自建开发工具系列 -Webkit 内存动量监控 UI(五)
发布于: 2 小时前
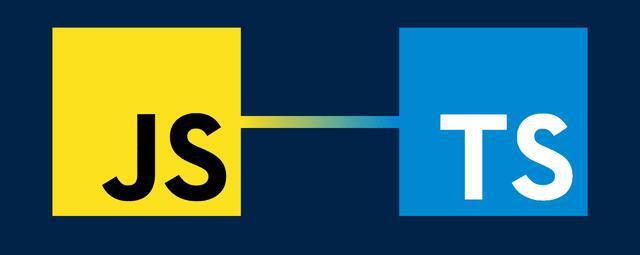
现在 UI 的基本逻辑有了,但其实只是制作工具的第一步-逻辑走通。为了更好的约束和规范工具的持续发展(脚踏实地,面向未来),本篇将要动手将 jsx 和 js 转换为 tsx 和 ts.
在修改 UI 的 App.js 到 App.tsx 的过程中,调整了类型和 Ref 的写法如下
类型为./config/api.ts:
export interface TWMProps {
screenCorner?: string,
toolsNode: HTMLDivElement
}
export interface UtilsProps {
(): {
update():void,
domElement: HTMLDivElement
}
}
export interface UtilsInterfaces {
update():void,
domElement: HTMLDivElement
}
复制代码
Ref 写法和增加的类型调整如下:
import * as React from 'react';
import './App.css';
import displayPanel from './utils';
-
+import { TWMProps, UtilsInterfaces } from './config/api';
class App extends React.Component {
+ toolsNode: React.RefObject<HTMLDivElement>;
+ twm: UtilsInterfaces;
- constructor(props) {
+ constructor(props:TWMProps) {
super(props);
- this.state = {
- twm: displayPanel()
- };
+ this.toolsNode = React.createRef();
+ this.twm = displayPanel()
}
componentDidMount() {
const updateLoop = () => {
- if (this.refs.toolsNode.appendChild) {
- this.refs.toolsNode.appendChild(this.state.twm.domElement);
+ if ((this.toolsNode.current as any).appendChild) {
+ (this.toolsNode.current as any).appendChild(this.twm.domElement);
}
- if (this.state.twm) {
- this.state.twm.update();
+ if (this.twm) {
+ this.twm.update();
}
- if (window.performance.memory.jsHeapSizeLimit) {
+ if ((window.performance as any).memory.jsHeapSizeLimit) {
window.setTimeout(updateLoop, 1000)
}
}
}
render() {
- return <div style={{top: '0px', right: '0px', position: 'fixed'}} ref="toolsNode" className="displayPanel" />;
+ return (
+ <div style={{top: '0px', right: '0px', position: 'fixed'}} ref={this.toolsNode} className="displayPanel" />
+ )
}
}
复制代码
对应的将工具类 utils.js 更改为 utils.ts 的更改如下:
-const displayPanel = function () {
+import { UtilsProps } from './config/api';
+const displayPanel: UtilsProps = function () {
let memoryBottom = 100;
let memoryTop = 0;
const TOOLS_HEIGHT = 30;
@@ -32,15 +33,15 @@ const displayPanel = function () {
nodeUI.appendChild(bar);
}
- const updateData = function (dom, height, color) {
- const child = dom.appendChild(dom.firstChild);
+ const updateData = function (dom: HTMLDivElement, height: number, color: string) {
+ const child = dom.appendChild(dom.firstChild as HTMLDivElement) as HTMLDivElement;
child.style.height = `${height}px`;
if (color) child.style.backgroundColor = color;
};
- const refreshGraph = function (dom, oHFactor, hFactor) {
- [].forEach.call(dom.children, (c) => {
- const cHeight = c.style.height.substring(0, c.style.height.length - 2);
+ const refreshGraph = function (dom: HTMLDivElement, oHFactor: number, hFactor: number) {
+ [].forEach.call(dom.children, (c:HTMLDivElement) => {
+ const cHeight: number = Number(c.style.height.substring(0, c.style.height.length - 2));
// 转换到 MB
const newVal = TOOLS_HEIGHT - ((TOOLS_HEIGHT - cHeight) / oHFactor) * hFactor;
@@ -49,22 +50,22 @@ const displayPanel = function () {
});
};
- // 避免没有 window.performance.memory 的浏览器崩溃
- if (window.performance && !performance.memory) {
- performance.memory = { usedJSHeapSize: 0, totalJSHeapSize: 0 };
+ // 避免没有 window.(performance as any).memory 的浏览器崩溃
+ if (window.performance && !(performance as any).memory) {
+ (performance as any).memory = { usedJSHeapSize: 0, totalJSHeapSize: 0 };
}
let precision;
let i;
- function bytesToMB(bytes) {
+ function bytesToMB(bytes: number) {
precision = Math.pow(10, 0);
i = Math.floor(Math.log(bytes) / Math.log(1024));
return `${Math.round((bytes * precision) / Math.pow(1024, i)) / precision} MB`;
}
let lastTime = Date.now();
- let lastUsedHeap = performance.memory.usedJSHeapSize;
+ let lastUsedHeap = (performance as any).memory.usedJSHeapSize;
let delta = 0;
let color = 'black';
let ms = 0;
@@ -79,8 +80,8 @@ const displayPanel = function () {
if (Date.now() - lastTime < 1000 / 1) return;
lastTime = Date.now();
- delta = performance.memory.usedJSHeapSize - lastUsedHeap;
- lastUsedHeap = performance.memory.usedJSHeapSize;
+ delta = (performance as any).memory.usedJSHeapSize - lastUsedHeap;
+ lastUsedHeap = (performance as any).memory.usedJSHeapSize;
// GC 判定,如果是清空内存,则使用绿色标识
color = delta < 0 ? 'green' : 'black';
@@ -88,7 +89,7 @@ const displayPanel = function () {
ms = lastUsedHeap;
memoryBottom = Math.min(memoryBottom, ms);
memoryTop = Math.max(memoryTop, ms);
- noticeLabel.textContent = window.performance.memory.jsHeapSizeLimit ? `内存使用: ${bytesToMB(ms)}` : 'Only Webkit';
+ noticeLabel.textContent = (window.performance as any).memory.jsHeapSizeLimit ? `内存使用: ${bytesToMB(ms)}` : 'Only Webkit';
mbValue = ms / (1024 * 1024);
复制代码
这里的对比使用了 git diff > compare.txt 命令,为了方便查看,还将 tsx 和 ts 恢复成以前的 js 文件类型方便 git diff 比对
最终,将文件分别从 js 改为 tsx 和 ts 后,还需修改 tsconfig.json 以便构建:
--- a/tsconfig.json
+++ b/tsconfig.json
@@ -1,28 +1,17 @@
{
"compilerOptions": {
- "target": "es5",
- "module": "esnext",
- "lib": [
- "DOM",
- "ES2015"
- ],
- "allowJs": true,
- "declaration": true,
- "outDir": "./lib",
+ "target": "es5",
+ "module": "commonjs",
+ "lib": ["DOM", "ES2015"],
+ "allowJs": true,
+ "declaration": true,
+ "outDir": "./lib",
"rootDir": "./src",
- "strict": true,
+ "strict": true,
"esModuleInterop": true,
- "skipLibCheck": true,
- "allowSyntheticDefaultImports": true,
- "forceConsistentCasingInFileNames": true,
- "noFallthroughCasesInSwitch": true,
- "moduleResolution": "node",
- "resolveJsonModule": true,
- "isolatedModules": true,
- "noEmit": true,
"jsx": "react-jsx"
},
"include": [
- "src/**/*"
+ "src/**/*.tsx"
]
}
复制代码
现在,配合前文设置的 npm run build:ts 的构建命令,经过运行后,获得了转换成 es5 的 js 工具包和.d.ts 的类型文件
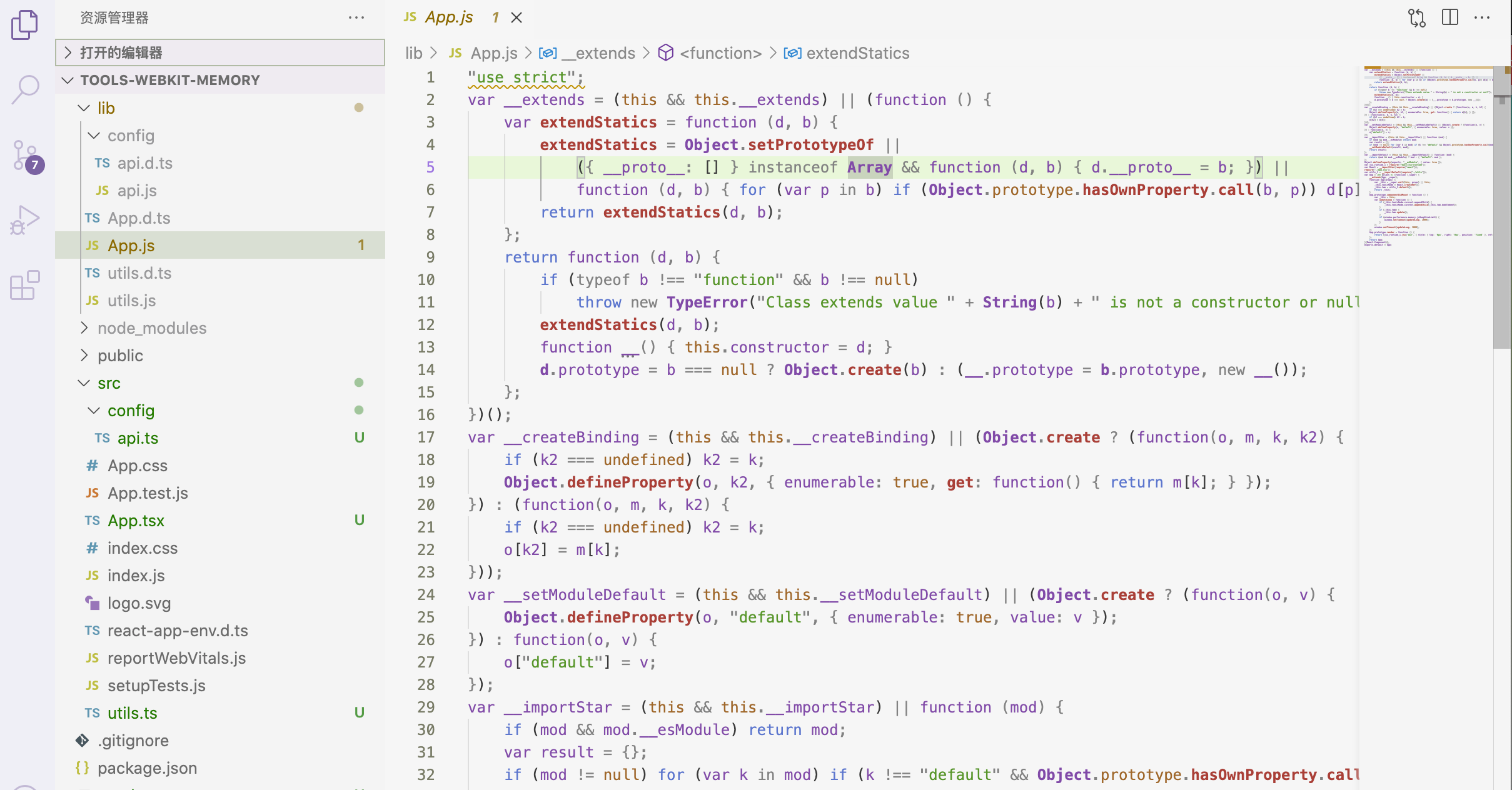
现在,这个工具库脚踏实地(ES5)面向未来(Typescript)的基础终于有了。
划线
评论
复制
发布于: 2 小时前阅读数: 5
版权声明: 本文为 InfoQ 作者【Tim】的原创文章。
原文链接:【http://xie.infoq.cn/article/06b3326fb9a4e703fddef4189】。文章转载请联系作者。
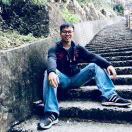
Tim
关注
还未添加个人签名 2018.05.01 加入
还未添加个人简介
评论