代码随想录 Day10 - 栈与队列(上)
作者:jjn0703
- 2023-07-07 江苏
本文字数:1306 字
阅读完需:约 4 分钟
相关概念
栈
先进后出,主要 API:push(), pop(), Java 中的主要实现类 Stack()
队列
先进先出,主要 API:offer(), poll(), peek(),Java 中的主要实现类 Queue 接口,如 LinkedList
作业题
232. 用栈实现队列
package jjn.carl.stack_queue;
import java.util.Stack;
/**
* @author Jjn
* @since 2023/7/7 22:50
*/
public class MyQueue {
private final Stack<Integer> first;
private final Stack<Integer> second;
public MyQueue() {
this.first = new Stack<>();
this.second = new Stack<>();
}
public void push(int x) {
first.push(x);
}
public int pop() {
if (second.isEmpty()) {
while (!first.isEmpty()) {
second.push(first.pop());
}
}
return second.pop();
}
public int peek() {
if (second.isEmpty()) {
while (!first.isEmpty()) {
second.push(first.pop());
}
}
return second.peek();
}
public boolean empty() {
return first.isEmpty() && second.isEmpty();
}
public static void main(String[] args) {
MyQueue myQueue = new MyQueue();
myQueue.push(1);
myQueue.push(2);
System.out.println("myQueue.peek() = " + myQueue.peek());
System.out.println("myQueue.pop() = " + myQueue.pop());
System.out.println("myQueue.empty() = " + myQueue.empty());
}
}
复制代码
225. 用队列实现栈
package jjn.carl.stack_queue;
import java.util.LinkedList;
import java.util.Queue;
/**
* @author Jjn
* @since 2023/7/7 23:03
*/
public class MyStack {
private final Queue<Integer> first;
private final Queue<Integer> second;
public MyStack() {
this.first = new LinkedList<>();
this.second = new LinkedList<>();
}
public void push(int x) {
while (!first.isEmpty()) {
second.offer(first.poll());
}
first.offer(x);
while (!second.isEmpty()) {
first.offer(second.poll());
}
}
public int pop() {
return first.peek() == null ? -1 : first.poll();
}
public int top() {
return first.peek() == null ? -1 : first.peek();
}
public boolean empty() {
return first.isEmpty();
}
public static void main(String[] args) {
MyStack myStack = new MyStack();
myStack.push(1);
myStack.push(2);
System.out.println("myStack.top() = " + myStack.top());
System.out.println("myStack.pop() = " + myStack.pop());
System.out.println("myStack.empty() = " + myStack.empty());
}
}
复制代码
划线
评论
复制
发布于: 3 小时前阅读数: 8
版权声明: 本文为 InfoQ 作者【jjn0703】的原创文章。
原文链接:【http://xie.infoq.cn/article/42f68ab12c60d30719a321eff】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
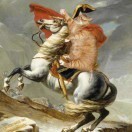
jjn0703
关注
Java工程师/终身学习者 2018-03-26 加入
USTC硕士/健身健美爱好者/Java工程师.
评论