Jsoup 解析 html
/**
Jsoup 查找 DOM 元素
*/
@Test
public void test2() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements itemElements = doc.getElementsByClass("post_item"); // 根据样式名称来查询 DOM
System.out.println("=======输出 post_item==============");
for (Element e : itemElements) {
System.out.println(e.html());//获取里面所有的 html 包括文本
System.out.println("\n");
}
Elements widthElements = doc.getElementsByAttribute("width"); // 根据属性名称来查询 DOM(id class type 等),用的少一般很难找用这种方法
System.out.println("=======输出 with 的 DOM==============");
for (Element e : widthElements) {
System.out.println(e.toString());//不能用 e.html() 这里需要输出 DOM
}
//<a href="https://www.cnblogs.com/rope/" target="_blank"><img width="48" height="48" class="pfs" src="//pic.cnblogs.com/face/1491596/20180916131220.png" alt=""/></a>
Elements targetElements = doc.getElementsByAttributeValue("target", "_blank");
System.out.println("=======输出 target-_blank 的 DOM==============");
for (Element e : targetElements) {
System.out.println(e.toString());
}
}
四、Jsoup 使用选择器语法查找 DOM 元素
我们前面通过标签名,Id,Class 样式等来搜索 DOM,这些是不能满足实际开发需求的,很多时候我们需要寻找有规律的 DOM 集合,很多个有规律的标签层次,这时候,选择器就用上了。css jquery 都有,Jsoup 支持 css,jquery 类似的选择器语法。
/**
有层级关系
*/
@Test
public void test3() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements linkElements = doc.select("#post_list .post_item .post_item_body h3 a"); //通过选择器查找所有博客链接 DOM(范围重小到大)
for (Element e : linkElements) {
System.out.println("博客标题:" + e.text());//超链接的内容
}
System.out.println("--------------------带有 href 属性的 a 元素--------------------------------");
Elements hrefElements = doc.select("a[href]"); // 带有 href 属性的 a 元素
for (Element e : hrefElements) {
System.out.println(e.toString());
}
System.out.println("------------------------查找扩展名为.png 的图片----------------------------");
Elements imgElements = doc.select("img[src$=.png]"); // 查找扩展名为.png 的图片 DOM 节点
for (Element e : imgElements) {
System.out.println(e.toString());
}
System.out.println("------------------------获取第一个元素----------------------------");
Element element = doc.getElementsByTag("title").first(); // 获取 tag 是 title 的所有 DOM 元素
String title = element.text(); // 返回元素的文本
System.out.println("网页标题是:" + title);
}
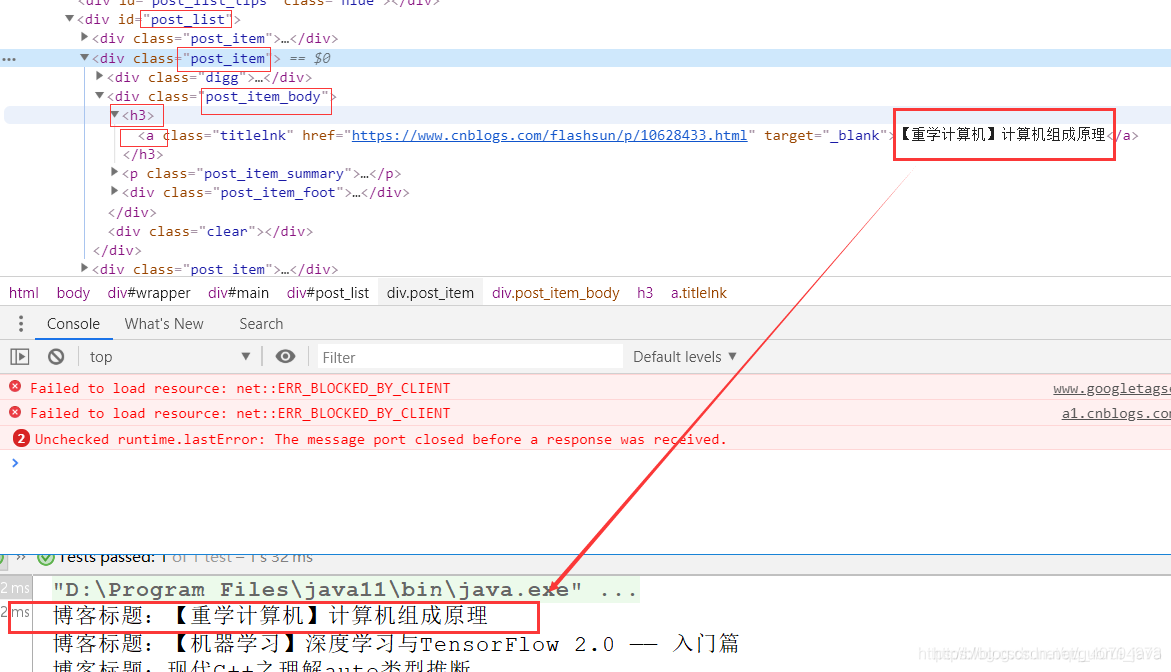
五、Jsoup 获取 DOM 元素属性值
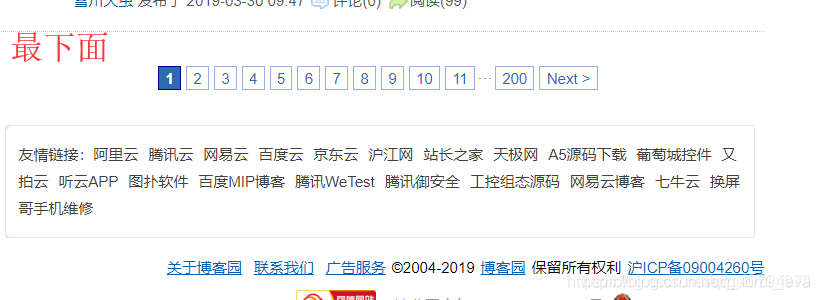
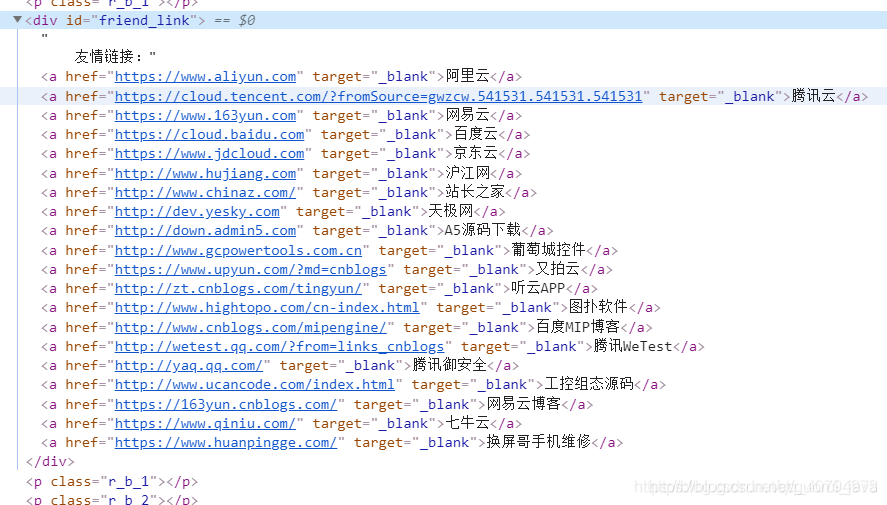
/**
获取 DOM 元素属性值
*/
@Test
public void test4() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements linkElements = doc.select("#post_list .post_item .post_item_body h3 a"); //通过选择器查找所有博客链接 DOM
for (Element e : linkElements) {
System.out.println("博客标题:" + e.text());//获取里面所有的文本
System.out.println("博客地址:" + e.attr("href"));
System.out.println("target:" + e.attr("target"));
}
System.out.println("------------------------友情链接----------------------------");
Element linkElement = doc.select("#friend_link").first();
System.out.println("纯文本:" + linkElement.text());//去掉 html
System.out.println("------------------------Html----------------------------");
System.out.println("Html:" + linkElement.html());
}
/**
获取文章的 url
*/
@Test
public void test5() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements linkElements = doc.select("#post_list .post_item .post_item_body h3 a"); //通过选择器查找所有博客链接 DOM
for (Element e : linkElements) {
System.out.println(e.attr("href"));
}
}
注意:Element 的几个获取内容的方法区别
text()? ? ? ? ? ? 获取的是去掉了 html 元素,也就是只用元素内容
toString()? ? ??DOM
html()? ? ? ? ? 获取里面所有的 html 包括文本
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.junit.Test;
import java.io.IOException;
public class Main {
/**
输入一个网址返回这个网址的字符串
*/
public String getHtml(String str) throws IOException {
CloseableHttpClient httpclient = HttpClients.createDefault(); // 创建 httpclient 实例
HttpGet httpget = new HttpGet(str); // 创建 httpget 实例
CloseableHttpResponse response = httpclient.execute(httpget); // 执行 get 请求
HttpEntity entity = response.getEntity(); // 获取返回实体
String content = EntityUtils.toString(entity, "utf-8");
response.close(); // 关闭流和释放系统资源
return content;
}
/**
爬取 博客园
1、网页标题
2、口号
*/
@Test
public void test() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements elements = doc.getElementsByTag("title"); // 获取 tag 是 title 的所有 DOM 元素
Element element = elements.get(0); // 获取第 1 个元素
String title = element.text(); // 返回元素的文本
System.out.println("网页标题:" + title);
Element element2 = doc.getElementById("site_nav_top"); // 获取 id=site_nav_top 的 DOM 元素
String navTop = element2.text(); // 返回元素的文本
System.out.println("口号:" + navTop);
}
/**
Jsoup 查找 DOM 元素
*/
@Test
public void test2() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements itemElements = doc.getElementsByClass("post_item"); // 根据样式名称来查询 DOM
System.out.println("=======输出 post_item==============");
for (Element e : itemElements) {
System.out.println(e.html());//获取里面所有的 html 包括文本
System.out.println("\n");
}
Elements widthElements = doc.getElementsByAttribute("width"); // 根据属性名称来查询 DOM(id class type 等),用的少一般很难找用这种方法
System.out.println("=======输出 with 的 DOM==============");
for (Element e : widthElements) {
System.out.println(e.toString());//不能用 e.html() 这里需要输出 DOM
}
//<a href="https://www.cnblogs.com/rope/" target="_blank"><img width="48" height="48" class="pfs" src="//pic.cnblogs.com/face/1491596/20180916131220.png" alt=""/></a>
Elements targetElements = doc.getElementsByAttributeValue("target", "_blank");
System.out.println("=======输出 target-_blank 的 DOM==============");
for (Element e : targetElements) {
System.out.println(e.toString());
}
}
/**
有层级关系
*/
@Test
public void test3() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements linkElements = doc.select("#post_list .post_item .post_item_body h3 a"); //通过选择器查找所有博客链接 DOM(范围重小到大)
for (Element e : linkElements) {
System.out.println("博客标题:" + e.text());//超链接的内容
}
System.out.println("--------------------带有 href 属性的 a 元素--------------------------------");
Elements hrefElements = doc.select("a[href]"); // 带有 href 属性的 a 元素
for (Element e : hrefElements) {
System.out.println(e.toString());
}
System.out.println("------------------------查找扩展名为.png 的图片----------------------------");
Elements imgElements = doc.select("img[src$=.png]"); // 查找扩展名为.png 的图片 DOM 节点
for (Element e : imgElements) {
System.out.println(e.toString());
}
System.out.println("------------------------获取第一个元素----------------------------");
Element element = doc.getElementsByTag("title").first(); // 获取 tag 是 title 的所有 DOM 元素
String title = element.text(); // 返回元素的文本
System.out.println("网页标题是:" + title);
}
/**
获取 DOM 元素属性值
*/
@Test
public void test4() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements linkElements = doc.select("#post_list .post_item .post_item_body h3 a"); //通过选择器查找所有博客链接 DOM
for (Element e : linkElements) {
System.out.println("博客标题:" + e.text());//获取里面所有的文本
System.out.println("博客地址:" + e.attr("href"));
System.out.println("target:" + e.attr("target"));
}
System.out.println("------------------------友情链接----------------------------");
Element linkElement = doc.select("#friend_link").first();
System.out.println("纯文本:" + linkElement.text());//去掉 html
System.out.println("------------------------Html----------------------------");
System.ou
t.println("Html:" + linkElement.html());
}
/**
获取文章的 url
*/
@Test
public void test5() throws IOException {
Document doc = Jsoup.parse(getHtml("http://www.cnblogs.com/")); // 解析网页 得到文档对象
Elements linkElements = doc.select("#post_list .post_item .post_item_body h3 a"); //通过选择器查找所有博客链接 DOM
for (Element e : linkElements) {
System.out.println(e.attr("href"));
}
}
}
六、Jsoup 工具类
public class JsoupUtil {
/**
获取 value 值
@param e
@return
*/
public static String getValue(Element e) {
return e.attr("value");
}
/**
获取
<tr>
和
</tr>
之间的文本
@param e
@return
*/
public static String getText(Element e) {
return e.text();
}
/**
识别属性 id 的标签,一般一个 html 页面 id 唯一
@param body
@param id
@return
*/
public static Element getID(String body, String id) {
Document doc = Jsoup.parse(body);
// 所有 #id 的标签
Elements elements = doc.select("#" + id);
// 返回第一个
return elements.first();
}
/**
识别属性 class 的标签
@param body
@param class
@return
*/
public static Elements getClassTag(String body, String classTag) {
Document doc = Jsoup.parse(body);
// 所有 #id 的标签
return doc.select("." + classTag);
}
/**
获取 tr 标签元素组
@param e
@return
*/
public static Elements getTR(Element e) {
return e.getElementsByTag("tr");
}
/**
获取 td 标签元素组
@param e
@return
*/
public static Elements getTD(Element e) {
return e.getElementsByTag("td");
}
/**
获取表元组
@param table
@return
*/
public static List<List<String>> getTables(Element table){
List<List<String>> data = new ArrayList<>();
for (Element etr : table.select("tr")) {
List<String> list = new ArrayList<>();
for (Element etd : etr.select("td")) {
String temp = etd.text();
//增加一行中的一列
list.add(temp);
}
//增加一行
data.add(list);
}
return data;
评论