使用 TypeScript 从零搭建自己的 Web 框架:领域特定语言 (DSL) 与 Prisma 模型
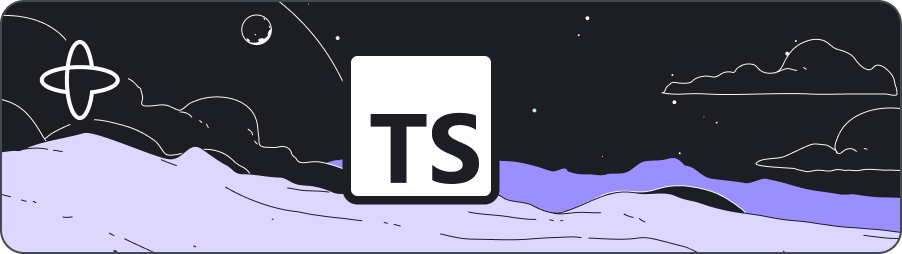
DSL(领域特定语言)是一种针对特定应用领域设计的编程语言或标记语言,旨在提供比通用编程语言更简洁、更直观的语法和工具集,以便开发者能够更高效地完成特定任务。DSL 的出现大大简化了复杂领域的工作流程,提高了开发效率,降低了出错率。
以 Prisma 模型为例,它是一种用于定义和操作数据库模式的 DSL。Prisma 模型提供了一种直观、简洁的方式来描述数据库中的实体、关系以及它们之间的约束。通过定义 Prisma 模型,开发者可以清晰地表达数据库的结构和规则,而无需直接编写繁琐的 SQL 语句或 ORM 配置。
这是一个定义 Prisma Schema 的例子:
创建一个 DSL 的步骤如下:
那么如何为上面的 Prisma 模型创建一个解析器呢?
解析器原理
解析器主要涉及到词法分析和语法分析两个关键步骤。
词法分析(也称为扫描器或词法器)根据语言的词法规则,从左到右扫描源程序的字符,将其分割成一个个 Token,并为每个 Token 附上相应的属性(如 Token 的类型、值等)。
语法分析,这一步使用语法规则集合(通常以文法的形式表示)来分析 Token 序列,确定它们之间的关系和结构。语法分析器会检查 Token 序列是否符合预定义的语法规则,从而验证输入数据是否满足特定的语法要求。
这是一个 TypeScript 实现词法分析器的例子:
nextToken 方法是词法分析器的核心,它不断读取字符并根据词法规则返回相应的记号。当遇到数字时,它会收集所有连续的数字字符并返回一个 INTEGER 类型的记号;当遇到加号或减号时,它会返回相应的记号;当遇到其他字符时,它会抛出一个错误。循环不断调用 nextToken 方法,直到返回 EOF 记号为止。
解析器生成器
手动编写解析器是一个复杂且漫长的过程,在实际开发中一般使用解析器生成器来创建解析器。peg.js 是一种基于 Parsing Expression Grammar(解析表达式语法)的解析器生成器,PEG 类似于正则表达式和巴科斯范式 BNF,我们使用它来生成一个 Prisma 模型解析器。
为了简单起见我们简化模型如下:
定义模型语法
生成解析器
使用解析器
运行
有了 AST(抽象语法树)之后我们就可以根据 AST 生成 SQL 和 DAO 层代码
总结
DSL(领域特定语言)允许我们以更直观、更贴近业务逻辑的方式表达和解决复杂问题。DSL 能够简化复杂系统的构建和维护过程,提高开发效率,同时减少错误和误解。通过 DSL,我们可以创建出针对特定领域的强大工具,从而推动业务创新和提升软件系统的整体质量。
评论