springboot+mybatis+druid 整合笔记
</dependency>
我这里 springboot 版本是最新版(2.5.2)。
2.2 配置
我这里 mybatis 的配置就一项,告诉系统我的 mapper.xml 在哪个位置
mybatis.mapper-locations=classpath:mapper/*.xml
数据源配置
spring.datasource.type= com.alibaba.druid.pool.DruidDataSource
spring.datasource.url= jdbc:mysql://127.0.0.1:3306/mybatis_test?useUnicode=true&characterEncoding=utf-8
spring.datasource.username= root
spring.datasource.password= root
spring.datasource.driverClassName= com.mysql.jdbc.Driver
spring.datasource.initialSize= 1
spring.datasource.minIdle= 1
spring.datasource.maxActive= 50
spring.datasource.maxWait= 60000
spring.datasource.timeBetweenEvictionRunsMillis= 60000
spring.datasource.minEvictableIdleTimeMillis= 300000
spring.datasource.validationQuery= SELECT 1 FROM DUAL
spring.datasource.testWhileIdle=true
spring.datasource.testOnBorrow= false
spring.datasource.testOnReturn= false
spring.datasource.poolPreparedStatements= true
spring.datasource.maxPoolPreparedStatementPerConnectionSize= 20
spring.datasource.filters= stat,wall,log4j
spring.datasource.connectionProperties= druid.stat.mergeSql=true;druid.stat.slowSqlMillis=500
这里整合数据源的话,由于没有用 druid 的 starter,需要自己手动创建下,也就是整个配置类
@Configuration
public class DruidDataSourceConfig {
/**
druid 数据库连接池配置
*/
@Value("${spring.datasource.url}")
private String dbUrl;
@Value("${spring.datasource.username}")
private String username;
@Value("${spring.datasource.password}")
private String password;
@Value("${spring.datasource.driverClassName}")
private String driverClassName;
@Value("${spring.datasource.initialSize}")
private int initialSize;
@Value("${spring.datasource.minIdle}")
private int minIdle;
@Value("${spring.datasource.maxActive}")
private int maxActive;
@Value("${spring.datasource.maxWait}")
private int maxWait;
@Value("${spring.datasource.timeBetweenEvictionRunsMillis}")
private int timeBetweenEvictionRunsMillis;
@Value("${spring.datasource.minEvictableIdleTimeMillis}")
private int minEvictableIdleTimeMillis;
@Value("${spring.datasource.validationQuery}")
private String validationQuery;
@Value("${spring.datasource.testWhileIdle}")
private boolean testWhileIdle;
@Value("${spring.datasource.testOnBorrow}")
private boolean testOnBorrow;
@Value("${spring.datasource.testOnReturn}")
private boolean testOnReturn;
@Value("${spring.datasource.poolPreparedStatements}")
private boolean poolPreparedStatements;
@Value("${spring.datasource.maxPoolPreparedStatementPerConnectionSize}")
private int maxPoolPreparedStatementPerConnectionSize;
@Value("${spring.datasource.filters}")
private String filters;
@Value("{spring.datasource.connectionProperties}")
private String connectionProperties;
/**
创建 druid 数据库连接池 bean
@return
*/
@Bean
@Primary
public DataSource dataSource(){
DruidDataSource datasource = new DruidDataSource();
datasource.setUrl(this.dbUrl);
datasource.setUsername(username);
datasource.setPassword(password);
datasource.setDriverClassName(driverClassName);
datasource.setInitialSize(initialSize);
datasource.setMinIdle(minIdle);
datasource.setMaxActive(maxActive);
datasource.setMaxWait(maxWait);
datasource.setTimeBetweenEvictionRunsMillis(timeBetweenEvictionRunsMillis);
datasource.setMinEvictableIdleTimeMillis(minEvictableIdleTimeMillis);
datasource.setValidationQuery(validationQuery);
datasource.setTestWhileIdle(testWhileIdle);
datasource.setTestOnBorrow(testOnBorrow);
datasource.setTestOnReturn(testOnReturn);
datasource.setPoolPreparedStatements(poolPreparedStatements);
datasource.setMaxPoolPreparedStatementPerConnectionSize(maxPoolPreparedStatementPerConnectionSize);
try {
datasource.setFilters(filters);
} catch (SQLException e) {
e.printStackTrace();
}
datasource.setConnectionProperties(connectionProperties);
return datasource;
}
}
3.写一个 demo 试试
这里我们就写个 demo 来试试效果,随便整个 user 表
3.1 表结构与实体
CREATE TABLE user
(
id
int(10) NOT NULL AUTO_INCREMENT,
username
varchar(255) COLLATE utf8_unicode_ci DEFAULT NULL,
password
varchar(255) COLLATE utf8_unicode_ci DEFAULT NULL,
PRIMARY KEY (id
)
) ENGINE=MyISAM AUTO_INCREMENT=5 DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
public class UserEntity implements Serializable {
private Integer id;
private String userName;
private String password;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
3.2 mapper.xml 编写
UserMapper.xml 文件。放到 resource 目录下的 mapper 目录下, 正好对应我们上面配置的 mapper 文件位置
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.testcanal.dao.UserDao">
<sql id="userField">
id,username,password
</sql>
<insert id="addUser" useGeneratedKeys="true" keyProperty="id">
insert into user (username,password) values (#{user.userName},#{user.password})
</insert>
</mapper>
3.3 dao 编写
@Mapper
public interface UserDao {
boolean addUser(@Param("user") UserEntity userEntity);
}
很简单,主要的是要 @Mapper 注解,不然的话不知道你是个 mapper 接口,也就没法给你创建代理类。
3.4 service 与 controller 编写
service 编写:
public interface UserService {
boolean add(UserEntity userEntity);
}
@Service
public class UserServiceImpl implements UserService {
@Resource
private UserDao userDao;
@Override
@Transactional
public boolean add(UserEntity userEntity) {
return userDao.addUser(userEntity);
}
}
controller 编写
@RestController
@RequestMapping("/test")
public class TestController {
@Autowired
private UserService userService;
@GetMapping("/user/add")
public UserEntity add( Integer id){
UserEntity userEntity=new UserEntity();
userEntity.setUserName("xxxx");
userEntit
y.setPassword("pass");
userService.add(userEntity);
return userEntity;
}
}
3.4 测试
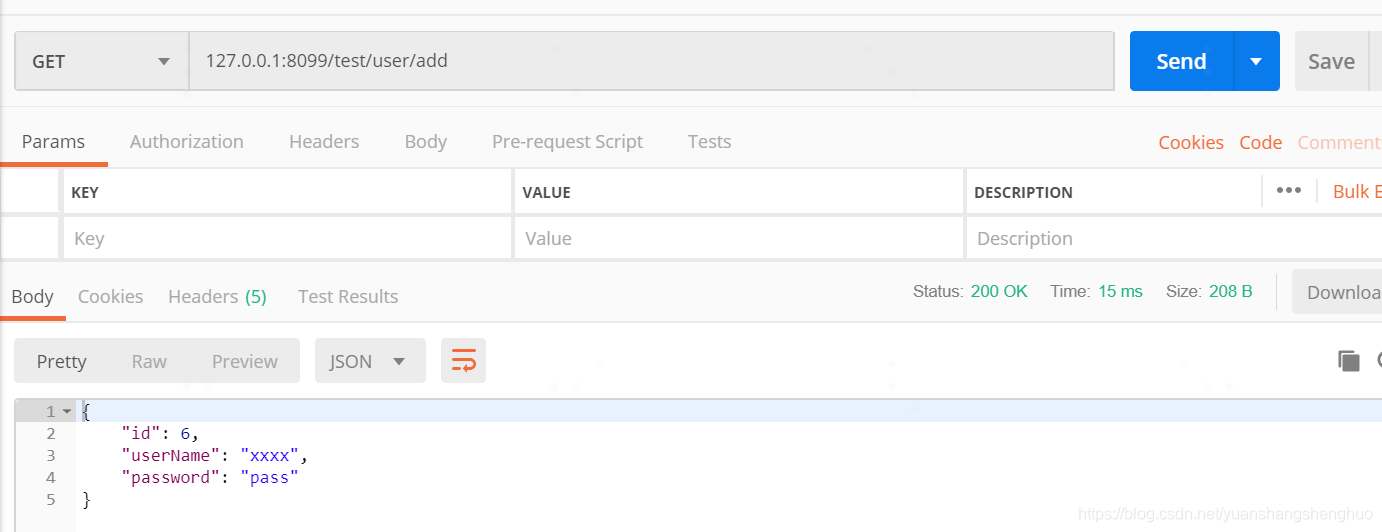
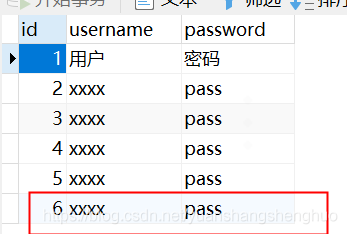
可以看到通了,到这里就完事了
4. druid starter 整合
上面我们在整合 druid 的时候,就是用了 druid 的普通依赖,这样有个问题就是需要我们手动写配置类进行初始化数据源。
评论