【Spring Boot 4】如何优雅的使用 Mybatis,linux 内核深度解析
mybatis-spring-boot-starter 主要由两种解决方案,一种是使用注解解决一切问题,一种的简化后的老传统。
当然任何模式都需要先引入 mybatis-spring-boot-starter 的 pom 文件,现在最新版本是
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
三、无配置注解版
============
1、添加 maven 文件
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
<dependency>
<groupId>com.oracle.ojdbc</groupId>
<artifactId>ojdbc8</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.21</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
2、application.yml 添加相关配置
spring:
datasource:
username: mine
password: mine
url: jdbc:oracle:thin:@127.0.0.1:1521:orcl
driver-class-name: oracle.jdbc.driver.OracleDriver
type: com.alibaba.druid.pool.DruidDataSource
initialSize: 5
minIdle: 5
maxActive: 20
maxWait: 60000
timeBetweenEvictionRunsMillis: 60000
minEvictableIdleTimeMillis: 300000
validationQuery: SELECT 1 FROM DUAL
testWhileIdle: true
testOnBorrow: false
testOnReturn: false
poolPreparedStatements: true
filters: stat,wall,log4j
maxPoolPreparedStatementPerConnectionSize: 20
useGlobalDataSourceStat: true
connectionProperties: druid.stat.mergeSql=true;druid.stat.slowSqlMillis=500
Spring Boot 会自动加载 spring.datasource.*相关配置,数据源就会自动注入到 sqlSessionFactory 中,sqlSessionFactory 会自动注入到 Mapper 中,对了,你一切都不用管了,直接拿起来使用就行了。
在启动类中添加对 mapper 包扫描 @MapperScan
@MapperScan(value="com.demo.mapper")
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
或者直接在 Mapper 类上面添加注解[@Mapper](
),建议使用上面那种,不然每个 mapper 加个注解也挺麻烦的
3、controller
@RestController
public class DeptController {
@Autowired
DepartmentMapper departmentMapper;
@GetMapping("/dept/{id}")
public Department getDepartment(@PathVariable("id") Integer id){
return departmentMapper.getDeptById(id);
}
@GetMapping("/dept")
public int insertDeptById(@PathVariable("id") Integer id,@PathVariable("departmentName") String departmentName){
int ret = departmentMapper.insertDept(id,departmentName);
return ret;
}
}
4、开发 mapper
package com.demo.mapper;
import com.demo.bean.Department;
import org.apache.ibatis.annotations.*;
public interface DepartmentMapper {
@Select("select * from SSH_DEPARTMENT where id=#{id}")
public Department getDeptById(Integer id);
@Delete("delete from SSH_DEPARTMENT where id=#{id}")
public int deleteDeptById(Integer id);
@Options(useGeneratedKeys = true,keyProperty = "id")
@Insert("insert into SSH_DEPARTMENT(department_name) values(#{departmentName})")
public int insertDept(Department department);
@Update("update SSH_DEPARTMENT set departmentName=#{DEPARTMENT_NAME} where id=#{id}")
public int updateDept(Department department);
}
[@Sel
ect](
)?是查询类的注解,所有的查询均使用这个
[@Result](
)?修饰返回的结果集,关联实体类属性和数据库字段一一对应,如果实体类属性和数据库属性名保持一致,就不需要这个属性来修饰。
[@Insert](
)?插入数据库使用,直接传入实体类会自动解析属性到对应的值
[@Update](
)?负责修改,也可以直接传入对象
[@delete](
)?负责删除
5、运行
上面三步就基本完成了相关 Mapper 层开发,使用的时候当作普通的类注入进入就可以了
(1)查询
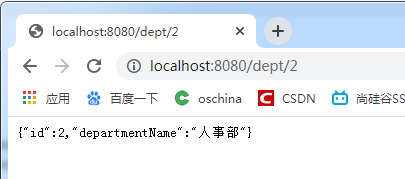
评论