设计模式 - 建造者模式
作者:生
- 2023-11-11 上海
本文字数:1642 字
阅读完需:约 5 分钟
//////////////////////////////////
建造者模式
当需要创建复杂对象时,可以使用建造者模式来封装对象的创建过程,使得客户端不需要知道具体的创建细节。
当对象的创建过程中涉及多个步骤或者需要根据不同的条件创建不同的对象时,可以使用建造者模式来组合这些步骤或条件,从而创建不同的对象。
当需要创建的对象具有复杂的内部结构或者需要进行多次创建时,可以使用建造者模式来简化对象的创建过程
/////////////////////////////////
package desginPattern;
import org.springframework.util.StringUtils;
/**
* 建造者模式
*/
public class Builder {
public static void main(String[] args) {
Goods goods = new Goods.Builder()
.setBurdenSheet("生牛乳;维生素;")
.setDescribe("巴氏杀菌乳品")
.setName("鲜奶")
.setType("烘焙乳品")
.setExpirationDate(7)
.build();
System.out.println(goods);
}
}
class Goods {
private String name;
private String type;
private String describe;
private int expirationDate;
private String burdenSheet;
private Goods(Builder builder) {
this.name = builder.name;
this.type = builder.type;
this.describe = builder.describe;
this.expirationDate = builder.expirationDate;
this.burdenSheet = builder.burdenSheet;
}
public String getName() {
return name;
}
public String getType() {
return type;
}
public String getDescribe() {
return describe;
}
public int getExpirationDate() {
return expirationDate;
}
public String getBurdenSheet() {
return burdenSheet;
}
@Override
public String toString() {
return "Goods{" +
"name='" + name + '\'' +
", type='" + type + '\'' +
", describe='" + describe + '\'' +
", expirationDate=" + expirationDate +
", burdenSheet='" + burdenSheet + '\'' +
'}';
}
static class Builder {
private String name;
private String type;
private String describe;
private int expirationDate;
private String burdenSheet;
public Goods build() {
// 校验数据
if (StringUtils.isEmpty(name)) {
throw new RuntimeException("name is empty.");
}
if (StringUtils.isEmpty(type)) {
throw new RuntimeException("type is empty.");
}
if (expirationDate < 1) {
throw new RuntimeException("expirationDate is invalid data.");
}
return new Goods(this);
}
public Builder setName(String name) {
this.name = name;
return this;
}
public Builder setType(String type) {
this.type = type;
return this;
}
public Builder setDescribe(String describe) {
this.describe = describe;
return this;
}
public Builder setExpirationDate(int expirationDate) {
this.expirationDate = expirationDate;
return this;
}
public Builder setBurdenSheet(String burdenSheet) {
this.burdenSheet = burdenSheet;
return this;
}
}
}
复制代码
划线
评论
复制
发布于: 刚刚阅读数: 5
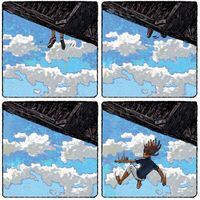
生
关注
还未添加个人签名 2019-08-17 加入
还未添加个人简介
评论