LeetCode 120. Triangle
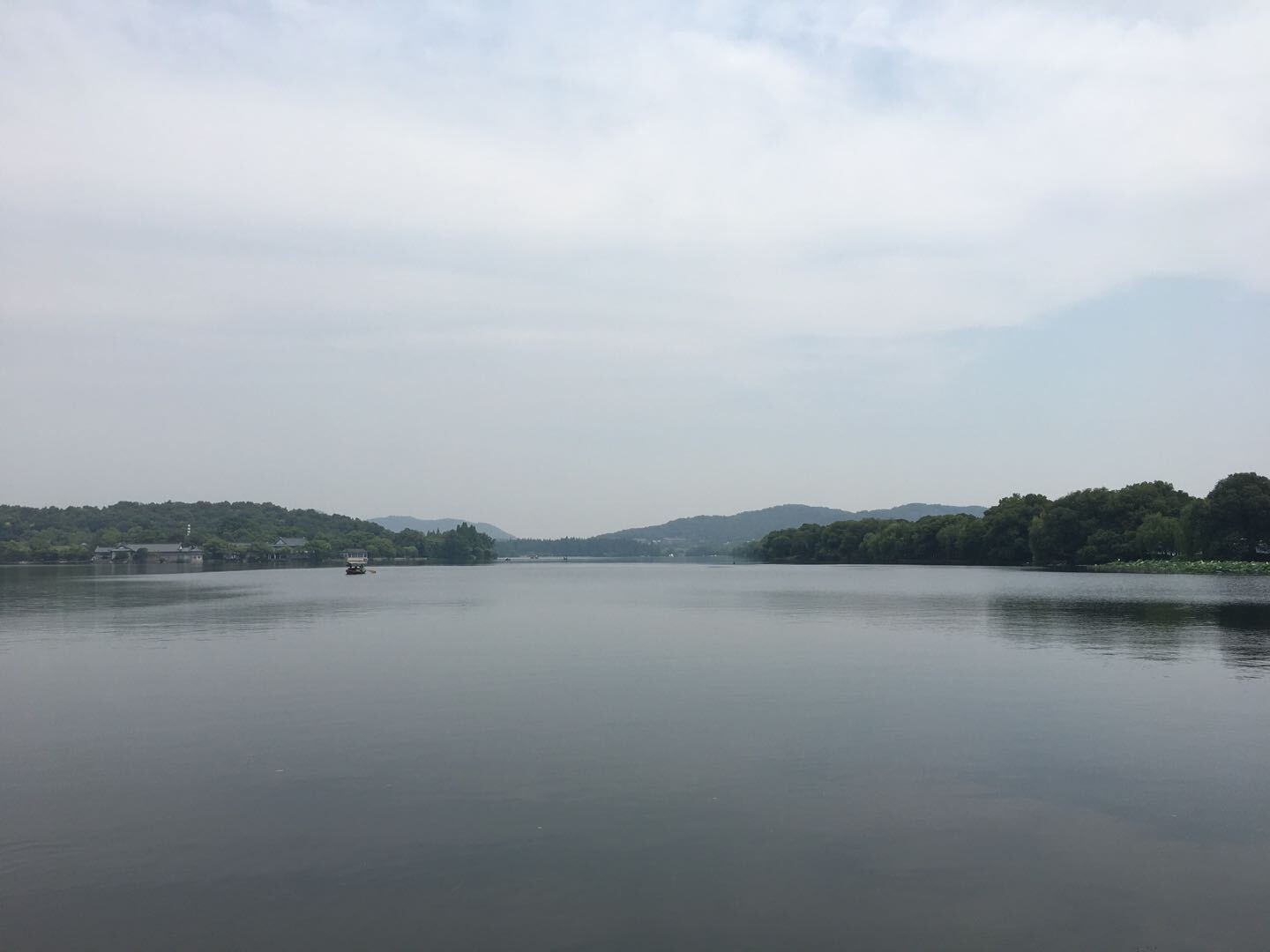
Description
Given a triangle, find the minimum path sum from top to bottom. Each step you may move to adjacent numbers on the row below.
For example, given the following triangle
The minimum path sum from top to bottom is 11 (i.e., 2 + 3 + 5 + 1 = 11).
Note:
Bonus point if you are able to do this using only O(n) extra space, where n is the total number of rows in the triangle.
问题分析
本题为典型的
矩阵路径最优问题
:从起点到终点的路径和最大、最小等;本题中节点 A 的下一个遍历节点可能是:正下方的节点、或右下方的节点;
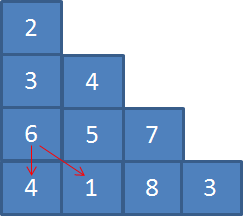
故,尝试使用
动态规划
进行求解:
令DP[i][j]
表示从根节点到节点任意节点A[i][j]
的最小路径和,则有:
进一步地
题目要求尽可能使用
O(N)
的空间复杂度,其中N为三角形的行数,也为最后一行的元素个数。考虑到上述递推公式中,当前行的结果仅与上一行节点有关,因此可将二维DP简化为一维DP;
实现时,为了避免遍历过程中,对上一行结果(正上方、左上方节点)的值进行修改,此处选择从右至左的遍历顺序,完成DP过程。
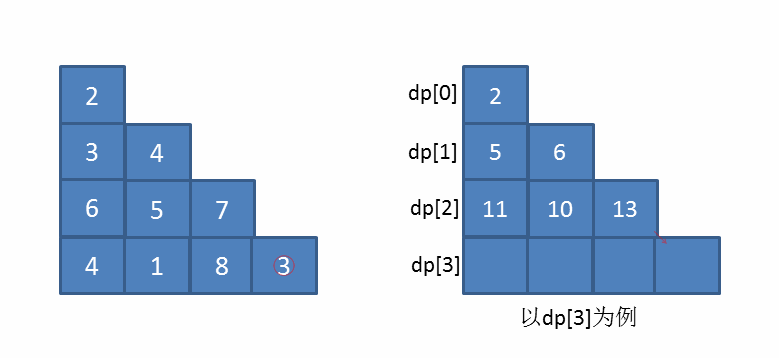
Submission
如有描述不当之处,欢迎留言互动!
版权声明: 本文为 InfoQ 作者【隔壁小王】的原创文章。
原文链接:【http://xie.infoq.cn/article/160550921d1f48e8224225fbc】。文章转载请联系作者。
评论