SpringBoot:四种读取 properties 文件的方式
- 2022-11-30 陕西
本文字数:4877 字
阅读完需:约 16 分钟
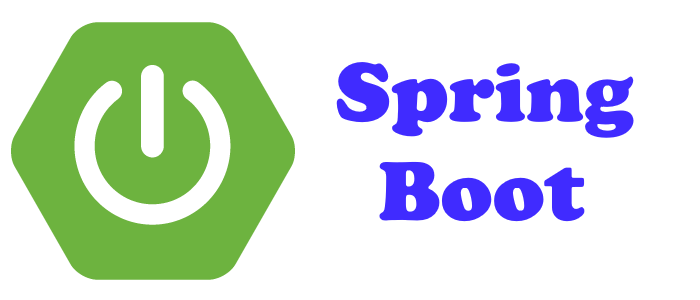
前言
在项目开发中经常会用到配置文件,配置文件的存在解决了很大一份重复的工作。今天就分享四种在 Springboot 中获取配置文件的方式。
注:前三种测试配置文件为 springboot 默认的application.properties
文件
#######################方式一#########################
com.battle.type3=Springboot - @ConfigurationProperties
com.battle.title3=使用@ConfigurationProperties获取配置文件
#map
com.battle.login[username]=admin
com.battle.login[password]=123456
com.battle.login[callback]=http://www.flyat.cc
#list
com.battle.urls[0]=http://ztool.cc
com.battle.urls[1]=http://ztool.cc/format/js
com.battle.urls[2]=http://ztool.cc/str2image
com.battle.urls[3]=http://ztool.cc/json2Entity
com.battle.urls[4]=http://ztool.cc/ua
#######################方式二#########################
com.battle.type=Springboot - @Value
com.battle.title=使用@Value获取配置文件
#######################方式三#########################
com.battle.type2=Springboot - Environment
com.battle.title2=使用Environment获取配置文件
一、@ConfigurationProperties 方式
自定义配置类:PropertiesConfig.java
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.boot.context.properties.ConfigurationProperties;
//import org.springframework.context.annotation.PropertySource;
import org.springframework.stereotype.Component;
/**
* 对应上方配置文件中的第一段配置
* @author battle
* @date 2017年6月1日 下午4:34:18
* @version V1.0
* @since JDK : 1.7 */
@Component
@ConfigurationProperties(prefix = "com.zyd")
// PropertySource默认取application.properties
// @PropertySource(value = "config.properties")
public class PropertiesConfig {
public String type3; public String title3;
public Map<String, String> login = new HashMap<String, String>();
public List<String> urls = new ArrayList<>();
public String getType3() {
return type3;
}
public void setType3(String type3) {
this.type3 = type3;
}
public String getTitle3() {
try {
return new String(title3.getBytes("ISO-8859-1"), "UTF-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
return title3;
}
public void setTitle3(String title3) {
this.title3 = title3;
}
public Map<String, String> getLogin() { return login; }
public void setLogin(Map<String, String> login) { this.login = login; }
public List<String> getUrls() { return urls; }
public void setUrls(List<String> urls) { this.urls = urls; } }
程序启动类:Applaction.java
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.zyd.property.config.PropertiesConfig;
@SpringBootApplication
@RestController
public class Applaction {
@Autowired private PropertiesConfig propertiesConfig;
/**
* 第一种方式:使用`@ConfigurationProperties`注解将配置文件属性注入到配置对象类中
* @throws UnsupportedEncodingException
* @since JDK 1.7 */
@RequestMapping( "/config" ) public Map<String, Object> configurationProperties()
{
Map<String, Object> map = new HashMap<String, Object>();
map.put( "type", propertiesConfig.getType3() );
map.put( "title", propertiesConfig.getTitle3() );
map.put( "login", propertiesConfig.getLogin() );
map.put( "urls", propertiesConfig.getUrls() );
return(map);
}
public static void main( String[] args ) throws Exception
{
SpringApplication application = new SpringApplication( Applaction.class );
application.run( args );
}
}
访问结果:
{"title":"使用 @ConfigurationProperties 获取配置文件",
"urls":["http://ztool.cc","http://ztool.cc/format/js","http://ztool.cc/str2image",
"http://ztool.cc/json2Entity","http://ztool.cc/ua"],
"login":{"username":"admin",
"callback":"http://www.flyat.cc","password":"123456"},
"type":"Springboot - @ConfigurationProperties"}
二、使用 @Value 注解方式
程序启动类:Applaction.java
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Map;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class Applaction {
@Value("${com.zyd.type}") private String type;
@Value("${com.zyd.title}") private String title;
/** * * 第二种方式:使用`@Value("${propertyName}")`注解 *
* @throws UnsupportedEncodingException * @since JDK 1.7 */
@RequestMapping("/value") public Map<String, Object> value() throws UnsupportedEncodingException {
Map<String, Object> map = new HashMap<String, Object>();
map.put("type", type);
// *.properties文件中的中文默认以ISO-8859-1方式编码,因此需要对中文内容进行重新编码
map.put("title", new String(title.getBytes("ISO-8859-1"), "UTF-8"));
return map;
}
public static void main(String[] args) throws Exception {
SpringApplication application = new SpringApplication(Applaction.class);
application.run(args);
} }
访问结果:
{"title":"使用 @Value 获取配置文件","type":"Springboot - @Value"}
三、使用 Environment 程序启动类:Applaction.java
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.core.env.Environment;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class Applaction {
@Autowired private Environment env;
/** * * 第三种方式:使用`Environment` * * @author zyd * @throws UnsupportedEncodingException * @since JDK 1.7 */
@RequestMapping("/env") public Map<String, Object> env() throws UnsupportedEncodingException {
Map<String, Object> map = new HashMap<String, Object>();
map.put("type", env.getProperty("com.zyd.type2"));
map.put("title", new String(env.getProperty("com.zyd.title2").getBytes("ISO-8859-1"), "UTF-8"));
return map;
}
public static void main(String[] args) throws Exception {
SpringApplication application = new SpringApplication(Applaction.class);
application.run(args);
}
}
访问结果:{"title":"使用 Environment 获取配置文件","type":"Springboot - Environment"}四、使用 PropertiesLoaderUtilsapp-config.properties
#### 通过注册监听器(`Listeners`) + `PropertiesLoaderUtils`的方式
com.battle.type=Springboot - Listeners
com.battle.title=使用Listeners + PropertiesLoaderUtils获取配置文件
com.battle.name=zyd
com.battle.address=Beijing
com.battle.company=in
PropertiesListener.java 用来初始化加载配置文件
import org.springframework.boot.context.event.ApplicationStartedEvent;
import org.springframework.context.ApplicationListener;
import com.zyd.property.config.PropertiesListenerConfig;
public class PropertiesListener implements ApplicationListener<ApplicationStartedEvent> {
private String propertyFileName;
public PropertiesListener(String propertyFileName) {
this.propertyFileName = propertyFileName;
}
@Override public void onApplicationEvent(ApplicationStartedEvent event) {
PropertiesListenerConfig.loadAllProperties(propertyFileName);
}
}
PropertiesListenerConfig.java 加载配置文件内容
import org.springframework.boot.context.event.ApplicationStartedEvent;
import org.springframework.context.ApplicationListener;
import com.zyd.property.config.PropertiesListenerConfig;
public class PropertiesListener implements ApplicationListener<ApplicationStartedEvent> {
private String propertyFileName;
public PropertiesListener(String propertyFileName) {
this.propertyFileName = propertyFileName;
}
@Override public void onApplicationEvent(ApplicationStartedEvent event) {
PropertiesListenerConfig.loadAllProperties(propertyFileName);
}
}
Applaction.java 启动类
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Map;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.zyd.property.config.PropertiesListenerConfig;
import com.zyd.property.listener.PropertiesListener;
@SpringBootApplication @RestController public class Applaction {
/** * * 第四种方式:通过注册监听器(`Listeners`) + `PropertiesLoaderUtils`的方式 * * @author zyd * @throws UnsupportedEncodingException * @since JDK 1.7 */
@RequestMapping("/listener") public Map<String, Object> listener() {
Map<String, Object> map = new HashMap<String, Object>();
map.putAll(PropertiesListenerConfig.getAllProperty());
return map;
}
public static void main(String[] args) throws Exception {
SpringApplication application = new SpringApplication(Applaction.class);
// 第四种方式:注册监听器 application.addListeners(new PropertiesListener("app-config.properties")); application.run(args); } }
访问结果:
{"com.battle.name":"zyd",
"com.battle.address":"Beijing",
"com.battle.title":"使用 Listeners + PropertiesLoaderUtils 获取配置文件",
"com.battle.type":"Springboot - Listeners",
"com.battle.company":"in"}
版权声明: 本文为 InfoQ 作者【@下一站】的原创文章。
原文链接:【http://xie.infoq.cn/article/c20af9e6a564360c2a6cf267e】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
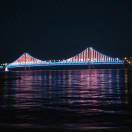
@下一站
懒人 2020-11-22 加入
都是黄泉预约客,何必难为每一天,执念太强,无法豁然。
评论