手撕代码系列 (四)
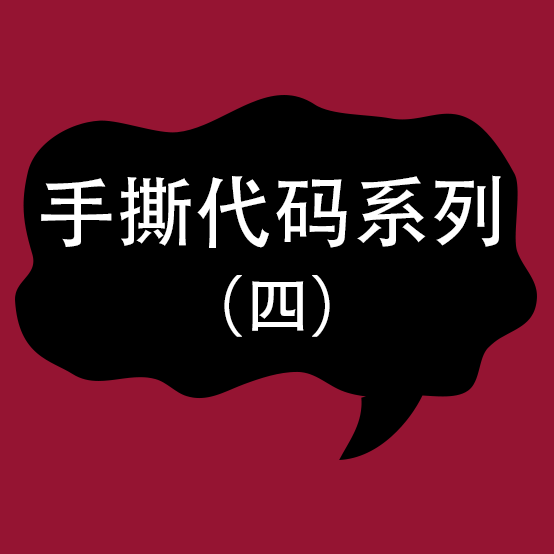
前言
系列首发于公众号『前端进阶圈』 ,若不想错过更多精彩内容,请“星标”一下,敬请关注公众号最新消息。
手撕代码系列(四)
手写触发控制器 Scheduler
当资源不足时将任务加入队列,当资源足够时,将等待队列中的任务取出执行
任务调度器-控制任务的执行,当资源不足时将任务加入等待队列,当资源足够时,将等待队列中的任务取出执行
在调度器中一般会有一个等待队列
queue
,存放当资源不够时等待执行的任务。具有并发数据限制,假设通过
max
设置允许同时运行的任务,还需要count
表示当前正在执行的任务数量。当需要执行一个任务 A 时,先判断
count==max
如果相等说明任务 A 不能执行,应该被阻塞,阻塞的任务放进queue
中,等待任务调度器管理。如果
count< max
说明正在执行的任务数没有达到最大容量,那么count++
执行任务 A,执行完毕后count--
。此时如果
queue
中有值,说明之前有任务因为并发数量限制而被阻塞,现在count < max
,任务调度器会将对头的任务弹出执行。
复制代码
统计页面中前三个标签出现的个数
复制代码
实现菲波那切数列 factorial
复制代码
lru-缓存
lru 是近期最少使用的缓存对象,核心是想要淘汰掉近期使用较少的对象
复制代码
使用 setInterVal 实现 setTimeout
复制代码
使用 setTimeout 实现 setInterVal
复制代码
特殊字符描述:
问题标注
Q:(question)
答案标注
R:(result)
注意事项标准:
A:(attention matters)
详情描述标注:
D:(detail info)
总结标注:
S:(summary)
分析标注:
Ana:(analysis)
提示标注:
T:(tips)
往期推荐:
最后:
版权声明: 本文为 InfoQ 作者【控心つcrazy】的原创文章。
原文链接:【http://xie.infoq.cn/article/4a24818106f70cb07ff310623】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
评论