架构师训练营 Week 03 作业
发布于: 2020 年 06 月 24 日
1 线程安全的单例实现
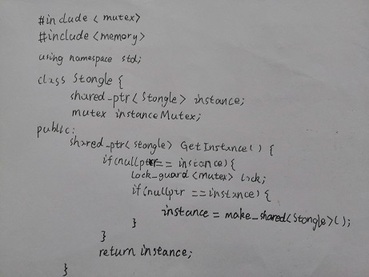
2 用组合设计模式编写程序
用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
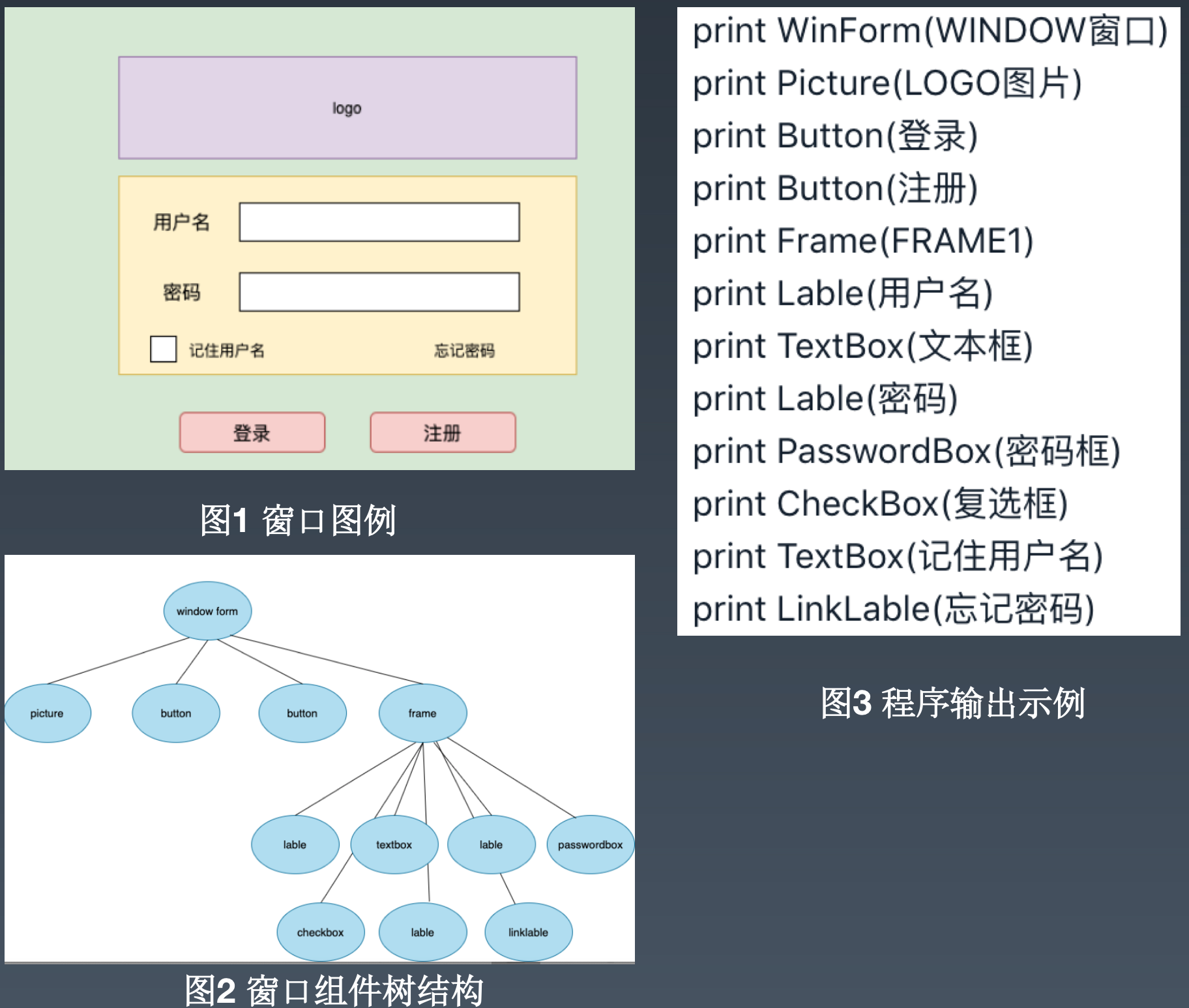
2.1 类图
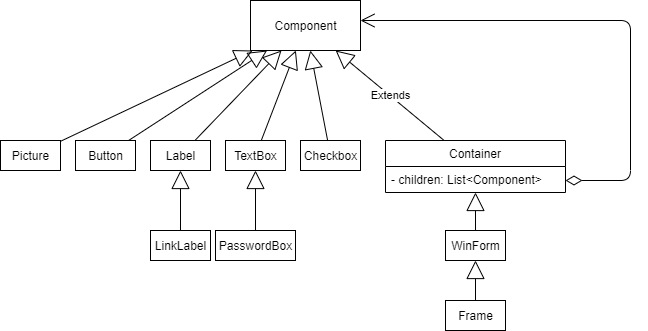
Component类是窗口组件抽象类,包含组件共有的属性;
Container类是组件容器类,负责储存子部件,
2.2 实现
2.2.1 组件接口
Component类:
class Component {protected: // fieldspublic: virtual void print() = 0;};
2.2.2 子控件
2.2.2.1 图片控件
class Picture : public Component {protected: string uri; string name; public: Picture(const string& uri, const string& name) : uri(uri) , name(name) { } void print() override { cout << "print Picture(" << name << ")" << endl; }};
2.2.2.2 按钮控件
class Button : public Component {protected: string text; public: Button(const string& text) : text(text) { } void print() override { cout << "print Button(" << text << ")" << endl; }};
2.2.2.3 标签控件
class Label : public Component {protected: string text; public: Label(const string& text) : text(text) { } void print() override { cout << "print Label(" << text << ")" << endl; }};
2.2.2.4 链接标签
class LinkLabel : public Component {protected: string text; string link; public: LinkLabel(const string& text, const string& link) : text(text) , link(link) { } void print() override { cout << "print LinkLabel(" << text << ")" << endl; }};
2.2.2.5 文本输入框
class TextBox : public Component {protected: string name; string text;public: TextBox(const string& name) : name(name) { } void print() override { cout << "print TextBox(" << name << ")" << endl; }};
2.2.2.6 密码输入框
class PasswordBox : public TextBox {public: PasswordBox(const string& name) : TextBox(name) { } void print() override { cout << "print PasswordBox(" << name << ")" << endl; }};
2.2.2.7 复选框
class CheckBox : public Component {protected: string text;public: CheckBox(const string& text) : text(text) { } void print() override { cout << "print CheckBox(" << text << endl; }};
2.2.3 组件容器
class Container : public Component {protected: list<shared_ptr<Component>> children;public: void add(shared_ptr<Component>& child) { children.push_back(child); } void add(shared_ptr<Component>&& child) { children.push_back(child); } void print() override { for (auto& child : children) { child->print(); } }};
2.2.3.1 窗口
class WinForm : public Container {protected: string title;public: WinForm(const string& title) : title(title) { } void print() override { cout << "print WinForm(" << title << ")" << endl; Container::print(); }};
2.2.3.2 Frame控件
class Frame : public WinForm {public: Frame(const string& title) : WinForm(title) { } void print() override { cout << "print Frame(" << title << ")" << endl; Container::print(); }};
2.2.4 客户端
int main(int argc, char** argv) { shared_ptr<WinForm> client = make_shared<WinForm>("WINDOW窗口"); client->add(make_shared<Picture>("./logo.jpg", "LOGO图片")); client->add(make_shared<Button>("登录")); client->add(make_shared<Button>("注册")); shared_ptr<Frame> frame = make_shared<Frame>("FRAME1"); frame->add(make_shared<Label>("用户名")); frame->add(make_shared<TextBox>("文本框")); frame->add(make_shared<Label>("密码")); frame->add(make_shared<PasswordBox>("密码框")); frame->add(make_shared<CheckBox>("复选框")); frame->add(make_shared<TextBox>("记住用户名")); frame->add(make_shared<LinkLabel>("忘记密码", "http://www.xxx.com")); client->add(frame); client->print(); return 0;}
2.3 运行效果
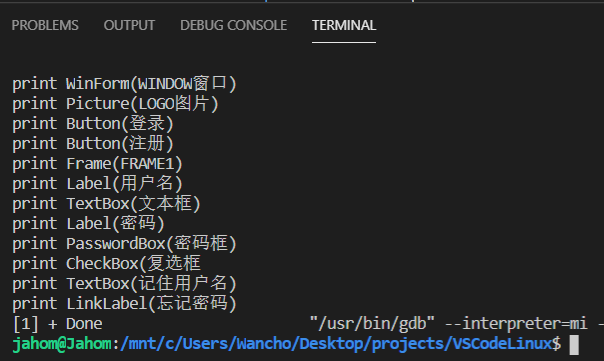
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 53
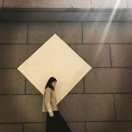
Wancho
关注
还未添加个人签名 2020.02.28 加入
还未添加个人简介
评论