MyBatis 之 Annotation
作者:andy
- 2022-10-27 北京
本文字数:1815 字
阅读完需:约 6 分钟
一、使用 Annotation 实现 CRUD
MyBatis 提供了 Annotation 注解的方式实现固定 SQL 的 CRUD 操作。但是需要注意的是,Java 程序使用 Annotation 有着许多的局限,注解无法实现动态 SQL 功能。
由此,可以编写 CRUD 接口,如下:
package org.fuys.owndb.dao;
import java.util.List;
import org.apache.ibatis.annotations.Delete;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import org.apache.ibatis.annotations.SelectKey;
import org.apache.ibatis.annotations.Update;
import org.fuys.owndb.vo.Goal;
public interface IGoalDao {
@Insert("INSERT INTO goal(name,description,start_time,end_time) VALUES(#{name},#{description},#{startTime},#{endTime})")
@SelectKey(before = false, keyProperty = "goalId", resultType = java.lang.String.class, statement = "select last_insert_id()")
public int insert(Goal goal) throws Exception;
@Update("UPDATE goal SET name=#{name},description=#{description},start_time=#{startTime},end_time=#{endTime} WHERE goalId = #{goalId}")
public int update(Goal goal) throws Exception;
@Delete("DELETE FROM goal WHERE goalId = #{goalId}")
public int delete(int goalId) throws Exception;
@Select("SELECT goalId,name,description,start_time AS startTime,end_time AS endTime FROM goal WHERE goalId = #{goalId}")
public Goal selectOne(int goalId) throws Exception;
@Select("SELECT goalId,name,description,start_time AS startTime,end_time AS endTime FROM goal WHERE name like concat(concat('%',#{name}),'%') and description like concat(concat('%',#{description}),'%')")
public List<Goal> selectList(Goal goal) throws Exception;
@Select("SELECT goalId,name,description,start_time AS startTime,end_time AS endTime FROM goal WHERE ${column} like concat(concat('%',#{keyword}),'%') LIMIT #{start},#{linesize}")
public List<Goal> selectSplit(@Param("start") int start, @Param("linesize") int linesize, @Param("column") String column,
@Param("keyword") String keyword) throws Exception;
@Select("SELECT count(goalId) FROM goal WHERE ${column} like concat(concat('%',#{keyword}),'%') ")
public int selectCount(@Param("column") String column, @Param("keyword") String keyword) throws Exception;
}
复制代码
但是编写了接口,还不够,还需要通过 SqlSessionFactory 获取 org.apache.ibatis.session.Configuration 对象,再由 Configuration 对编写的接口添加注册。流程如下:
SqlSessionFactory --> getConfiguration() --> Configuration --> addMapper()注册
测试部分代码示例如下:
@Test
public void testInsert() throws Exception {
// add mapper
MyBatisSqlSessionFactory.getSqlSessionFactory().getConfiguration().addMapper(IGoalDao.class);
IGoalDao igoalDao = MyBatisSqlSessionFactory.getSqlSession().getMapper(IGoalDao.class);
Goal goal = new Goal();
goal.setName("JJ - " + new Random().nextInt(99));
goal.setDescription("To be singer");
goal.setStartTime(new Date());
goal.setEndTime(new Date());
int len = igoalDao.insert(goal);
MyBatisSqlSessionFactory.getSqlSession().commit();
MyBatisSqlSessionFactory.closeSqlSession();
TestCase.assertEquals(len, 1);
logger.info(String.valueOf(len));
logger.info(goal);
}
@Test
public void testSelectSplit() throws Exception {
// add mapper
MyBatisSqlSessionFactory.getSqlSessionFactory().getConfiguration().addMapper(IGoalDao.class);
IGoalDao igoalDao = MyBatisSqlSessionFactory.getSqlSession().getMapper(IGoalDao.class);
List<Goal> goalList = igoalDao.selectSplit(1, 3, "name", "j");
MyBatisSqlSessionFactory.closeSqlSession();
TestCase.assertNotNull(goalList);
logger.info(goalList);
}
复制代码
综上所述,接口的子类可以通过注解来实现,但是,因为注解的方式无法实现动态 SQL,建议最好使用配置文件的方式进行开发。
划线
评论
复制
发布于: 刚刚阅读数: 4
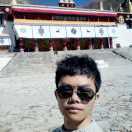
andy
关注
还未添加个人签名 2019-11-21 加入
还未添加个人简介
评论