SSM 整合 (功能模块的开发)
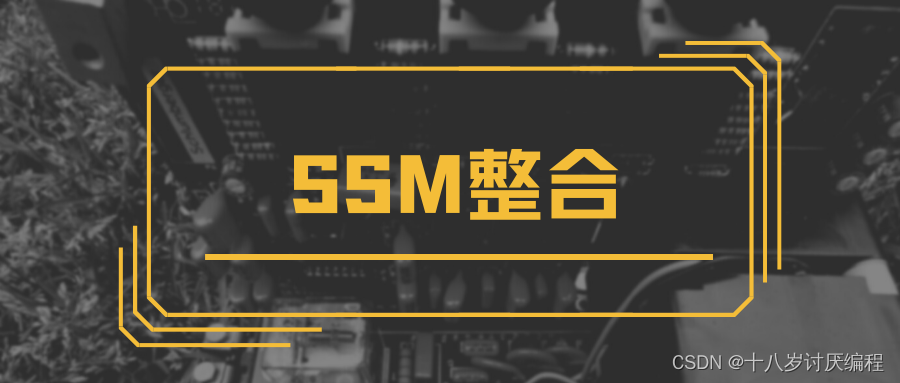
步骤 1:创建数据库及表
最后表长这样:
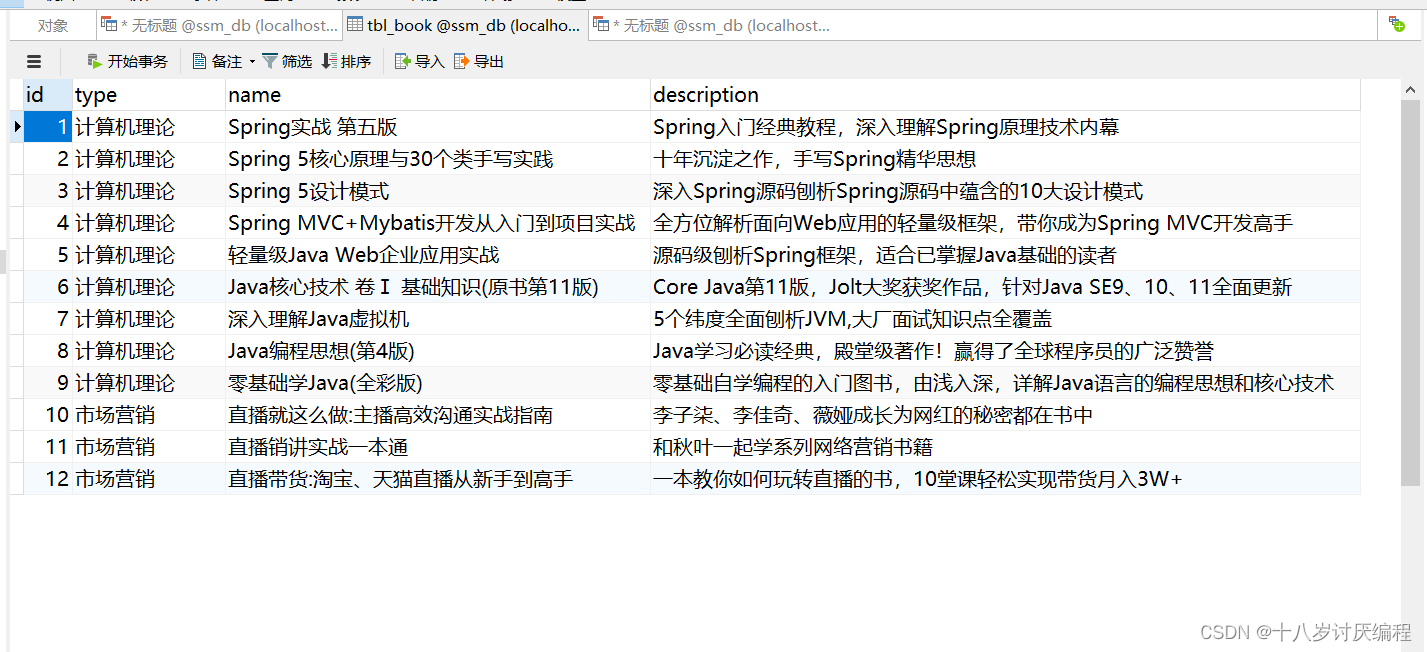
步骤 2:编写模型类
在这一步我们编写 POJO 的封装对象。我们把这个类放到 domain 文件夹中:
步骤 3:编写 Dao 接口
这一步我们实现数据层的接口。然后 MyBatis 的自动代理会帮我们创建实现类!
括号中的 type,name 等和 Book 中的属性对应。
这种写法前面圆括号中的 type,name 等和表里面的字段名对应。后面的括号中的 type,name 等和 Book 中的属性对应。
后面的都可以以此类推。
步骤 4:编写 Service 接口
这一步我们编写业务层的接口。文件写在 Service 文件夹下。
快速写法:
直接把 Dao 接口中定义的方法复制粘贴过来
在这个基础上进行修改
修改返回值的类型(对于增删改操作)
最好加上文档注释,方便其他人调用的时候可查阅
快速写法虽然快速,但是不推荐!因为业务层的接口最好是让别人见名知义,能够一眼看出是执行什么业务。
BookService:
步骤 5:编写 Service 实现类
此实现类放在 Service/Impl 文件夹中
这里就需要用到数据层接口 Dao 了,同时我们要把这个实现类定义为 bean。
BookServiceImpl:
有人看到很多方法我们都是直接 return true,那么什么时候 return false 呢?当我们在抛异常的时候就会 return false,我们会在后期处理这个问题,这个地方不详细赘述。
说明:
bookDao 在 Service 中注入的会提示一个红线提示,为什么呢?
BookDao 是一个接口,没有实现类,接口是不能创建对象的,所以最终注入的应该是代理对象
代理对象是由 Spring 的 IOC 容器来创建管理的
IOC 容器又是在 Web 服务器启动的时候才会创建
IDEA 在检测依赖关系的时候,没有找到适合的类注入,所以会提示错误提示
但是程序运行的时候,代理对象就会被创建,框架会使用 DI 进行注入,所以程序运行无影响。
如何解决上述问题?
可以不用理会,因为运行是正常的
设置错误提示级别
步骤 6:编写 Contorller 类
这里的 Controller 类我们放在 Controller 文件夹下。
这个控制器类我们同样要把它定义为 bean,同时我们要使用 REST 风格,并且将当前控制器返回值作为响应体。所以本来我们要两个注解 @Controller 与 @ResponseBody,优化之后我们直接使用 @RestController
然后我们要配置这个模块的公共映射使用 @RequestMapping(在类上)
Controller 属于表现层,在这里我们要调用业务层的接口,这里我们使用自动装配引入,也就是注解 @Autowired
我们删除和查询需要的 id 参数使用的是路径传递,所以使用注解 @PathVariable,记得括号的名字要与形参的名字相对应
因为 Book 类的参数是从我们提交过来的 json 数据中获取,所以要加上注解 @RequestBody
总结
功能模块[与具体的业务模块有关]
创建数据库表
根据数据库表创建对应的模型类
通过 Dao 层完成数据库表的增删改查(接口+自动代理)
编写 Service 层[Service 接口+实现类]
@Service
@Transactional
整合 Junit 对业务层进行单元测试
@RunWith
@ContextConfiguration
@Test
编写 Controller 层
接收请求 @RequestMapping @GetMapping @PostMapping @PutMapping @DeleteMapping
接收数据 简单、POJO、嵌套 POJO、集合、数组、JSON 数据类型
@RequestParam
@PathVariable
@RequestBody
转发业务层
@Autowired
响应结果
@ResponseBody
版权声明: 本文为 InfoQ 作者【fake smile by】的原创文章。
原文链接:【http://xie.infoq.cn/article/f2e74703cc76947d88e11292a】。未经作者许可,禁止转载。
评论