代码随想录 Day17 - 二叉树(四)
作者:jjn0703
- 2023-07-16 江苏
本文字数:1958 字
阅读完需:约 6 分钟
作业题
110. 平衡二叉树
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode() {}
* TreeNode(int val) { this.val = val; }
* TreeNode(int val, TreeNode left, TreeNode right) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
class Solution {
public boolean isBalanced(TreeNode root) {
if (root == null) {
return true;
}
int leftDepth = getDepth(root.left);
int rightDepth = getDepth(root.right);
if(Math.abs(leftDepth - rightDepth) > 1) {
return false;
}
return isBalanced(root.left) && isBalanced(root.right);
}
private int getDepth(TreeNode node) {
if (node == null) {
return 0;
}
int left = getDepth(node.left);
int right = getDepth(node.right);
return Math.max(left, right) + 1;
}
}
复制代码
257. 二叉树的所有路径
回溯法,递归遇到叶子节点(左右子树均为 null)则退回,遇到符合条件的,则构造字符串结果并且追加到字符串中。
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode() {}
* TreeNode(int val) { this.val = val; }
* TreeNode(int val, TreeNode left, TreeNode right) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
class Solution {
public List<String> binaryTreePaths(TreeNode root) {
List<String> result = new ArrayList<>();
buildPath(root, new ArrayList<>(), result);
return result;
}
private void buildPath(TreeNode node, List<Integer> res, List<String> result) {
res.add(node.val);
if (node.left == null && node.right == null) {
StringBuilder stringBuilder = new StringBuilder();
for (int i = 0; i < res.size(); i++) {
Integer path = res.get(i);
stringBuilder.append(path);
if (i < res.size() -1 ) {
stringBuilder.append("->");
}
}
result.add(stringBuilder.toString());
return;
}
if (node.left != null) {
buildPath(node.left, res, result);
res.remove(res.size() -1);
}
if (node.right != null) {
buildPath(node.right, res, result);
res.remove(res.size() -1);
}
}
}
复制代码
404. 左叶子之和
主要在于判断节点为左叶子节点,该类节点的特征为:是叶子节点,且是某个节点的左子节点。
package jjn.carl.binary_tree;
import commons.BinaryTreeHelper;
import commons.TreeNode;
import java.util.ArrayList;
import java.util.List;
/**
* @author Jiang Jining
* @since 2023-07-15 23:59
*/
public class LeetCode404 {
public int sumOfLeftLeaves(TreeNode root) {
if (root == null) {
return 0;
}
return dfs(root);
}
private int dfs(TreeNode node) {
int ans = 0;
if (node.left != null) {
ans += isLeafNode(node.left) ? node.left.val : dfs(node.left);
}
if (node.right != null && !isLeafNode(node.right)) {
ans += dfs(node.right);
}
return ans;
}
private boolean isLeafNode(TreeNode node) {
return node != null && node.left == null && node.right == null;
}
public static void main(String[] args) {
// [3,9,20,null,null,15,7]
List<Integer> input = new ArrayList<>();
input.addAll(List.of(3, 9, 20));
input.add(null);
input.add(null);
input.addAll(List.of(15, 7));
TreeNode root = BinaryTreeHelper.buildTree(input);
int sumOfLeftLeaves = new LeetCode404().sumOfLeftLeaves(root);
System.out.println("sumOfLeftLeaves = " + sumOfLeftLeaves);
}
}
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 3
版权声明: 本文为 InfoQ 作者【jjn0703】的原创文章。
原文链接:【http://xie.infoq.cn/article/7183c5acb3d4a5eb63b855be7】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
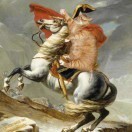
jjn0703
关注
Java工程师/终身学习者 2018-03-26 加入
USTC硕士/健身健美爱好者/Java工程师.
评论