Vue 基础学习(二)
作者:Studying_swz
- 2022-11-08 天津
本文字数:4902 字
阅读完需:约 16 分钟
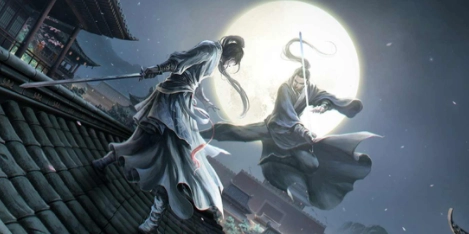
推荐 Vscode 编译器、vue 官网:https://cn.vuejs.org/v2/guide/installation.html
一、vue 的 template
前端代码规范:缩进两个空格打开:文件---首选项---用户片段---新建
{
// Place your 全局 snippets here. Each snippet is defined under a snippet name and has a scope, prefix, body and
// description. Add comma separated ids of the languages where the snippet is applicable in the scope field. If scope
// is left empty or omitted, the snippet gets applied to all languages. The prefix is what is
// used to trigger the snippet and the body will be expanded and inserted. Possible variables are:
// $1, $2 for tab stops, $0 for the final cursor position, and ${1:label}, ${2:another} for placeholders.
// Placeholders with the same ids are connected.
// Example:
// "Print to console": {
// "scope": "javascript,typescript",
// "prefix": "log",
// "body": [
// "console.log('$1');",
// "$2"
// ],
// "description": "Log output to console"
// }
"vue": {
"prefix": "vue",
"body": [
"<!DOCTYPE html>",
"<html lang='en'>",
"<head>",
" <meta charset='UTF-8'>",
" <meta name='viewport' content='width=device-width, initial-scale=1.0'>",
" <title>Document</title>",
"</head>",
"<body>",
"<div id='app'>",
" {{message}}",
"</div>",
"<script src='../js/vue.js'></script>",
"<script>",
" const app = new Vue({",
" el:'#app', //用于挂载要管理的元素",
" data:{ //定义数据",
" message: '你好啊,李银河!',",
" name: 'codewhy'",
" }",
" })",
"</script>",
"</body>",
"</html>",
],
"description": "vue实例对象"
}
}
复制代码
使用:直接输入 prefix 的值,回车即可。
二、Mustache 插值语法
注:大括号插值法{{message}}
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
</head>
<body>
<div id='app'>
<!-- mustache语法,不仅仅可以直接写变量,也可以写简单的表达式 -->
{{firstname + " "+ lastname}}
{{counter * 2}}
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
firstname: 'codewhy',
lastname: 'byy',
counter: 100
}
})
</script>
</body>
</html>
复制代码
三、v-once
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
</head>
<body>
<div id='app'>
<h2>{{message}}</h2>
<h2 v-once>{{message}}</h2>
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
name: 'codewhy'
}
})
</script>
</body>
</html>
复制代码
注:只在第一次渲染,后面不会渲染。
四、v-html
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
</head>
<body>
<div id='app'>
<h2 >{{url}}</h2>
<h2 v-html="url"></h2>
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
url: '<a href="www.baidu.com">百度一下</a>'
}
})
</script>
</body>
</html>
复制代码
注:解析标签
五、v-text
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
</head>
<body>
<div id='app'>
<h2 v-text="message"></h2>
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
name: 'codewhy'
}
})
</script>
</body>
</html>
复制代码
注:不推荐,不灵活,不如 Mustache
六、v-pre
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
</head>
<body>
<div id='app'>
<h2 v-pre>{{message}}</h2>
<h2>{{message}}</h2>
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
name: 'codewhy'
}
})
</script>
</body>
</html>
复制代码
注:原封不动的显示,不解析数据
七、v-cloak
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
<style>
[v-cloak]{
display: none;
}
</style>
</head>
<body>
<div id='app' v-cloak>
{{message}}
</div>
<script src='../js/vue.js'></script>
<script>
//在vue解析之前,div中有一个属性v-cloak
//在vue解析之后,div中没有一个属性v-cloak
setTimeout(function(){
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
name: 'codewhy'
}
})
},1000)
</script>
</body>
</html>
复制代码
注:因为 html 从头到尾渲染,为了避免某些数据来不及渲染,我们可以通过 v-cloak 来设置 style 属性,没有渲染成功之前,显示为空,而不是直接是{{message}}。
八、v-bind 基本使用🚜
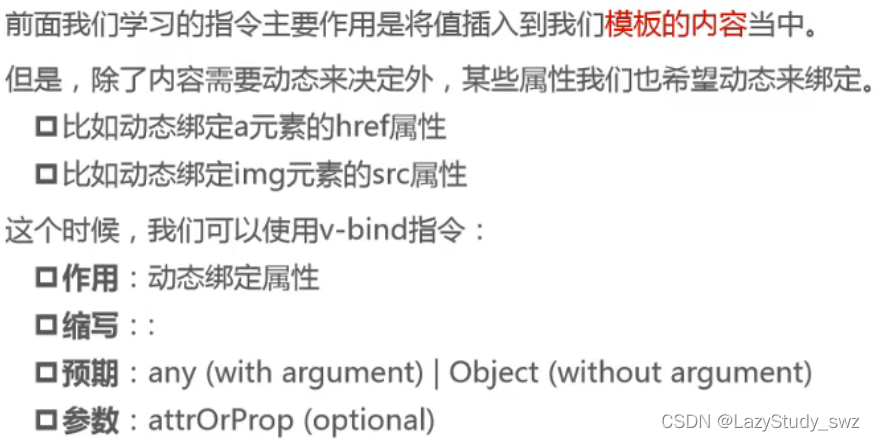
注:前面主要针对模板的内容,属性需要 v-bind
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
</head>
<body>
<div id='app'>
<!-- 这里是错误的,不可以使用mustache语法 -->
<!-- <img src="{{imgurl}}" alt=""> -->
<!-- 正确做法 -->
<img v-bind:src="imgurl" alt="">
<a v-bind:href="aHref" >百度一下</a>
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
name: 'codewhy',
imgurl: "https://pic4.zhimg.com/v2-589b604591675be07573676ddcab2ff4_720w.jpg?source=d6434cab",
aHref: 'https://www.baidu.com'
}
})
</script>
</body>
</html>
复制代码
语法糖:直接写
:
九、v-bind 动态绑定 class 属性(对象语法)🚜
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
<style>
.active{
color:red;
}
</style>
</head>
<body>
<div id='app'>
<!-- 字符串没有意义 -->
<!-- <h2 class="active">{{message}}</h2>
<h2 :class="active">{{message}}</h2> -->
<!-- 对象绑定,有意义 -->
<!-- <h2 :class="{类名1:boolean,类名2:boolean}">{{message}}</h2> -->
<h2 class="title" :class="{active:isActive,line:isLine}">{{message}}</h2>
<button v-on:click="btnClick">按钮</button>
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
isActive: true,
isLine: true,
},
methods:{
btnClick:function(){
this.isActive = !this.isActive
}
}
})
</script>
</body>
</html>
复制代码
简化:当 class 属性太长的时候
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
<style>
.active{
color:red;
}
</style>
</head>
<body>
<div id='app'>
<!-- 字符串没有意义 -->
<!-- <h2 class="active">{{message}}</h2>
<h2 :class="active">{{message}}</h2> -->
<!-- 对象绑定,有意义 -->
<!-- <h2 :class="{类名1:boolean,类名2:boolean}">{{message}}</h2> -->
<h2 class="title" :class="getClasses()">{{message}}</h2>
<button v-on:click="btnClick">按钮</button>
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
isActive: true,
isLine: true,
},
methods:{
btnClick:function(){
this.isActive = !this.isActive
},
getClasses:function(){
return {active:this.isActive,line:this.isLine}
}
}
})
</script>
</body>
</html>
复制代码
十、v-bind 动态绑定 class 属性(数组语法)🚜
意义不大,原始的 class 即可完成,有时候变量可能需要。
十一、作业
注:点击变色
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset='UTF-8'>
<meta name='viewport' content='width=device-width, initial-scale=1.0'>
<title>Document</title>
<style>
.active{
color:red;
}
</style>
</head>
<body>
<div id='app'>
<ul>
<li :class="{active:isActive==index}" v-on:click="colorChoose(index)" v-for="(item,index) in movies">
{{index}}--------- {{item}}
</li>
</ul>
</div>
<script src='../js/vue.js'></script>
<script>
const app = new Vue({
el:'#app', //用于挂载要管理的元素
data:{ //定义数据
message: '你好啊,李银河!',
movies: ['夏洛特烦恼','大话西游','桃姐','人世间'],
isActive: 0
},
methods:{
colorChoose:function(index){
this.isActive = index;
}
}
})
</script>
</body>
</html>
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 6
版权声明: 本文为 InfoQ 作者【Studying_swz】的原创文章。
原文链接:【http://xie.infoq.cn/article/3753a2f9637b2208c39fac16f】。文章转载请联系作者。
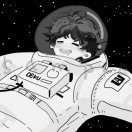
Studying_swz
关注
还未添加个人签名 2020-12-23 加入
还未添加个人简介
评论