架构师训练营 -week05- 作业
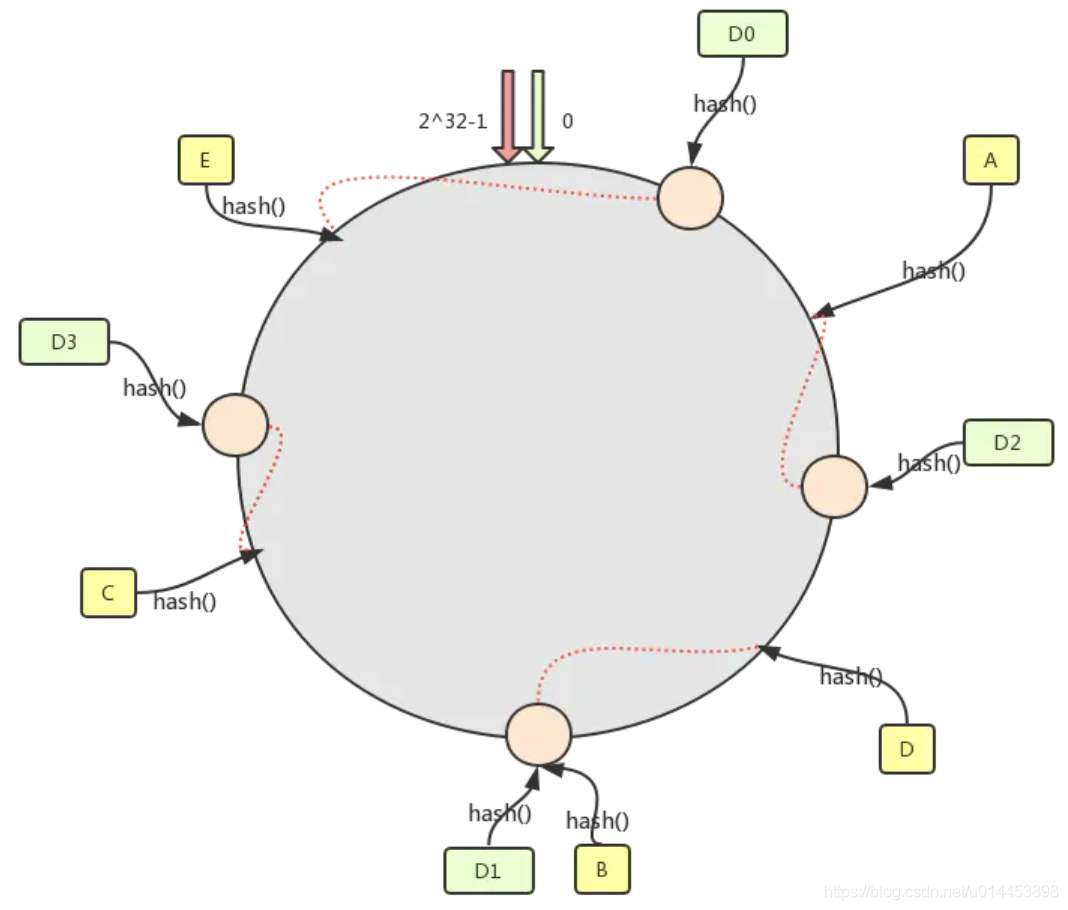
作业:
用你熟悉的编程语言实现一致性 hash 算法。
编写测试用例测试这个算法,测试 100 万 KV 数据,10 个服务器节点的情况下,计算这些 KV 数据在服务器上分布数量的标准差,以评估算法的存储负载不均衡性。
参考了一些网上的实现方案,主要思路和代码如下:
基本思路:
造一个hash环
在哈希环上映射服务器节点
增加虚拟节点,分布在hash环上
路由找到对应的节点
主要代码:
计算标准差:
初始化了10个Node(ip地址+端口),当虚拟节点(VirtualNode)值为1的时候,计算的结果偏差比较大,当VirtualNode=10的时候,就变得较为均衡了。
VirtualNode = 1
{10.10.9.210-8080=995, 10.10.9.210-8081=7378, 10.8.1.11-8080=15805, 10.8.1.11-8081=62, 10.8.1.11-8082=3894, 10.8.3.99-8080=31893, 10.8.3.99-8081=18163, 10.8.3.99-8082=17097, 10.9.11.105-8080=3042, 10.9.11.105-8081=1671}
VirtualNode = 10
{10.10.9.210-8080=10545, 10.10.9.210-8081=9479, 10.8.1.11-8080=6283, 10.8.1.11-8081=9143, 10.8.1.11-8082=11848, 10.8.3.99-8080=9668, 10.8.3.99-8081=10147, 10.8.3.99-8082=10693, 10.9.11.105-8080=15840, 10.9.11.105-8081=6354}
VirtualNode = 100
{10.10.9.210-8080=10077, 10.10.9.210-8081=10599, 10.8.1.11-8080=11064, 10.8.1.11-8081=9359, 10.8.1.11-8082=9086, 10.8.3.99-8080=9936, 10.8.3.99-8081=9750, 10.8.3.99-8082=11025, 10.9.11.105-8080=8476, 10.9.11.105-8081=10628}
主要代码:
代码地址:
https://github.com/liuqi1024/geektime-arch-train/tree/main/week05
评论