常见数据结构与代码实现方案
作者:测吧(北京)科技有限公司
- 2024-07-31 北京
本文字数:2357 字
阅读完需:约 8 分钟
更多软件测试学习资料戳
掌握常见的数据结构及其代码实现方案对于编程和软件开发至关重要。以下是一些常见的数据结构及其在 Python 中的实现示例。
1. 数组(Array)
数组是一种线性数据结构,其中元素按顺序存储,可以通过索引访问。
实现
在 Python 中,数组可以用列表实现:
# 创建数组
arr = [1, 2, 3, 4, 5]
# 访问元素
print(arr[0]) # 输出: 1
# 更新元素
arr[1] = 20
print(arr) # 输出: [1, 20, 3, 4, 5]
# 添加元素
arr.append(6)
print(arr) # 输出: [1, 20, 3, 4, 5, 6]
# 删除元素
arr.pop(2)
print(arr) # 输出: [1, 20, 4, 5, 6]
复制代码
2. 链表(Linked List)
链表是一种线性数据结构,其中每个元素都是一个节点,包含数据和指向下一个节点的指针。
实现
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
last = self.head
while last.next:
last = last.next
last.next = new_node
def print_list(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print(None)
# 使用链表
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
ll.print_list() # 输出: 1 -> 2 -> 3 -> None
复制代码
3. 栈(Stack)
栈是一种线性数据结构,遵循后进先出(LIFO)原则。
实现
class Stack:
def __init__(self):
self.stack = []
def push(self, item):
self.stack.append(item)
def pop(self):
if not self.is_empty():
return self.stack.pop()
def peek(self):
if not self.is_empty():
return self.stack[-1]
def is_empty(self):
return len(self.stack) == 0
def size(self):
return len(self.stack)
# 使用栈
s = Stack()
s.push(1)
s.push(2)
s.push(3)
print(s.pop()) # 输出: 3
print(s.peek()) # 输出: 2
print(s.size()) # 输出: 2
复制代码
4. 队列(Queue)
队列是一种线性数据结构,遵循先进先出(FIFO)原则。
实现
class Queue:
def __init__(self):
self.queue = []
def enqueue(self, item):
self.queue.append(item)
def dequeue(self):
if not self.is_empty():
return self.queue.pop(0)
def is_empty(self):
return len(self.queue) == 0
def size(self):
return len(self.queue)
# 使用队列
q = Queue()
q.enqueue(1)
q.enqueue(2)
q.enqueue(3)
print(q.dequeue()) # 输出: 1
print(q.size()) # 输出: 2
复制代码
5. 哈希表(Hash Table)
哈希表是一种以键值对存储数据的结构,通过哈希函数实现快速查找。
实现
class HashTable:
def __init__(self):
self.table = [None] * 10
def hash_function(self, key):
return hash(key) % len(self.table)
def insert(self, key, value):
index = self.hash_function(key)
if not self.table[index]:
self.table[index] = []
self.table[index].append((key, value))
def get(self, key):
index = self.hash_function(key)
if self.table[index]:
for k, v in self.table[index]:
if k == key:
return v
return None
# 使用哈希表
ht = HashTable()
ht.insert('name', 'Alice')
ht.insert('age', 25)
print(ht.get('name')) # 输出: Alice
print(ht.get('age')) # 输出: 25
复制代码
6. 二叉树(Binary Tree)
二叉树是一种树形数据结构,每个节点最多有两个子节点。
实现
class TreeNode:
def __init__(self, data):
self.data = data
self.left = None
self.right = None
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.data, end=" ")
inorder_traversal(root.right)
# 创建二叉树
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
# 中序遍历
inorder_traversal(root) # 输出: 4 2 5 1 3
复制代码
7. 图(Graph)
图是一种由节点和边组成的数据结构。
实现
class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
def bfs(self, start):
visited = set()
queue = [start]
while queue:
vertex = queue.pop(0)
if vertex not in visited:
print(vertex, end=" ")
visited.add(vertex)
queue.extend([v for v in self.graph.get(vertex, []) if v not in visited])
# 创建图并进行广度优先搜索
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("广度优先搜索(BFS):")
g.bfs(2) # 输出: 2 0 3 1
复制代码
结论
掌握这些常见的数据结构及其实现方案,对于解决实际问题和提升编程技能非常重要。通过理解其基本原理和实现方法,可以更好地选择和优化数据结构,以提高程序的效率和性能。
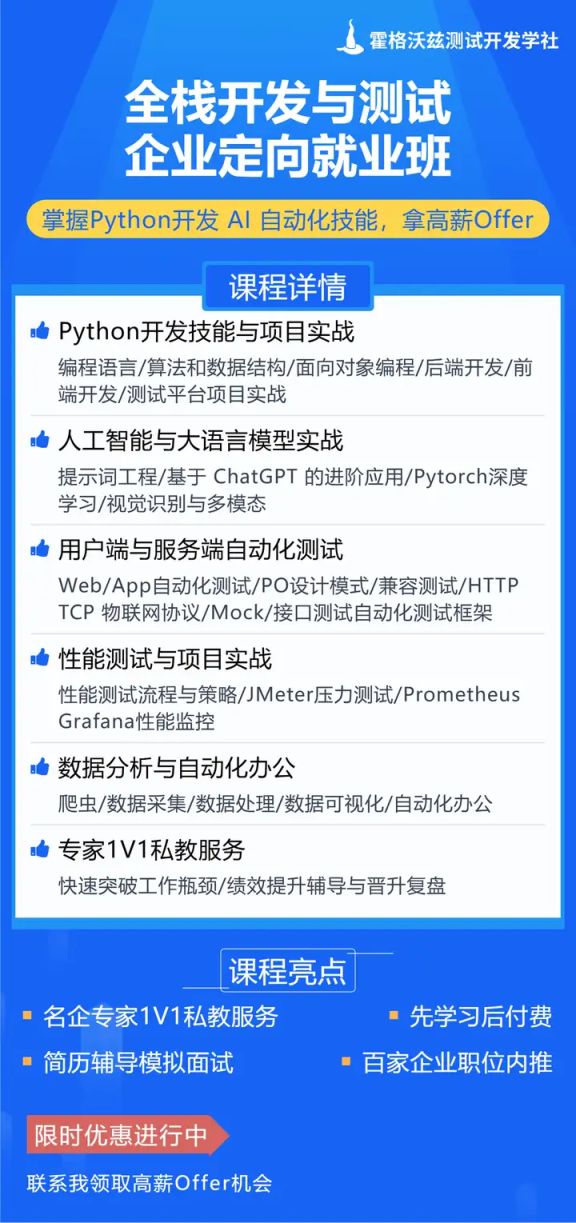
划线
评论
复制
发布于: 刚刚阅读数: 2
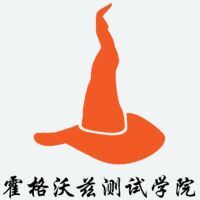
测吧(北京)科技有限公司
关注
社区:ceshiren.com 微信:ceshiren2023 2022-08-29 加入
微信公众号:霍格沃兹测试开发 提供性能测试、自动化测试、测试开发等资料、实事更新一线互联网大厂测试岗位内推需求,共享测试行业动态及资讯,更可零距离接触众多业内大佬
评论