代码随想录 Day52 - 动态规划(十三)
作者:jjn0703
- 2023-08-25 江苏
本文字数:1743 字
阅读完需:约 6 分钟
作业题
300. 最长递增子序列
链接:https://leetcode.cn/problems/longest-increasing-subsequence/description/
package jjn.carl.dp;
import java.util.Scanner;
/**
* @author Jjn
* @since 2023/8/25 20:04
*/
public class LeetCode300 {
public int lengthOfLIS(int[] nums) {
int length = nums.length;
if (length == 0) {
return 0;
}
int[] dp = new int[length];
int result = 1;
dp[0] = 1;
for (int i = 1; i < length; i++) {
dp[i] = 1;
for (int j = 0; j < i; j++) {
if(nums[j] < nums[i]) {
dp[i] = Math.max(dp[i], dp[j] + 1);
}
}
result = Math.max(result, dp[i]);
}
return result;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int total = scanner.nextInt();
int[] nums = new int[total];
for (int i = 0; i < total; i++) {
nums[i] = scanner.nextInt();
}
int length = new LeetCode300().lengthOfLIS(nums);
System.out.println(length);
}
}
复制代码
674. 最长连续递增序列
链接:https://leetcode.cn/problems/longest-continuous-increasing-subsequence/description/
package jjn.carl.dp;
import java.util.Arrays;
import java.util.Scanner;
/**
* @author Jjn
* @since 2023/8/25 21:27
*/
public class LeetCode674 {
public int findLengthOfLCIS(int[] nums) {
if (nums.length == 0) {
return 0;
}
int[] dp = new int[nums.length];
int result = 1;
Arrays.fill(dp, 1);
for (int i = 1; i < nums.length; i++) {
if (nums[i] > nums[i - 1]) {
dp[i] = dp[i - 1] + 1;
}
result = Math.max(dp[i], result);
}
return result;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int total = scanner.nextInt();
int[] nums = new int[total];
for (int i = 0; i < total; i++) {
nums[i] = scanner.nextInt();
}
int length = new LeetCode674().findLengthOfLCIS(nums);
System.out.println(length);
}
}
复制代码
718. 最长重复子数组
链接:https://leetcode.cn/problems/maximum-length-of-repeated-subarray/description/
package jjn.carl.dp;
import java.util.Scanner;
/**
* @author Jjn
* @since 2023/8/25 23:31
*/
public class LeetCode718 {
public int findLength(int[] nums1, int[] nums2) {
int length1 = nums1.length;
int length2 = nums2.length;
int result = 0;
int[][] dp = new int[length1 + 1][length2 + 1];
for (int i = 1; i <= length1; i++) {
for (int j = 1; j <= length2; j++) {
if (nums1[i - 1] == nums2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1] + 1;
result = Math.max(result, dp[i][j]);
}
}
}
return result;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int m = scanner.nextInt();
int n = scanner.nextInt();
int[] nums1 = new int[m];
int[] nums2 = new int[n];
for (int i = 0; i < m; i++) {
nums1[i] = scanner.nextInt();
}
for (int i = 0; i < n; i++) {
nums2[i] = scanner.nextInt();
}
int length = new LeetCode718().findLength(nums1, nums2);
System.out.println(length);
}
}
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 3
版权声明: 本文为 InfoQ 作者【jjn0703】的原创文章。
原文链接:【http://xie.infoq.cn/article/ddc9011c4036b17a06c10f9d7】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
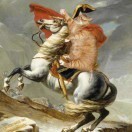
jjn0703
关注
Java工程师/终身学习者 2018-03-26 加入
USTC硕士/健身健美爱好者/Java工程师.
评论