HashMap 源码解析
- 2023-09-02 广东
本文字数:4580 字
阅读完需:约 15 分钟
大纲
基础入门
数组的优势/劣势
链表的优势/劣势
有没有一种方式整合 2 种数据结构的优势?散列表
散列表的特点?
什么是哈希?
HashMap 原理讲解
HashMap 的继承体系是怎样的?
Node 的数据结构分析?
底层存储结构分析?
put 数据原理分析?
什么是 hash 碰撞?
什么是链化?
jdk8 为什么引入红黑树?
HashMap 扩容原理?
手撕源码
核心的概念
核心的属性
默认的初始化容量:static final int DEFAULT_INITIAL_CAPACITY = 1 << 4
最大容量:static final int MAXIMUM_CAPACITY = 1 << 30
默认的负载因子:static final float DEFAULT_LOAD_FACTOR = 0.75f
树化阈值:static final int TREEIFY_THRESHOLD = 8
树退化阈值:static final int UNTREEIFY_THRESHOLD = 6
可以被树化的散列表最小容量:static final int MIN_TREEIFY_CAPACITY = 64
散列表:transient Node<K,V>[] table
第一次使用才初始化
键值对的数量:transient int size
扩容阈值:int threshold
负载因子:final float loadFactor
Node
散列表节点的基本类型
static class Node<K,V> implements Map.Entry<K,V> {
final int hash;
final K key;
V value;
Node<K,V> next;
}
构造器
HashMap 提供了多个构造器,最终调用的是下面这个:
public HashMap(int initialCapacity, float loadFactor) {
// 参数校验 ...
this.loadFactor = loadFactor;
this.threshold = tableSizeFor(initialCapacity);
}
/**
* Returns a power of two size for the given target capacity.
*/
static final int tableSizeFor(int cap) {
int n = -1 >>> Integer.numberOfLeadingZeros(cap - 1);
return (n < 0) ? 1 : (n >= MAXIMUM_CAPACITY) ? MAXIMUM_CAPACITY : n + 1;
}
代码很简单,唯一需要搞清楚的就是为什么要调用tableSizeFor
方法把初始化容器大小要处理成 2 的次方?
Chatgpt 给出的答案如下:
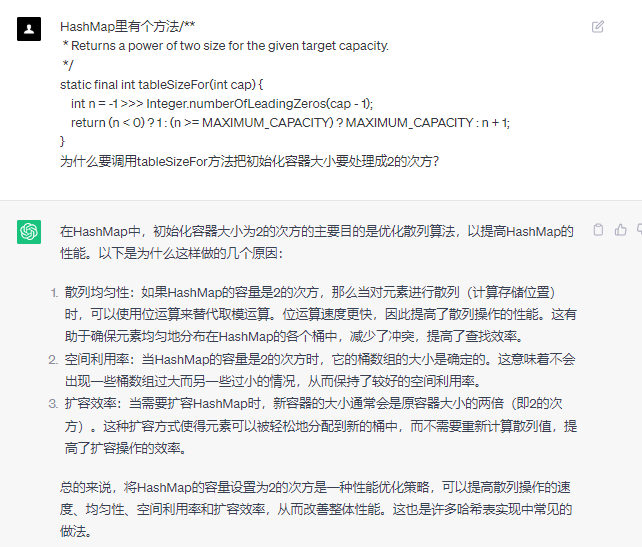
构造器还支持传入一个 Map 来实例化
写一个简单的 junit case 去 debug 一下
@Test
void testHashMapInstance() {
HashMap<Object, Object> oldMap = new HashMap<>();
oldMap.put("name", "sourceCodeExplorer");
oldMap.put("age", 18);
oldMap.put("hobby", new String[]{"sport,reading"});
System.out.println(oldMap);
HashMap<Object, Object> newMap = new HashMap<>(oldMap);
System.out.println(newMap);
}
public HashMap(Map<? extends K, ? extends V> m) {
this.loadFactor = DEFAULT_LOAD_FACTOR;
putMapEntries(m, false);
}
/**
* Implements Map.putAll and Map constructor.
*
* @param m the map
* @param evict false when initially constructing this map, else
* true (relayed to method afterNodeInsertion).
*/
final void putMapEntries(Map<? extends K, ? extends V> m, boolean evict) {
int s = m.size();
if (s > 0) {
if (table == null) { // pre-size
float ft = ((float)s / loadFactor) + 1.0F;
int t = ((ft < (float)MAXIMUM_CAPACITY) ?
(int)ft : MAXIMUM_CAPACITY);
if (t > threshold)
threshold = tableSizeFor(t);
}
else if (s > threshold)
resize();
// 拷贝原map的所有节点到新的map中
for (Map.Entry<? extends K, ? extends V> e : m.entrySet()) {
K key = e.getKey();
V value = e.getValue();
putVal(hash(key), key, value, false, evict);
}
}
}
存值方法 put()
public V put(K key, V value) {
return putVal(hash(key), key, value, false, true);
}
// 计算哈希值
static final int hash(Object key) {
int h;
// key的哈希值与右移16位的结果进行异或运算来实现
// 好处:1)减少冲突,降低碰撞 2) 分布更均匀
return (key == null) ? 0 : (h = key.hashCode()) ^ (h >>> 16);
}
final V putVal(int hash, K key, V value, boolean onlyIfAbsent,
boolean evict) {
Node<K,V>[] tab; Node<K,V> p; int n, i;
if ((tab = table) == null || (n = tab.length) == 0)
n = (tab = resize()).length;
if ((p = tab[i = (n - 1) & hash]) == null)
tab[i] = newNode(hash, key, value, null);
else {
Node<K,V> e; K k;
if (p.hash == hash &&
((k = p.key) == key || (key != null && key.equals(k))))
e = p;
else if (p instanceof TreeNode)
e = ((TreeNode<K,V>)p).putTreeVal(this, tab, hash, key, value);
else {
for (int binCount = 0; ; ++binCount) {
if ((e = p.next) == null) {
p.next = newNode(hash, key, value, null);
if (binCount >= TREEIFY_THRESHOLD - 1) // -1 for 1st
treeifyBin(tab, hash);
break;
}
if (e.hash == hash &&
((k = e.key) == key || (key != null && key.equals(k))))
break;
p = e;
}
}
if (e != null) { // existing mapping for key
V oldValue = e.value;
if (!onlyIfAbsent || oldValue == null)
e.value = value;
afterNodeAccess(e);
return oldValue;
}
}
++modCount;
if (++size > threshold)
resize();
afterNodeInsertion(evict);
return null;
}
扩容方法 resize()
final Node<K,V>[] resize() {
Node<K,V>[] oldTab = table;
int oldCap = (oldTab == null) ? 0 : oldTab.length;
int oldThr = threshold;
int newCap, newThr = 0;
if (oldCap > 0) {
if (oldCap >= MAXIMUM_CAPACITY) {
threshold = Integer.MAX_VALUE;
return oldTab;
}
else if ((newCap = oldCap << 1) < MAXIMUM_CAPACITY &&
oldCap >= DEFAULT_INITIAL_CAPACITY)
newThr = oldThr << 1; // double threshold
}
else if (oldThr > 0) // initial capacity was placed in threshold
newCap = oldThr;
else { // zero initial threshold signifies using defaults
newCap = DEFAULT_INITIAL_CAPACITY;
newThr = (int)(DEFAULT_LOAD_FACTOR * DEFAULT_INITIAL_CAPACITY);
}
if (newThr == 0) {
float ft = (float)newCap * loadFactor;
newThr = (newCap < MAXIMUM_CAPACITY && ft < (float)MAXIMUM_CAPACITY ?
(int)ft : Integer.MAX_VALUE);
}
threshold = newThr;
@SuppressWarnings({"rawtypes","unchecked"})
Node<K,V>[] newTab = (Node<K,V>[])new Node[newCap];
table = newTab;
if (oldTab != null) {
for (int j = 0; j < oldCap; ++j) {
Node<K,V> e;
if ((e = oldTab[j]) != null) {
oldTab[j] = null;
if (e.next == null)
newTab[e.hash & (newCap - 1)] = e;
else if (e instanceof TreeNode)
((TreeNode<K,V>)e).split(this, newTab, j, oldCap);
else { // preserve order
Node<K,V> loHead = null, loTail = null;
Node<K,V> hiHead = null, hiTail = null;
Node<K,V> next;
do {
next = e.next;
if ((e.hash & oldCap) == 0) {
if (loTail == null)
loHead = e;
else
loTail.next = e;
loTail = e;
}
else {
if (hiTail == null)
hiHead = e;
else
hiTail.next = e;
hiTail = e;
}
} while ((e = next) != null);
if (loTail != null) {
loTail.next = null;
newTab[j] = loHead;
}
if (hiTail != null) {
hiTail.next = null;
newTab[j + oldCap] = hiHead;
}
}
}
}
}
return newTab;
}
取值方法 get()
public V get(Object key) {
Node<K,V> e;
return (e = getNode(hash(key), key)) == null ? null : e.value;
}
final Node<K,V> getNode(int hash, Object key) {
Node<K,V>[] tab; Node<K,V> first, e; int n; K k;
if ((tab = table) != null && (n = tab.length) > 0 &&
(first = tab[(n - 1) & hash]) != null) {
if (first.hash == hash && // always check first node
((k = first.key) == key || (key != null && key.equals(k))))
return first;
if ((e = first.next) != null) {
if (first instanceof TreeNode)
return ((TreeNode<K,V>)first).getTreeNode(hash, key);
do {
if (e.hash == hash &&
((k = e.key) == key || (key != null && key.equals(k))))
return e;
} while ((e = e.next) != null);
}
}
return null;
}
经典错误
初始容量计算错误
需求是存 2 个元素
// 错误
Map<String,Object> map = new HashMap<>(2);
map.put("name", "code");
map.put("age", 28);
// 正确
Map<String, Object> map = new HashMap<>((int) (2 / 0.75f + 1));
map.put("name", "code");
map.put("age", 28);
使用完 map 不做 clear
See Also
小刘源码讲师的 HashMap 视频教程:https://www.bilibili.com/video/BV1LJ411W7dP/?vd_source=3afcc36db719cf17067a572101ab4393
版权声明: 本文为 InfoQ 作者【前行】的原创文章。
原文链接:【http://xie.infoq.cn/article/0b47c0cd97b58280bc7497165】。文章转载请联系作者。
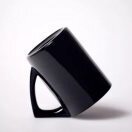
前行
还未添加个人签名 2018-09-06 加入
还未添加个人简介
评论