Flutter Tab
发布于: 2021 年 05 月 14 日
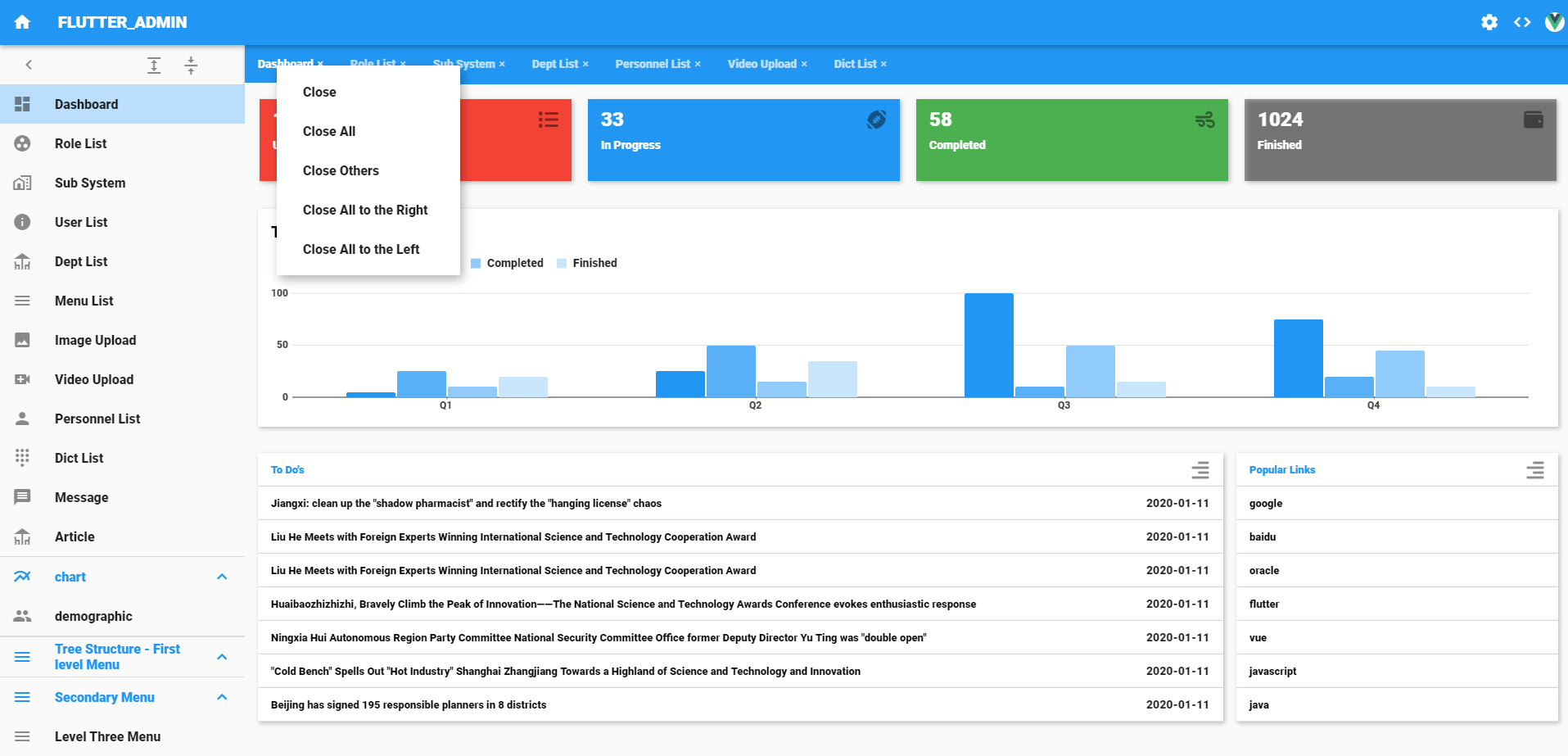
Flutter Tab
本文通过提供几个例子介绍 Flutter Tab 的使用
http://cairuoyu.com/flutter_demo
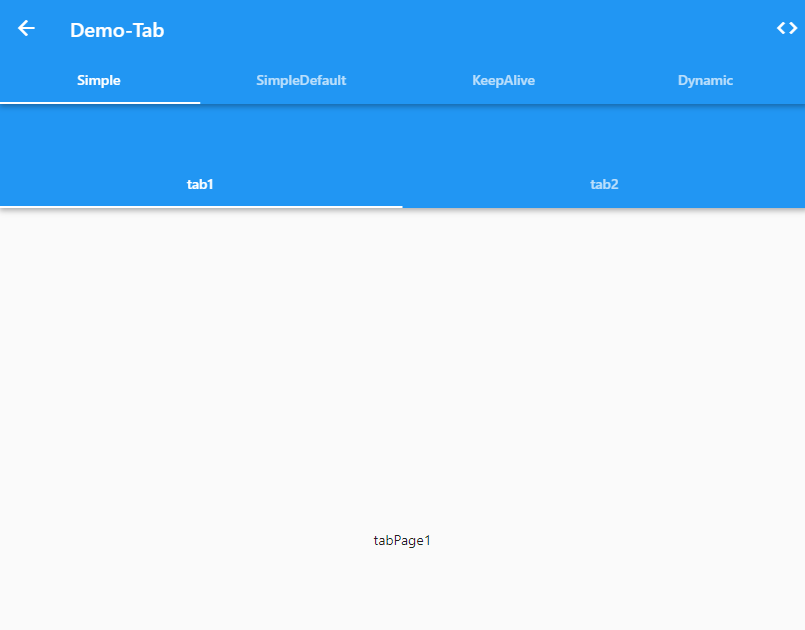
Demo
使用 TabController
使用时需要 with TickerProviderStateMixin
List<Tab> tabs = <Tab>[
Tab(text: 'tab1'),
Tab(text: 'tab2'),
];
List<Widget> tabBarViews = <Widget>[
Center(child: Text('tabPage1')),
Center(child: Text('tabPage2')),
];
复制代码
class TabSimple extends StatefulWidget {
@override
_TabSimpleState createState() => _TabSimpleState();
}
class _TabSimpleState extends State<TabSimple> with TickerProviderStateMixin {
TabController _tabController;
@override
void initState() {
super.initState();
_tabController = TabController(length: tabs.length, vsync: this);
}
@override
Widget build(BuildContext context) {
var result = Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
bottom: TabBar(controller: _tabController, tabs: tabs),
),
body: TabBarView(controller: _tabController, children: tabBarViews),
);
return result;
}
}
复制代码
使用 DefaultTabController
简单的功能可以使用 DefaultTabController,它其实是对 TabController 的封装,可以减少代码量
class TabSimpleDefault extends StatelessWidget {
@override
Widget build(BuildContext context) {
var result = DefaultTabController(
length: tabs.length,
child: Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
bottom: TabBar(tabs: tabs),
),
body: TabBarView(children: tabBarViews),
),
);
return result;
}
}
复制代码
切换 Tab 时保持状态
当切换 Tab 时,页面会调用 init 和 build 重新渲染,从而失去状态。官方推荐使用 AutomaticKeepAliveClientMixin 来控制每个页面是否需要保持状态
import 'package:flutter/material.dart';
import 'data.dart';
class TabKeepAlive extends StatelessWidget {
@override
Widget build(BuildContext context) {
var result = DefaultTabController(
length: tabs.length,
child: Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
bottom: TabBar(tabs: tabs),
),
body: TabBarView(children: [
KeepAlivePage(
title: 'page1',
),
KeepAlivePage(
title: 'page2',
),
]),
),
);
return result;
}
}
class KeepAlivePage extends StatefulWidget {
final String title;
const KeepAlivePage({Key key, this.title}) : super(key: key);
@override
_KeepAlivePageState createState() => _KeepAlivePageState();
}
class _KeepAlivePageState extends State<KeepAlivePage> with AutomaticKeepAliveClientMixin {
@override
void initState() {
super.initState();
print('init--' + widget.title);
}
@override
Widget build(BuildContext context) {
super.build(context);
print('build--' + widget.title);
var result = TextField(
decoration: InputDecoration(hintText: '请输入' + widget.title),
);
return result;
}
@override
bool get wantKeepAlive => true;
}
复制代码
动态 Tab
可动态增加或减少 Tab
class TabDynamic extends StatefulWidget {
@override
_TabDynamicState createState() => _TabDynamicState();
}
class _TabDynamicState extends State<TabDynamic> with TickerProviderStateMixin {
TabController tabController;
int tabNum = 3;
@override
Widget build(BuildContext context) {
int index = tabController?.index ?? 0;
index = tabNum > index ? index : tabNum - 1;
tabController?.dispose();
tabController =
TabController(length: tabNum, vsync: this, initialIndex: index);
tabController.animateTo(tabNum - 1);
var tabs = List.generate(
tabNum,
(index) => Tab(
child: Row(
children: [
Text('tab' + index.toString()),
IconButton(
splashRadius: 10,
iconSize: 10,
icon: Icon(Icons.close_outlined),
onPressed: () {
setState(() {
tabNum--;
});
},
)
],
),
// icon: Icon(Icons.add),
),
);
var tabPages = List.generate(
tabNum,
(index) => Center(
child: TestPage(index),
),
);
var tabBar = TabBar(
isScrollable: true,
tabs: tabs,
controller: tabController,
);
var tabBarView = TabBarView(
children: tabPages,
controller: tabController,
);
var result = Scaffold(
appBar: AppBar(automaticallyImplyLeading: false, bottom: tabBar),
body: tabBarView,
floatingActionButton: FloatingActionButton(
child: Icon(Icons.add),
onPressed: () {
setState(() {
tabNum++;
});
},
),
);
return result;
}
}
复制代码
附
源码地址:
https://github.com/cairuoyu/flutter_demo
更多复杂功能参考:
https://github.com/cairuoyu/flutter_admin/blob/master/lib/pages/layout/layout_center.dart
划线
评论
复制
发布于: 2021 年 05 月 14 日阅读数: 14
Geek_7e907c
关注
还未添加个人签名 2021.01.18 加入
还未添加个人简介
评论