Java 中让两个线程交替执行的实现方式
作者:Janwee
- 2023-03-14 四川
本文字数:1950 字
阅读完需:约 6 分钟
Question: There're 2 threads, one prints 'A', 'B' and 'C', the other prints '1', '2' and '3', how to make them print "1A2B3C"?
问题:有两个线程,一个打印'A'、 'B' 、 'C', 另一个打印 '1'、 '2' 、 '3',怎样让他们打印"1A2B3C"?
使用 synchronized
关键字和 wait
, notify/notifyAll
方法
class Solution1 {
private final Object lock = new Object();
public static void main(String[] args) {
new Solution1().print();
}
private void print() {
new Thread(() -> {
int i = 0;
while (true) {
synchronized (lock) {
try {
System.out.print(i + 1);
lock.notify();
if (i++ == 2) return;
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
new Thread(() -> {
int i = 0;
while (true) {
synchronized (lock) {
try {
System.out.print((char) ('A' + i));
lock.notify();
if (i++ == 2) return;
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
}
}
复制代码
使用 Lock
和 Condition
class Solution2 {
private Lock lock = new ReentrantLock();
private Condition c1 = lock.newCondition();
private Condition c2 = lock.newCondition();
public static void main(String[] args) {
new Solution2().print();
}
private void print() {
new Thread(() -> {
int i = 0;
while (true) {
lock.lock();
try {
System.out.print(i + 1);
c2.signal();
if (i++ == 2) return;
c1.await();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}).start();
new Thread(() -> {
int i = 0;
while (true) {
try {
if (i == 0) {
TimeUnit.MILLISECONDS.sleep(10);
}
lock.lock();
System.out.print((char) ('A' + i));
c1.signal();
if (i++ == 2) return;
c2.await();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}).start();
}
}
复制代码
使用 Semaphore
class Solution3 {
private Semaphore s1 = new Semaphore(1);
private Semaphore s2 = new Semaphore(0);
public static void main(String[] args) {
new Solution3().print();
}
private void print() {
new Thread(() -> {
int i = 0;
while (true) {
try {
s1.acquire();
System.out.print(i + 1);
s2.release();
if (i++ == 2) return;
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}).start();
new Thread(() -> {
int i = 0;
while (true) {
try {
s2.acquire();
System.out.print((char) ('A' + i));
s1.release();
if (i++ == 2) return;
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}).start();
}
}
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 3
版权声明: 本文为 InfoQ 作者【Janwee】的原创文章。
原文链接:【http://xie.infoq.cn/article/5dc5c7648ab223ed3a45d06bb】。文章转载请联系作者。
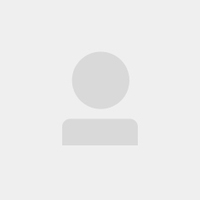
Janwee
关注
Life isn't a problem to solve. 2020-07-26 加入
软件工程师,高质量编码实践者。
评论