需要修改的新密码:");
String password = TSUtility.readKeyBoard(8, false);
this.password = password;
System.out.println("修改成功!");
} else if (num.equals("4")) {
System.out.println("你确定退出吗?Y/N");
char ch = TSUtility.readConfirmSelection();
if (ch == 'Y') {
System.out.println("正在退出...");
TSUtility.loadSpecialEffects();
flag = false;
}
} else {
System.out.println("输入错误!请输入“1”或者“2”或者“3”或者“4”:");
}
}
}
}
团队修改界面 Teamview:
package view;
/*
管理团队模块的配置管理
*/
import com.sun.org.apache.xpath.internal.objects.XNumber;
评论