ARTS-WEEK5-23.9.11~23.9.17
Algorithm 一道算法题
无重复最长子串:详见https://leetcode.cn/problems/longest-substring-without-repeating-characters/
Review 读一篇英文文章
Act with Prudencehttps://97-things-every-x-should-know.gitbooks.io/97-things-every-programmer-should-know/content/en/thing_01/
"Whatever you undertake, act with prudence and consider the consequences" Anon
无论你做什么,都要谨慎行事并考虑后果
No matter how comfortable a schedule looks at the beginning of an iteration, you can't avoid being under pressure some of the time. If you find yourself having to choose between "doing it right" and "doing it quick" it is often appealing to "do it quick" on the understanding that you'll come back and fix it later. When you make this promise to yourself, your team, and your customer, you mean it. But all too often the next iteration brings new problems and you become focused on them. This sort of deferred work is known as technical debt and it is not your friend. Specifically, Martin Fowler calls this deliberate technical debt in his taxonomy of technical debt, which should not be confused with inadvertent technical debt.
无论迭代开始时的计划看起来多么舒适,你都无法避免在某些时候面临压力。如果你发现自己不得不在“做正确”和“做快速”之间选择,通常会倾向于“做快速”,理解为之后再来修复它。当你向自己、团队和客户做出这个承诺时,你是认真的。但往往下一个迭代会带来新的问题,你会集中精力解决它们。这种被推迟的工作被称为技术债务,它不是你的朋友。具体来说,马丁·福勒在他的技术债务分类中将这称为有意的技术债务,不应与无意的技术债务混淆。
Technical debt is like a loan: You benefit from it in the short-term, but you have to pay interest on it until it is fully paid off. Shortcuts in the code make it harder to add features or refactor your code. They are breeding grounds for defects and brittle test cases. The longer you leave it, the worse it gets. By the time you get around to undertaking the original fix there may be a whole stack of not-quite-right design choices layered on top of the original problem making the code much harder to refactor and correct. In fact, it is often only when things have got so bad that you must fix it, that you actually do go back to fix it. And by then it is often so hard to fix that you really can't afford the time or the risk.
技术债务就像一笔贷款:你在短期内会从中获益,但你必须支付利息,直到它完全偿还为止。在代码中采用的捷径会使添加功能或重构代码变得更加困难。它们是缺陷和脆弱测试案例的温床。你拖延的时间越长,问题就会变得越糟。当你最终决定着手解决最初的问题时,可能会有一堆不完全正确的设计选择堆叠在最初的问题之上,使得代码变得更加难以重构和修复。事实上,通常只有在事情变得如此糟糕,你不得不解决它时,你才会真正回去修复它。而到那时,它通常已经如此难以修复,以至于你真的无法承担时间或风险。
There are times when you must incur technical debt to meet a deadline or implement a thin slice of a feature. Try not to be in this position, but if the situation absolutely demands it, then go ahead. But (and this is a big BUT) you must track technical debt and pay it back quickly or things go rapidly downhill. As soon as you make the decision to compromise, write a task card or log it in your issue tracking system to ensure that it does not get forgotten.
有时候,为了满足截止日期或实施功能的一小部分,你必须承担技术债务。尽量避免陷入这种情况,但如果情况绝对需要,那就去做。但(这是一个很大的“但是”)你必须跟踪技术债务,并迅速偿还,否则情况会迅速恶化。一旦你决定妥协,就写下一个任务卡或在问题跟踪系统中记录下来,以确保不会被遗忘。
If you schedule repayment of the debt in the next iteration, the cost will be minimal. Leaving the debt unpaid will accrue interest and that interest should be tracked to make the cost visible. This will emphasize the effect on business value of the project's technical debt and enables appropriate prioritization of the repayment. The choice of how to calculate and track the interest will depend on the particular project, but track it you must.
如果你在下一个迭代中安排还清债务,成本将最小化。不还债务会导致利息累积,应该跟踪这些利息以使成本可见。这将强调项目技术债务对业务价值的影响,并使还款得以适当优先考虑。如何计算和跟踪利息的选择将取决于具体项目,但你必须跟踪它。
Pay off technical debt as soon as possible. It would be imprudent to do otherwise.
尽快偿还技术债务是明智的选择,否则会是不明智的决定。
By Seb Rose
Technique/Tips 分享一个小技术
JaveScript 对象的继承机制
什么是原型: 原型是面向对应编程的一种设计模式,JaveScript 基于原型,实现继承、封装。
原型是 JavaScript 的一个强大且非常灵活的功能,使得重用代码和组合对象成为可能。
看一个小例子
当我们在控制台输入 myObject.的时候,会多出许多属性和方法,如:
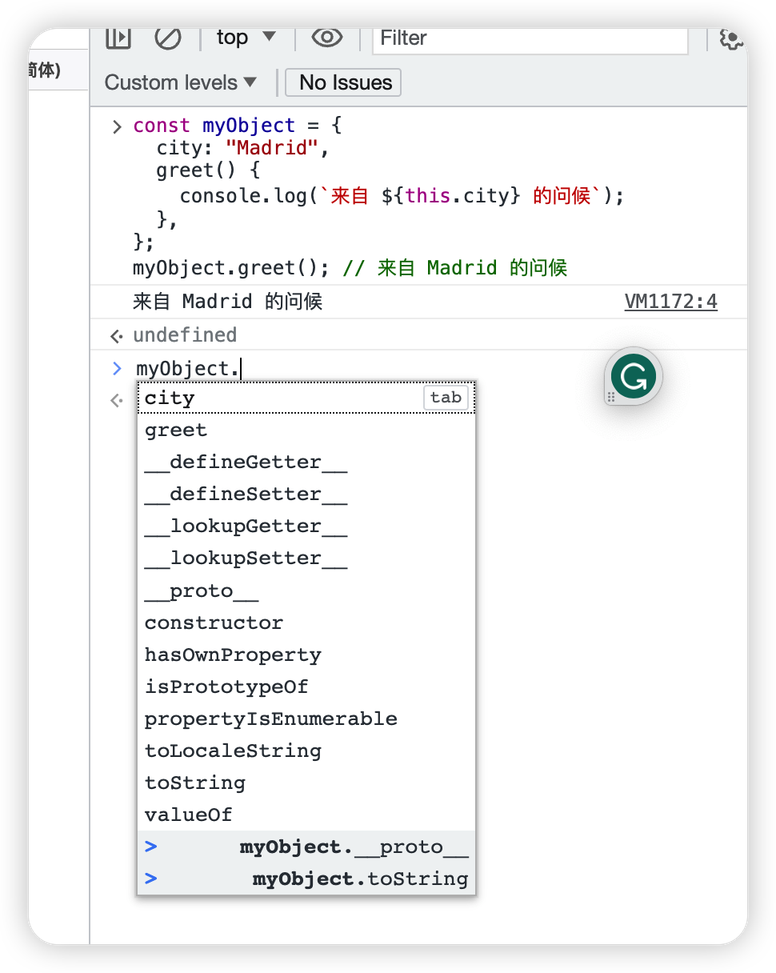
这些额外的属性是什么,它们是从哪里来的?
JavaScript 中所有的对象都有一个内置属性,称为它的 prototype(原型)。
它本身是一个对象,故原型对象也会有它自己的原型,逐渐构成了原型链。原型链终止于拥有 null
作为其原型的对象上。
当你试图访问一个对象的属性时:如果在对象本身中找不到该属性,就会在原型中搜索该属性。如果仍然找不到该属性,那么就搜索原型的原型,以此类推,直到找到该属性,或者到达链的末端,在这种情况下,返回 undefined
。如图:
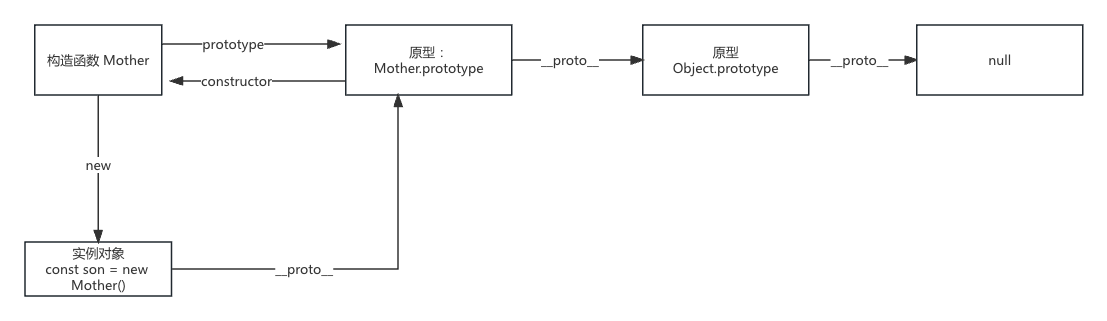
new 关键字的作用
创建一个新对象 son
新对象会被执行[[prototype]]链接,son.__proto__=Mother.prototype
新对象和函数调用 this 绑定 Mothe.call(son,'son')
执行 constructor 方法,如果 constructor 方法没有返回值,那么会自动返回 this
Share 分享一个观点
学习是没有捷径的,是逆人性的,你需要长期地付出时间和精力--陈皓老师
评论