c++ 笔记——类
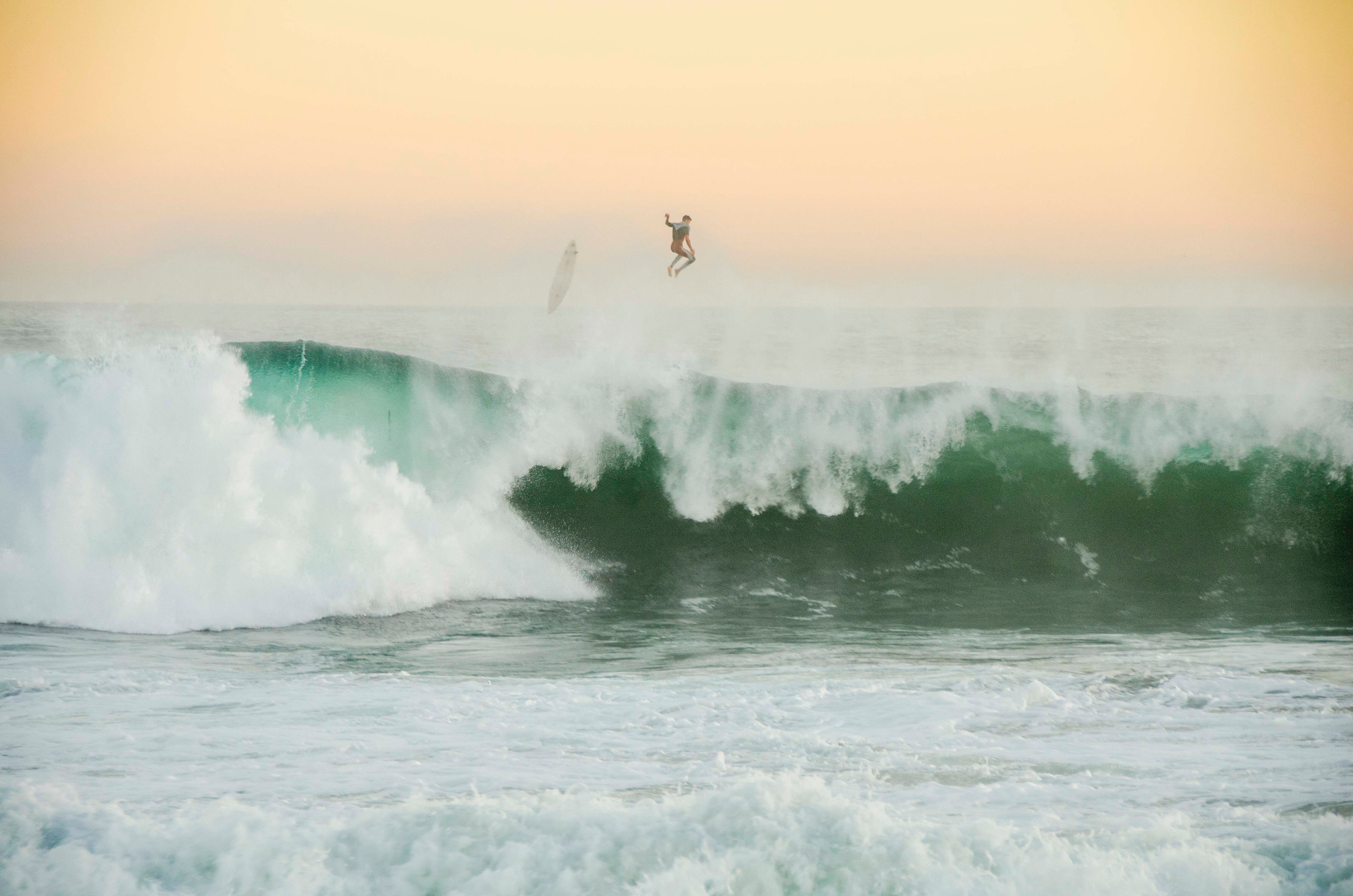
对于这样的定义是放在.h文件里面
对于这样一个类,
如果是在栈中创建的:Human human;
堆中创建的:Human *p = new Human();(是需要在堆上new出一块空间)
1 在栈上操作
对于一个类撰写和定义,可以这么说,应该分为两个部分
一个是关于 .h文件
另一个部分 .cpp文件,是对 .h文件的具体的展示
接着就是主函数的调用
使用g++进行编译
g++ -std=c++11 -g -o class Human.cpp class.cpp
-o是输出,输出的名字是class,使用是c++11的标准库

从析构函数上来看,在函数结束后,进行自动销毁。
2 在堆上操作
在堆上操作,则是定义Human上使用 Human *human = new Human();
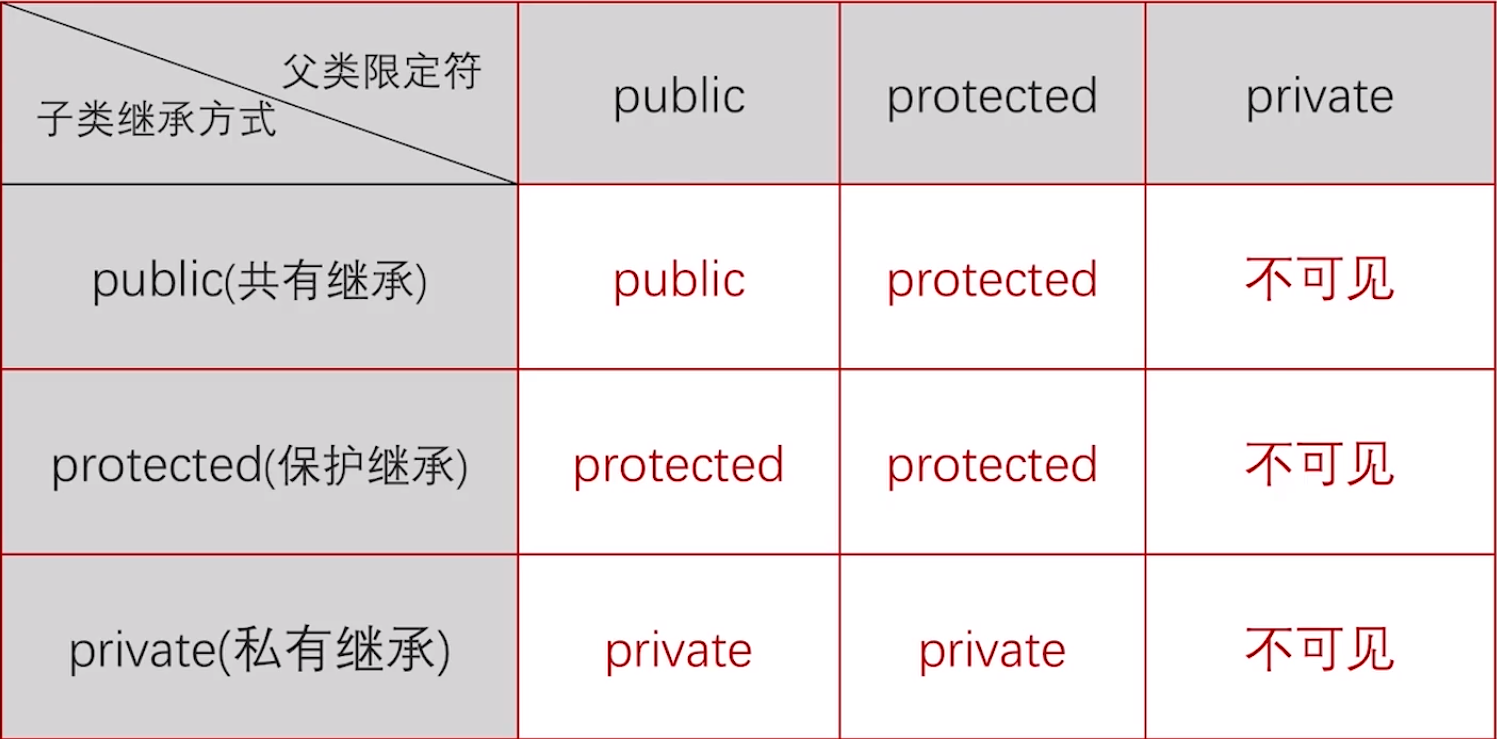
3 补充
堆空间和栈空间
堆空间:从低地址到高地址 new和delete
栈空间:从高地址向低地址存储
两边向中间增加地址块
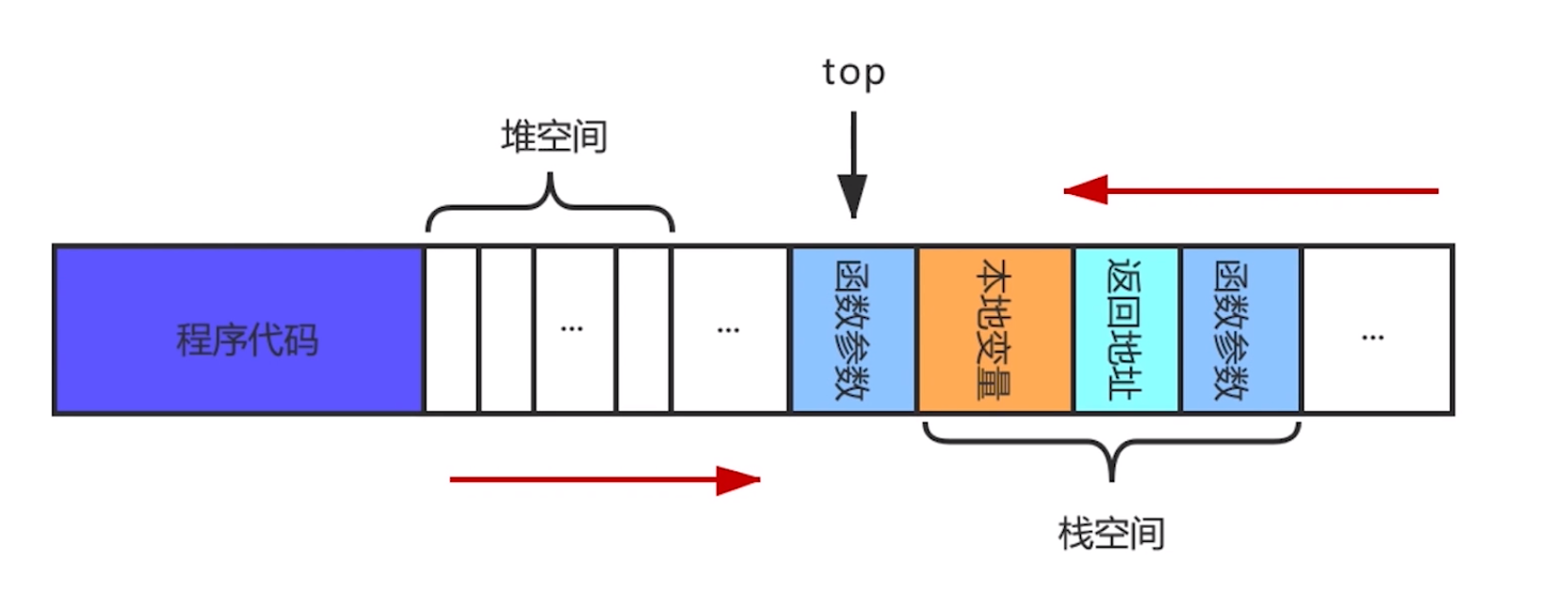
版权声明: 本文为 InfoQ 作者【菜鸟小sailor 🐕】的原创文章。
原文链接:【http://xie.infoq.cn/article/f55b9b417895e12c5abedb56c】。未经作者许可,禁止转载。
评论