Python 基础(十四) | Python 之禅与时间复杂度分析
⭐本专栏旨在对 Python 的基础语法进行详解,精炼地总结语法中的重点,详解难点,面向零基础及入门的学习者,通过专栏的学习可以熟练掌握 python 编程,同时为后续的数据分析,机器学习及深度学习的代码能力打下坚实的基础。
🔥本文已收录于 Python 基础系列专栏: Python基础系列教程 欢迎订阅,持续更新。
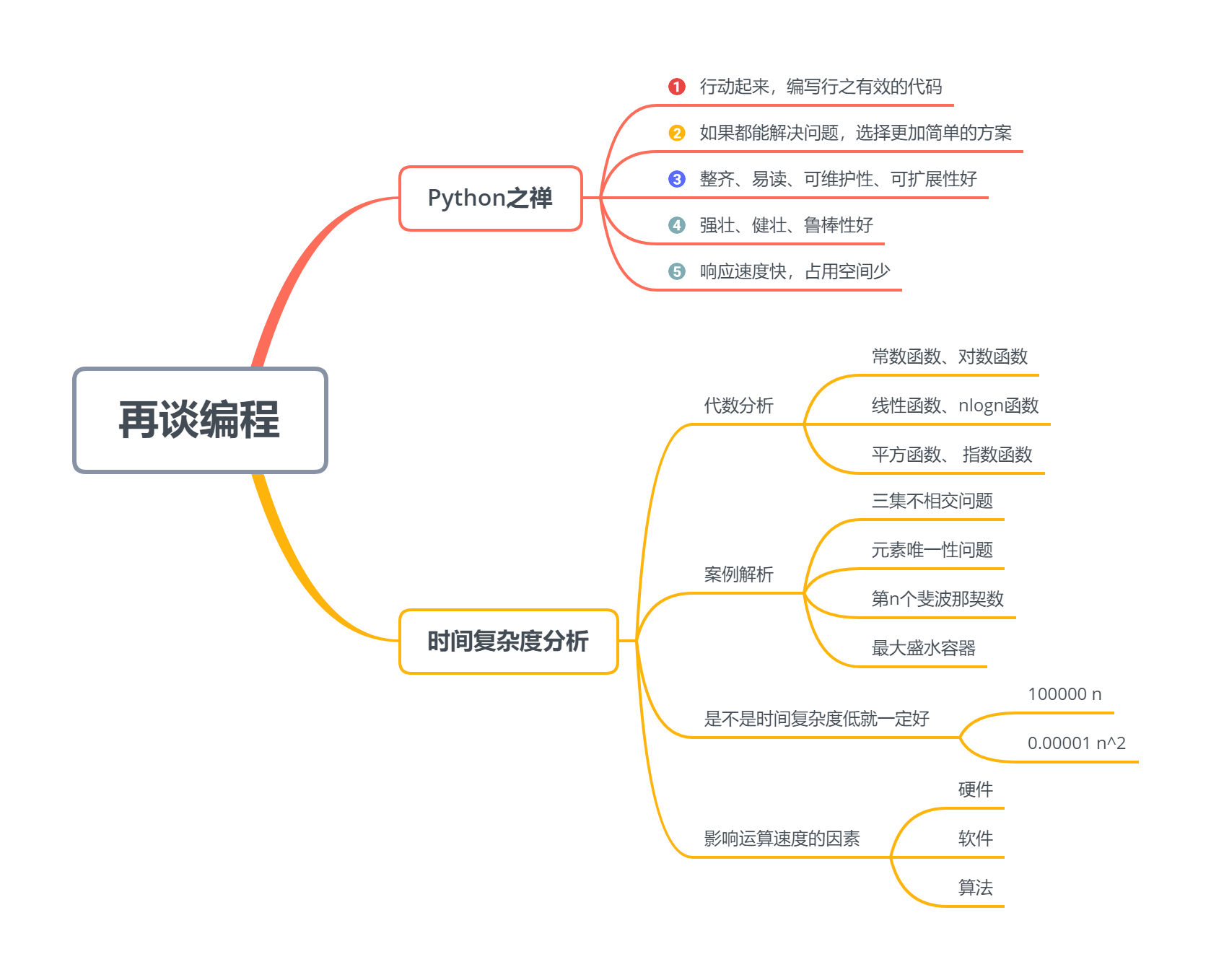
14.1 Python 之禅
Beautiful is better than ugly
整齐、易读胜过混乱、晦涩
Simple is better than complex
简约胜过复杂
Complex is better than complicated
复杂胜过晦涩
Flat is better than nested
扁平胜过嵌套
Now is better than never.
Although never is often better than right now.
理解一:先行动起来,编写行之有效的代码,不要企图一开始就编写完美无缺的代码
理解二:做比不做要好,但是盲目的不加思考的去做还不如不做
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
如果方案很难解释,很可能不是有一个好的方案,反之亦然
一些感悟
1、首先要行动起来,编写行之有效的代码;
2、如果都能解决问题,选择更加简单的方案;
3、整齐、易读、可维护性、可扩展性好;
4、强壮、健壮、鲁棒性好;
5、响应速度快,占用空间少。
有些时候,鱼和熊掌不可兼得,根据实际情况进行相应的取舍
14.2 时间复杂度分析
14.2.1 代数分析
求最大值和排序
寻找最大值的时间复杂度为 O(n)
选择排序时间复杂度 O(n^2)
代数分析
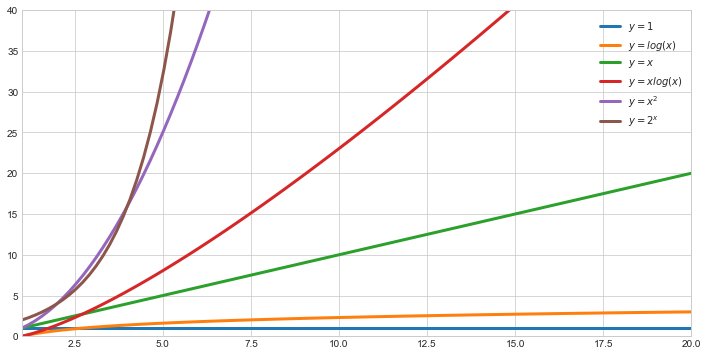
我们的最爱:常数函数和对数函数
勉强接受:线性函数和 nlogn 函数
难以承受:平方函数和指数函数
14.2.2 三集不相交问题
问题描述:
假设有 A、B、C 三个序列,任一序列内部没有重复元素,欲知晓三个序列交集是否为空
14.2.3 元素唯一性问题
问题描述:A 中的元素是否唯一
时间复杂度为 O(nlogn)
14.2.4 第 n 个斐波那契数
a(n+2) = a(n+1) + a(n)
O(2^n)
O(n)
14.2.5 最大盛水容器
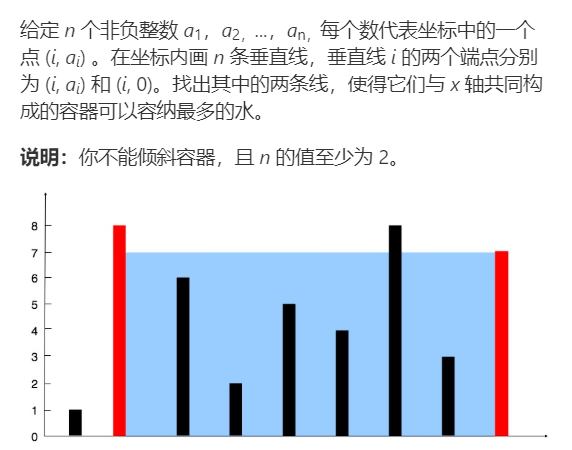
暴力求解——双循环
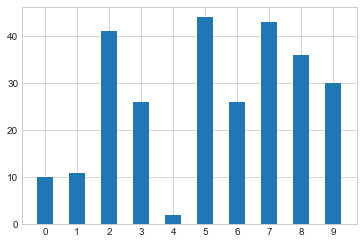
双向指针
14.2.6 是不是时间复杂度低就一定好?
不一定,试比较 100000 VS 0.00001
14.2.7 影响运算速度的因素
硬件
软件
算法
版权声明: 本文为 InfoQ 作者【timerring】的原创文章。
原文链接:【http://xie.infoq.cn/article/c8c073e05d850d598ff5ed3ae】。未经作者许可,禁止转载。
评论