软件测试学习笔记丨 Selenium 常见控件定位方法 (八大定位方式)
作者:测试人
- 2024-01-26 北京
本文字数:2862 字
阅读完需:约 9 分钟
Selenium 常见控件定位方法
通过 name 属性值定位
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
#自定义一个函数
def name_position_method():
#实例化chromedriver对象
driver = webdriver.Chrome()
#打开一个网页
driver.get('http://www.baidu.com')
#强等3秒
time.sleep(3)
#如果没有报错,证明元素找到了
#如果报错no such element,代表元素定位可能出错
#通过元素的name属性值查找“更多”选项,并实现点击操作
driver.find_element(By.NAME, 'tj_briicon').click()
#强等5秒
time.sleep(5)
#if __name__ == '__main__':的作用:限制class_name_position_method()函数只能作为本脚本内的函数直接被调用,而不能被其他脚本文件import调用
if __name__ == '__main__':
name_position_method()
复制代码
通过 id 属性值定位
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
#自定义一个函数
def id_position_method():
#实例化chromedriver对象
driver = webdriver.Chrome()
#打开网站
driver.get('http://www.baidu.com')
#强等3秒
time.sleep(3)
#定位,第一个参数传递定位方式,第二个参数传递定位元素,调用这个方法的返回值为WebElement
#通过元素的id属性值查找“百度一下”按钮,并实现点击操作
driver.find_element(By.ID, 'su').click()
#强等5秒
time.sleep(5)
#if __name__ == '__main__':的作用:限制class_name_position_method()函数只能作为本脚本内的函数直接被调用,而不能被其他脚本文件import调用
if __name__ == '__main__':
id_position_method()
复制代码
通过 css 选择器表达式定位
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
#自定义一个函数
def css_selector_position_method():
#实例化chromedriver对象
driver = webdriver.Chrome()
#打开网站
driver.get('http://www.baidu.com')
#强等3秒
time.sleep(3)
#通过元素的css选择器表达式,查找“文库”选项,并实现点击操作
driver.find_element(By.CSS_SELECTOR, '#s-top-left > a:nth-child(8)').click()
#强等5秒
time.sleep(5)
#if __name__ == '__main__':的作用:限制class_name_position_method()函数只能作为本脚本内的函数直接被调用,而不能被其他脚本文件import调用
if __name__ == '__main__':
css_selector_position_method()
复制代码
通过 xpath 定位
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
#自定义一个函数
def xpath_position_method():
#实例化chromedriver对象
driver = webdriver.Chrome()
#打开网站
driver.get('http://www.baidu.com')
#强等3秒
time.sleep(3)
#通过元素的xpath路径,查找“网盘”选项,并实现点击操作
driver.find_element(By.XPATH, '/html/body/div[2]/div[1]/div[3]/a[7]').click()
#强等5秒
time.sleep(5)
#if __name__ == '__main__':的作用:限制class_name_position_method()函数只能作为本脚本内的函数直接被调用,而不能被其他脚本文件import调用
if __name__ == '__main__':
xpath_position_method()
复制代码
通过 class 类名定位
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
#自定义一个函数
def class_name_position_method():
#实例化chromedriver对象
driver = webdriver.Chrome()
#打开网站
driver.get('http://www.baidu.com')
#强等3秒
time.sleep(3)
#通过元素的类名CLASS_NAME,查找“新闻”选项,并实现点击操作
driver.find_element(By.CLASS_NAME, 'mnav').click()
#强等5秒
time.sleep(5)
#if __name__ == '__main__':的作用:限制class_name_position_method()函数只能作为本脚本内的函数直接被调用,而不能被其他脚本文件import调用
if __name__ == '__main__':
class_name_position_method()
复制代码
通过 link_text 文本链接定位
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
#自定义一个函数
def link_text_position_method():
#实例化chromedriver对象
driver = webdriver.Chrome()
#打开网站
driver.get('http://www.baidu.com')
#强等3秒
time.sleep(3)
#通过文本链接的方式:1.元素一定是a标签 2.输入的元素为标签内的文本
#通过元素的link_text 文本链接,查找“hao123”选项,并实现点击操作
driver.find_element(By.LINK_TEXT, "hao123").click()
#强等5秒
time.sleep(5)
#if __name__ == '__main__':的作用:限制class_name_position_method()函数只能作为本脚本内的函数直接被调用,而不能被其他脚本文件import调用
if __name__ == '__main__':
link_text_position_method()
复制代码
通过 partial_link_text 部分文本链接定位
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
#自定义一个函数
def partial_link_text_position_method():
#实例化chromedriver对象
driver = webdriver.Chrome()
#打开网站
driver.get('http://www.baidu.com')
#强等3秒
time.sleep(3)
#通过文本链接的方式:1.元素一定是a标签 2.输入的元素为标签内的文本
#通过元素的partial link text 部分文本链接,如果多个元素匹配,则只会选择第一个元素,查找“hao123”选项,并实现点击操作
driver.find_element(By.LINK_TEXT, "hao123").click()
#强等5秒
time.sleep(5)
#if __name__ == '__main__':的作用:限制class_name_position_method()函数只能作为本脚本内的函数直接被调用,而不能被其他脚本文件import调用
if __name__ == '__main__':
partial_link_text_position_method()
复制代码
通过 tag_name 元素本身标签名称定位
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
#自定义一个函数
def tag_name_position_method():
#实例化chromedriver对象
driver = webdriver.Chrome()
#打开网站
driver.get('https://cn.bing.com/?mkt=zh-cn')
#强等3秒
time.sleep(3)
#通过元素的本身标签名称,查找“图片”选项,并实现点击操作
driver.find_element(By.TAG_NAME, "a").click()
#强等5秒
time.sleep(5)
#if __name__ == '__main__':的作用:限制class_name_position_method()函数只能作为本脚本内的函数直接被调用,而不能被其他脚本文件import调用
if __name__ == '__main__':
tag_name_position_method()
复制代码
霍格沃兹的测试管理班是专门面向测试与质量管理人员的一门课程,通过提升从业人员的团队管理、项目管理、绩效管理、沟通管理等方面的能力,使测试管理人员可以更好的带领团队、项目以及公司获得更快的成长。提供 1v1 私教指导,BAT 级别的测试管理大咖量身打造职业规划。
划线
评论
复制
发布于: 14 分钟前阅读数: 5
版权声明: 本文为 InfoQ 作者【测试人】的原创文章。
原文链接:【http://xie.infoq.cn/article/278b4714001183c8f613f870d】。文章转载请联系作者。
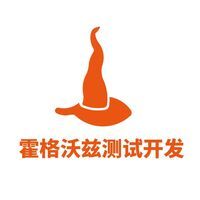
测试人
关注
专注于软件测试开发 2022-08-29 加入
霍格沃兹测试开发学社,测试人社区:https://ceshiren.com/t/topic/22284
评论