2024-01-17:lc 的 30. 串联所有单词的子串
2024-01-17:用 go 语言,给定一个字符串 s 和一个字符串数组 words。 words 中所有字符串 长度相同。
s 中的 串联子串 是指一个包含 words 中所有字符串以任意顺序排列连接起来的子串。
例如,如果 words = ["ab","cd","ef"],
那么 "abcdef", "abefcd","cdabef",
"cdefab","efabcd", 和 "efcdab" 都是串联子串,
"acdbef" 不是串联子串,因为他不是任何 words 排列的连接。
返回所有串联字串在 s 中的开始索引。
你可以以 任意顺序 返回答案。
1 <= s.length <= 10^4,
1 <= words.length <= 5000,
1 <= words[i].length <= 30。
words[i] 和 s 由小写英文字母组成。
输入:s = "barfoothefoobarman", words = ["foo","bar"]。
输出:[0,9]。
来自 lc 的 30. 串联所有单词的子串。
答案 2024-01-17:
来自左程云。
大体过程如下:
定义一些常量和变量,包括
BASE
和MAXN
,以及存储结果的切片ans
。实现
hashValue
函数,用于计算字符串的哈希值。这里使用一个基于索引的简单哈希函数将字符串映射为一个唯一的整数。实现
buildHash
函数,用于构建字符串的前缀哈希数组。通过动态规划的方式计算每个位置的哈希值。实现
hashValueRange
函数,用于计算子串的哈希值。利用前缀哈希数组,根据子串的起始和结束位置计算哈希值。创建一个哈希表
mapCount
用于存储words
中每个单词的出现次数。构建字符串
s
的前缀哈希数组hash
。创建一个数组
pow
,用于存储 BASE 的幂次方,便于后续计算子串的哈希值。创建一个滑动窗口
window
,用于记录当前窗口中每个单词出现的次数。循环遍历
s
中每个起始位置的可能性(即从 0 到wordLen
-1)。在每个起始位置,初始化一个变量
debt
用于记录还需要凑齐的单词数。在每个起始位置,遍历
words
中的单词,依次将其添加到窗口中,并更新debt
的值。如果
debt
等于 0,表示窗口中已经包含了所有words
中的单词,则将当前起始位置加入结果数组ans
中。对于每个起始位置,向右移动窗口,同时更新窗口中单词的出现次数。
检查窗口中的哈希值和单词出现次数是否符合要求,如果符合则将当前起始位置加入结果数组
ans
中。清空滑动窗口
window
。返回结果数组
ans
。
总的时间复杂度:O(n * m * k),其中 n 是字符串 s
的长度,m 是 words
的长度,k 是单词的平均长度。
总的额外空间复杂度:O(n),其中 n 是字符串 s
的长度,主要用于存储哈希表、前缀哈希数组和结果数组。
go 完整代码如下:
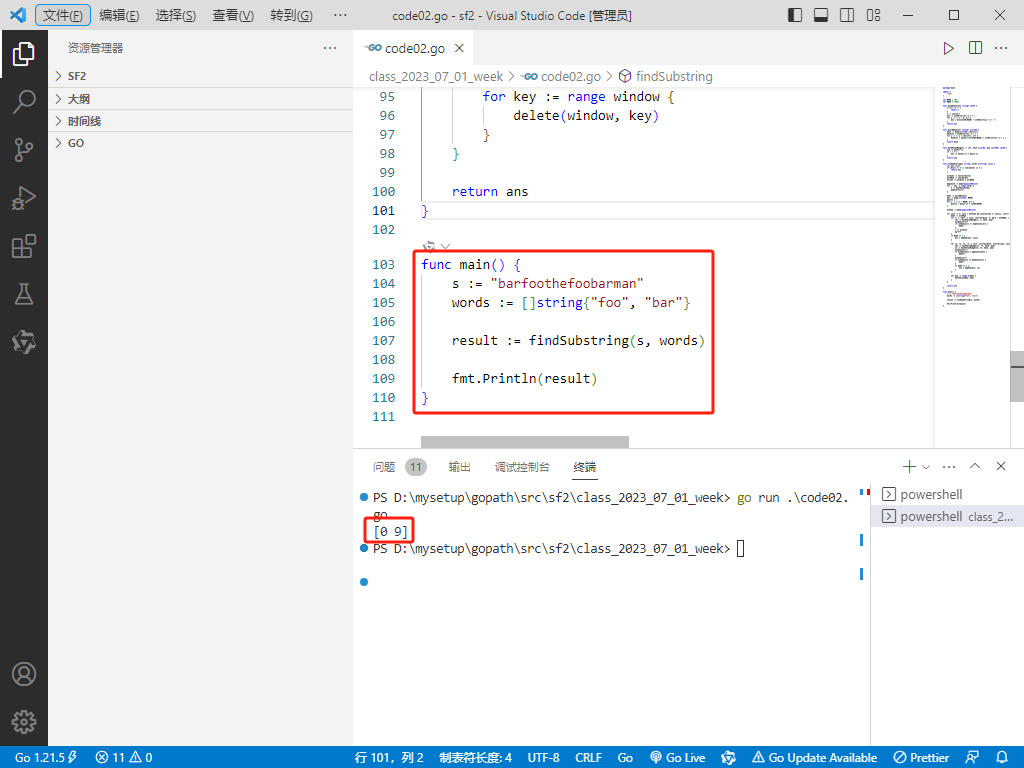
版权声明: 本文为 InfoQ 作者【福大大架构师每日一题】的原创文章。
原文链接:【http://xie.infoq.cn/article/e7c5af948f6c76d7e5d35eca5】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
评论