SpringBoot 集成 ElasticSearch
- 2023-05-06 湖南
本文字数:8241 字
阅读完需:约 27 分钟
这篇文章主要给大家从 0 基础开始用 SpringBoot 集成第三方的插件或者功能。
第一步,导入 jar 包,注意这里的 jar 包版本可能和你导入的不一致,所以需要修改
<properties>
<properties>
<java.version>1.8</java.version>
<elasticsearch.version>7.6.2</elasticsearch.version>
</properties>
<!-- elasticsearch -->
<!--es客户端-->
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
<version>7.6.2</version>
</dependency>
<!--springboot的elasticsearch服务-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
第二步,编写配置类
这段代码是一个基本的 Elasticsearch Java 客户端的配置类,用于创建一个 RestHighLevelClient
实例。
其中 RestHighLevelClient
是 Elasticsearch Java 客户端的高级别别名,是基于 LowLevelClient
之上的封装,提供了一些更加方便的方法和功能。
在这段代码中,使用了 @Value
注解来注入三个配置项,包括 hostname
,port
和 scheme
。这三个配置项分别表示 Elasticsearch 服务器的主机名或 IP 地址,端口号和通信协议。然后使用 RestClient.builder()
方法来创建一个 RestClient 实例,传入 Elasticsearch 服务器的地址和端口号,最后将 RestClient
实例传入 RestHighLevelClient
的构造函数中,即可创建一个 RestHighLevelClient
实例。
需要注意的是,这段代码中的 RestHighLevelClient
实例是一个单例对象,只需要在应用程序启动时创建一次即可,因此这个类应该被配置为一个 Spring Bean,以便在需要时注入到其他类中使用。
import org.apache.http.HttpHost;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestHighLevelClient;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ElasticSearchClientConfig {
@Value("${elasticSearch.hostname}")
private String hostname;
@Value("${elasticSearch.port}")
private Integer port;
@Value("${elasticSearch.scheme}")
private String scheme;
@Bean
public RestHighLevelClient restHighLevelClient(){
return new RestHighLevelClient(
RestClient.builder(new HttpHost(hostname,port,scheme))
);
}
}
第三步,填写 yml
elasticSearch:
hostname: 127.0.0.1
port: 9200
scheme: http
第四步,编写 util 类
这是一个 Java 类,实现了 Elasticsearch API 的一些基本功能。它定义了创建、检查是否存在、删除索引、添加、修改和删除文档以及搜索文档的方法。该类使用 Elasticsearch API 的 RESTful 客户端来执行这些操作。
以下是每种方法的概述:
createIndex(字符串索引)
:使用给定的名称创建一个索引。existIndex(字符串索引)
:检查是否存在具有给定名称的索引。deleteIndex(字符串索引)
:删除具有给定名称的索引。addDocument(动态动态,字符串索引)
:使用给定的名称将文档添加到索引中。existDocument(字符串索引,字符串文档)
:检查具有给定 ID 的文档是否存在于具有给定名称的索引中。getDocument(字符串索引,字符串文档)
:从具有给定名称的索引中检索具有给定 ID 的文档。updateDocument(动态动态、字符串索引、字符串文档)
:在具有给定名称的索引中更新具有给定 ID 的文档。deleteDocument(字符串索引,字符串文档)
:从具有给定名称的索引中删除具有给定 ID 的文档。bulkAddDocument(List<Dynamic>dynamics)
:在一个批次中将多个具有给定名称的文档添加到索引中。searchDocument(字符串索引)
:根据搜索查询在索引中搜索具有给定名称的文档。
import com.alibaba.fastjson.JSON;
import com.wangfugui.apprentice.dao.domain.Dynamic;
import lombok.extern.slf4j.Slf4j;
import org.elasticsearch.action.admin.indices.delete.DeleteIndexRequest;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.delete.DeleteRequest;
import org.elasticsearch.action.delete.DeleteResponse;
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.action.get.GetResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.index.IndexResponse;
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.action.support.master.AcknowledgedResponse;
import org.elasticsearch.action.update.UpdateRequest;
import org.elasticsearch.action.update.UpdateResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.client.indices.CreateIndexRequest;
import org.elasticsearch.client.indices.CreateIndexResponse;
import org.elasticsearch.client.indices.GetIndexRequest;
import org.elasticsearch.common.unit.TimeValue;
import org.elasticsearch.common.xcontent.XContentType;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.index.query.TermQueryBuilder;
import org.elasticsearch.rest.RestStatus;
import org.elasticsearch.search.SearchHit;
import org.elasticsearch.search.builder.SearchSourceBuilder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Component;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
/**
* @author MaSiyi
* @version 1.0.0 2021/11/25
* @since JDK 1.8.0
*/
@Component
@Slf4j
public class ElasticSearchUtil {
@Autowired
@Qualifier("restHighLevelClient")
private RestHighLevelClient client;
//索引的创建
public CreateIndexResponse createIndex(String index) throws IOException {
//1.创建索引的请求
CreateIndexRequest request = new CreateIndexRequest(index);
//2客户端执行请求,请求后获得响应
CreateIndexResponse response = client.indices().create(request, RequestOptions.DEFAULT);
log.info("索引的创建{}", response);
return response;
}
//索引是否存在
public Boolean existIndex(String index) throws IOException {
//1.创建索引的请求
GetIndexRequest request = new GetIndexRequest(index);
//2客户端执行请求,请求后获得响应
boolean exist = client.indices().exists(request, RequestOptions.DEFAULT);
log.info("索引是否存在-----" + exist);
return exist;
}
//删除索引
public Boolean deleteIndex(String index) throws IOException {
DeleteIndexRequest request = new DeleteIndexRequest(index);
AcknowledgedResponse delete = client.indices().delete(request, RequestOptions.DEFAULT);
log.info("删除索引--------" + delete.isAcknowledged());
return delete.isAcknowledged();
}
//添加文档
public IndexResponse addDocument(Dynamic dynamic, String index) throws IOException {
IndexRequest request = new IndexRequest(index);
//设置超时时间
request.timeout("1s");
//将数据放到json字符串
request.source(JSON.toJSONString(dynamic), XContentType.JSON);
//发送请求
IndexResponse response = client.index(request, RequestOptions.DEFAULT);
log.info("添加文档-------" + response.toString());
log.info("添加文档-------" + response.status());
return response;
}
//文档是否存在
public Boolean existDocument(String index, String documents) throws IOException {
//文档的 没有index
GetRequest request = new GetRequest(index, documents);
//没有indices()了
boolean exist = client.exists(request, RequestOptions.DEFAULT);
log.info("文档是否存在-----" + exist);
return exist;
}
//获取文档
public GetResponse getDocument(String index, String documents) throws IOException {
GetRequest request = new GetRequest(index, documents);
GetResponse response = client.get(request, RequestOptions.DEFAULT);
log.info("获取文档-----" + response.getSourceAsString());
log.info("获取文档-----" + response);
return response;
}
//修改文档
public UpdateResponse updateDocument(Dynamic dynamic, String index, String documents) throws IOException {
//修改是id为1的
UpdateRequest request = new UpdateRequest(index, documents);
request.timeout("1s");
request.doc(JSON.toJSONString(dynamic), XContentType.JSON);
UpdateResponse response = client.update(request, RequestOptions.DEFAULT);
log.info("修改文档-----" + response);
log.info("修改文档-----" + response.status());
return response;
}
//删除文档
public RestStatus deleteDocument(String index, String documents) throws IOException {
DeleteRequest request = new DeleteRequest(index, documents);
request.timeout("1s");
DeleteResponse response = client.delete(request, RequestOptions.DEFAULT);
log.info("删除文档------" + response.status());
return response.status();
}
//批量添加文档
public BulkResponse bulkAddDocument(List<Dynamic> dynamics) throws IOException {
//批量操作的Request
BulkRequest request = new BulkRequest();
request.timeout("1s");
//批量处理请求
for (int i = 0; i < dynamics.size(); i++) {
request.add(
new IndexRequest("lisen_index")
.id("" + (i + 1))
.source(JSON.toJSONString(dynamics.get(i)), XContentType.JSON)
);
}
BulkResponse response = client.bulk(request, RequestOptions.DEFAULT);
//response.hasFailures()是否是失败的
log.info("批量添加文档-----" + response.hasFailures());
// 结果:false为成功 true为失败
return response;
}
//查询文档
public SearchResponse searchDocument(String index) throws IOException {
SearchRequest request = new SearchRequest(index);
//构建搜索条件
SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();
//设置了高亮
sourceBuilder.highlighter();
//term name为cyx1的
TermQueryBuilder termQueryBuilder = QueryBuilders.termQuery("name", "cyx1");
sourceBuilder.query(termQueryBuilder);
sourceBuilder.timeout(new TimeValue(60, TimeUnit.SECONDS));
request.source(sourceBuilder);
SearchResponse response = client.search(request, RequestOptions.DEFAULT);
log.info("查询文档-----" + JSON.toJSONString(response.getHits()));
log.info("=====================");
for (SearchHit documentFields : response.getHits().getHits()) {
log.info("查询文档--遍历参数--" + documentFields.getSourceAsMap());
}
return response;
}
public IndexResponse addDocumentId(Dynamic dynamic, String index, String id) throws IOException {
IndexRequest request = new IndexRequest(index);
//设置超时时间
request.id(id);
//将数据放到json字符串
request.source(JSON.toJSONString(dynamic), XContentType.JSON);
//发送请求
IndexResponse response = client.index(request, RequestOptions.DEFAULT);
log.info("添加文档-------" + response.toString());
log.info("添加文档-------" + response.status());
return response;
}
}
第五步,编写 controller 类
这是一个 Java 类,实现了 Elasticsearch API 的一些基本功能。它定义了用于创建、检查存在性、删除索引、添加、修改和删除文档,以及搜索文档的方法。该类使用 Elasticsearch API 的 RESTful 客户端执行这些操作。
以下是每个方法的概述:
createIndex(String index)
创建索引的方法。existIndex(String index)
检查给定名称的索引是否存在的方法。deleteIndex(String index)
删除给定名称的索引的方法。addDocument(Dynamic dynamic, String index)
将文档添加到给定名称的索引的方法。existDocument(String index, String documents)
检查给定名称的索引中是否存在具有给定 ID 的文档的方法。getDocument(String index, String documents)
从给定名称的索引中检索具有给定 ID 的文档的方法。updateDocument(Dynamic dynamic, String index, String documents)
在给定名称的索引中更新具有给定 ID 的文档的方法。deleteDocument(String index, String documents)
从给定名称的索引中删除具有给定 ID 的文档的方法。bulkAddDocument(List<Dynamic> dynamics)
在单个批处理中将多个文档添加到给定名称的索引的方法。searchDocument(String index)
基于搜索查询在给定名称的索引中搜索文档的方法。
import com.wangfugui.apprentice.common.util.ElasticSearchUtil;
import com.wangfugui.apprentice.common.util.ResponseUtils;
import com.wangfugui.apprentice.dao.domain.Dynamic;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
import java.util.List;
/**
* @author MaSiyi
* @version 1.0.0 2021/11/25
* @since JDK 1.8.0
*/
@RestController
@RequestMapping("/elasticSearch")
@Api(tags = "elasticSearch操作")
public class ElasticSearchController {
@Autowired
private ElasticSearchUtil elasticSearchUtil;
/**索引的创建*/
@PostMapping("/createIndex")
@ApiOperation("索引的创建")
public ResponseUtils createIndex(@RequestParam String index) throws IOException {
return ResponseUtils.success(elasticSearchUtil.createIndex(index));
}
/**索引是否存在*/
@GetMapping("/existIndex")
@ApiOperation("索引是否存在")
public ResponseUtils existIndex(@RequestParam String index) throws IOException {
return ResponseUtils.success(elasticSearchUtil.existIndex(index));
}
/**删除索引*/
@DeleteMapping("/deleteIndex")
@ApiOperation("删除索引")
public ResponseUtils deleteIndex(@RequestParam String index) throws IOException {
return ResponseUtils.success(elasticSearchUtil.deleteIndex(index));
}
/**添加文档*/
@PostMapping("/addDocument")
@ApiOperation("添加文档随机id")
public ResponseUtils addDocument(@RequestBody Dynamic dynamic, @RequestParam String index) throws IOException {
return ResponseUtils.success(elasticSearchUtil.addDocument(dynamic,index));
}
/**添加文档*/
@PostMapping("/addDocument")
@ApiOperation("添加文档自定义id")
public ResponseUtils addDocumentId(@RequestBody Dynamic dynamic, @RequestParam String index,@RequestParam String id) throws IOException {
return ResponseUtils.success(elasticSearchUtil.addDocumentId(dynamic,index,id));
}
/**文档是否存在*/
@GetMapping("/existDocument")
@ApiOperation("文档是否存在")
public ResponseUtils existDocument(@RequestParam String index, @RequestParam String documents) throws IOException {
return ResponseUtils.success(elasticSearchUtil.existDocument(index,documents));
}
/**获取文档*/
@GetMapping("/getDocument")
@ApiOperation("获取文档")
public ResponseUtils getDocument(@RequestParam String index, @RequestParam String documents) throws IOException {
return ResponseUtils.success(elasticSearchUtil.getDocument(index,documents));
}
/**修改文档*/
@ApiOperation("修改文档")
@PutMapping("/updateDocument")
public ResponseUtils updateDocument(@RequestBody Dynamic dynamic, @RequestParam String index, @RequestParam String documents) throws IOException {
return ResponseUtils.success(elasticSearchUtil.updateDocument(dynamic,index,documents));
}
/**删除文档*/
@ApiOperation("删除文档")
@DeleteMapping("/deleteDocument")
public ResponseUtils deleteDocument(@RequestParam String index, @RequestParam String documents) throws IOException {
return ResponseUtils.success(elasticSearchUtil.deleteDocument(index,documents));
}
/**批量添加文档*/
@ApiOperation("批量添加文档")
@PostMapping("/bulkAddDocument")
public ResponseUtils bulkAddDocument(@RequestBody List<Dynamic> dynamics) throws IOException {
return ResponseUtils.success(elasticSearchUtil.bulkAddDocument(dynamics));
}
/**查询文档*/
@ApiOperation("查询文档")
@GetMapping("/searchDocument")
public ResponseUtils searchDocument(@RequestParam String index) throws IOException {
return ResponseUtils.success(elasticSearchUtil.searchDocument(index));
}
}
第六步,测试即可
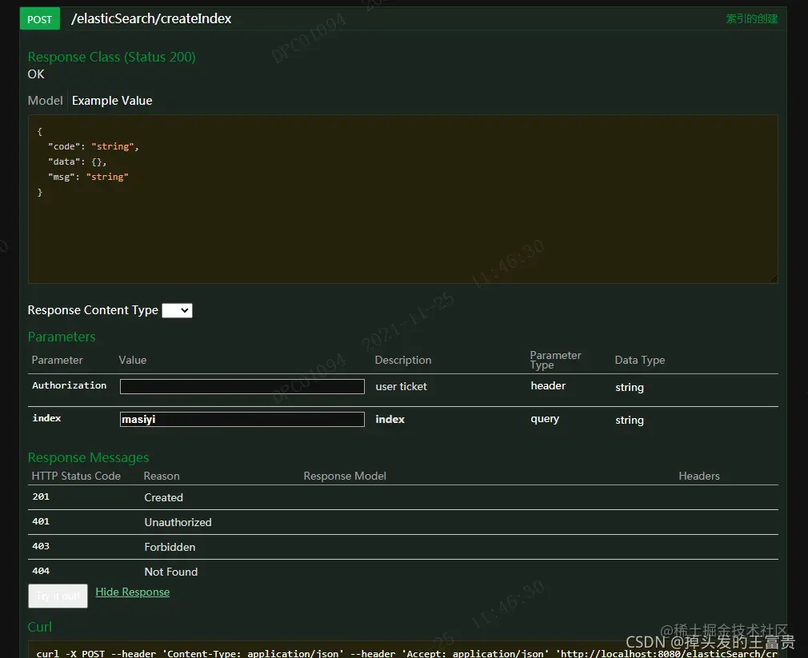
成功!!
作者:掉头发的王富贵
链接:https://juejin.cn/post/7229493617366384697
来源:稀土掘金
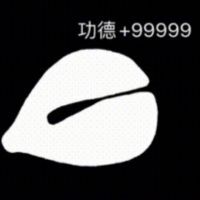
做梦都在改BUG
还未添加个人签名 2021-07-28 加入
公众号:该用户快成仙了
评论