1
Spring Boot 或 Spring Cloud 快速实现文件上传
作者:做梦都在改BUG
- 2023-03-10 湖南
本文字数:3369 字
阅读完需:约 11 分钟
Spring Boot 或 Spring Cloud 快速实现文件上传
很多时候我们都需要在 Spring Boot 或 Spring Cloud 中快速集成文件上传功能,但是对于新手来说增加文件上传功能需要查阅很多文档。这里给出了示例可以帮助您快速将文件上传功能集成到系统中来。
第一步,我们需要在 application.yml 中配置文件上传的大小
spring:
servlet:
multipart:
max-file-size: 1500MB
max-request-size: 1500MB
复制代码
第二步,为了能快速处理文件名和 URL,我们要用到 FilenameUtils,在 pom.xml 的 dependencies 中引入 Apache Commons IO,注意是否已经有引用,避免版本冲突
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
复制代码
第三步,写一个 Controller,处理文件上传的请求
import org.apache.commons.io.FilenameUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import java.io.File;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.UUID;
/**
* 文件上传控制器
*
* @author 杨若瑜
*/
@RestController
@RequestMapping("/platform/")
public class UploadFileController {
// 相对于项目根路径的上传路径
private static final String UPLOAD_FOLDER = "/upload/";
// 返回给前端的服务器根路径(分布式、CDN场景很有用)
private static final String URL_SERVER = "http://127.0.0.1:8080/";
// 允许上传的文件扩展名
private static final String[] ALLOW_EXTENSIONS = new String[]{
// 图片
"jpg", "jpeg", "png", "gif", "bmp",
// 压缩包
"zip", "rar", "gz", "7z", "cab",
// 音视频,
"wav", "avi", "mp4", "mp3", "m3u8", "ogg", "wma", "wmv", "rm", "rmvb", "aac", "mov", "asf", "flv",
// 文档
"doc", "docx", "xls", "xlsx", "ppt", "pptx", "pot", "txt", "csv", "md", "pdf"
};
/**
* 判断文件名是否允许上传
* @param fileName 文件名
* @return
*/
public boolean isAllow(String fileName) {
String ext = FilenameUtils.getExtension(fileName).toLowerCase();
for (String allowExtension : ALLOW_EXTENSIONS) {
if (allowExtension.toLowerCase().equals(ext)) {
return true;
}
}
return false;
}
/**
* 上传文件
* @param request 请求
* @param file 文件,与前台提交表单的file相一致
* @return 返回JSON
*/
@RequestMapping("upload")
public Object upload(HttpServletRequest request, @RequestParam("file") MultipartFile file) {
String webAppFolder = request.getServletContext().getRealPath("/");
String fileName = file.getOriginalFilename();
if (isAllow(fileName)) {
String ext = FilenameUtils.getExtension(fileName).toLowerCase();
String newFileName = UUID.randomUUID().toString().replace("-", "");
// 自动创建上传目录
String targetPath = FilenameUtils.normalize(webAppFolder + "/" + UPLOAD_FOLDER);
String targetFile = FilenameUtils.normalize(targetPath + "/" + newFileName + "." + ext);
new File(targetPath).mkdirs();
try {
String urlPath = URL_SERVER + "/" + UPLOAD_FOLDER + "/" + newFileName + "." + ext;
file.transferTo(new File(targetFile));
Map<String, Object> resJson = new LinkedHashMap<>();
resJson.put("status", "success");
resJson.put("data", FilenameUtils.normalize(urlPath,true).replace("http:/","http://").replace("https:/","https://"));
return resJson;
} catch (Exception e) {
e.printStackTrace();
Map<String, Object> resJson = new LinkedHashMap<>();
resJson.put("status", "error");
resJson.put("message", "文件上传失败:" + e.getMessage());
return resJson;
}
} else {
Map<String, Object> resJson = new LinkedHashMap<>();
resJson.put("status", "error");
resJson.put("message", "该类型文件不允许上传");
return resJson;
}
}
}
复制代码
第四步、写一个测试网页 upload.html,启动并测试一下是否好用。
<!doctype html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>上传文件测试</title>
</head>
<body>
<form action="/platform/upload" method="post" enctype="multipart/form-data">
请选择文件:<input type="file" name="file"><br>
<input type="submit" value="上传">
</form>
</body>
</html>
复制代码
选择一张照片,上传
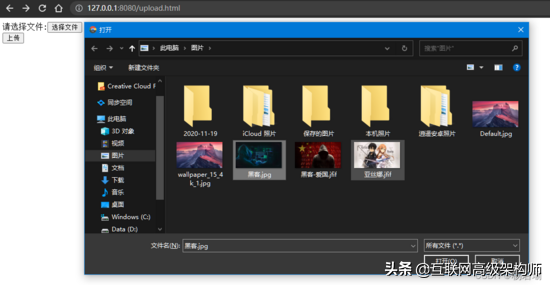
此时后台会返回一个 JSON,我们打开 data 所指向的图片看看是否上传成功:

果然,图片已经上传成功
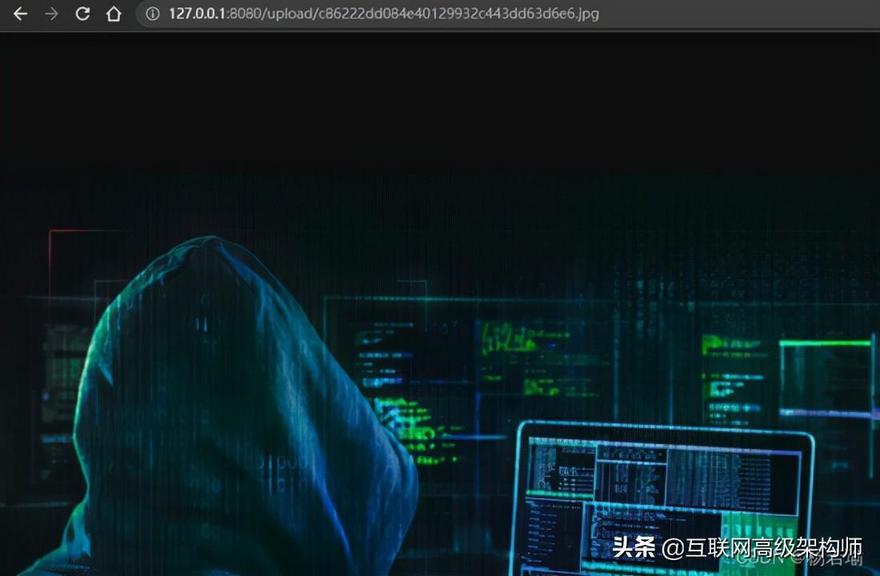
至此,如何使用 Spring Boot 或 Spring Cloud 实现文件上传功能就写到这里。最后需要补充的是,如果你的使用场景使用 ajax 或 App 上传,表单提交类型必须为 multipart/form-data,并且以 post 的方式提交。
这里放上 jQuery 的范例:
// userInfoAvatar是一个input,并且type为file
var file = document.getElementById('userInfoAvatar').files[0];
var formData = new FormData();
formData.append("file",file);
$.ajax({
type: 'POST',
url: '/platform/upload',
data: formData,
contentType: false,
processData: false,
dataType: "json",
mimeType: "multipart/form-data",
success: function(data) {
// 成功时回调
},
error : function(data){
// 失败时回调
}
});
复制代码
放上 axios 范例:
// userInfoAvatar是一个input,并且type为file
let file = document.getElementById('userInfoAvatar').files[0];
let formData = new FormData();
formData.append("file",file);
axios({
method: 'POST',
url: '/platform/upload',
data:formData,
headers: {
'Content-Type': 'multipart/form-data'
}
})
.then((data)=>{
console.log(data)
})
.catch((ex)=>{
console.error(ex)
})
复制代码
放上 Http Client Fluent API 范例:
String fileName = 文件名;
byte[] bytes = 文件的二进制;
MultipartEntityBuilder builder = MultipartEntityBuilder.create()
.setMode(HttpMultipartMode.BROWSER_COMPATIBLE)
.setCharset(Charset.forName("utf-8"));
builder.addBinaryBody("file", bytes, ContentType.MULTIPART_FORM_DATA, fileName);
String responseJson = Request.Post("http://127.0.0.1:8080/platform/upload")
.connectTimeout(20000)
.socketTimeout(20000)
.body(builder.build())
.execute().returnContent().asString();
System.out.println(responseJson);
复制代码
其他的框架如法炮制即可。真正放到正式环境之前记得要加强安全防护,对用户进行鉴权。
作者:杨若瑜
链接:https://blog.csdn.net/yry0304/article/details/121857218
来源平台:CSDN
划线
评论
复制
发布于: 2023-03-10阅读数: 21
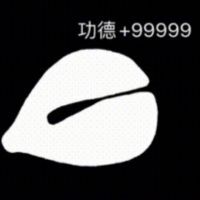
做梦都在改BUG
关注
还未添加个人签名 2021-07-28 加入
公众号:该用户快成仙了
评论