鸿蒙应用示例:购物车侧滑删除、侧滑收藏、计算价格
作者:zhongcx
- 2024-10-11 广东
本文字数:3203 字
阅读完需:约 11 分钟
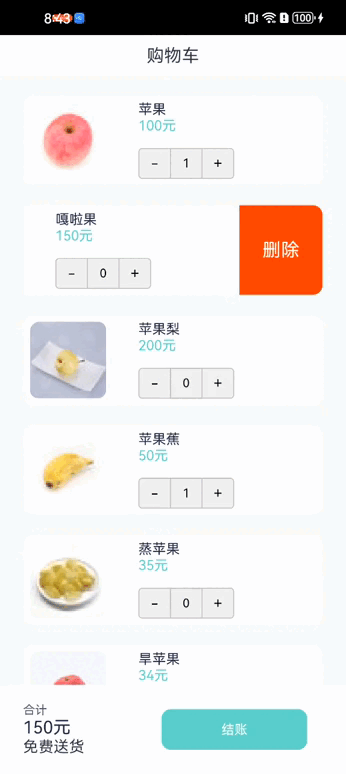
在鸿蒙应用开发中,实现购物车功能并进行屏幕适配是一个常见的需求。通过侧滑删除、侧滑收藏和价格计算等功能,可以为用户提供便捷的购物体验。下面将介绍一个购物车示例的实现方法,并结合屏幕适配技术进行详细说明。
示例代码解析
以上代码实现了一个购物车功能的示例,包括商品展示、侧滑收藏、侧滑删除和价格计算等功能。通过定义 BeanItem 类和使用 List 和 ListItem 组件展示商品信息,同时通过 swipeAction 实现了侧滑收藏和侧滑删除的功能。在价格计算部分,通过遍历商品列表并计算总价,实现了价格的动态更新。
屏幕适配技术应用
在示例中,使用了 lpx 单位来设置字体大小、宽高等属性,以实现屏幕适配。通过合理设置单位和布局,可以在不同设备上实现一致的视觉效果和用户体验。同时,针对不同设备类型的展示效果,可以通过响应式布局和自适应布局进行调整,以适配不同屏幕尺寸和分辨率的设备。
结语
通过以上示例代码和屏幕适配技术的应用,可以为开发者提供一个实践的范例,帮助他们更好地理解和掌握鸿蒙应用的开发技巧。购物车功能作为一个常见的应用场景,结合屏幕适配技术的应用,可以为用户提供更加友好和一致的购物体验。
通过合理使用不同单位和布局技术,可以有效地解决鸿蒙应用在不同设备上的屏幕适配问题,提供一致且优秀的用户体验。希望这篇文章能够帮助开发者更好地理解和掌握鸿蒙应用的屏幕适配技巧。
以上是关于鸿蒙应用示例的相关介绍,希望对开发者在实际开发中有所帮助。
import { promptAction } from '@kit.ArkUI'
class BeanItem {
name: string = ""
price: number = 0
count: number = 0
img: string = ''
constructor(name: string, price: number, count: number, img: string) {
this.name = name
this.price = price
this.count = count
this.img = img
}
}
@Entry
@Component
struct test {
@State dataArr: Array<BeanItem> = [
new BeanItem('苹果', 100, 0,
'https://xxx.jpg'),
new BeanItem('嘎啦果', 150, 0,
'https://xxx.jpg'),
new BeanItem('苹果梨', 200, 0, 'https://xxx.jpg'),
new BeanItem('苹果蕉', 50, 0,
'https://xxx.jpg'),
new BeanItem('伏苹果', 48, 0, 'https://xxx.jpg'),
new BeanItem('蒸苹果', 35, 0,
'https://xxx.jpg'),
new BeanItem('旱苹果', 34, 0, 'https://xxx.jpg'),
new BeanItem('煮苹果', 70, 0, 'https://xxx.jpg'),
new BeanItem('苹果酥', 157, 0, 'https://xxx.jpg'),
new BeanItem('苹果糊', 53, 0,
'https://xxx.jpg')
]
@State priceCount: number = 0;
getPriceCount() {
let count = 0;
for (let i = 0; i < this.dataArr.length; i++) {
count += this.dataArr[i].count * this.dataArr[i].price
}
this.priceCount = count
}
@Builder
itemStart(index: number) {
Row() {
Text('收藏').fontColor("#ffffff").fontSize('40lpx')
.textAlign(TextAlign.Center)
.width('180lpx')
}
.height('100%')
.backgroundColor("#FFC107")
.justifyContent(FlexAlign.SpaceEvenly)
.borderRadius({ topLeft: 10, bottomLeft: 10 })
.onClick(() => {
promptAction.showToast({
message: '【' + this.dataArr[index].name + '】收藏成功',
duration: 2000,
bottom: '400lpx'
});
})
}
@Builder
itemEnd(index: number) {
Row() {
Text('删除').fontColor("#ffffff").fontSize('40lpx')
.textAlign(TextAlign.Center)
.width('180lpx')
}
.height('100%')
.backgroundColor("#FF3D00")
.justifyContent(FlexAlign.SpaceEvenly)
.borderRadius({ topRight: 10, bottomRight: 10 })
.onClick(() => {
promptAction.showToast({
message: '【' + this.dataArr[index].name + '】已删除',
duration: 2000,
bottom: '400lpx'
});
this.dataArr.splice(index, 1)
this.getPriceCount();
})
}
build() {
Column() {
Text('购物车')
.width('100%')
.height('88lpx')
.fontSize('38lpx')
.backgroundColor("#ffffff")
.textAlign(TextAlign.Center)
List({ space: '44lpx' }) {
ForEach(this.dataArr, (item: BeanItem, index: number) => {
ListItem() {
Row() {
Image(item.img)
.width('193lpx')
.height('193lpx')
.alt(item.img)
.borderRadius(10)
.padding('14lpx')
Column() {
Text(item.name)
.fontSize('30lpx')
.fontColor("#222B45")
Text(item.price.toString() + '元')
.fontSize("30lpx")
.fontColor("#65DACC")
Blank()
Counter() {
Text(item.count.toString())
.fontColor("#000000")
.fontSize('26lpx')
}.backgroundColor("#0F000000")
.onInc(() => {
item.count++
this.dataArr[index] = new BeanItem(item.name, item.price, item.count, item.img)
this.getPriceCount()
})
.onDec(() => {
if (item.count == 0) {
return;
}
item.count--
this.dataArr[index] = new BeanItem(item.name, item.price, item.count, item.img)
this.getPriceCount()
})
}.margin({ left: '56lpx' })
.height('167lpx')
.alignItems(HorizontalAlign.Start)
}.backgroundColor("#ffffff")
.borderRadius(10)
.width('100%')
}.width('100%').margin({ top: index == 0 ? 20 : 0, bottom: index == this.dataArr.length - 1 ? 20 : 0 })
.swipeAction({ start: this.itemStart(index), end: this.itemEnd(index) })
})
}
.width('648lpx')
.layoutWeight(1)
Row() {
Column() {
Text('合计').fontSize('26lpx').fontColor("#515C6F")
Text(this.priceCount + '元').fontSize('38lpx').fontColor("#222B45")
Text('免费送货')
}.margin({ left: '50lpx' })
.justifyContent(FlexAlign.Start)
.alignItems(HorizontalAlign.Start)
.width('300lpx')
Row() {
Text('结账').fontColor("#FFFFFF").fontSize('28lpx')
}
.onClick(() => {
promptAction.showToast({
message: '结算成功',
duration: 2000,
bottom: '400lpx'
});
})
.width('316lpx')
.height('88lpx')
.backgroundColor("#65DACC")
.borderRadius(10)
.justifyContent(FlexAlign.Center)
}.width('100%').height('192lpx').backgroundColor("#ffffff")
}
.backgroundColor("#F8FAFB")
.width('100%')
.height('100%')
}
}
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 4
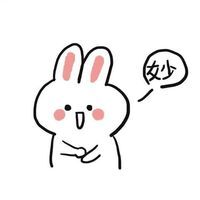
zhongcx
关注
还未添加个人签名 2024-09-27 加入
还未添加个人简介
评论