ES6 新特性(五)
作者:阡陌r
- 2023-10-29 江苏
本文字数:1344 字
阅读完需:约 4 分钟
class
基本语法
// ES5通过构造函数实例化对象
function Phone(brand, price) {
this.brand = brand;
this.price = price;
}
Phone.prototype.call = function() {
console.log('I can call!');
}
//实例化对象
let huawei = new Phone('Huawei', 5999);
huawei.call(); // I can call!
console.log(huawei);
// ES6通过类实例化对象
class Phone{
constructor(brand, price) {
this.brand = brand;
this.price = price;
}
call() {
console.log('I can call!');
}
}
let onePlus = new Phone('onePlus', 2999);
console.log(onePlus);
复制代码
静态成员
class 的静态成员属于 class,不属于对象,且对象无法访问静态成员(区别于 Java 语法)
class Phone{
static size = '5.5inch';
static change() {
console.log('I can change the world');
}
}
let xiaomi = new Phone();
console.log(Phone.size); // 5.5inch
Phone.change(); // I can change the world
console.log(xiaomi.size); // undefined
xiaomi.change(); // Uncaught TypeError: xiaomi.change is not a function
复制代码
继承
ES5 使用构造函数实现继承
// 手机
function Phone(brand, price) {
this.brand = brand;
this.price = price;
}
Phone.prototype.call = function() {
console.log('I can call!');
}
// 智能手机
function SmartPhone(brand, price, color, size) {
Phone.call(this, brand, price);
this.color = color;
this.size = size;
}
// 设置子构造函数的原型
SmartPhone.prototype = new Phone;
SmartPhone.constructor = SmartPhone; // 校正
// 声明子类方法
SmartPhone.prototype.phote = function() {
console.log('I can take photo');
}
SmartPhone.prototype.playGame = function() {
console.log('I can play game');
}
let meizu = new SmartPhone('meizu', 2999, 'black', '6.2inch');
console.log(meizu); // 见【图1】
复制代码
图 1
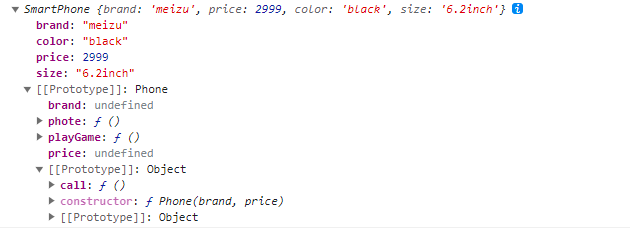
ES6class 实现继承
class Phone{
// 构造方法
constructor(brand, price) {
this.brand = brand;
this.price = price;
}
// 成员属性
call() {
console.log('I can call');
}
}
class SmartPhone extends Phone{
// 构造方法
constructor(brand, price, color, size) {
super(brand, price);
this.color = color;
this.size = size;
}
photo() {
console.log('I can take photo');
}
playGame() {
console.log('I can play game');
}
}
let vivo = new SmartPhone('vivo', 1999, 'white', '6.8inch');
console.log(vivo); // 见【图2】
复制代码
【图 2】
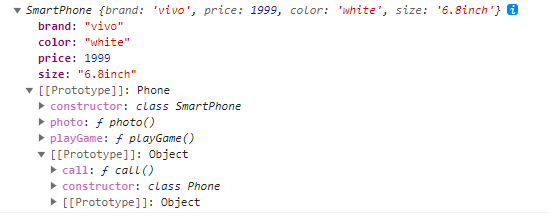
子类对父类方法的重写
在子类中声明同名的方法,会“覆盖”父类的方法。原理就是作用域链
getter 与 setter 的设置
class Phone{
get price() {
console.log('价格属性被读取了');
return '19999';
}
set price(newVal) {
console.log('价格属性被修改了')
}
}
let iPhone = new Phone();
let price = iPhone.price; // 价格属性被读取了
console.log(price); // 19999
iPhone.price = 'free'; // 价格属性被修改了
复制代码
getter&setter 的应用场景:
getter 适用于获取动态数据,如平均值等需要计算所得的值
setter 适用于拦截非法值
划线
评论
复制
发布于: 刚刚阅读数: 7
版权声明: 本文为 InfoQ 作者【阡陌r】的原创文章。
原文链接:【http://xie.infoq.cn/article/fcd43fa0eb5916158330da3dd】。文章转载请联系作者。
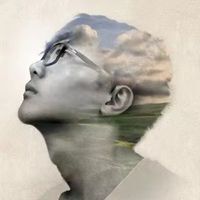
阡陌r
关注
即行于阡陌,也归于浩瀚 2018-03-26 加入
还未添加个人简介
评论