LeetCode 题解:152. 乘积最大子数组,动态规划,JavaScript,详细注释
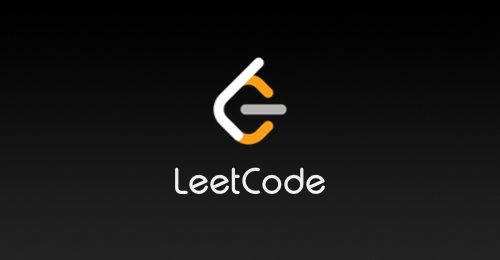
原题链接:https://leetcode-cn.com/problems/maximum-product-subarray/
解题思路:
子数组至少包含一个元素,意味着
nums[i]
必然存在于子数组中。nums[i]
可能与它之前或者之后的元素组成子数组。如果从前往后遍历,子数组元素为nums[i]
到nums[i + j]
的情况,会在遍历到nums[i + j]
的时候一起处理。因此遍历时只需要考虑nums[i]
与其之前元素的组合情况即可。由于
nums
中的数字有正有负,那么nums[i]
之前的子数组成绩也有正有负。但负数的乘积为正,也有可能成为最大值,因此需要保留。那么每个位置的乘积有 3 种情况:
* nums[i]
与之前子数组的乘积较大值,将其保存在数组maxProducts
中。
* nums[i]
与之前子数组的乘积较小值,将其保存在数组minProducts
中。
* nums[i]
不与之前的子数组相乘,它自己作为结果。
位置
i
的最大值,只需要对比maxProducts[i]
和nums[i]
即可,状态转移方程为:max = Math.max(max, maxProducts[i]);
。
复制代码
i
的状态只与i - 1
有关,maxProducts
和minProducts
可以简化为变量。
复制代码
版权声明: 本文为 InfoQ 作者【Lee Chen】的原创文章。
原文链接:【http://xie.infoq.cn/article/fad8c8a38a6c8e5dee20ce6f2】。文章转载请联系作者。
评论