JavaMail 使用 POP3/SMTP 服务发送 QQ 邮件
- 2022-10-13 江西
本文字数:6176 字
阅读完需:约 1 分钟
一、说明
邮件服务器
为用户提供接收邮件的功能, 有发邮件的 SMTP 服务器,收邮件的 POP3 服务器
电子邮箱
用户在邮件服务器上申请的账户
邮件客户端
通常使用 IMAP/APOP/POP3/SMTP 协议收发电子邮件的软件
邮件传输协议
SMTP 协议(Simple Mail Transfer Protocol)简单邮件传输协议(默认端口 25),邮件客户端和 SMTP 邮件服务器之间通信规则
POP/POP3 协议(Post Office Protocol)邮件接收协议(默认端口 110),把存储在 POP 协议邮件服务器的邮件下载到用户计算机中,但不能对邮件进行在线操作
IMAP 协议(Internet Message Access Protocol)Internet 消息访问协议(默认端口 143),可以通过客户端直接对服务器上的邮件在线操作
邮件发送基本流程
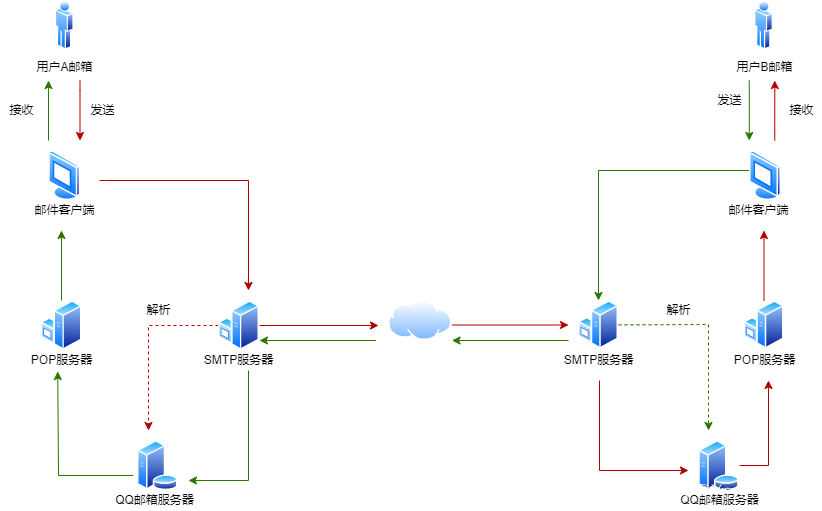
二、理解
Session 类
定义邮件配置信息
Message 类
实例对象表示一份电子邮件
Transport 类
实现邮件发送协议对象
Store 类
实现邮件接受协议对象
三、实现
1.导入 jar 包
使用 Java 应用程序发送 E-mail 需要 JavaMail API 和 Java Activation Framework (JAF)
JavaMail jar 包下载:JavaMail mail.jar 1.4.5Java activation jar 包下载:JavaBeans(TM) Activation Framework
2.用户认证
什么是授权码?
授权码是 QQ 邮箱推出的,用于登录第三方客户端的专用密码
适用于登录以下服务:POP3/IMAP/SMTP/Exchange/CardDAV/CalDAV 服务
怎么获取授权码?
QQ 邮箱需要手动开启 POP3/SMTP 服务,开启后会得到邮箱客户端连接的授权密码,登录其它邮件客户端需要此授权码
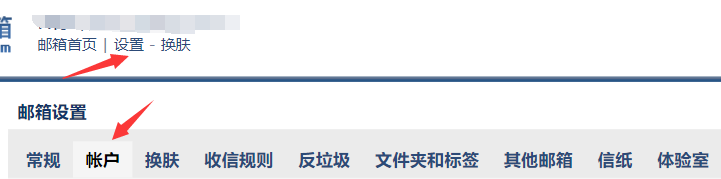
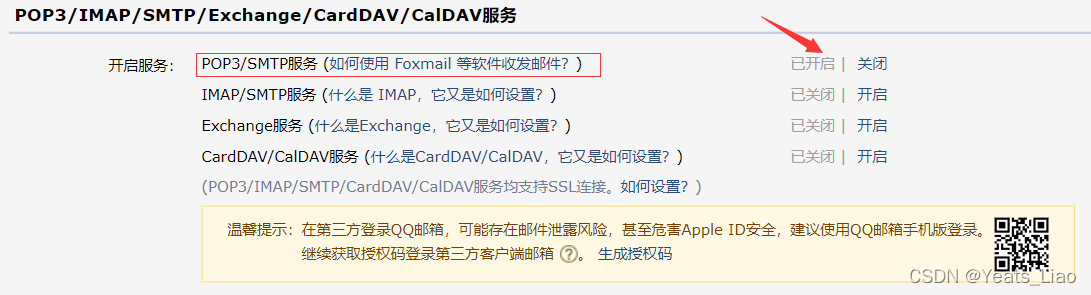
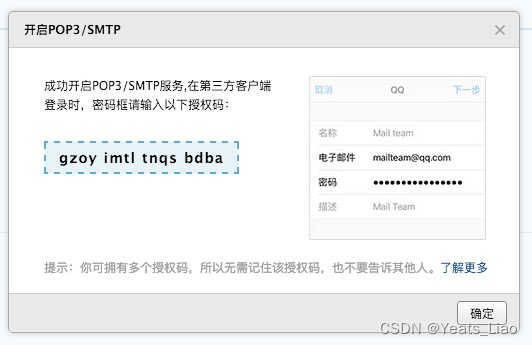
设置用户认证以及 SSL 加密
// 设置用户认证以及SSL加密
properties.put("mail.smtp.auth", "true");
MailSSLSocketFactory sf = new MailSSLSocketFactory();
sf.setTrustAllHosts(true);
properties.put("mail.smtp.ssl.enable", "true");
properties.put("mail.smtp.ssl.socketFactory", sf);
// 获取默认session对象
Session session = Session.getDefaultInstance(properties,new Authenticator(){
public PasswordAuthentication getPasswordAuthentication() {
//设置发件人邮件用户名、授权码
return new PasswordAuthentication("xxxxxxxx@qq.com", "vyvvxhilstaxbffb");
}
});
3.发送邮件
创建步骤
配置连接邮件协议、服务器参数
设置用户认证,获取
session
对象设置发件人,收件人
创建邮件
MimeMessage
对象设置邮件标题,正文,附件等
发送邮件
简单的 Email
创建SendEmail
类,发送一封简单的 Email
import java.security.GeneralSecurityException;
import java.util.Properties;
import javax.mail.Authenticator;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import com.sun.mail.util.MailSSLSocketFactory;
public class SendEmail {
public static void main(String [] args) throws GeneralSecurityException {
// 收件人电子邮箱
String toEmail = "xxxxxxx@qq.com";
// 发件人电子邮箱
String fromEmail = "xxxxxxxxx@qq.com";
// 指定发送邮件的主机为QQ 邮件服务器 smtp.qq.com
String emailHost = "smtp.qq.com";
// 邮件接收的协议
String storeType = "pop3";
// 获取系统属性
Properties properties = System.getProperties();
// 设置邮件服务器
properties.setProperty("mail.smtp.host", emailHost);
// 设置用户认证以及SSL加密
properties.put("mail.smtp.auth", "true");
MailSSLSocketFactory sf = new MailSSLSocketFactory();
sf.setTrustAllHosts(true);
properties.put("mail.smtp.ssl.enable", "true");
properties.put("mail.smtp.ssl.socketFactory", sf);
// 获取默认session对象
Session session = Session.getDefaultInstance(properties,new Authenticator(){
public PasswordAuthentication getPasswordAuthentication() {
//设置发件人邮件用户名、授权码
return new PasswordAuthentication("xxxxxxxx@qq.com", "xxxxxxxxxxxx");
}
});
try{
// 创建默认的 MimeMessage 对象
MimeMessage message = new MimeMessage(session);
// SetFrom 设置发件人
message.setFrom(new InternetAddress(fromEmail));
// SetTo 设置收件人
message.addRecipient(Message.RecipientType.TO, new InternetAddress(toEmail));
// SetSubject 设置标题
message.setSubject("Hello! This is the Subject Line!");
// 设置邮件正文
message.setText("I'm Yeats_Liao");
// 发送邮件
Transport.send(message);
System.out.println("Sent message successfully");
}catch (MessagingException mex) {
mex.printStackTrace();
}
}
}
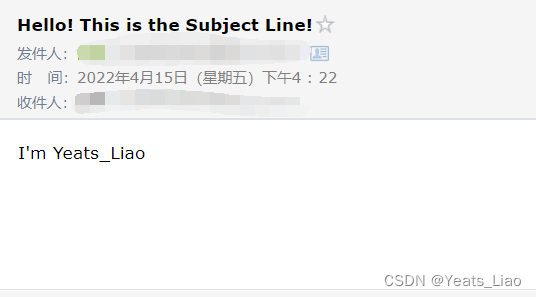
带 HTML 的 E-mail
使用 .setContent()
方法设置 HTML 内容,发送一封 HTML E-mail
message.setContent(
"<h1>You Have a Promotion</h1> " +
"<h3>Your First Name :</h3>" +
"<h3>Your Last Name :</h3>" +
"<h5>Your Employee ID :</h5>" ,
"text/html");
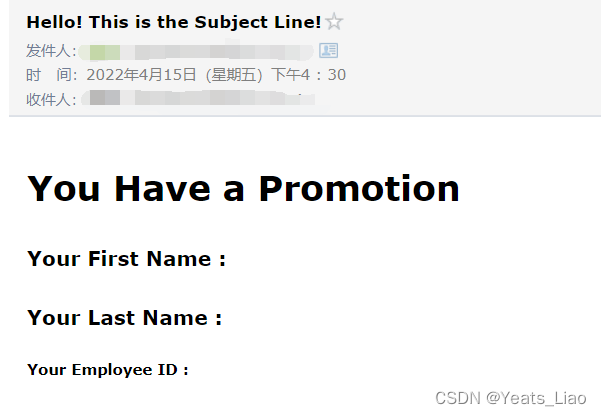
带图片的 Email
DataHandler
类为在多种不同源和格式下可用的数据提供一致的接口文字附带图片
MimeMultipart
的类型必须是related
图片的显示方式
Disposition
必须为INLINE
必须明确指定
Content-Type
头信息(Content-Type,image/png)
package com.bedroom;
import java.security.GeneralSecurityException;
import java.util.Properties;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import com.sun.mail.util.MailSSLSocketFactory;
public class SendEmail {
public static void main(String [] args) throws GeneralSecurityException {
// 收件人电子邮箱
String toEmail = "xxxxxxxxx@qq.com";
// 发件人电子邮箱
String fromEmail = "xxxxxxxxx@qq.com";
// 指定发送邮件的主机为QQ 邮件服务器 smtp.qq.com
String emailHost = "smtp.qq.com";
// 邮件接收的协议
String storeType = "pop3";
// 获取系统属性
Properties properties = System.getProperties();
// 设置邮件服务器
properties.setProperty("mail.smtp.host", emailHost);
// 设置用户认证以及SSL加密
properties.put("mail.smtp.auth", "true");
MailSSLSocketFactory sf = new MailSSLSocketFactory();
sf.setTrustAllHosts(true);
properties.put("mail.smtp.ssl.enable", "true");
properties.put("mail.smtp.ssl.socketFactory", sf);
// 获取默认session对象
Session session = Session.getDefaultInstance(properties,new Authenticator(){
public PasswordAuthentication getPasswordAuthentication() {
//设置发件人邮件用户名、授权码
return new PasswordAuthentication("xxxxxxxxxx@qq.com", "xxxxxxxxxx");
}
});
try{
// 创建默认的 MimeMessage 对象
MimeMessage message = new MimeMessage(session);
// SetFrom 设置发件人
message.setFrom(new InternetAddress(fromEmail));
// SetTo 设置收件人 To收件人 CC抄送 BCC密送
message.addRecipient(Message.RecipientType.TO, new InternetAddress(toEmail));
// SetSubject 设置标题
message.setSubject("Hello! This is the Subject Line!");
// 设置邮件正文,一个Multipart对象包含一个或多个bodypart对象
MimeMultipart multipart = new MimeMultipart();
// 读取本地图片,将图片数据添加到节点
MimeBodyPart image_1 = new MimeBodyPart();
image_1.setDataHandler(new DataHandler(new FileDataSource("D:\\Project\\ideaProject\\Demo2\\file\\1.png")));
image_1.setContentID("image_1");
image_1.setDisposition(MimeBodyPart.INLINE);
image_1.setHeader("Content-Type", "image/png");
//创建文本节点,添加正文数据
MimeBodyPart text_1 = new MimeBodyPart();
text_1.setContent("I'm Yeats_Liao" +
"</br><img src=\"cid:image_1\">",
"text/html;charset=UTF-8");
//将文本和图片添加到multipart,描述数据关系
multipart.addBodyPart(text_1);
multipart.addBodyPart(image_1);
multipart.setSubType("related");
// 将multipart节点,设置到消息中
message.setContent(multipart);
// 保存设置
message.saveChanges();
// 发送邮件
Transport.send(message);
System.out.println("Sent message successfully");
}catch (MessagingException mex) {
mex.printStackTrace();
}
}
}
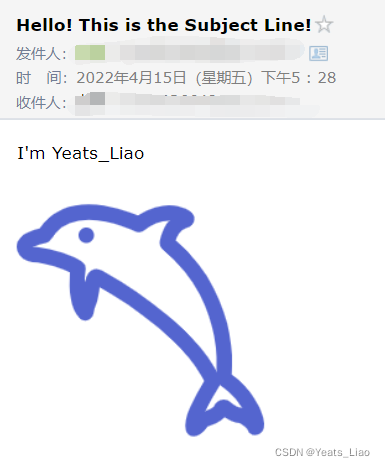
包含附件的邮件
package com.bedroom;
import java.io.UnsupportedEncodingException;
import java.security.GeneralSecurityException;
import java.util.Properties;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.mail.*;
import javax.mail.internet.*;
import com.sun.mail.util.MailSSLSocketFactory;
public class SendEmail {
public static void main(String [] args) throws GeneralSecurityException {
// 收件人电子邮箱
String toEmail = "xxxxxxxx@qq.com";
// 发件人电子邮箱
String fromEmail = "xxxxxxx@qq.com";
// 指定发送邮件的主机为QQ 邮件服务器 smtp.qq.com
String emailHost = "smtp.qq.com";
// 邮件接收的协议
String storeType = "pop3";
// 获取系统属性
Properties properties = System.getProperties();
// 设置邮件服务器
properties.setProperty("mail.smtp.host", emailHost);
// 设置用户认证以及SSL加密
properties.put("mail.smtp.auth", "true");
MailSSLSocketFactory sf = new MailSSLSocketFactory();
sf.setTrustAllHosts(true);
properties.put("mail.smtp.ssl.enable", "true");
properties.put("mail.smtp.ssl.socketFactory", sf);
// 获取默认session对象
Session session = Session.getDefaultInstance(properties,new Authenticator(){
public PasswordAuthentication getPasswordAuthentication() {
//设置发件人邮件用户名、授权码
return new PasswordAuthentication("xxxxxxxx@qq.com", "xxxxxxxx");
}
});
try{
// 创建默认的 MimeMessage 对象
MimeMessage message = new MimeMessage(session);
// SetFrom 设置发件人
message.setFrom(new InternetAddress(fromEmail));
// SetTo 设置收件人 To收件人 CC抄送 BCC密送
message.addRecipient(Message.RecipientType.TO, new InternetAddress(toEmail));
// SetSubject 设置标题
message.setSubject("Hello! This is the Subject Line!");
// 设置邮件正文,一个Multipart对象包含一个或多个bodypart对象
MimeMultipart multipart = new MimeMultipart();
// 读取本地图片,将图片数据添加到节点
MimeBodyPart image_1 = new MimeBodyPart();
image_1.setDataHandler(new DataHandler(new FileDataSource("D:\\Project\\ideaProject\\Demo2\\file\\1.png")));
image_1.setContentID("image_1");
image_1.setDisposition(MimeBodyPart.INLINE);
image_1.setHeader("Content-Type", "image/png");
//创建文本节点,添加正文数据
MimeBodyPart text_1 = new MimeBodyPart();
text_1.setContent("I'm Yeats_Liao" +
"</br><img src=\"cid:image_1\">",
"text/html;charset=UTF-8");
//创建附件节点,读取本地文件,并读取附件名称
MimeBodyPart file1 = new MimeBodyPart();
DataHandler dataHandler2 = new DataHandler(new FileDataSource("D:\\Project\\ideaProject\\Demo2\\file\\1.txt"));
file1.setDataHandler(dataHandler2);
file1.setFileName(MimeUtility.encodeText(dataHandler2.getName()));
//将文本和图片添加到multipart,描述数据关系
multipart.addBodyPart(text_1);
multipart.addBodyPart(image_1);
multipart.addBodyPart(file1);
multipart.setSubType("mixed");
// 将multipart节点,设置到消息中
message.setContent(multipart);
// 保存设置
message.saveChanges();
// 发送邮件
Transport.send(message);
System.out.println("Sent message successfully");
}catch (MessagingException | UnsupportedEncodingException mex) {
mex.printStackTrace();
}
}
}
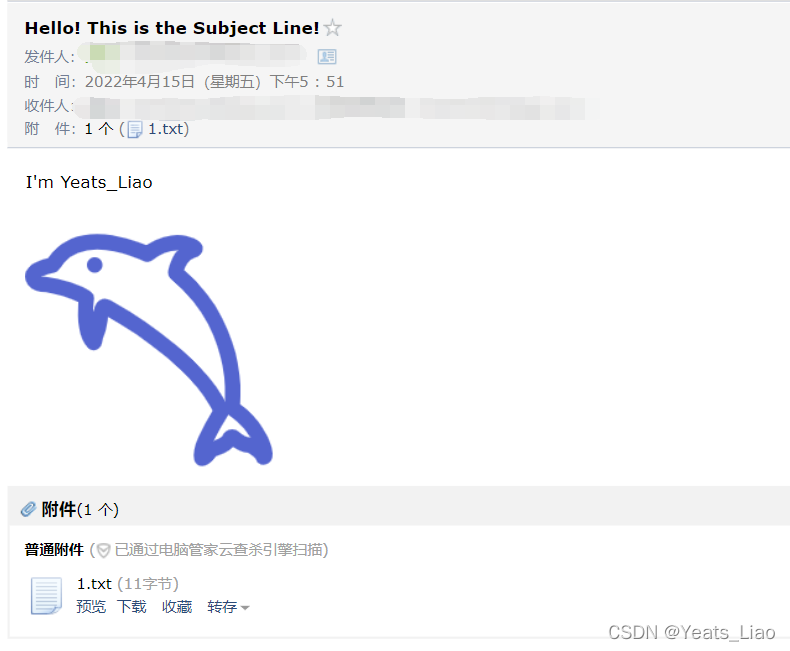
如果还需添加收件人或抄送人,.addRecipient()
方法即可,To 收件人 CC 抄送 BCC 密送
message.addRecipient(MimeMessage.RecipientType.TO,new InternetAddress("xxxxxxx@qq.com","personal","UTF-8"));
版权声明: 本文为 InfoQ 作者【Yeats_Liao】的原创文章。
原文链接:【http://xie.infoq.cn/article/eeb76f79911a835d011cf3f67】。文章转载请联系作者。
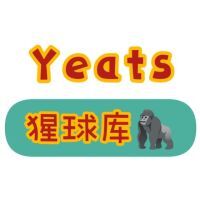
Yeats_Liao
Hello,World! 2022-10-02 加入
这里更多的是记录个人学习,如果有侵权内容请联系我! 个人邮箱是:yeats_liao@foxmail.com
评论