代码随想录 Day53 - 动态规划(十四)
作者:jjn0703
- 2023-08-26 江苏
本文字数:1656 字
阅读完需:约 5 分钟
作业题
1143.最长公共子序列
链接:https://leetcode.cn/problems/longest-common-subsequence/description/
package jjn.carl.dp;
import java.util.Scanner;
/**
* @author Jjn
* @since 2023/8/26 09:28
*/
public class LeetCode1143 {
public int longestCommonSubsequence(String text1, String text2) {
int[][] dp = new int[text1.length() + 1][text2.length() + 1];
for (int i = 1; i <= text1.length(); i++) {
for (int j = 1; j <= text2.length(); j++) {
if (text1.charAt(i - 1) == text2.charAt(j - 1)) {
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
return dp[text1.length()][text2.length()];
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String text1 = scanner.next();
String text2 = scanner.next();
int i = new LeetCode1143().longestCommonSubsequence(text1, text2);
System.out.println(i);
}
}
复制代码
1035.不相交的线
链接:https://leetcode.cn/problems/uncrossed-lines/
package jjn.carl.dp;
import java.util.Scanner;
/**
* @author Jjn
* @since 2023/8/26 10:00
*/
public class LeetCode1035 {
public int maxUncrossedLines(int[] nums1, int[] nums2) {
int m = nums1.length;
int n = nums2.length;
int[][] dp = new int[m + 1][n + 1];
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (nums1[i - 1] == nums2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
return dp[m][n];
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int m = scanner.nextInt();
int n = scanner.nextInt();
int[] nums1 = new int[m];
int[] nums2 = new int[n];
for (int i = 0; i < m; i++) {
nums1[i] = scanner.nextInt();
}
for (int i = 0; i < n; i++) {
nums2[i] = scanner.nextInt();
}
int maxUncrossedLines = new LeetCode1035().maxUncrossedLines(nums1, nums2);
System.out.println(maxUncrossedLines);
}
}
复制代码
53.最大子序和
链接:https://leetcode.cn/problems/maximum-subarray/
package jjn.carl.dp;
import java.util.Scanner;
/**
* @author Jjn
* @since 2023/8/26 10:18
*/
public class LeetCode53 {
public int maxSubArray(int[] nums) {
if (nums.length == 0) {
return 0;
}
int[] dp = new int[nums.length + 1];
int result = nums[0];
dp[0] = nums[0];
for (int i = 1; i < nums.length; i++) {
dp[i] = Math.max(dp[i - 1] + nums[i], nums[i]);
result = Math.max(result, dp[i]);
}
return result;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int total = scanner.nextInt();
int[] nums = new int[total];
for (int i = 0; i < total; i++) {
nums[i] = scanner.nextInt();
}
int maxSubArray = new LeetCode53().maxSubArray(nums);
System.out.println(maxSubArray);
}
}
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 3
版权声明: 本文为 InfoQ 作者【jjn0703】的原创文章。
原文链接:【http://xie.infoq.cn/article/52c1b603bd1b7d474faaae813】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
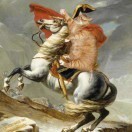
jjn0703
关注
Java工程师/终身学习者 2018-03-26 加入
USTC硕士/健身健美爱好者/Java工程师.
评论